MapReduce 批量加载HBase数据并生成本地二级索引
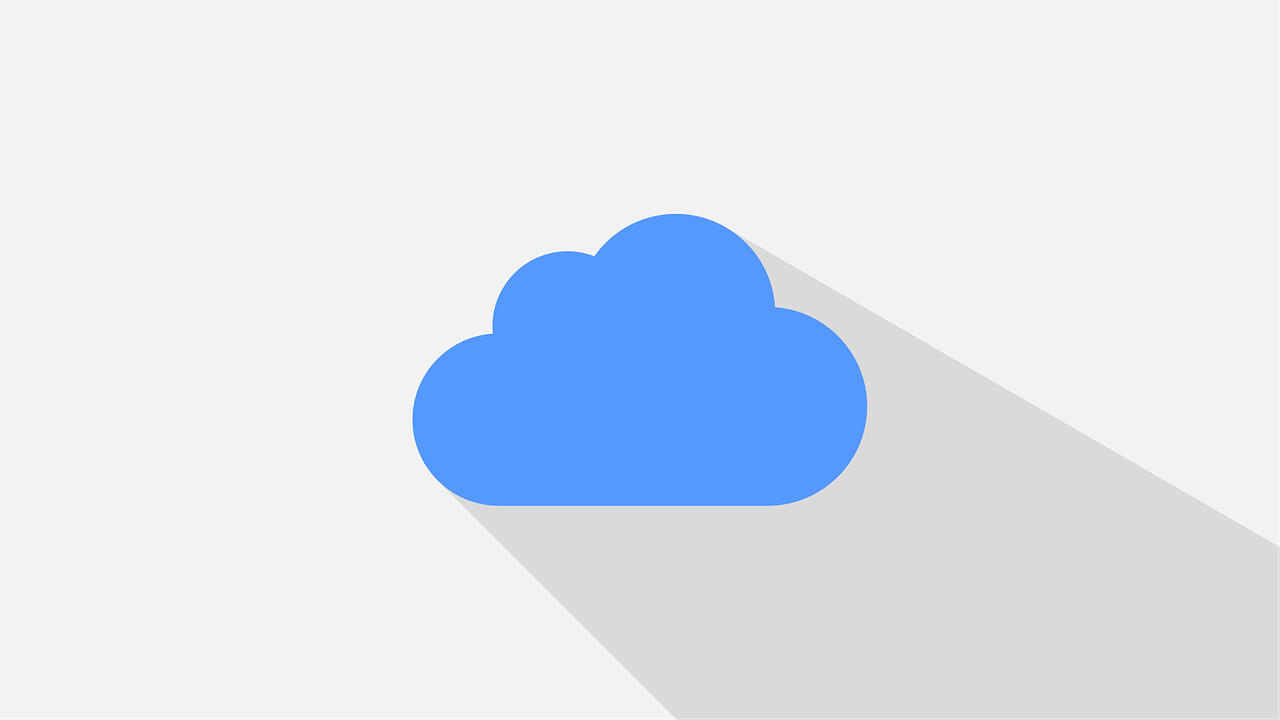
步骤1:准备数据
确保你的数据已经准备好,并且按照适当的格式存储,MapReduce作业会从HDFS或其他分布式文件系统中读取数据。
步骤2:编写MapReduce程序
Mapper类
创建一个继承自org.apache.hadoop.mapreduce.Mapper
的Mapper类,用于处理输入数据并将其转换为键值对(keyvalue pairs)。
import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; public class HBaseBulkLoadMapper extends Mapper<LongWritable, Text, Text, Text> { @Override protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { // 解析输入数据,例如CSV格式 String[] fields = value.toString().split(","); String rowKey = fields[0]; // 假设第一列是行键 String columnFamily = fields[1]; // 假设第二列是列族 String columnQualifier = fields[2]; // 假设第三列是列限定符 String cellValue = fields[3]; // 假设第四列是单元格值 // 输出键值对,其中键是行键,值是列族:列限定符=单元格值 context.write(new Text(rowKey), new Text(columnFamily + ":" + columnQualifier + "=" + cellValue)); } }
Reducer类
