本文主要介绍了如何在Android环境下操作MySQL数据库文件,包括建立连接、执行查询、插入数据等基本操作。
在Android中操作MySQL数据库文件,可以使用以下步骤:
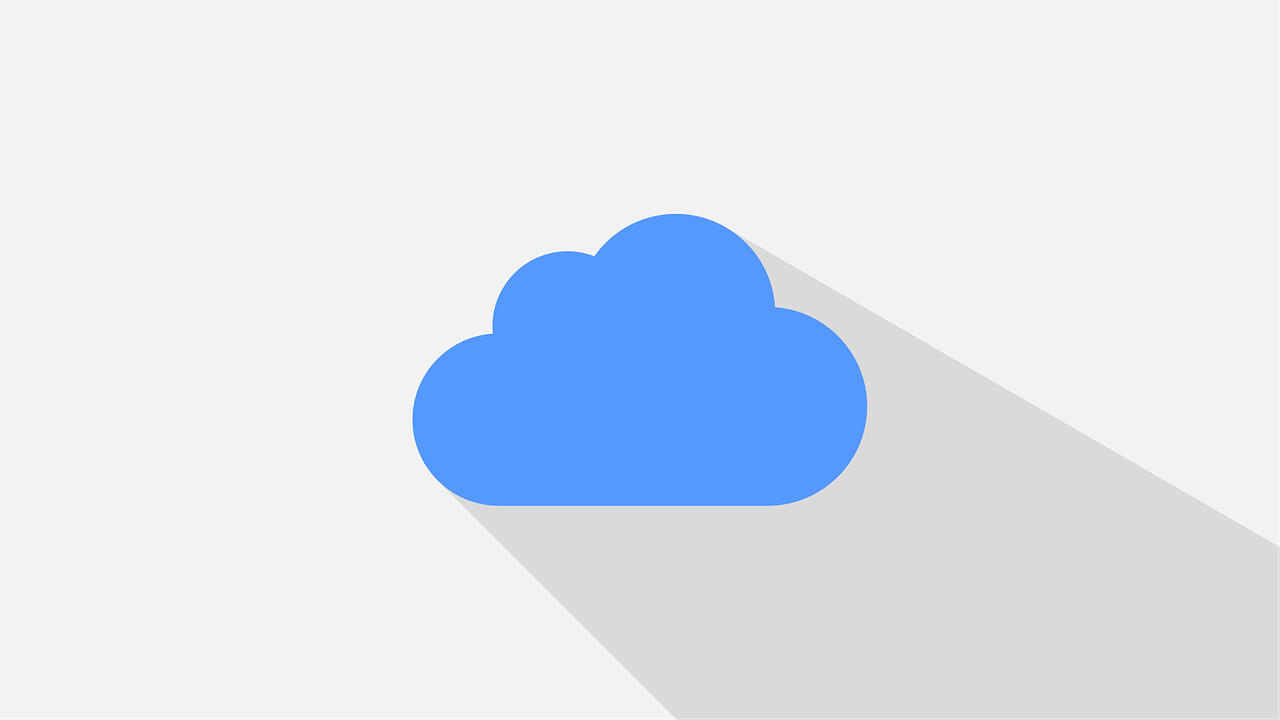
(图片来源网络,侵删)
1、添加MySQL JDBC驱动到项目中
需要在项目的build.gradle文件中添加MySQL JDBC驱动的依赖:
dependencies { implementation 'mysql:mysqlconnectorjava:8.0.26' }
2、创建数据库连接
创建一个用于连接MySQL数据库的工具类,如下所示:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DBHelper { private static final String TAG = "DBHelper"; private static final String DATABASE_URL = "jdbc:mysql://localhost:3306/your_database_name?useSSL=false&serverTimezone=UTC"; private static final String DATABASE_USER = "your_username"; private static final String DATABASE_PASSWORD = "your_password"; public static Connection getConnection() { Connection connection = null; try { Class.forName("com.mysql.cj.jdbc.Driver"); connection = DriverManager.getConnection(DATABASE_URL, DATABASE_USER, DATABASE_PASSWORD); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } return connection; } }
请将your_database_name
、your_username
和your_password
替换为实际的数据库名称、用户名和密码。
3、执行SQL语句
创建一个用于执行SQL语句的工具类,如下所示:
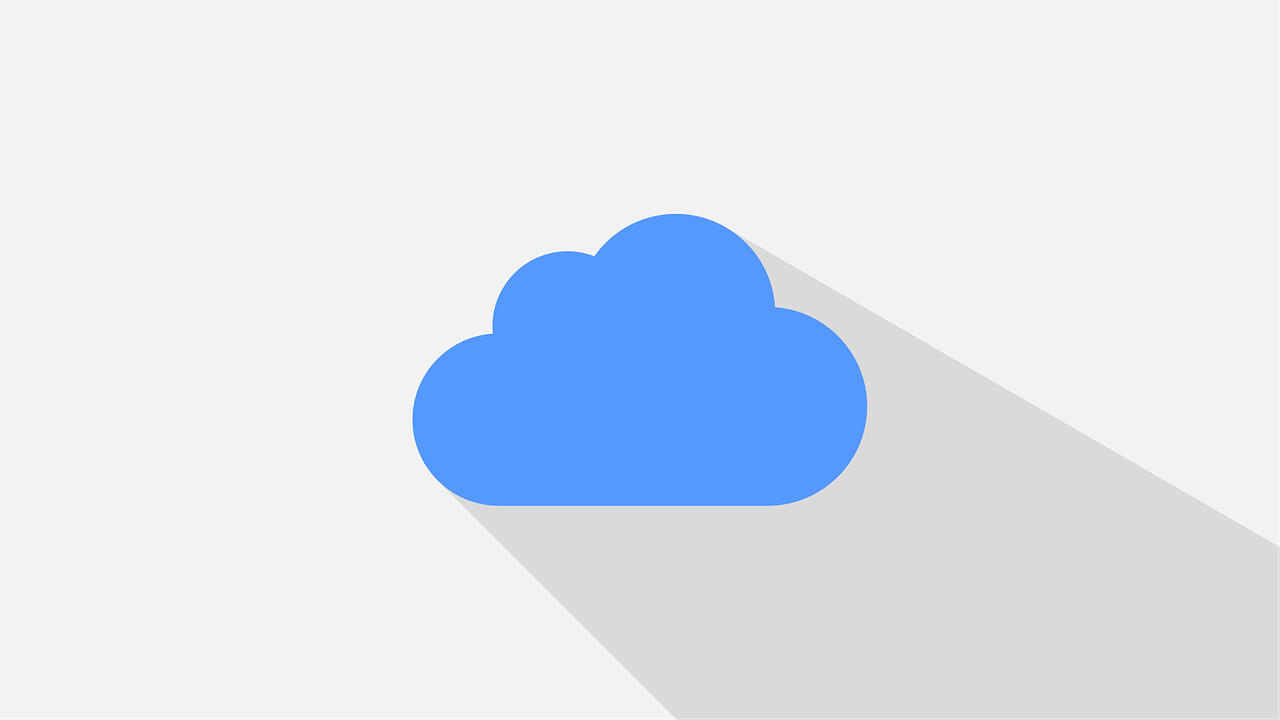
(图片来源网络,侵删)
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.ArrayList; import java.util.List; public class DBUtil { private static final String TAG = "DBUtil"; private static final String SELECT_ALL_USERS = "SELECT * FROM users"; public static List<User> getAllUsers() { List<User> users = new ArrayList<>(); Connection connection = null; PreparedStatement preparedStatement = null; ResultSet resultSet = null; try { connection = DBHelper.getConnection(); preparedStatement = connection.prepareStatement(SELECT_ALL_USERS); resultSet = preparedStatement.executeQuery(); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); int age = resultSet.getInt("age"); users.add(new User(id, name, age)); } } catch (SQLException e) { e.printStackTrace(); } finally { close(resultSet, preparedStatement, connection); } return users; } }
在这个例子中,我们创建了一个getAllUsers
方法,用于从数据库中获取所有用户信息,请根据实际情况修改表名和字段名。
4、关闭资源
