在MapReduce中,参数传递通常通过配置对象(Configuration)来实现,在MapReduce程序中,可以通过设置和获取配置对象的参数来实现页面间参数传递,以下是详细步骤:
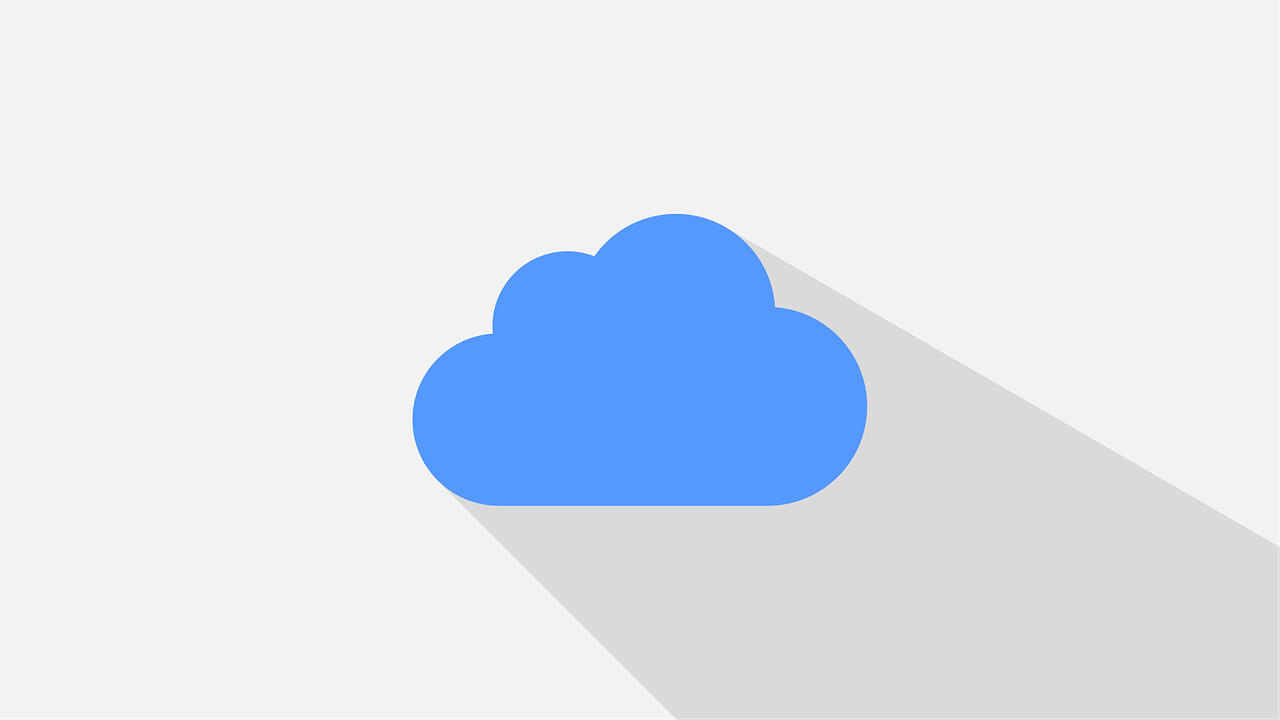
1. 设置参数
在MapReduce程序中,可以通过以下方法设置参数:
1.1 在驱动程序中设置参数
在驱动程序中,可以通过Configuration
对象的set
方法设置参数。
Configuration conf = new Configuration(); conf.set("param_name", "param_value");
1.2 在Mapper或Reducer中设置参数
在Mapper或Reducer中,可以通过setup
方法设置参数。
public void setup(Context context) throws IOException, InterruptedException { Configuration conf = context.getConfiguration(); conf.set("param_name", "param_value"); }
2. 获取参数
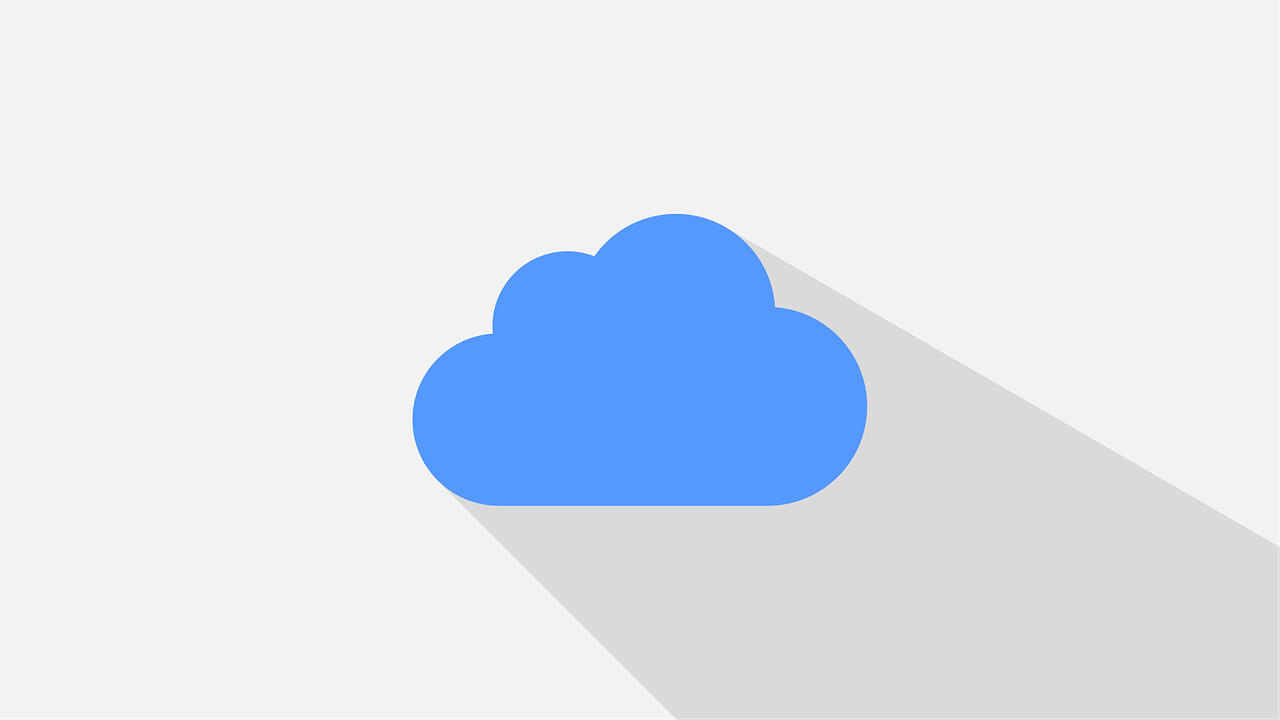
在MapReduce程序中,可以通过以下方法获取参数:
2.1 在Mapper或Reducer中获取参数
在Mapper或Reducer中,可以通过Context
对象的getConfiguration
方法获取Configuration
对象,然后通过get
方法获取参数。
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { Configuration conf = context.getConfiguration(); String paramValue = conf.get("param_name"); }
3. 参数传递示例
以下是一个简单的MapReduce程序,用于演示如何设置和获取参数:
// 导入所需的库 import java.io.IOException; import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class ParameterPassingExample { public static class MyMapper extends Mapper<LongWritable, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String[] words = value.toString().split("\s+"); for (String w : words) { word.set(w); context.write(word, one); } } } public static class MyReducer extends Reducer<Text, IntWritable, Text, IntWritable> { private IntWritable result = new IntWritable(); public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } result.set(sum); context.write(key, result); } } public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); conf.set("param_name", "param_value"); Job job = Job.getInstance(conf, "parameter passing example"); job.setJarByClass(ParameterPassingExample.class); job.setMapperClass(MyMapper.class); job.setCombinerClass(MyReducer.class); job.setReducerClass(MyReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
在这个示例中,我们设置了名为param_name
的参数,其值为param_value
,然后在Mapper和Reducer中,我们可以通过Context
对象获取这个参数。
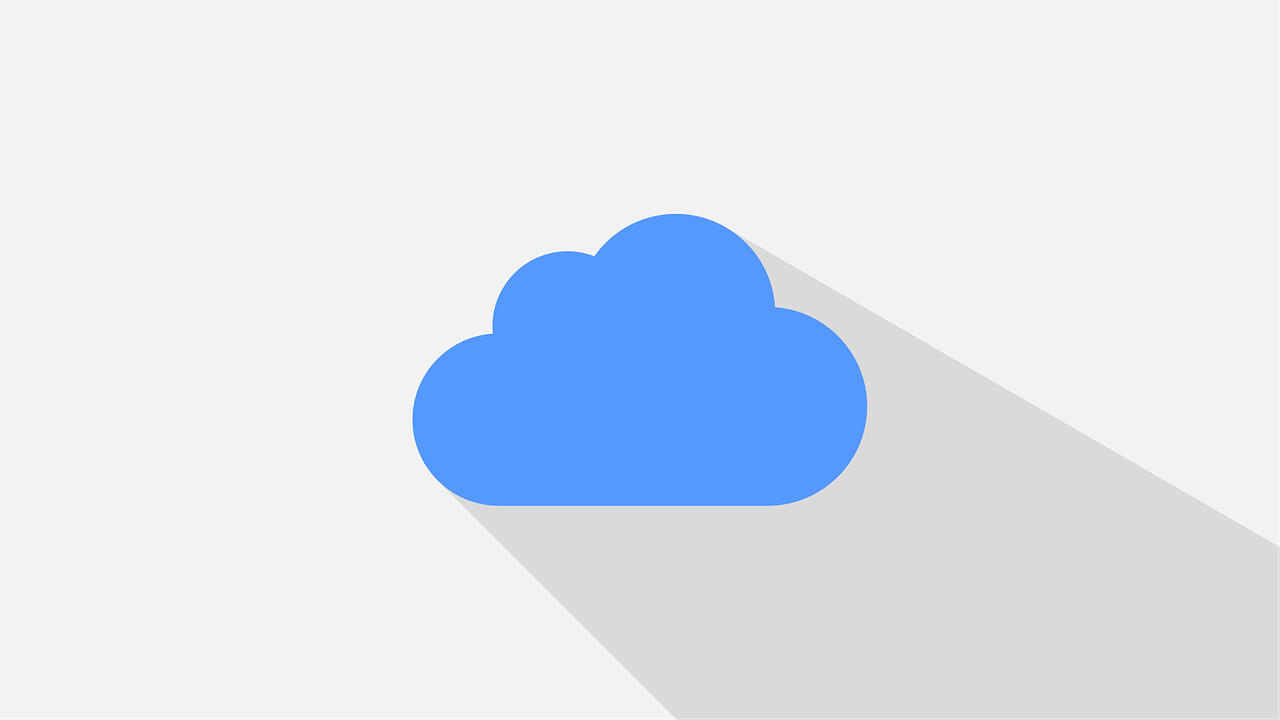
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/878722.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复