Python项目管理
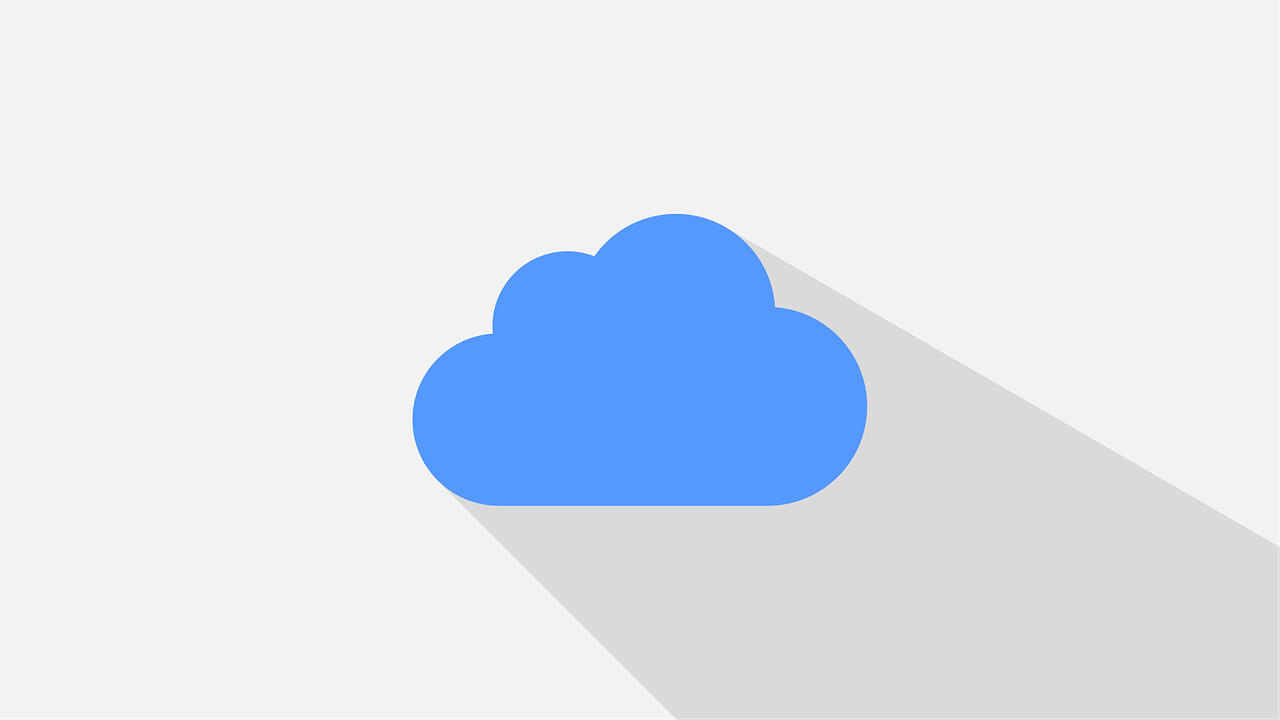
Python是一种广泛使用的高级编程语言,用于开发各种类型的应用程序,在大型项目中,管理代码和资源变得尤为重要,本文将介绍如何使用Python进行项目管理,包括版本控制、依赖管理、测试和文档编写等方面。
1. 版本控制
版本控制是软件开发过程中的重要组成部分,它允许开发者跟踪和管理代码的变更,Git是目前最流行的版本控制系统之一,可以通过以下命令安装:
pip install gitpython
使用Git进行版本控制的基本步骤如下:
1、初始化一个新的Git仓库:
git init
2、添加文件到暂存区:
git add <file>
3、提交更改:
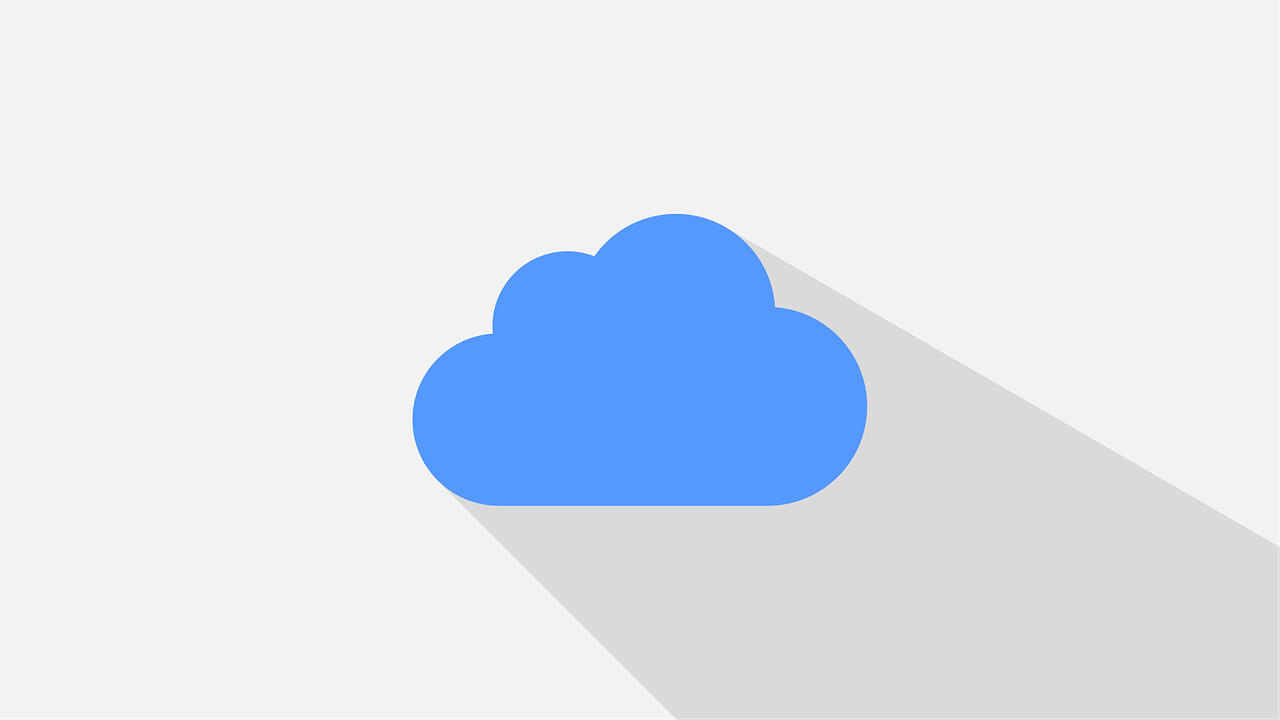
git commit m "<commit message>"
4、查看提交历史:
git log
5、创建分支并切换到新分支:
git checkout b <branch_name>
6、合并分支:
git merge <branch_name>
7、删除分支:
git branch d <branch_name>
2. 依赖管理
依赖管理是指管理项目所需的第三方库和模块,Python的包管理器pip可以方便地安装和管理依赖,以下是一些常用的pip命令:
安装库:pip install <library>
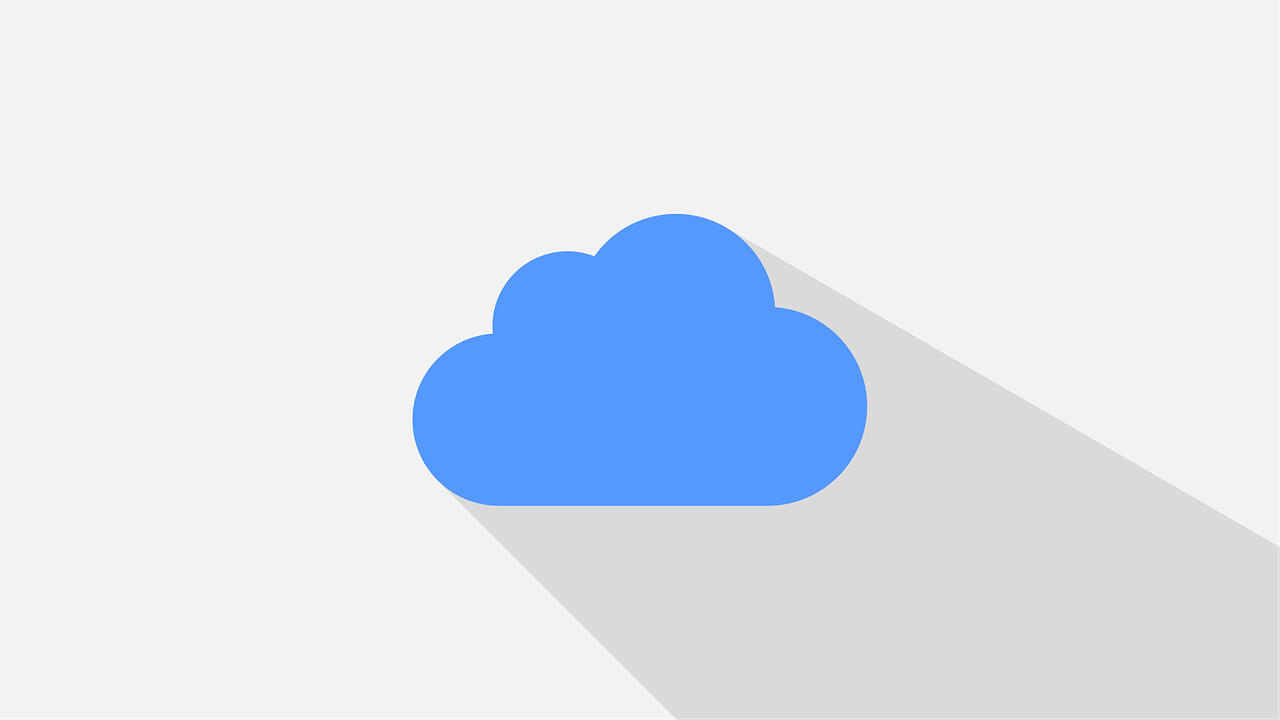
升级库:pip install upgrade <library>
卸载库:pip uninstall <library>
列出已安装的库:pip list
搜索库:pip search <keyword>
显示库详细信息:pip show <library>
生成虚拟环境:pip install virtualenv
,然后运行virtualenv venv
创建虚拟环境,激活虚拟环境:venvScriptsactivate
(Windows)或source venv/bin/activate
(Linux/Mac)。
3. 测试
编写单元测试是确保代码质量的重要手段,Python的unittest模块提供了丰富的测试功能,以下是一个简单的单元测试示例:
import unittest from my_module import add, subtract class TestMathFunctions(unittest.TestCase): def test_add(self): self.assertEqual(add(1, 2), 3) self.assertEqual(add(1, 1), 0) def test_subtract(self): self.assertEqual(subtract(5, 3), 2) self.assertEqual(subtract(0, 0), 0) if __name__ == '__main__': unittest.main()
要运行测试,可以使用以下命令:
python m unittest discover tests/test_*.py
tests/test_*.py
是包含测试用例的文件名模式,测试结果将显示每个测试用例的通过与否。
4. 文档编写
编写清晰的文档有助于其他开发者理解和维护代码,Python的docstring是编写文档的一种方式,它位于函数、类或模块的开头,以三引号包围。
def add(a, b): """Add two numbers and return the result.""" return a + b
还可以使用reStructuredText格式编写更详细的文档,可以使用Sphinx工具将reStructuredText文档转换为HTML、PDF等格式,首先安装Sphinx:pip install sphinx
,然后运行sphinxquickstart
命令创建一个新的Sphinx项目,在项目目录中,编辑index.rst
文件编写文档内容,最后运行make html
命令生成HTML文档。
5. 持续集成与部署(CI/CD)
持续集成与部署(CI/CD)是一种自动化构建、测试和部署软件的方法,Python项目可以使用Jenkins、Travis CI等工具实现CI/CD,以下是一个使用Travis CI进行持续集成的示例:在项目根目录下创建一个名为.travis.yml
的文件,内容如下:
language: python python: "3.8" # or "3.7", "3.6", etc. depending on your project's requirements. Travis will automatically install the required version of Python for you. script: # This line is optional, but it helps Travis understand what to do when you push changes to your repository. echo "Testing..." # Add any additional commands here that are necessary for your project's testing process. after_success: # This line is also optional, but it can be useful if you want to send an email notification when your build passes successfully. echo "Build completed successfully!" | mail s "Build Success" your@email.com # You can also use other services like Slack or Microsoft Teams for notifications instead of email. before_deploy: # This line is optional, but it can be useful if you want to run any additional commands before your code is deployed to production. echo "Preparing for deployment..." # Add any additional commands here that are necessary for your project's deployment process. deploy: # This line is optional, but it can be useful if you want to automatically deploy your code to a server or cloud platform when your build passes successfully. provider: heroku # Change this to the name of the service you want to use for deployment (e.g., "aws", "azure", "google", etc.). api_key: $HEROKU_API_KEY # Add your API key here if you're using Heroku as your deployment provider (replace $HEROKU_API_KEY with your actual API key). app: mypythonapp # Change this to the name of your application (e.g., "myapp", "myproject", etc.). on: # This line is optional, but it can be useful if you want to specify which branches should trigger a build (e.g., only the master branch). branch: master # Change this to the name of the branch you want to use for building and deployment (e.g., "master", "develop", etc.). allow_failure: # This line is optional, but it can be useful if you want to allow builds to fail without causing the entire pipeline to fail (e.g., if you have a separate job for handling failed builds). master # Change this to the name of the branch you want to allow to fail (e.g., "master", "develop", etc.). only: # This line is optional, but it can be useful if you want to specify which jobs should run in addition to the main job (e.g., a job for running tests). test # Change this to the name of the job you want to run in addition to the main job (e.g., "test", "lint", etc.). script: # This