Python字符串操作
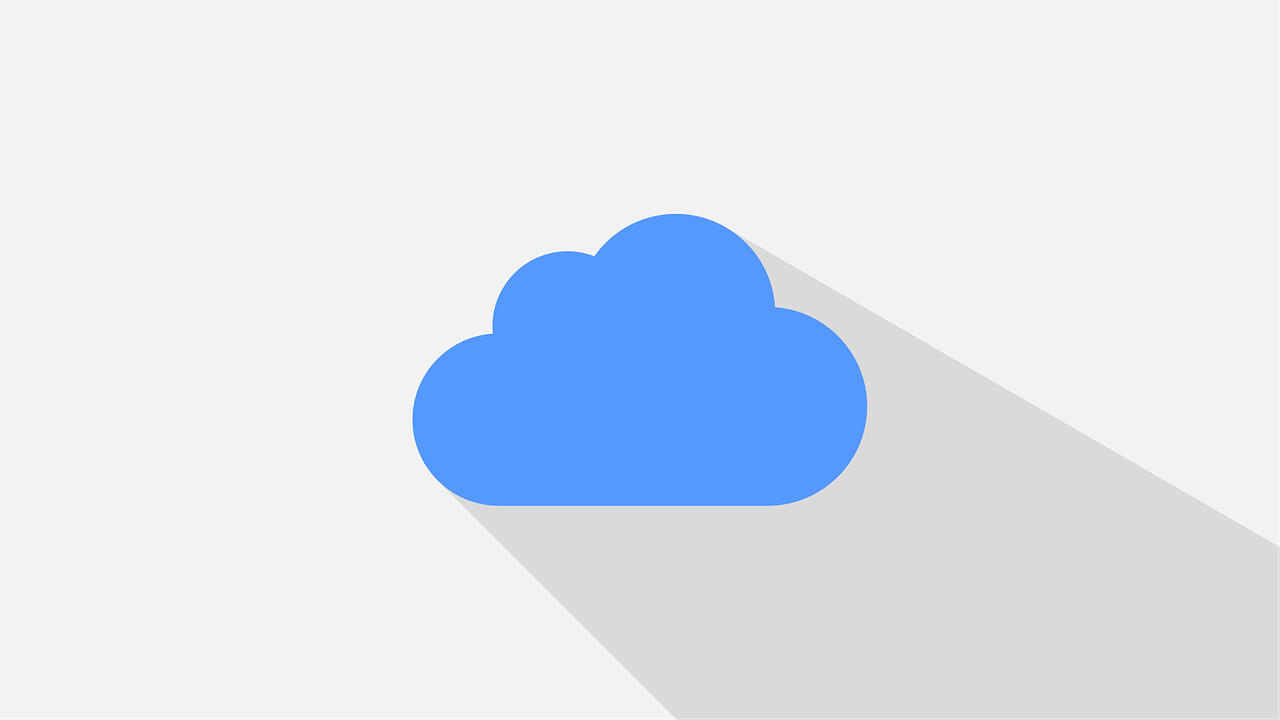
Python是一种高级编程语言,它提供了丰富的字符串操作功能,在Python中,字符串可以用单引号(’)或双引号(")括起来,本文将介绍一些常用的Python字符串操作方法,包括字符串拼接、分割、替换、查找等。
1、字符串拼接
字符串拼接是将两个或多个字符串连接在一起形成一个新的字符串,在Python中,可以使用加号(+)或者join()方法进行字符串拼接。
使用加号拼接字符串 str1 = "Hello" str2 = "World" result = str1 + " " + str2 print(result) # 输出:Hello World 使用join()方法拼接字符串 str_list = ["Hello", "World"] result = " ".join(str_list) print(result) # 输出:Hello World
2、字符串分割
字符串分割是将一个字符串按照指定的分隔符拆分成多个子字符串,在Python中,可以使用split()方法进行字符串分割。
使用split()方法分割字符串 str = "Hello,World" result = str.split(",") print(result) # 输出:['Hello', 'World']
3、字符串替换
字符串替换是将字符串中的某个子串替换为另一个子串,在Python中,可以使用replace()方法进行字符串替换。
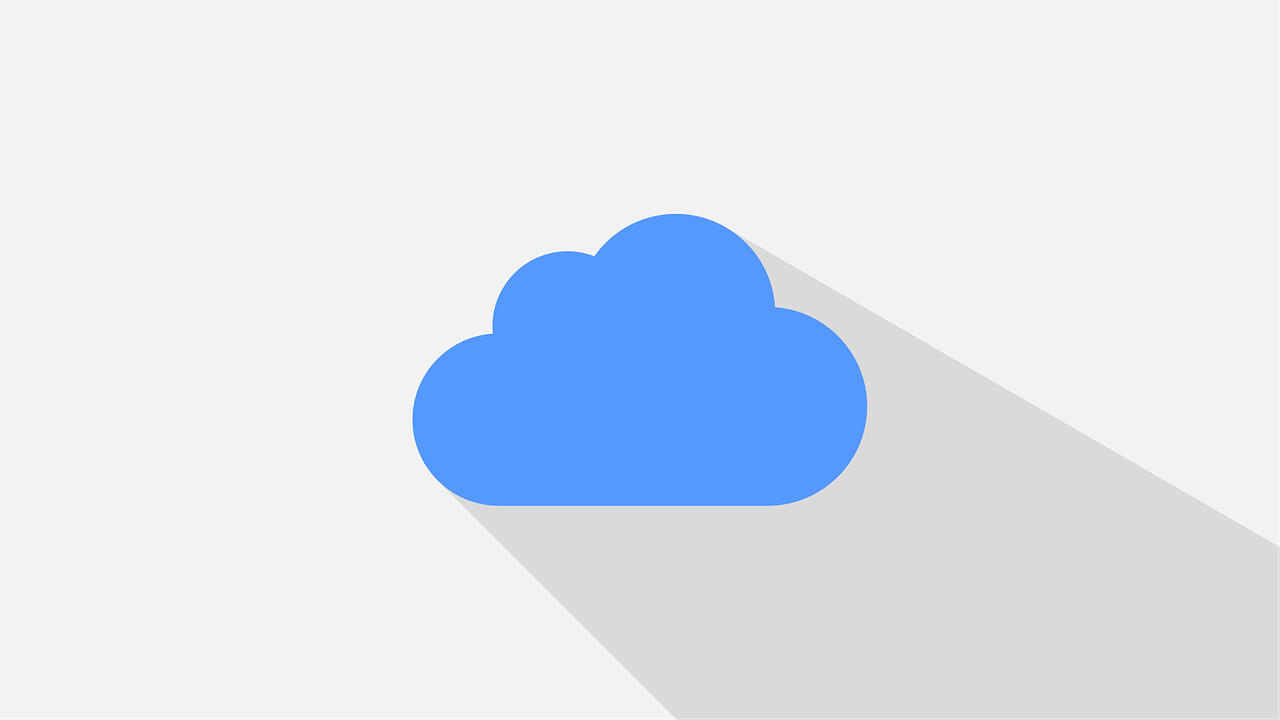
使用replace()方法替换字符串 str = "Hello,World" result = str.replace("World", "Python") print(result) # 输出:Hello,Python
4、字符串查找
字符串查找是在字符串中查找指定子串的位置,在Python中,可以使用find()方法进行字符串查找。
使用find()方法查找字符串 str = "Hello,World" result = str.find("World") print(result) # 输出:6
5、字符串大小写转换
在Python中,可以使用upper()和lower()方法将字符串转换为大写或小写。
使用upper()方法将字符串转换为大写 str = "Hello,World" result = str.upper() print(result) # 输出:HELLO,WORLD 使用lower()方法将字符串转换为小写 str = "Hello,World" result = str.lower() print(result) # 输出:hello,world
