MapReduce是一种编程模型,用于处理和生成大数据集,它由两个阶段组成:Map阶段和Reduce阶段,在读取MySQL数据时,我们可以使用Hadoop的MapReduce框架来处理这些数据,以下是一个简单的示例,展示了如何使用MapReduce从MySQL数据库中读取数据。
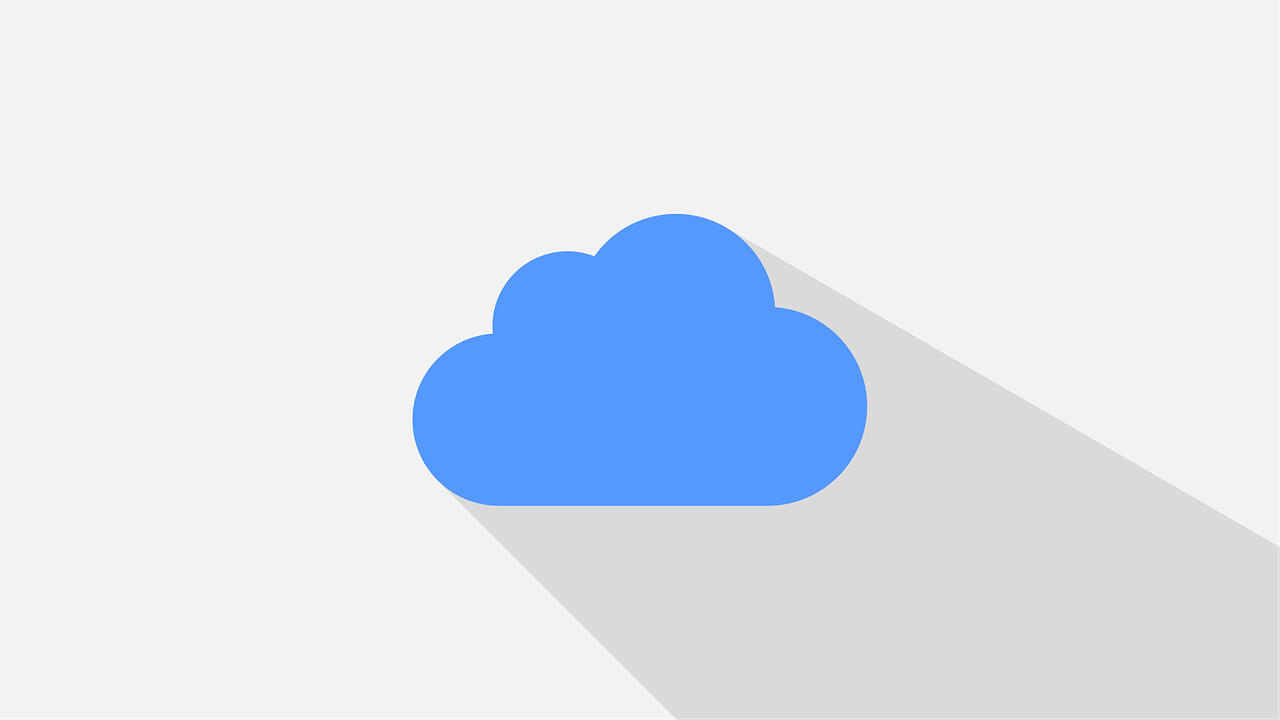
1、确保已经安装了Hadoop和MySQL JDBC驱动程序。
2、创建一个Java项目,并添加以下依赖项到项目的pom.xml文件中(如果使用Maven):
<dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysqlconnectorjava</artifactId> <version>8.0.26</version> </dependency> <dependency> <groupId>org.apache.hadoop</groupId> <artifactId>hadoopcore</artifactId> <version>3.3.1</version> </dependency> </dependencies>
3、编写一个Mapper类,用于从MySQL数据库中读取数据:
import java.io.IOException; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; public class MysqlMapper extends Mapper<LongWritable, Text, Text, Text> { private Connection connection; private Statement statement; private ResultSet resultSet; @Override protected void setup(Context context) throws IOException, InterruptedException { try { Class.forName("com.mysql.cj.jdbc.Driver"); connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); statement = connection.createStatement(); resultSet = statement.executeQuery("SELECT * FROM mytable"); } catch (Exception e) { e.printStackTrace(); } } @Override protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { try { while (resultSet.next()) { String id = resultSet.getString("id"); String name = resultSet.getString("name"); context.write(new Text(id), new