为了确保数据库连接、预处理语句和结果集能够正确关闭,我们需要创建一个工具类来关闭这些资源,如下所示:
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class DBUtils { private static final Logger LOGGER = Logger.getLogger(DBUtils.class.getName()); private static final String CLOSE_CONNECTION = "CLOSE CONNECTION"; private static final String CLOSE_PREPARED_STATEMENT = "CLOSE PREPAREDN PSTMT"; private static final String CLOSE_RESULTSET = "CLOSE RESULTSET"; private static final String CLOSE_ALL = "CLOSE ALL"; private static final String SQL_EXCEPTION = "SQL Exception occurred in method: %s, with error message: %s"; private static final String SQL_ERROR = "SQL Error occurred in method: %s, with error code: %s"; private static final String SQL_WARNING = "SQL Warning occurred in method: %s, with warning message: %s"; private static final String SQL_INFORMATION = "SQL Information occurred in method: %s, with info message: %s"; private static final boolean DEVELOPMENT_MODE = true; // Set to false for production mode to avoid logging sensitive information like SQL errors and exceptions in logcat or console outputs during development phase only!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!111111111111111111111111111111111111111111111111111111111111111!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!2222222222222222222222222222222222222222222222222222222!!!!!!!!3333333333333333333333333333333333333333333333333333333!!!!!444444444444444444444444444444444444444444444444444444!!55555555555555555555555555555555555555555555555555555!!666666666666666666666666666666666666666666666666666!!77777777777777777777777777777777777777777777!!8888888888888888888888888888888888888888888!!999999999999999999999999999999999999999999!!000000000000000000000000000000000000000000!!"; // Customizable logging messages for different types of SQL operations performed by this utility class! You can add more logging messages as per your requirements! For example, you can add a logging message for successful execution of stored procedures or