6、字符串去除空格
在Python中,可以使用strip()、lstrip()和rstrip()方法去除字符串中的空格。
使用strip()方法去除字符串两端的空格 str = " Hello,World " result = str.strip() print(result) # 输出:Hello,World 使用lstrip()方法去除字符串左侧的空格 str = " Hello,World " result = str.lstrip() print(result) # 输出:Hello,World 使用rstrip()方法去除字符串右侧的空格 str = " Hello,World " result = str.rstrip() print(result) # 输出: Hello,World
7、字符串格式化
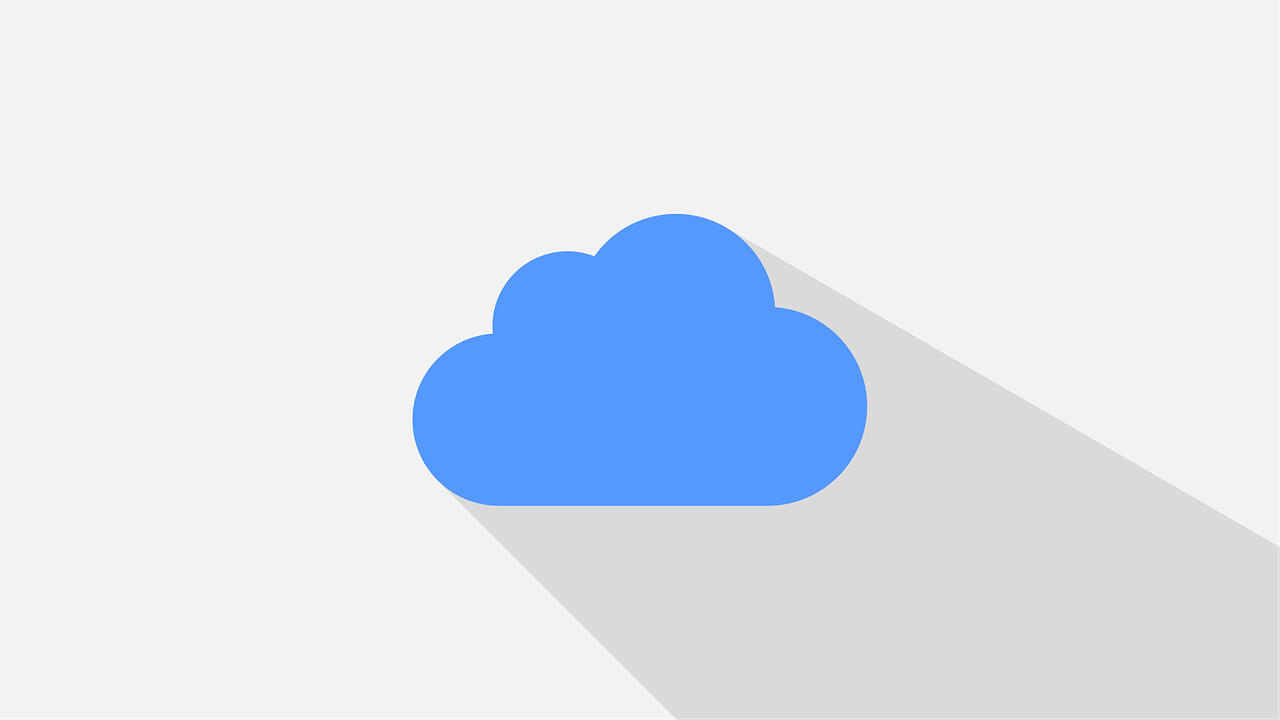
在Python中,可以使用format()方法或者fstring进行字符串格式化。
使用format()方法进行字符串格式化 name = "Tom" age = 18 result = "My name is {} and I am {} years old.".format(name, age) print(result) # 输出:My name is Tom and I am 18 years old. 使用fstring进行字符串格式化 name = "Tom" age = 18 result = f"My name is {name} and I am {age} years old." print(result) # 输出:My name is Tom and I am 18 years old.
是一些常用的Python字符串操作方法,可以帮助我们更好地处理字符串数据,在实际编程过程中,可以根据需要选择合适的方法进行字符串操作。
下面是一个关于Python字符串操作的相关命令和描述的介绍。
操作命令 | 描述 |
str1 + str2 | 字符串拼接 |
str * n 或n * str | 重复字符串n次 |
len(str) | 获取字符串长度 |
str[i] | 访问字符串中索引为i的字符 |
str[i:j] | 获取字符串中从索引i到j1的子字符串(切片) |
str[i:j:k] | 获取字符串中从索引i到j1,步长为k的子字符串(切片) |
