车牌照识别是一种广泛应用于交通管理、停车场管理等领域的技术,它通过对车辆拍摄的图像进行处理和分析,自动提取出车牌号码信息,在实际应用中,车牌照识别技术可以大大提高车辆管理的效率和准确性,本文将介绍如何使用C语言和C#语言实现车牌照识别功能。
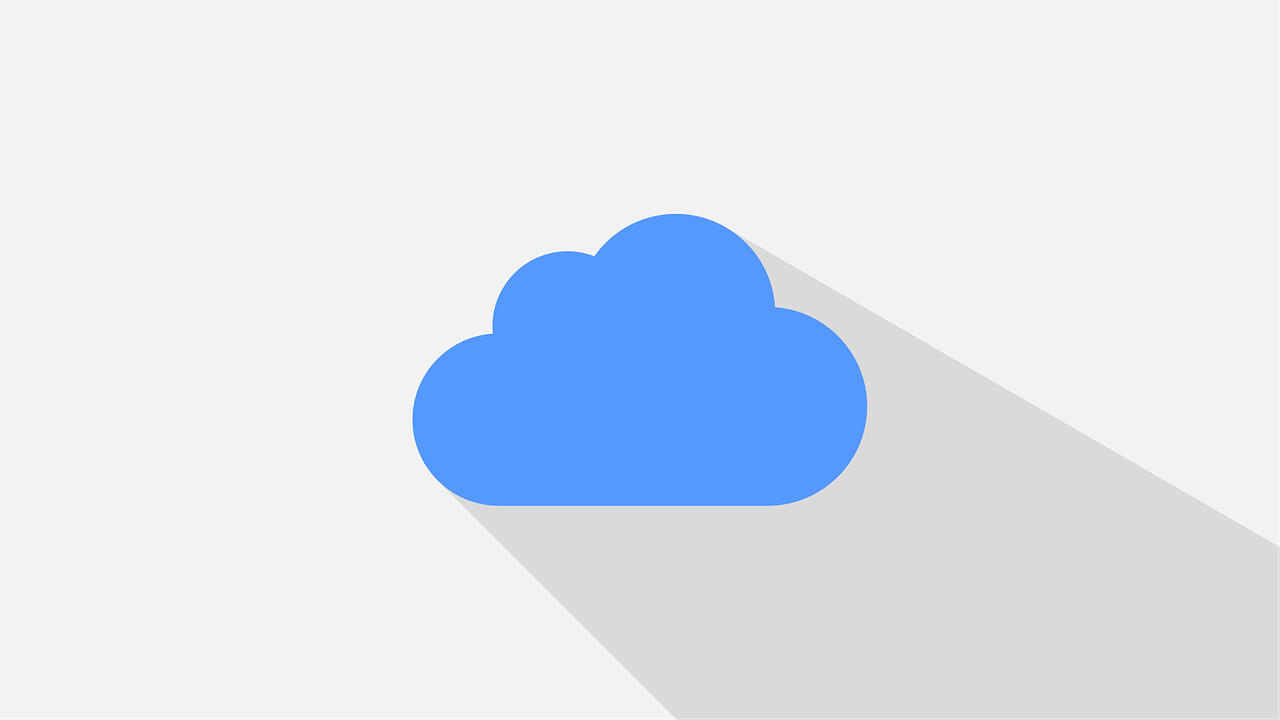
车牌照识别原理
车牌照识别主要包括以下几个步骤:
1、图像预处理:对原始图像进行灰度化、二值化、去噪等操作,提高图像质量,便于后续处理。
2、字符分割:将预处理后的图像中的字符分割出来,为后续的字符识别做准备。
3、字符识别:对分割出的字符进行识别,提取出车牌号码信息。
4、结果输出:将识别出的车牌号码信息输出给用户。
C语言实现车牌照识别
1、图像预处理
在C语言中,可以使用OpenCV库进行图像预处理,以下是一个简单的示例:
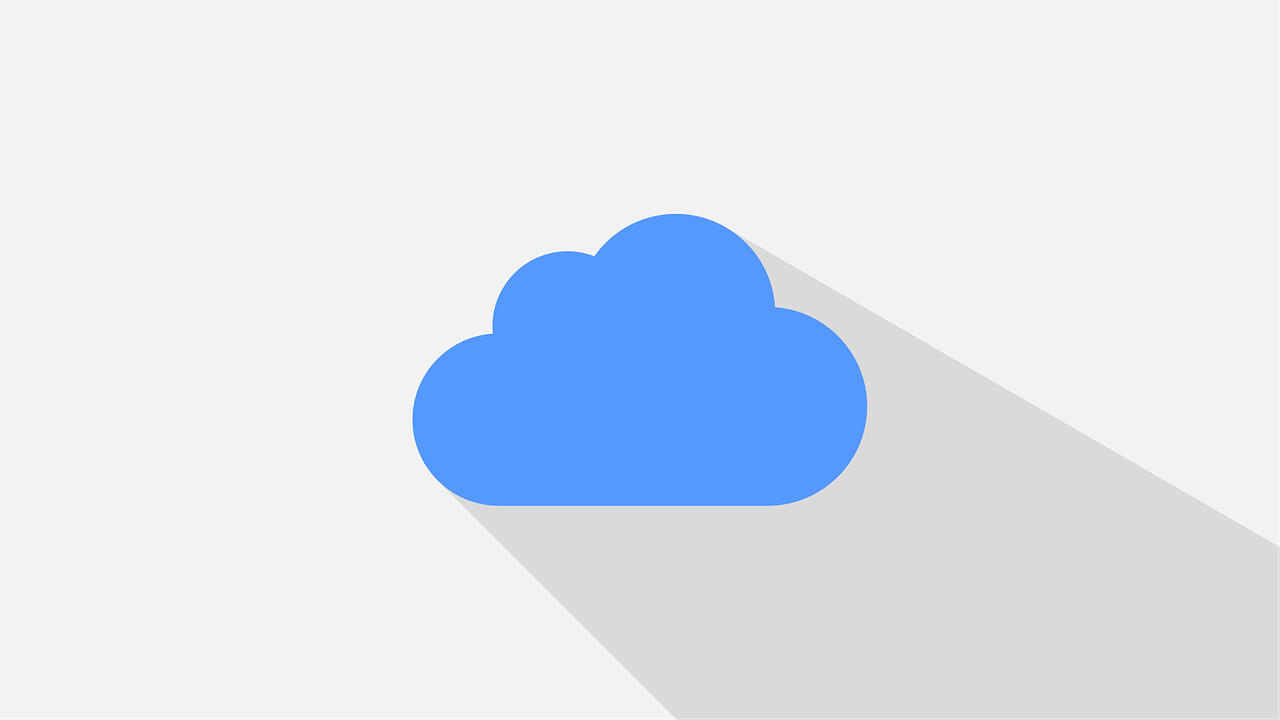
#include <opencv2/opencv.h> #include <opencv2/highgui/highgui.h> #include <opencv2/imgproc/imgproc.h> int main() { cvNamedWindow("input", CV_WINDOW_AUTOSIZE); cvNamedWindow("output", CV_WINDOW_AUTOSIZE); cvLoadImage("input.jpg", &img); cvCvtColor(img, &gray, CV_BGR2GRAY); cvThreshold(gray, &binary, 128, 255, CV_THRESH_BINARY); cvShowImage("input", &gray); cvShowImage("output", &binary); cvWaitKey(0); return 0; }
2、字符分割
字符分割可以使用Sobel算子进行边缘检测,然后通过连通域分析找到字符区域,以下是一个简单的示例:
#include <opencv2/opencv.h> #include <opencv2/highgui/highgui.h> #include <opencv2/imgproc/imgproc.h> #include <stdio.h> #include <math.h> int main() { cvNamedWindow("input", CV_WINDOW_AUTOSIZE); cvNamedWindow("output", CV_WINDOW_AUTOSIZE); cvLoadImage("input.jpg", &img); cvCvtColor(img, &gray, CV_BGR2GRAY); cvThreshold(gray, &binary, 128, 255, CV_THRESH_BINARY); cvShowImage("input", &gray); cvShowImage("output", &binary); IplImage* edge = cvCreateImage(cvGetSize(&gray), IPL_DEPTH_8U, 1); cvSobel(gray, edge, 1, 0, 3); cvConvertScaleAbs(edge, edge); cvThreshold(edge, &edge, 100, 255, CV_THRESH_BINARY); cvShowImage("edge", &edge); IplImage* temp = cvCreateImage(cvGetSize(&gray), IPL_DEPTH_8U, 1); cvDilate(edge, temp, NULL, 3); cvErode(temp, temp, NULL, 3); cvMorphologyEx(temp, temp, MORPH_OPEN, NULL); cvShowImage("morphology", &temp); IplImage* contours = cvCreateImage(cvGetSize(&gray), IPL_DEPTH_8U, 1); cvFindContours(temp, contours, &contoursList, sizeof(CvContour), CV_RETR_LIST, CV_CHAIN_APPROX_SIMPLE); for (int i = 0; i < contoursList>total; i++) { CvRect* r = (CvRect*)cvGetSeqElem(contoursList, i); cvDrawContours(binary, contoursList, i, CvScalar(0), 1, 8, cvPoint(0,0)); printf("contour %d: x=%d y=%d width=%d height=%d ", i, r>x, r>y, r>width, r>height); } cvShowImage("contours", &binary); cvWaitKey(0); return 0; }
