javascript,const mysql = require('mysql');,,const connection = mysql.createConnection({, host: 'localhost',, user: 'root',, password: 'your_password',, database: 'your_database',});,,connection.connect();,,const createTableSql =
CREATE TABLE your_table (, id INT AUTO_INCREMENT PRIMARY KEY,, name VARCHAR(255) NOT NULL,, age INT,);,,connection.query(createTableSql, (error, results, fields) => {, if (error) throw error;, console.log('Table created successfully!');,});,,connection.end();,
`,,请将上述代码中的
your_password、
your_database和
your_table`替换为实际的值。在Node.js中创建MySQL数据库表,你需要使用一个库来与MySQL数据库进行交互,最流行的选择之一是mysql
或mysql2
模块,下面是如何使用这些库以及如何创建一个MySQL数据库表的详细步骤。
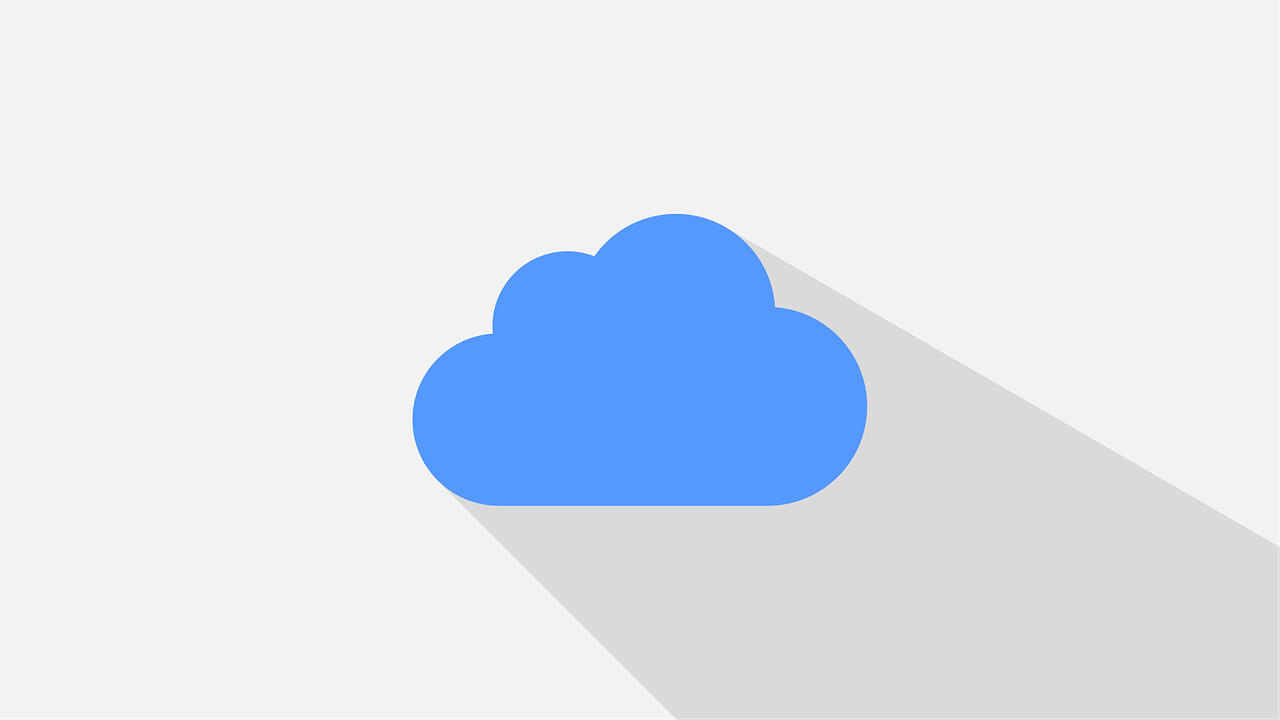
安装MySQL驱动
我们需要安装Node.js的MySQL驱动,打开终端并运行以下命令:
npm install mysql2
这会将mysql2
模块安装到你的项目中。
连接到MySQL数据库
接下来创建一个JavaScript文件(例如app.js
),并在其中引入mysql2
模块,设置数据库连接参数,如下所示:
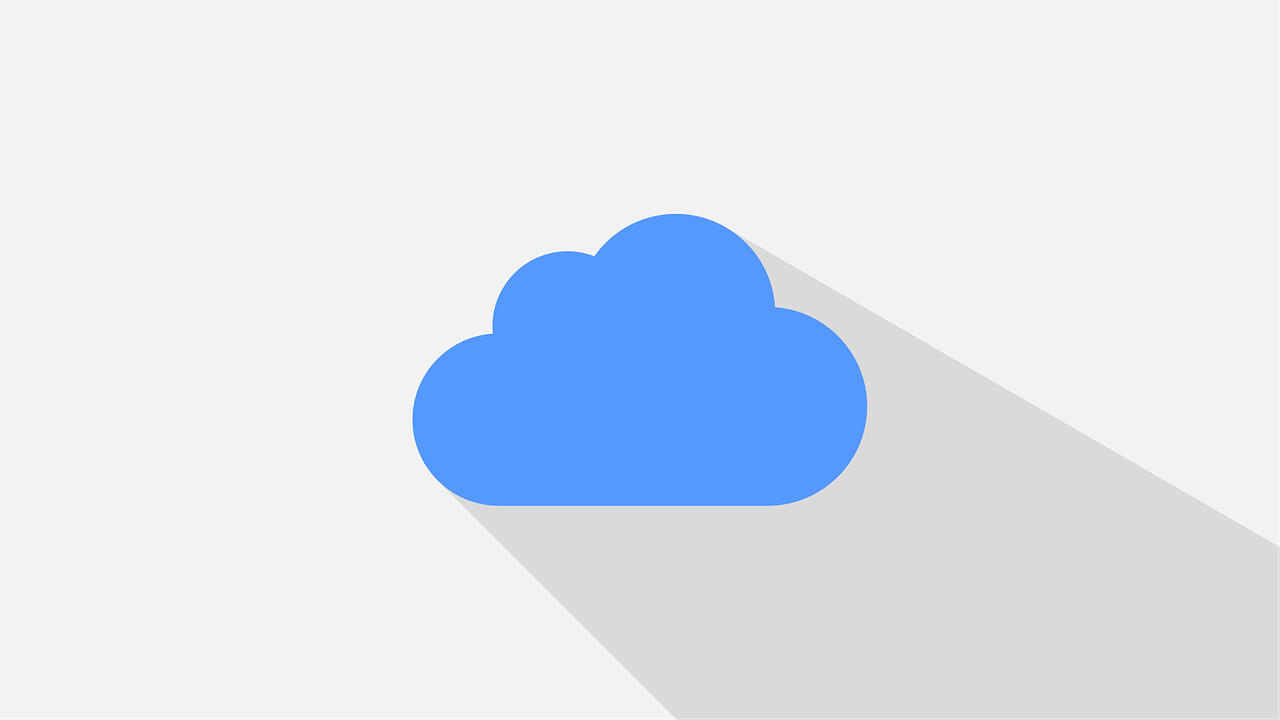
const mysql = require('mysql2/promise'); const dbConfig = { host: 'localhost', // 数据库地址 user: 'root', // 数据库用户名 password: 'password', // 数据库密码 database: 'mydb' // 使用的数据库名 }; async function main() { const connection = await