Text(name)); } } catch (Exception e) { e.printStackTrace(); } } @Override protected void cleanup(Context context) throws IOException, InterruptedException { try { if (resultSet != null) { resultSet.close(); } if (statement != null) { statement.close(); } if (connection != null) { connection.close(); } } catch (Exception e) { e.printStackTrace(); } } }
4、编写一个Reducer类,用于处理从Mapper传递过来的数据:
import java.io.IOException; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Reducer; public class MysqlReducer extends Reducer<Text, Text, Text, Text> { @Override protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException { for (Text value : values) { context.write(key, value); } } }
5、编写一个驱动程序,用于运行MapReduce作业:
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class MysqlMapReduce { public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "Mysql MapReduce"); job.setJarByClass(MysqlMapReduce.class); job.setMapperClass(MysqlMapper.class); job.setReducerClass(MysqlReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(Text.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
6、编译并运行程序,将MySQL数据作为输入传递给MapReduce作业,注意,这里的示例代码仅用于演示目的,实际应用中可能需要根据具体需求进行调整。
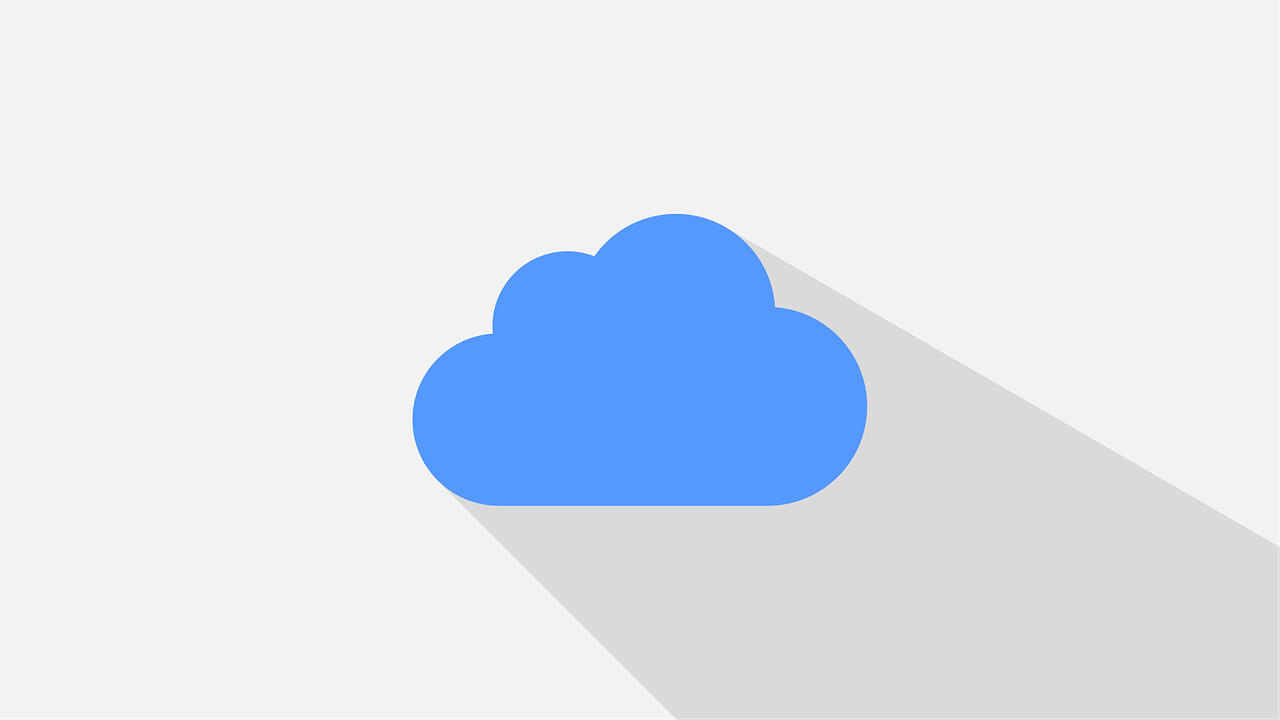
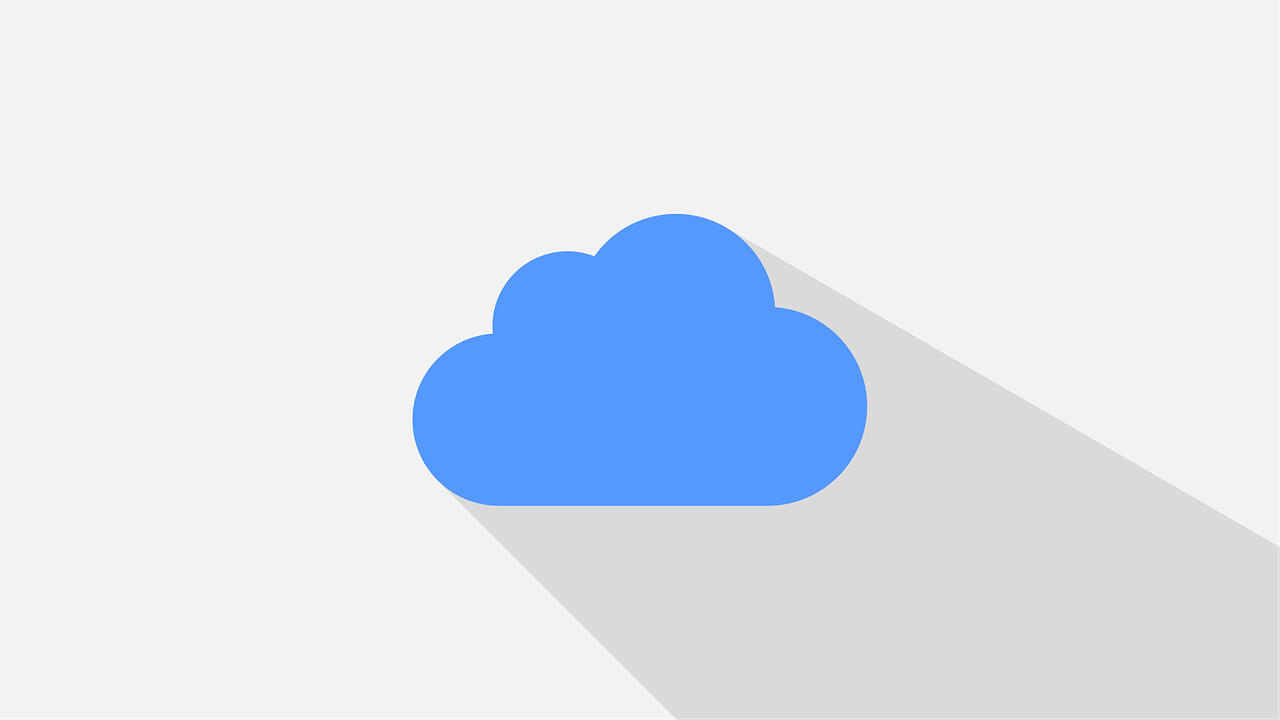
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/856273.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复