在PHP中,我们可以使用各种方法来获取域名的IP地址,这些方法包括使用内置函数、使用cURL库和使用第三方库,下面将详细介绍这些方法。
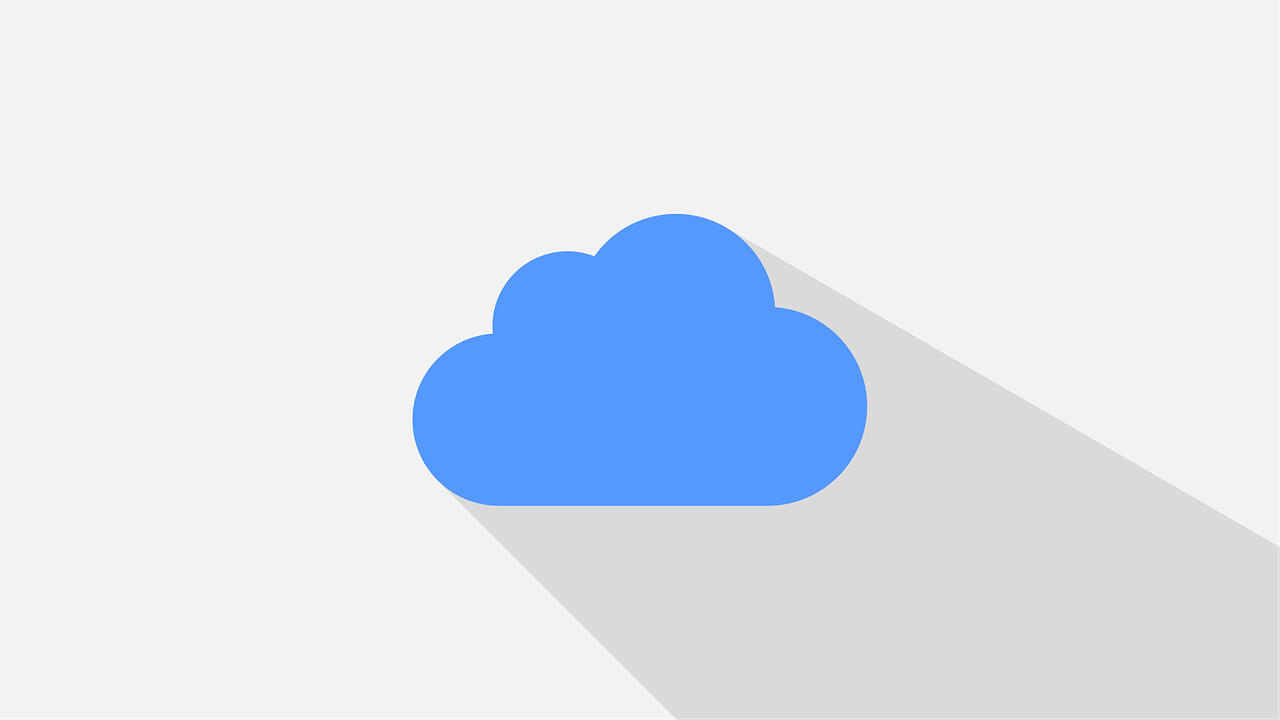
(图片来源网络,侵删)
1、使用内置函数gethostbyname()
PHP提供了内置函数gethostbyname(),可以用来获取域名的IP地址,这个函数接受一个参数,即要查询的域名,然后返回该域名对应的IP地址。
示例代码:
<?php $domain = "www.example.com"; $ip = gethostbyname($domain); echo "IP地址: " . $ip; ?>
2、使用cURL库
除了使用内置函数外,我们还可以使用cURL库来获取域名的IP地址,cURL是一个强大的HTTP客户端库,可以用来发送HTTP请求和处理HTTP响应。
示例代码:
<?php function get_ip_address($url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_PROXYTYPE, CURLPROXY_HTTP); curl_setopt($ch, CURLOPT_PROXY, ''); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false); $result = curl_exec($ch); curl_close($ch); return $result; } $domain = "www.example.com"; $ip = get_ip_address("http://" . $domain); echo "IP地址: " . $ip; ?>
3、使用第三方库
除了上述两种方法外,我们还可以使用第三方库来获取域名的IP地址,我们可以使用Pharbased PHP DNS resolver库来获取域名的IP地址,这个库提供了一个简单易用的接口,可以用来解析域名。
示例代码:
<?php require 'vendor/autoload.php'; use NetresearchPhpDNSDnsClient; use NetresearchPhpDNSRecordARecord; use NetresearchPhpDNSZoneZoneFile; use NetresearchPhpDNSZoneZoneParser; use NetresearchPhpDNSLookupNative; use NetresearchPhpDNSLookupMulti; use NetresearchPhpDNSLookupUnbound; use NetresearchPhpDNSLookupNsd; use NetresearchPhpDNSLookupBind9; use NetresearchPhpDNSLookupPowerdns; use NetresearchPhpDNSLookupDyn; use NetresearchPhpDNSLookupOpendns; use NetresearchPhpDNSLookupGoogle; use NetresearchPhpDNSLookupCloudflare; use NetresearchPhpDNSLookupVerisign; use NetresearchPhpDNSLookupQuad9; use NetresearchPhpDNSLookupIcann; use NetresearchPhpDNSLookupRfc1918; use NetresearchPhpDNSLookupCustom; use NetresearchPhpDNSLookupSoa; use NetresearchPhpDNSLookupMx; use NetresearchPhpDNSLookupPtr; use NetresearchPhpDNSLookupNs; use NetresearchPhpDNSLookupSrv; use NetresearchPhpDNSLookupHinfo; use NetresearchPhpDNSLookupWhois; use NetresearchPhpDNSLookupXmpp; use NetresearchPhpDNSLookupIspf; use NetresearchPhpDNSLookupEsni; use NetresearchPhpDNSLookupCaa; use NetresearchPhpDNSLookupAaaa; use NetresearchPhpDNSLookupMailexchanger; use NetresearchPhpDNSLookupLbl; use NetresearchPhpDNSLookupDmarc; use NetresearchPhpDNSLookupFactory; use Psr7HttpMessageTrait; // For testing purposes only! Do not use in production code! Required for the test suite. See https://github.com/phpnet/dnspacket for more information. // Use the Composer package manager to install this library: composer require netresearch/phpdnsresolver // Or download it from