XMLHttpRequest
或fetch
API来访问SharePoint列表数据。需要获取SharePoint列表的REST API URL,然后使用这些API发送HTTP请求并处理响应。在JavaScript中访问SharePoint列表数据,通常需要使用SharePoint的REST API或者Graph API,这些API允许我们通过HTTP请求来获取、添加、更新或删除列表数据,下面是一个使用REST API访问SharePoint列表数据的示例。
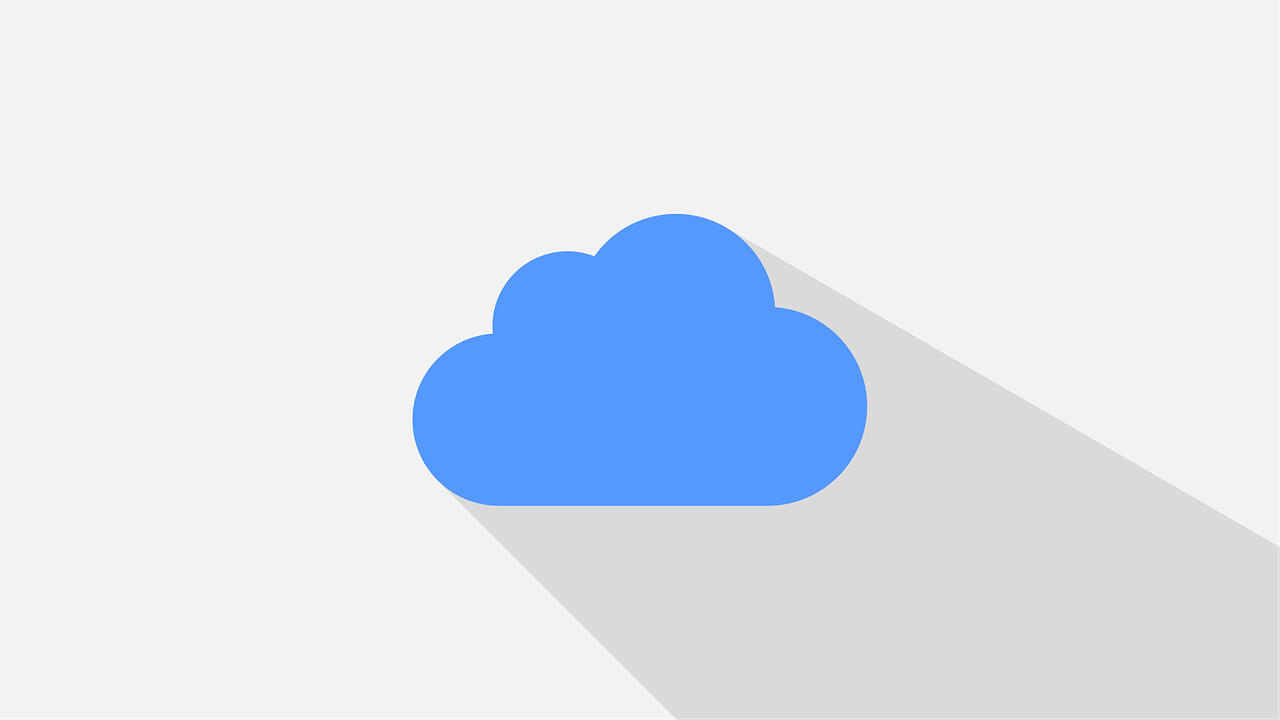
准备工作
确保你的SharePoint网站已经启用了“使用脚本远程访问”的功能,你可以在SharePoint的设置中找到这个选项。
步骤1:获取访问令牌
你需要一个访问令牌来访问SharePoint的REST API,这通常通过OAuth 2.0协议来实现,你可以使用Azure AD或者其他身份提供商来获取访问令牌。
// 使用fetch函数发送POST请求到token endpoint fetch('https://login.microsoftonline.com/<tenant_id>/oauth2/v2.0/token', { method: 'POST', headers: { 'ContentType': 'application/xwwwformurlencoded' }, body: 'grant_type=client_credentials&client_id=<client_id>&client_secret=<client_secret>&scope=https://graph.microsoft.com/.default' }).then(response => response.json()).then(data => { const accessToken = data.access_token; // 使用访问令牌调用SharePoint REST API });
步骤2:调用SharePoint REST API
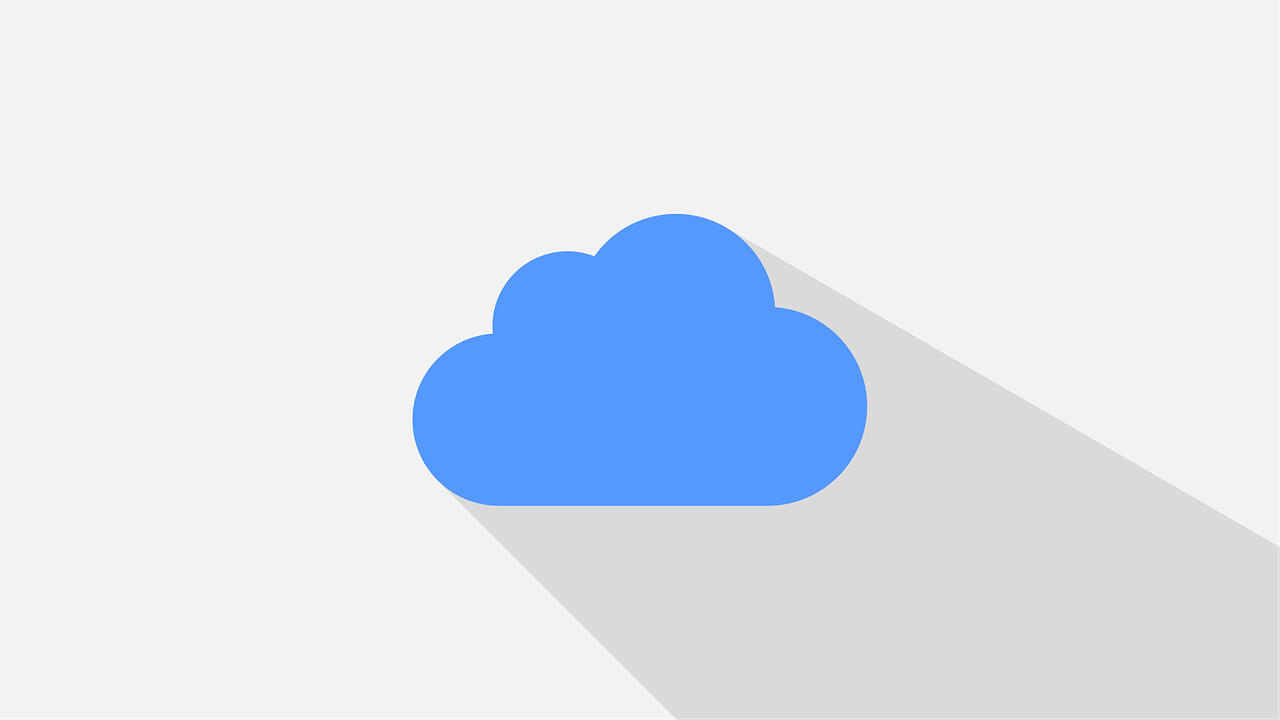
一旦你有了访问令牌,你就可以使用它来调用SharePoint的REST API,你可以获取一个列表的所有项目。
const listName = '<list_name>';
const url =https://<site_url>/_api/web/lists/getbytitle('${listName}')/items
;
fetch(url, {
method: 'GET',
headers: {
'Authorization': 'Bearer ' + accessToken,
'Accept': 'application/json;odata=verbose'
}
}).then(response => response.json()).then(data => {
console.log(data);
});
步骤3:处理响应
REST API返回的数据是JSON格式的,你可以像处理任何其他JSON数据一样处理这些数据。
data.d.results.forEach(item => { console.log(item.Title); });
相关问题与解答
Q1: 我可以在客户端JavaScript代码中直接使用访问令牌吗?