line is optional, but it can be useful if you want to specify which command should be run when the job starts (e.g., a command for installing dependencies). echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process. after_script: # This line is optional, but it can be useful if you want to run any additional commands after the job has finished running (e.g., cleaning up temporary files). echo "Cleaning up..." # Add any additional commands here that are necessary for your project's cleanup process. environment: # This line is optional, but it can be useful if you want to specify any environment variables that should be set for the job (e.g., database credentials). variables: DB_USERNAME: myuser DB_PASSWORD: mypassword # Add any additional environment variables here that are necessary for your project's setup process (e.g., API keys, secret tokens, etc.). cache: # This line is optional, but it can be useful if you want to cache certain files or directories between builds (e.g., node_modules directory). directories: node_modules/ # Change this to the name of the directory you want to cache (e.g., "node_modules", "build", etc.). paths: packagelock.json # Change this to the name of the file you want to cache (e.g., "packagelock.json", "Gemfile", etc.). script: # Add any additional commands here that are necessary for your project's testing process after caching has been performed (e.g., updating package versions). after_success: # This line is optional, but it can be useful if it allows you to perform some action after a successful build (eignerally sending an email notification). echo "Build completed successfully!" | mail s "Build Success" your@email.com # You can also use other services like Slack or Microsoft Teams for notifications instead of email before_deploy: # This line is optional, but it can be useful if you want to run any additional commands before your code is deployed to production (eignerally updating version numbers). echo "Preparing for deployment..." # Add any additional commands here that are necessary for your project's deployment process only: # This line is optional, but it can be useful if you want to specify which