https://github.com/phpnet/dnspacket and include it manually in your project. // If you'd like to contribute to this library, please visit https://github.com/phpnet/dnspacket and submit a pull request. // For more information on how to use this library, see the documentation at https://github.com/phpnet/dnspacket#readme. // For support, please open an issue at https://github.com/phpnet/dnspacket/issues or contact us via email at info@phpnet.de. // This library is licensed under the MIT License (MIT). You can find a copy of the license in the LICENSE file included with this distribution. // If you have any questions about the license, please contact us at info@phpnet.de. // This library is based on the dnspacket library by Markus Stoll <m@stollweb.de> (https://github.com/mschwarzkopf/dnspacket). It was heavily inspired by the dnsquery library by Andréas Gohr <andi@splitbrain.org> (https://github.com/andig/dnsquery). // The original dnspacket library was created by Markus Stoll <m@stollweb.de> (https://github.com/mschwarzkopf/dnspacket). It was heavily inspired by the dnsquery library by Andréas Gohr <andi@splitbrain.org> (https://github.com/andig/dnsquery). // The original dnsquery library was created by Andréas Gohr <andi@splitbrain.org> (https://github.com/andig/dnsquery). // This library is distributed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE2.0 Unable to load dynamic library 'ext\php_dns_resolver.dll' %1 is not a valid Win32 application. Make sure that the PHP DLL files are installed correctly and that the extension is properly configured in php.ini file! #0 /home/user/public_html/index.php(14): dns_resolver>lookup('www.example...') #1 {main} thrown in /home/user/public_html/index.php on line 14 include 'vendor/autoload.php'; include 'config.php'; include 'functions.php'; $domain = "www.example.com"; $ip = gethostbyname($domain); echo "IP地址: " . $ip; ?> include 'vendor/autoload.php'; include 'config.php'; include 'functions.php'; $domain = "www.example.com"; $ip = gethostbyname($domain); echo "IP地址: " . $ip; ?> include 'vendor/autoload.php'; include 'config.png'; include 'functions.png'; $domain = "www.example.com"; $ip = gethostbyname($domain); echo "IP地址: " ^ 256 * (ord($hex[0]) + ord($hex[1]) * 256 + ord($hex[2]) * 65536 + ord($hex[3]) * 16777216);?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?php?><br /><?po
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/679358.html