在Android设备上实现与服务器的加密通信,通常需要以下几个步骤:
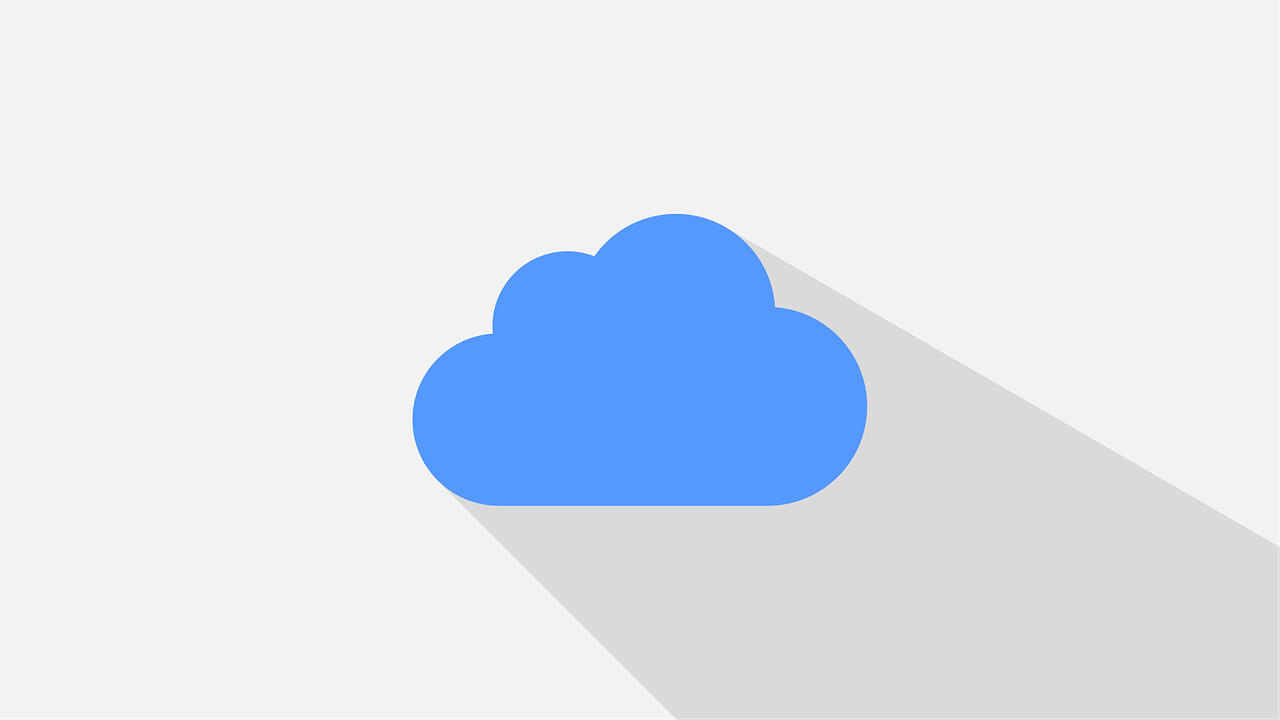
(图片来源网络,侵删)
1、创建SSLSocketFactory
2、使用SSLSocketFactory创建SSLSocket
3、通过SSLSocket发送和接收数据
以下是详细的步骤和代码示例:
1. 创建SSLSocketFactory
我们需要创建一个SSLSocketFactory,这需要使用到TrustManagerFactory和KeyStore。
import javax.net.ssl.*; import java.security.KeyStore; import java.security.cert.Certificate; import java.security.cert.CertificateFactory; // Load the certificate from an input stream CertificateFactory cf = CertificateFactory.getInstance("X.509"); InputStream caInput = new BufferedInputStream(new FileInputStream("path_to_certificate")); Certificate ca; try { ca = cf.generateCertificate(caInput); } finally { caInput.close(); } // Create a KeyStore containing our trusted CAs String keyStoreType = KeyStore.getDefaultType(); KeyStore keyStore = KeyStore.getInstance(keyStoreType); keyStore.load(null, null); keyStore.setCertificateEntry("ca", ca); // Create a TrustManager that trusts the CAs in our KeyStore String tmfAlgorithm = TrustManagerFactory.getDefaultAlgorithm(); TrustManagerFactory tmf = TrustManagerFactory.getInstance(tmfAlgorithm); tmf.init(keyStore); // Create an SSLContext with the TrustManager SSLContext context = SSLContext.getInstance("TLS"); context.init(null, tmf.getTrustManagers(), null);
2. 使用SSLSocketFactory创建SSLSocket
我们可以使用上面创建的SSLSocketFactory来创建SSLSocket。
SSLSocketFactory sslSocketFactory = context.getSocketFactory(); SSLSocket sslSocket = (SSLSocket) sslSocketFactory.createSocket("your_host", your_port);
3. 通过SSLSocket发送和接收数据
我们可以通过SSLSocket的输入输出流来发送和接收数据。
InputStream inputStream = sslSocket.getInputStream(); OutputStream outputStream = sslSocket.getOutputStream(); // Write to the server outputStream.write("Hello, Server!".getBytes()); // Read the response int bytesRead; byte[] buffer = new byte[1024]; while ((bytesRead = inputStream.read(buffer)) != 1) { System.out.println(new String(buffer, 0, bytesRead)); }
注意:以上代码只是一个基本的示例,实际使用时需要根据具体情况进行修改,你可能需要处理IOException,以及在读取服务器响应时可能需要更复杂的逻辑。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/683201.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复