mysql.createConnection(dbConfig); if (connection) { console.log('Connected to the database.'); connection.end(); } else { console.error('Error connecting to the database.'); } } main().catch(console.error);
确保你已经有一个正在运行的MySQL服务器,并且替换上述代码中的配置以匹配你的设置。
创建表
我们将编写一个函数来创建一个新的数据表,假设我们要在一个名为users
的表中存储用户信息,表结构可能如下:
id
(主键,自增)
name
(姓名,字符串类型)
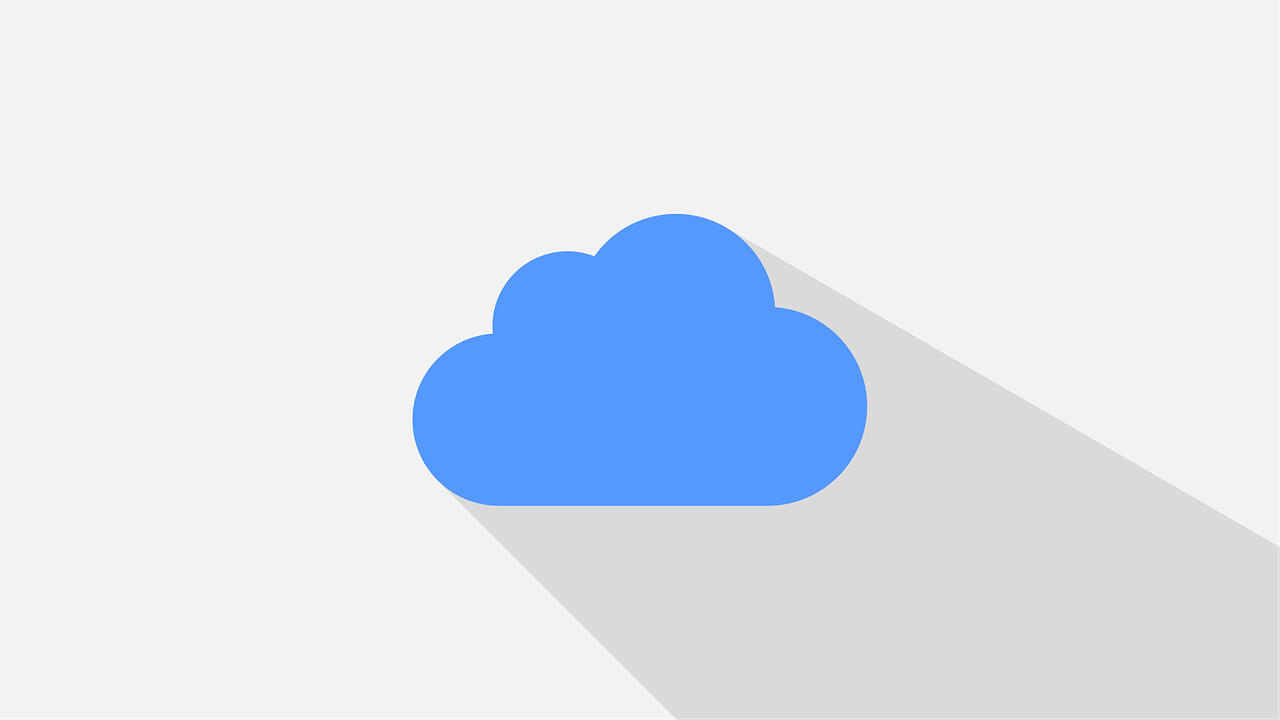
email
(电子邮件,字符串类型)
created_at
(创建时间,日期时间类型)
我们可以扩展上面的脚本,添加一个建表的函数:
async function createTable() { const sql = `CREATE TABLE IF NOT EXISTS users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL UNIQUE, created_at DATETIME DEFAULT CURRENT_TIMESTAMP );`; try { const connection = await mysql.createConnection(dbConfig); await connection.query(sql); console.log('Table created successfully.'); connection.end(); } catch (error) { console.error('Error creating table:', error); } } createTable().catch(console.error);
这个函数定义了一个SQL语句来创建users
表,并使用connection.query()
方法执行它。
完整示例代码
将上述所有部分合并到一个文件中,完整的示例代码如下:
const mysql = require('mysql2/promise'); const dbConfig = { host: 'localhost', user: 'root', password: 'password', database: 'mydb' }; async function main() { // Connect to the database const connection = await mysql.createConnection(dbConfig); if (connection) { console.log('Connected to the database.'); connection.end(); } else { console.error('Error connecting to the database.'); } } async function createTable() { const sql = `CREATE TABLE IF NOT EXISTS users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL UNIQUE, created_at DATETIME DEFAULT CURRENT_TIMESTAMP );`; try { const connection = await mysql.createConnection(dbConfig); await connection.query(sql); console.log('Table created successfully.'); connection.end(); } catch (error) { console.error('Error creating table:', error); } } main().catch(console.error); createTable().catch(console.error);
相关问题与解答
Q1: 如果数据库连接失败怎么办?
A1: 如果数据库连接失败,你应该检查数据库服务是否正在运行,确认你提供的主机名、端口、用户名和密码是否正确,确保网络没有阻止对数据库服务器的访问,如果错误信息表明是权限问题,你可能需要为数据库用户授权或者修改用户的权限。
Q2: 如果我想删除已存在的表怎么办?
A2: 如果你想删除已存在的表,可以使用DROP TABLE IF EXISTS
语句来删除表,只需将上述示例中的CREATE TABLE IF NOT EXISTS
替换为DROP TABLE IF EXISTS
即可,但是要注意,这样做会永久删除该表及其所有数据,所以请谨慎操作。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/914651.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复