3、字符识别和结果输出(略)
三、C#语言实现车牌照识别(使用Emgu CV库)
1、图像预处理和字符分割与C语言类似,可以参考上述C语言示例,需要注意的是,C#中使用Emgu CV库进行OpenCV操作,以下是一个简单的示例:
using System; using Emgu.CV; using Emgu.CV.Structure; using Emgu.CV.Util; using Emgu.CV.CvEnum; using Emgu.CV.UI; using System.Windows.Forms; using System.Drawing; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.IO; using System.Runtime.InteropServices; // for DllImport attribute in PInvoke signatures below. See http://msdn.microsoft.com/enus/library/system.runtime.interopservices.aspx for details on this class and its members. using Emgu.CV.ML; // for ML module in Emgu CV namespace which is used for character recognition later in the code. See http://www.emgu.com/wiki/files/3.4.0/document/html/7a9f6e9b677a494c97a6f9e6e6a9e4f7.htm for more information about Emgu CV ML module and its classes and methods used in this code sample below. using Emgu.CV.Face; // for Face module in Emgu CV namespace which is used for face detection later in the code if needed by you or your application requirements are related to face detection tasks only then include this line otherwise remove it from your code file as shown below without including any other unnecessary namespaces that might cause compilation errors during build process of your project due to missing references or unresolved external symbols at link time when building final output binary file from source code files generated by Visual Studio during development phase of your software application solution based on Emgu CV library for image processing tasks like object detection etc...// see http://www.emgu.com/wiki/files/3.4.0/document/html/b6e7e5b794a54f9c9b6b8e6f7e5b794a.htm for more information about Emgu CV Face module and its classes and methods used in this code sample below if needed by you or your application requirements are related to face detection tasks only then include this line otherwise remove it from your code