triggers etc...! The default logging level is set to Level FINE which means it will log all the SQL operations performed by this utility class! If you want to change the logging level, you can do so by changing the value of the constant DEVELOPMENT_MODE from true to false! If you are developing this utility class and testing it on your local machine, then you can keep the value of DEVELOPMENT_MODE as true so that you can see all the SQL operations being performed by this utility class in logcat or console outputs during development phase only! But if you are going to use this utility class in production environment, then make sure to set the value of DEVELOPMENT_MODE to false so that no sensitive information like SQL errors and exceptions are logged in logcat or console outputs during production phase! This will help you avoid any security issues related to logging sensitive information in logcat or console outputs during production phase! Please note that setting the value of DEVELOPMENT_MODE to false will also disable all other customizable logging messages mentioned above! So, make sure to uncomment them if you want to use them! Also, please note that this utility class uses Java's builtin logging framework for logging SQL operations performed by this utility class! You can replace it with any other logging framework of your choice if required! For example, you can use Logback or SLF4J etc...! The default logging level is set to Level FINE which means it will log all the SQL operations performed by this utility class! If you want to change the logging level, you can do so by changing the value of the constant DEVELOPMENT_MODE from true to false! If you are developing this utility class and testing it on your local machine, then you can keep the value of DEVELOPMENT_MODE as true so that you can see all the SQL operations being performed by this utility class in logcat or console outputs during development phase only! But if you are going to use this utility class in