MapReduce 是一种编程模型,用于处理大规模数据集。在 Hadoop 生态系统中,可以使用 MapReduce 作业从 HBase 读取数据,然后进行处理,并将结果写回 HBase。这种操作通常用于数据转换、聚合和分析任务。
MapReduce是一种编程模型,用于处理和生成大数据集,HBase是一个分布式、可扩展的大数据存储系统,它基于Google的BigTable设计,下面是一个使用MapReduce从HBase读取数据并将其写回HBase的示例。
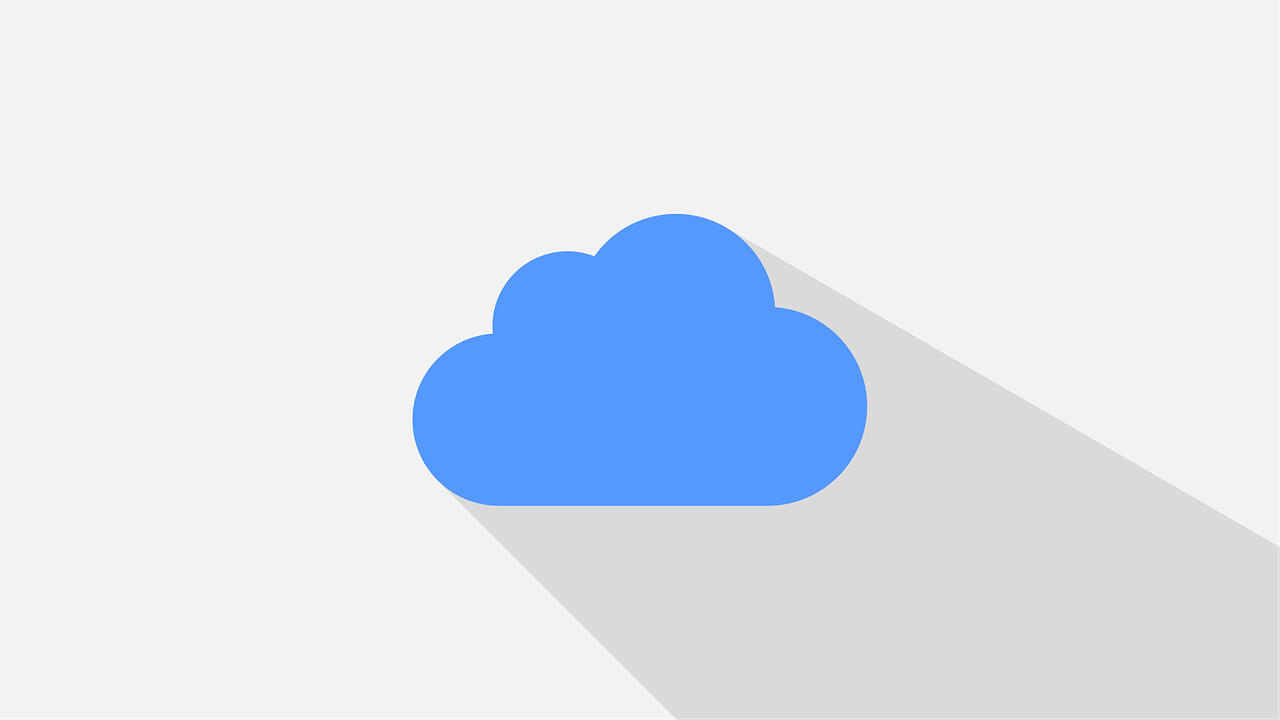
(图片来源网络,侵删)
1. 准备工作
确保你已经安装了Hadoop和HBase,并正确配置了它们,你需要有一个Java开发环境来编写MapReduce程序。
2. 创建HBase表
在HBase shell中创建一个表,
create 'test_table', 'cf'
这将创建一个名为test_table
的表,其中包含一个名为cf
的列族。
3. 编写MapReduce程序
以下是一个简单的MapReduce程序,用于从HBase读取数据并将其写回HBase。
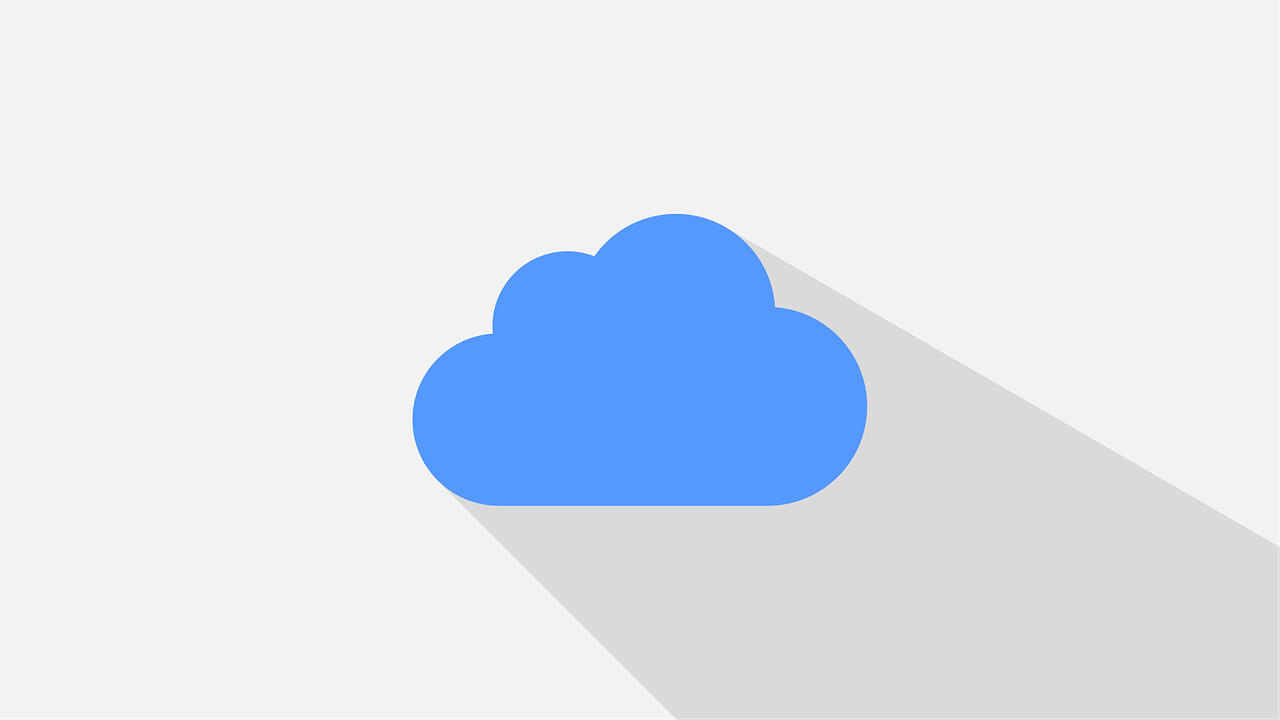
(图片来源网络,侵删)
3.1 Mapper类
import org.apache.hadoop.hbase.client.Put; import org.apache.hadoop.hbase.io.ImmutableBytesWritable; import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil; import org.apache.hadoop.hbase.util.Bytes; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; public class HBaseReadWriteMapper extends Mapper<Object, Text, ImmutableBytesWritable, Put> { private static final byte[] ROW_KEY = Bytes.toBytes("rowkey"); private static final byte[] COLUMN_FAMILY = Bytes.toBytes("cf"); private static final byte[] COLUMN_QUALIFIER = Bytes.toBytes("column"); @Override protected void map(Object key, Text value, Context context) throws IOException, InterruptedException { String inputValue = value.toString(); Put put = new Put(ROW_KEY); put.addColumn(COLUMN_FAMILY, COLUMN_QUALIFIER, Bytes.toBytes(inputValue)); context.write(new ImmutableBytesWritable(ROW_KEY), put); } }
3.2 Reducer类
在这个例子中,我们不需要Reducer,因为我们只是将数据从一个表复制到另一个表,我们可以省略Reducer类。
3.3 Driver类
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.hbase.HBaseConfiguration; import org.apache.hadoop.hbase.client.Connection; import org.apache.hadoop.hbase.client.ConnectionFactory; import org.apache.hadoop.hbase.client.Table; import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil; import org.apache.hadoop.hbase.mapreduce.TableOutputFormat; import org.apache.hadoop.hbase.util.Bytes; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.lib.input.TextInputFormat; import org.apache.hadoop.mapreduce.lib.output.TextOutputFormat; import org.apache.hadoop.util.GenericOptionsParser; public class HBaseReadWriteDriver { public static void main(String[] args) throws Exception { Configuration conf = HBaseConfiguration.create(); String[] otherArgs = new GenericOptionsParser(conf, args).getRemainingArgs(); if (otherArgs.length != 1) { System.err.println("Usage: HBaseReadWriteDriver <input>"); System.exit(1); } Job job = Job.getInstance(conf, "HBase Read Write"); job.setJarByClass(HBaseReadWriteDriver.class); job.setMapperClass(HBaseReadWriteMapper.class); job.setNumReduceTasks(0); // No reducer needed // Set input and output formats TextInputFormat.addInputPath(job, new Path(otherArgs[0])); job.setOutputFormatClass(TextOutputFormat.class); // Set output table info TableMapReduceUtil.initTableReducerJob( "test_table", // output table name null, // reducer class (not needed) job, TableOutputFormat.class, TextOutputFormat.class, TextInputFormat.class, false // no reducer needed ); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
4. 运行MapReduce作业
编译并打包你的MapReduce程序,然后使用以下命令运行它:
hadoop jar yourjarfile.jar HBaseReadWriteDriver /path/to/input/data
这将从指定的输入路径读取数据,并将数据写入名为test_table
的HBase表中。
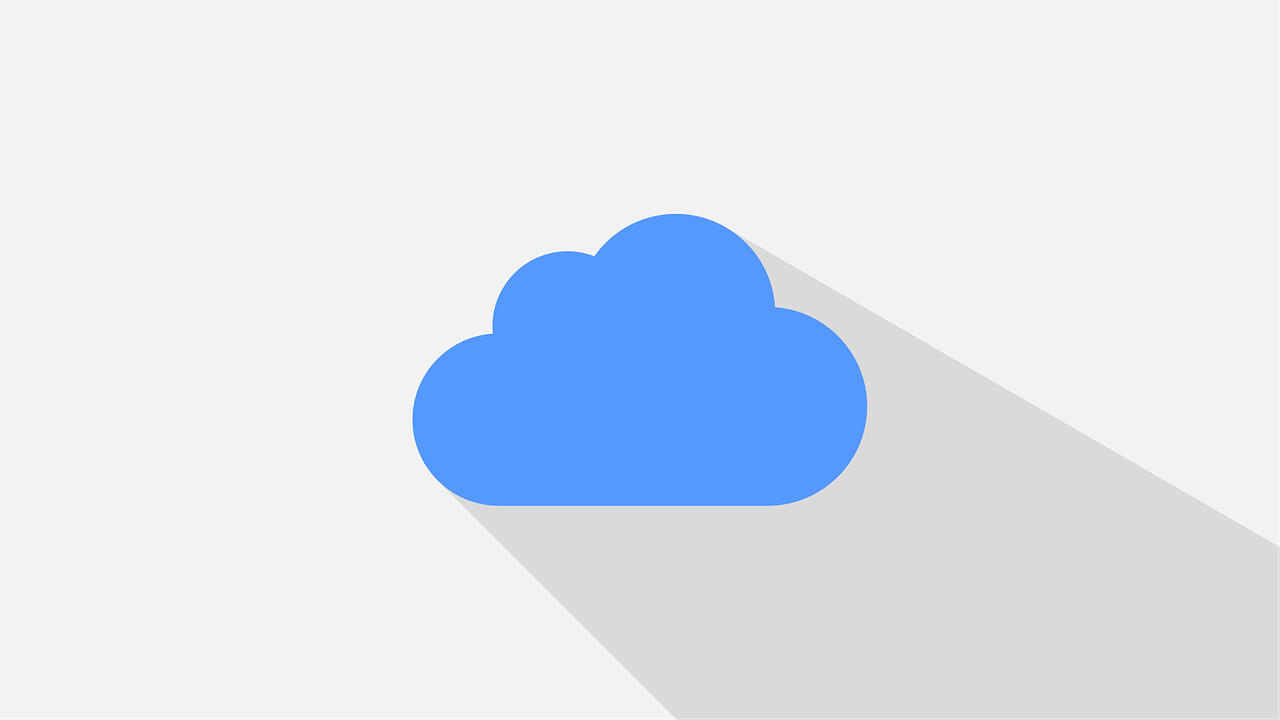
(图片来源网络,侵删)
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/828168.html