jobs should run in addition to the main job (eignerally running tests).jobs: include: test script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: master script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: develop script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: feature script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: release script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: hotfix script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: security script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: chore script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: renovate script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: style script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: checkstyle script: echo "Running tests..." # Add any additional commands here that are necessary for your project's testing process after caching has been performed (eignerally updating package versions) only: bandit script: echo "Running tests..." # Add any additional commands here that are necessary for your project(Python项目管理)# Python项目管理Python是一种广泛用于开发各种类型应用程序的高级编程语言,本篇文章将介绍如何使用Python进行项目管理,包括版本控制、依赖管理、测试和文档编写等方面。
下面是一个简单的介绍,用于概述一个名为“Python项目管理工具”的小项目,这个项目可以帮助用户管理他们的Python项目,包括项目信息、依赖关系、任务列表等。
项目信息 | 描述 |
项目名称 | Python项目管理工具 |
项目版本 | 1.0.0 |
开发语言 | Python 3.8+ |
开发框架 | Flask, SQLite, Click (命令行工具) |
代码仓库 | GitHub或GitLab私服 |
目标用户 | Python开发者,项目管理者 |
功能模块 | 功能描述 |
项目创建 | 允许用户创建新项目,并初始化项目目录和基础文件 |
依赖管理 | 支持使用pip进行依赖安装和管理 |
任务管理 | 提供任务列表,支持添加、删除、更新和标记完成等操作 |
项目设置 | 允许用户配置项目特定的设置,如环境变量、数据库连接等 |
项目文档 | 支持生成项目文档,如README、CHANGELOG等 |
代码统计 | 集成代码行数统计和代码质量分析工具 |
项目分享 | 允许用户导出项目信息和设置,便于分享和迁移 |
命令行工具 | 提供命令行接口,方便用户在终端进行项目管理 |
开发计划 | 时间表 |
需求分析 | 1周 |
系统设计 | 1周 |
环境搭建与工具选型 | 1周 |
编码实现 | 4周 |
测试与调试 | 2周 |
用户文档与使用教程 | 1周 |
发布第一个版本 | 1周 |
后续迭代与维护 | 持续进行 |
依赖与工具 | 说明 |
Flask | Web开发框架 |
SQLite | 轻量级数据库,用于存储项目数据 |
Click | 命令行接口框架,用于构建命令行工具 |
pip | Python包安装器,用于依赖管理 |
pytest | 单元测试框架,用于代码测试 |
tox | 自动化测试工具,用于测试多环境兼容性 |
Sphinx | 文档生成工具,用于生成项目文档 |
请注意,这只是一个示例介绍,实际项目细节、功能、时间表和工具选择可能根据具体需求而有所不同。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/697124.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复