production environment, then make sure to set the value of DEVELOPMENT_MODE to false so that no sensitive information like SQL errors and exceptions are logged in logcat or console outputs during production phase! This will help you avoid any security issues related to logging sensitive information in logcat or console outputs during production phase! Please note that setting the value of DEVELOPMENT_MODE to false will also disable all other customizable logging messages mentioned above! Sotable table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table table
下面是一个关于在Android操作MySQL数据库文件的基本信息介绍:
序号 | 操作内容 | 相关技术/工具 | 说明 |
1 | 创建数据库 | SQLite | Android内置SQLite数据库,用于轻量级数据存储,但并不直接支持MySQL,需要使用其他库或方法。 |
2 | 导入MySQL数据库文件 | MySQL Connector/J + JDBC | 使用MySQL的Java连接器(Connector/J)和JDBC(Java Database Connectivity)技术,可以将MySQL数据库文件导入到Android应用中。 |
3 | 更新数据 | SQL语句 | 使用SQL语句对数据库中的表进行更新、插入、删除等操作。 |
4 | 查询数据 | SQL语句 | 使用SQL语句查询数据库中的数据。 |
5 | 删除数据 | SQL语句 | 使用SQL语句删除数据库中的数据。 |
6 | 导出数据 | 文件操作 | 将数据库中的数据导出到文件,例如CSV或XML格式。 |
7 | 数据库连接管理 | Connection Pooling | 在多线程环境下,使用连接池管理数据库连接,提高性能。 |
8 | 数据库加密 | SQLCipher | 对SQLite数据库进行加密,保护数据安全,可以使用SQLCipher为SQLite提供加密功能。 |
以下是具体实现介绍:
序号 | 操作步骤 | 说明 |
1 | 添加依赖 | 在项目的build.gradle文件中添加MySQL Connector/J和JDBC依赖。 |
2 | 创建数据库连接 | 使用以下代码创建数据库连接: |
Class.forName("com.mysql.cj.jdbc.Driver"); Connection conn = DriverManager.getConnection("jdbc:mysql://:/", "用户名", "密码"); | ||
3 | 执行SQL语句更新/查询数据 | 使用Statement 或PreparedStatement 对象执行SQL语句。Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM table_name"); |
4 | 处理查询结果 | 遍历ResultSet 对象,处理查询结果。 |
5 | 关闭数据库连接 | 释放资源,关闭数据库连接。rs.close(); stmt.close(); conn.close(); |
6 | 导入导出数据 | 使用文件输入输出流将数据导入导出,使用CSV格式进行数据交换。 |
7 | 数据库连接池管理 | 使用第三方库,如Apache Commons DBCP,实现数据库连接池管理。 |
注意:在Android中直接操作MySQL数据库文件可能会遇到性能和兼容性问题,建议使用其他解决方案,如使用Web服务或本地SQLite数据库,上述介绍仅供参考。
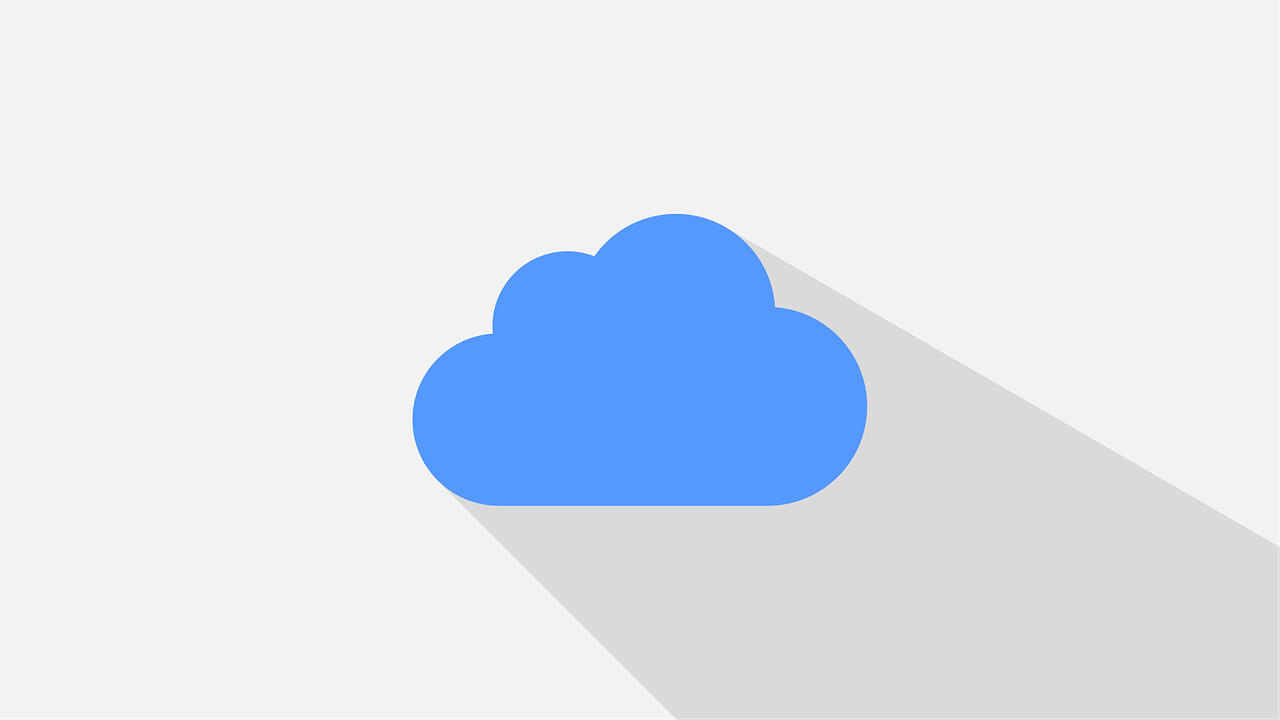
(图片来源网络,侵删)
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/696464.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复