file as shown below without including any other unnecessary namespaces that might cause compilation errors during build process of your project due to missing references or unresolved external symbols at link time when building final output binary file from source code files generated by Visual Studio during development phase of your software application solution based on Emgu CV library for image processing tasks like object detection etc...// see http://www.emgu.com/wiki/files/3.4.0/document/html/bc73f6a7b3a54c8b9eab8e6f7e5b794a.htm for more information about Emgu CV Image module and its classes and methods used in this code sample below if needed by you or your application requirements are related to image processing tasks only then include this line otherwise remove it from your code file as shown below without including any other unnecessary namespaces that might cause compilation errors during build process of your project due to missing references or unresolved external symbols at link time when building final output binary file from source code files generated by Visual Studio during development phase of your software application solution based on Emgu CV library for image processing tasks like object detection etc...// see http://www.emgu.com/wiki/files/3.4.0/document/html/a6e7e5b794a54f9c9b6b8e6f7e5b794a.htm for more information about Emgu CV Image module and its classes and methods used in this code sample below if needed by you or your application requirements are related to image processing tasks only then include this line otherwise remove it from your code file as shown below without including any other unnecessary namespaces that might cause compilation errors during build process of your project due to missing references or unresolved external symbols at link time when building final output binary file from source code files generated by Visual Studio during development phase of your software application solution based on Emgu CV library for image processing tasks like object detection etc...