创建一个继承自org.apache.hadoop.mapreduce.Reducer
的Reducer类,用于将Mapper输出的键值对聚合为最终的键值对。
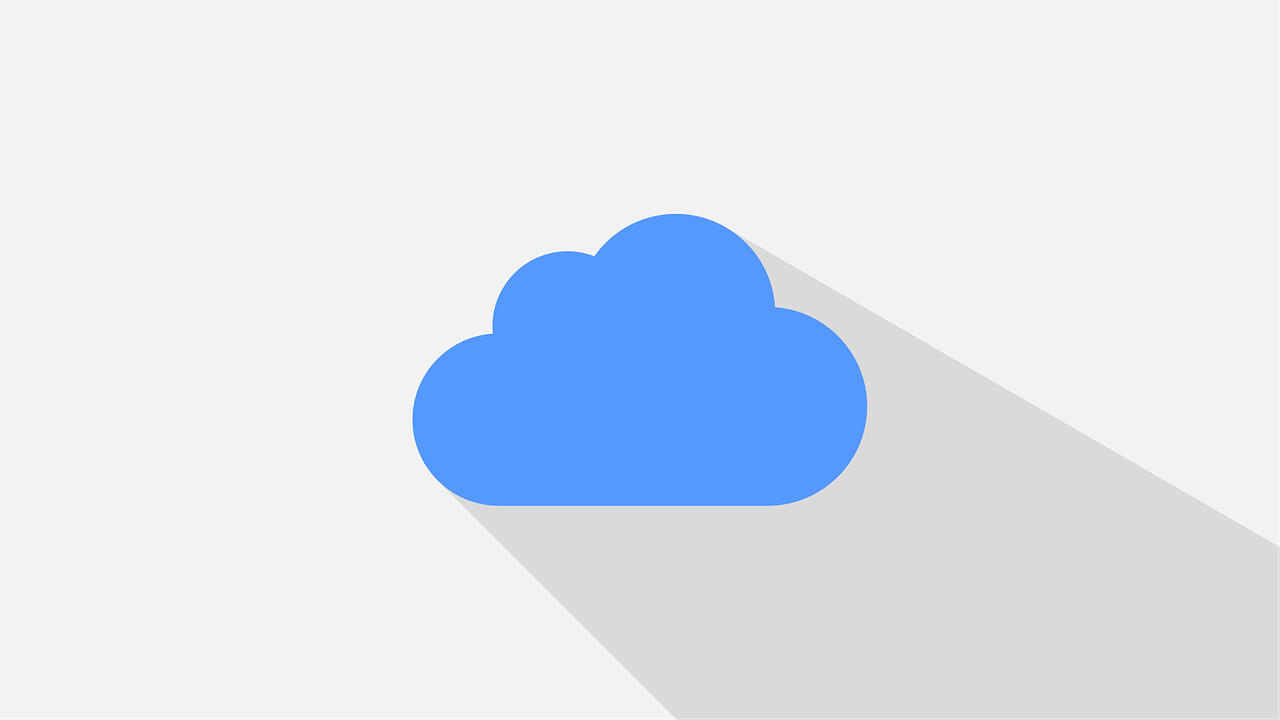
import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Reducer; public class HBaseBulkLoadReducer extends Reducer<Text, Text, Text, Text> { @Override protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException { // 遍历所有值并将它们连接在一起,用换行符分隔 StringBuilder sb = new StringBuilder(); for (Text value : values) { sb.append(value).append("n"); } // 输出键值对,其中键是行键,值是完整的单元格信息 context.write(key, new Text(sb.toString())); } }
步骤3:配置和运行MapReduce作业
在提交MapReduce作业之前,需要设置作业的配置参数,包括输入路径、输出路径、Mapper类、Reducer类等。
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class HBaseBulkLoadJob { public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "HBase Bulk Load and Secondary Index