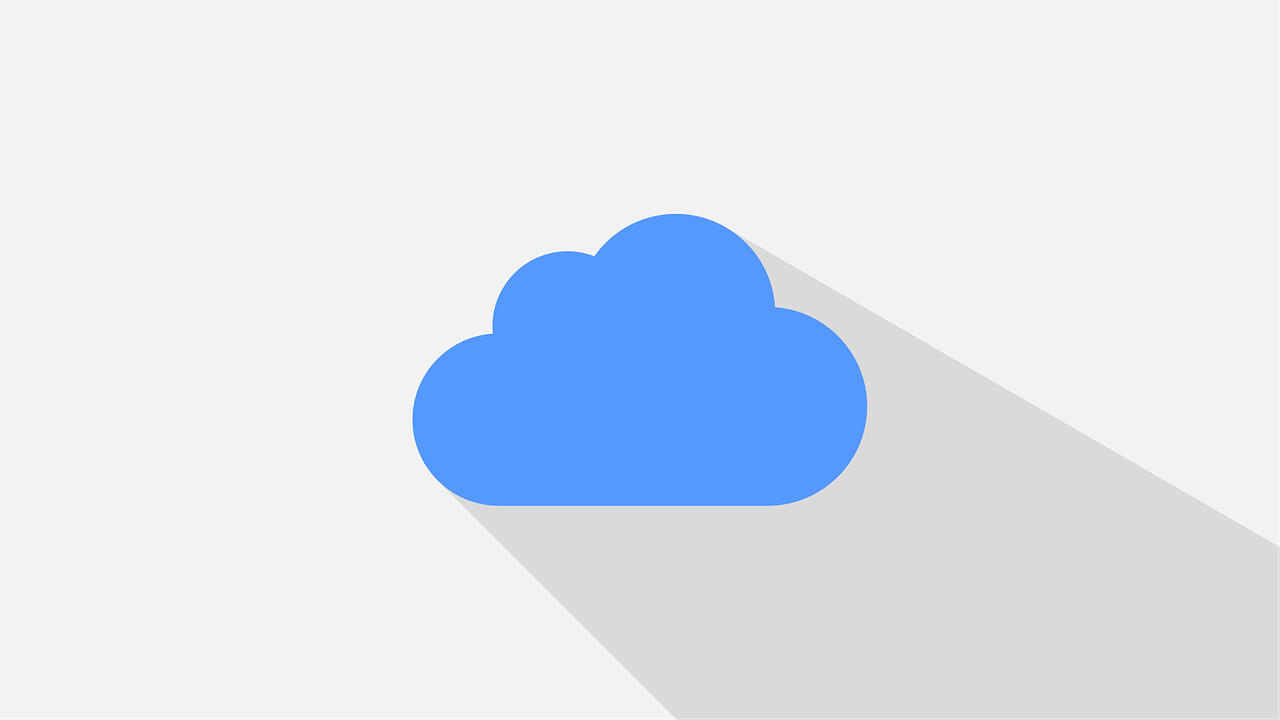
A1: 虽然技术上可行,但这通常不是一个好主意,将访问令牌暴露在客户端代码中可能会导致安全问题,最好的做法是在服务器端处理认证和授权,然后通过安全的API来与客户端代码交互。
Q2: 我可以使用Graph API代替REST API吗?
A2: 是的,你可以使用Microsoft Graph API来访问SharePoint数据,Graph API提供了更丰富的功能和更好的性能,它的学习曲线可能比REST API更陡峭。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/982609.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复