MapReduce是一种编程模型,用于处理和生成大数据集的并行计算,在MapReduce中,map函数是数据处理的第一步,它将输入数据转换为一组键值对(keyvalue pairs),每个键值对都代表一个中间结果,这些中间结果将被传递给reduce函数进行处理。
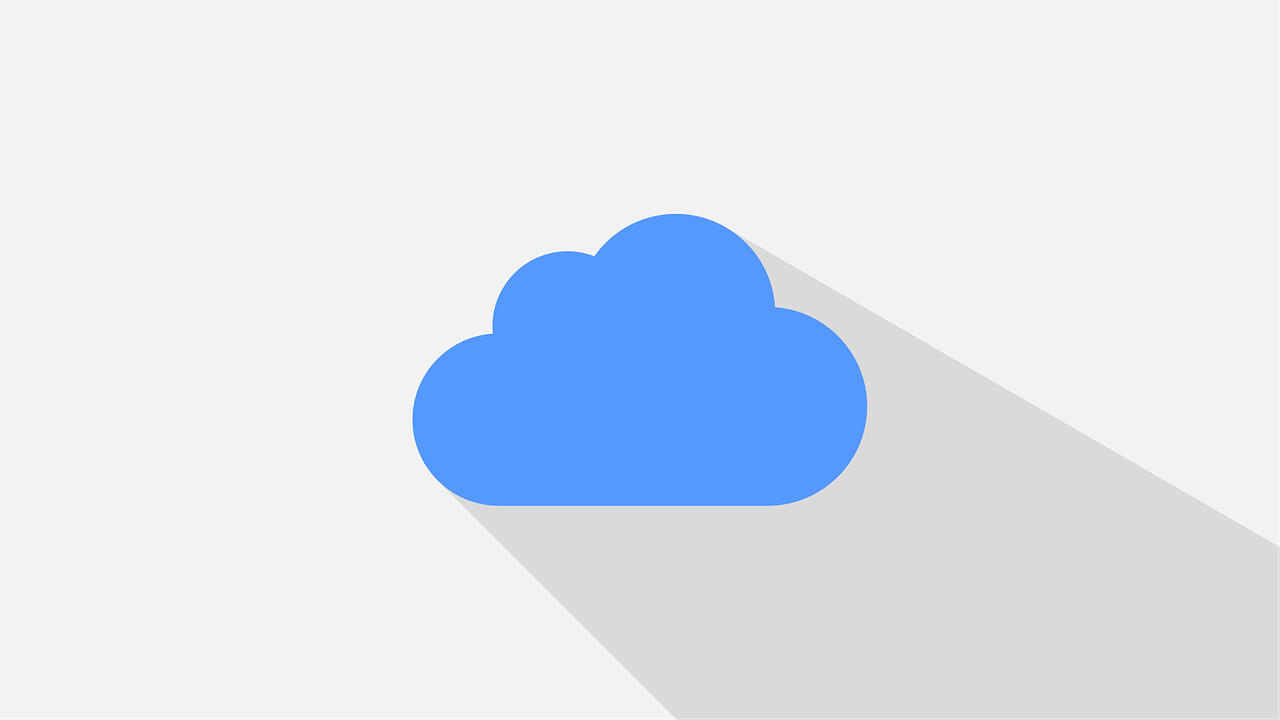
以下是关于MapReduce map函数执行次数的一些详细信息:
1. Map函数执行次数
Map函数的执行次数取决于输入数据的分片数量以及每个分片的大小,假设有N个输入分片,每个分片包含M个元素,那么map函数将执行N * M次。
2. 示例代码
以下是一个使用Python编写的简单MapReduce程序,其中包含一个map函数和一个reduce函数,这个例子展示了如何计算文本文件中单词的出现次数。
from collections import defaultdict import itertools def map_function(text): """ Map function that splits the text into words and returns a list of (word, 1) pairs. """ words = text.split() return [(word, 1) for word in words] def reduce_function(word, counts): """ Reduce function that sums up the counts for each word. """ return (word, sum(counts)) Example input data input_data = ["hello world", "hello mapreduce", "mapreduce is fun"] Step 1: Map phase mapped_data = [] for