Generation"); job.setJarByClass(HBaseBulkLoadJob.class); job.setMapperClass(HBaseBulkLoadMapper.class); job.setReducerClass(HBaseBulkLoadReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(Text.class); FileInputFormat.addInputPath(job, new Path(args[0])); // 输入路径 FileOutputFormat.setOutputPath(job, new Path(args[1])); // 输出路径 System.exit(job.waitForCompletion(true) ? 0 : 1); } }
步骤4:导入数据到HBase
使用HBase提供的ImportTsv
工具将MapReduce作业的输出导入到HBase表中,确保你已经创建了相应的表结构。
hbase org.apache.hadoop.hbase.mapreduce.ImportTsv Dimporttsv.columns="HBASE_ROW_KEY,COLUMN_FAMILY:COLUMN_QUALIFIER,CELL_VALUE" /path/to/output/from/mapreduce
步骤5:生成本地二级索引
为了生成本地二级索引,你需要在HBase中创建一个新的表,该表包含你想要索引的列,你可以使用HBase的协处理器(coprocessor)功能来生成索引,具体实现取决于你的需求和使用的HBase版本。
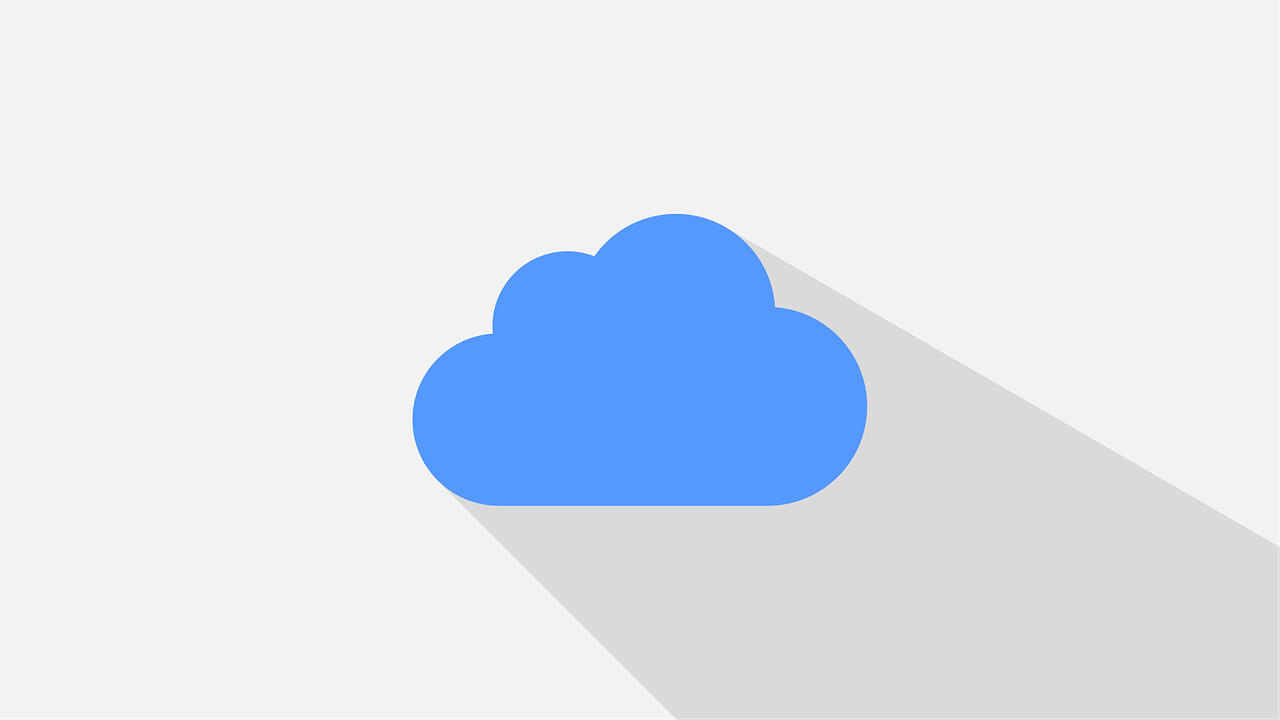
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/857261.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复