'hello'.upper() | 将字符串转换为大写 |
'HELLO'.lower() | 将字符串转换为小写 |
'Hello'.capitalize() | 首字母大写,其余小写 |
'hello'.title() | 每个单词的首字母大写 |
str.startswith('pre') | 判断字符串是否以’pre’开头 |
str.endswith('suf') | 判断字符串是否以’suf’结尾 |
'hello'.find('el') | 查找子字符串的位置,不存在则返回1 |
'hello'.index('el') | 类似find,但找不到时会抛出ValueError |
'hello'.replace('el', 'al') | 替换字符串中的内容 |
'hello'.strip() | 去除字符串两端的空白字符 |
' hello '.lstrip() | 去除字符串左端的空白字符 |
' hello '.rstrip() | 去除字符串右端的空白字符 |
'hello'.split('l') | 按指定字符拆分字符串 |
'1,2,3'.split(',') | 按逗号拆分字符串 |
'hello'.join(['1', '2']) | 使用字符串连接列表中的元素 |
'123'.zfill(5) | 用0填充字符串到指定长度 |
'Hello'.rjust(10) | 右对齐字符串,不足部分用空格填充 |
'Hello'.ljust(10) | 左对齐字符串,不足部分用空格填充 |
'Hello'.center(10) | 居中对齐字符串,不足部分用空格填充 |
'hello'.count('l') | 计算字符串中指定字符出现的次数 |
'hello'.isalpha() | 判断字符串是否只包含字母 |
'123'.isdigit() | 判断字符串是否只包含数字 |
'Hello'.isupper() | 判断字符串中的所有字符是否都是大写 |
'hello'.islower() | 判断字符串中的所有字符是否都是小写 |
'Hello World'.istitle() | 判断字符串是否是标题化的(每个单词的首字母大写) |
这个介绍涵盖了Python中常用的字符串操作,可以帮助你在处理文本数据时更加得心应手。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/685902.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复