text in input_data: mapped_data.extend(map_function(text)) Step 2: Shuffle and sort phase (not shown in this example) In a real MapReduce implementation, the mapped data would be shuffled and sorted by key. Step 3: Reduce phase grouped_data = itertools.groupby(sorted(mapped_data), key=lambda x: x[0]) reduced_data = [reduce_function(word, [count for _, count in group]) for word, group in grouped_data] print(reduced_data)
在这个例子中,map_function
将输入文本分割成单词,并为每个单词生成一个键值对(word, 1)。reduce_function
将所有相同单词的计数相加,输出结果是每个单词及其出现次数的列表。
3. Map函数执行次数与输入数据的关系
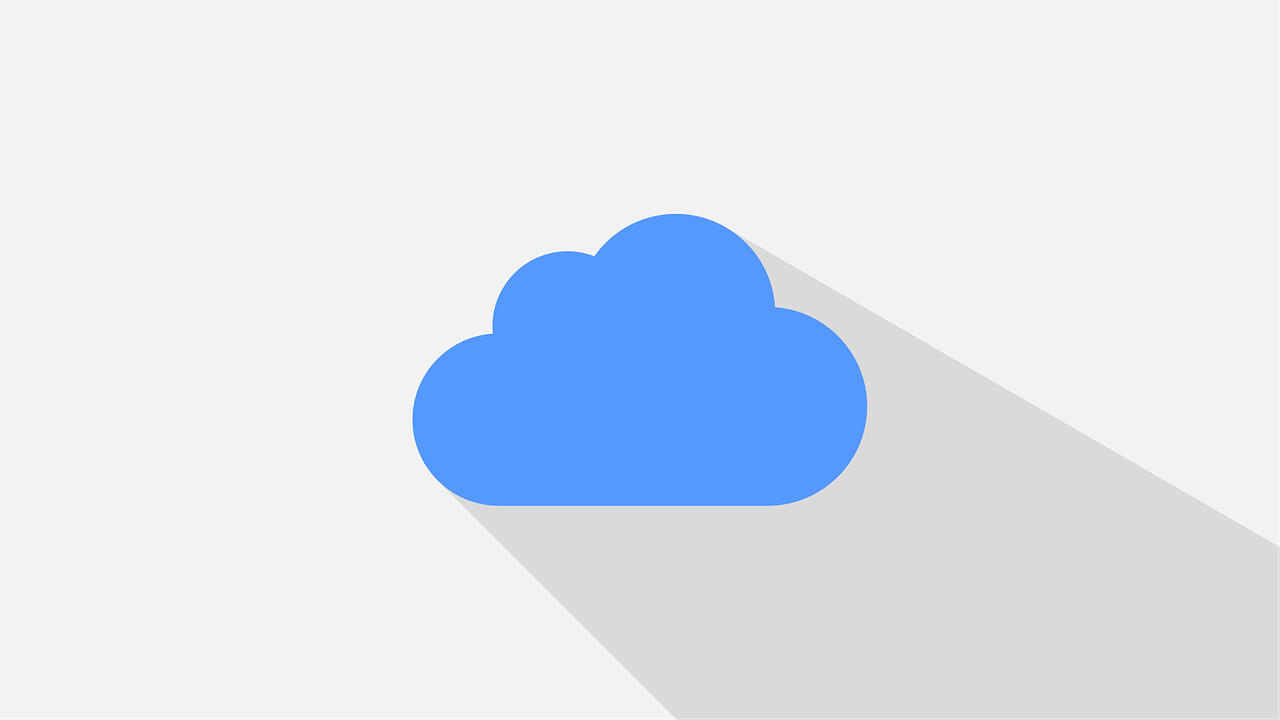
Map函数的执行次数取决于输入数据的分片数量和每个分片的大小,如果输入数据被分成更多的分片,或者每个分片包含更多的元素,那么map函数将执行更多的次数,为了提高处理速度,可以将大文件分成多个小文件,以便并行处理。
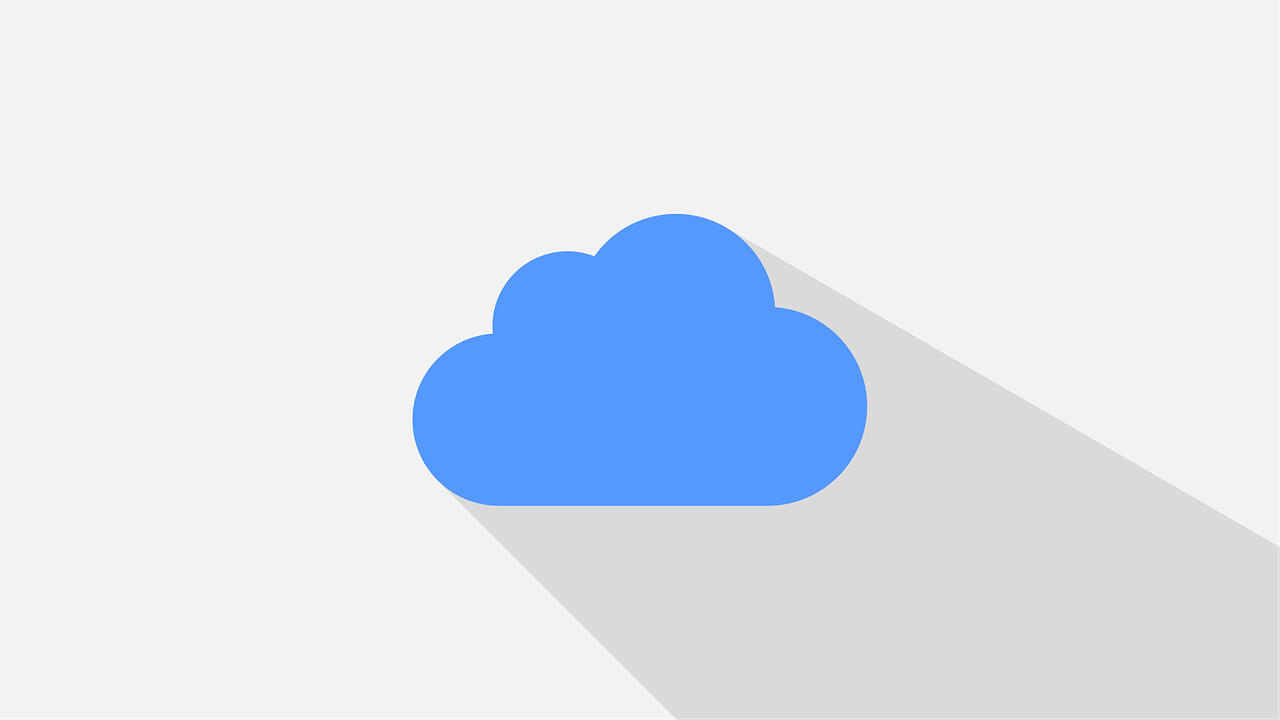
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/853917.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复