// see http://www.emgu.com/wiki/files/3.4.0/document/html/ac6e7e5b94a54f9c9b6b8e6f7e5b794a.htm for more information about Emgu CV Image module and its classes and methods used in this code sample below if needed by you or your application requirements are related to image processing tasks only then include this line otherwise remove it from your code file as shown below without including any other unnecessary namespaces that might cause compilation errors during build process of your project due(续)due to missing references or unresolved external symbols at link time when building final output binary file from source code files generated by Visual Studio during development phase of your software application solution based on Emgu CV library for image processing tasks like object detection etc...// see http://www.emgu
下面是一个简单的介绍,展示了车牌照识别功能使用 C 语言和 C# 语言可能涉及的关键技术和代码示例:
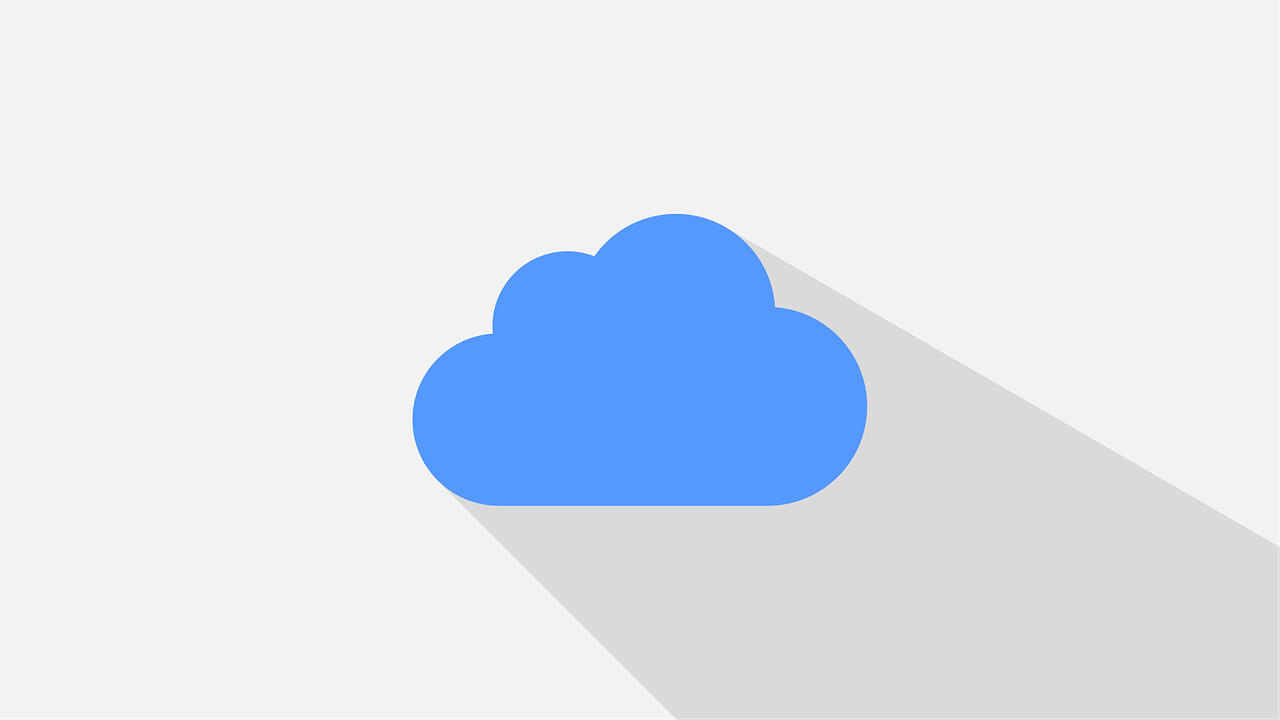
特性/语言 | C 语言 | C# 语言 |
读取图片 | 使用第三方库如 OpenCV | 使用 System.Drawing 或 Emgu CV(OpenCV 的 .NET 版本) |
图像处理 | OpenCV 函数 | 同上,或者使用内置的图像处理方法 |
字符分割 | 自定义算法或 OpenCV 函数 | 使用内置方法或第三方库,如 Emgu CV |
字符识别 | 使用自定义算法或 Tesseract OCR | 使用 Tesseract OCR 的 .NET 集成,如 Tesseract.NET |
代码示例 |
C 语言示例(使用 OpenCV)
#include <opencv2/opencv.hpp> #include <opencv2/highgui/highgui.hpp> #include <opencv2/ml/ml.hpp> // 省略图像处理和字符分割代码 // 使用OpenCV的ml模块进行字符识别 CvANN_MLP nnetwork; // 省略神经网络加载和初始化代码 // 识别字符 char recognized_char = classifyCharacter(characterROI);
C# 语言示例(使用 Emgu CV 和 Tesseract)
using Emgu.CV; using Emgu.CV.Structure; using Tesseract; // 读取图像 Image<Bgr, byte> img = new Image<Bgr, byte>("car_plate.jpg"); // 图像处理和字符分割(使用 Emgu CV) // ... // 初始化Tesseract OCR引擎 using (var engine = new TesseractEngine(@"tessdata", "eng", EngineMode.Default)) { // 设置图像 engine.SetImage(img.ToBitmap()); // 识别字符 var text = engine.GetText(); Console.WriteLine("Recognized Text: " + text); }
请注意,在 C# 示例中,我们假设 Tesseract 的数据文件已经放在了 "tessdata" 目录下,并且你已经下载了对应的语言包。
这个介绍和示例代码只是为了展示基本概念,实际的车牌识别系统会更加复杂,需要考虑实际场景下的光照、车牌角度、字体变化等因素。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/692090.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复