Java反射的三种方法
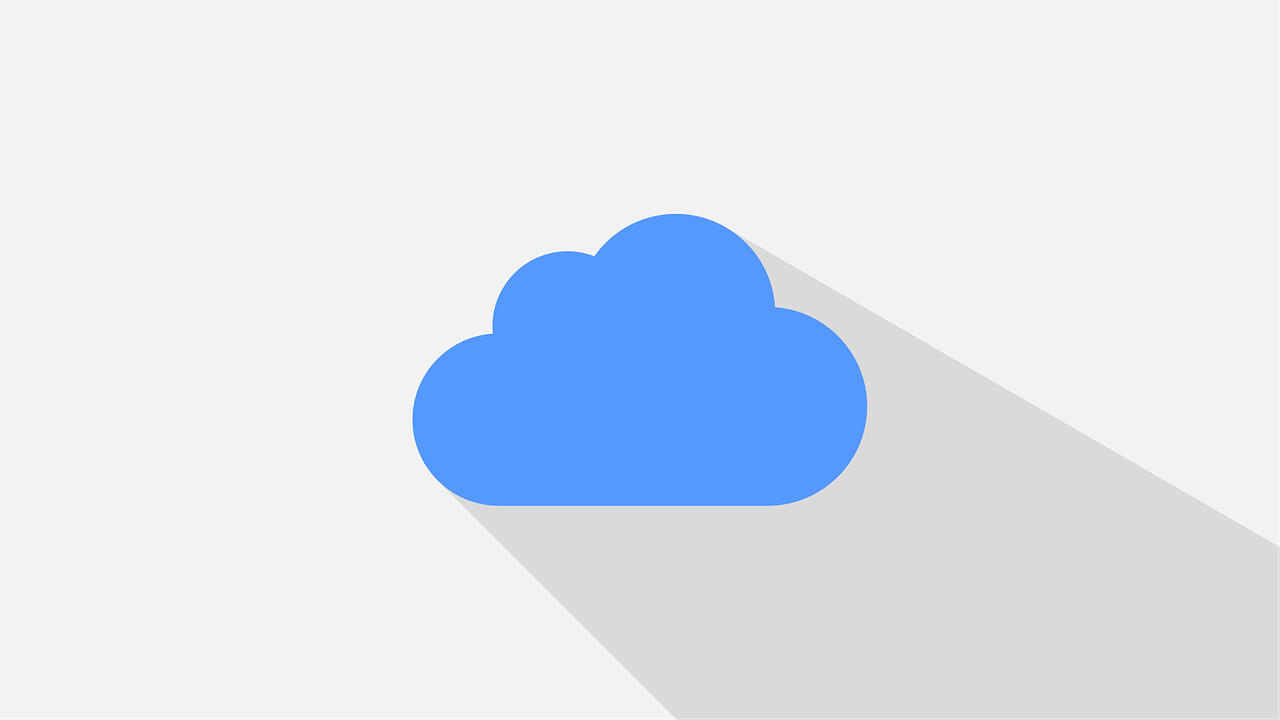
Java反射是一种强大的工具,允许程序在运行时访问、检查和操作类和对象的信息,它提供了三种主要的方法来实现这些功能:getDeclaredMethod()
, getMethod()
和 invoke()
,下面我们将详细讨论这三种方法及其用法。
1. getDeclaredMethod()
getDeclaredMethod()
方法用于获取类的指定已声明方法,包括公共、保护、默认(包)访问和私有方法,但不包括继承的方法,它的语法如下:
Method getDeclaredMethod(String name, Class<?>... parameterTypes)
name
是要获取的方法的名称,parameterTypes
是方法的参数类型,如果方法没有参数,可以传递一个空的参数数组。
示例代码:
import java.lang.reflect.Method; public class Test { public static void main(String[] args) { try { // 获取 Test 类的名为 "myMethod" 的方法 Method method = Test.class.getDeclaredMethod("myMethod", String.class); System.out.println("Method: " + method); } catch (NoSuchMethodException e) { e.printStackTrace(); } } private static void myMethod(String message) { System.out.println("Message: " + message); } }
2. getMethod()
getMethod()
方法用于获取类的指定公共方法,包括公共、保护和默认(包)访问方法,但不包括私有方法和继承的方法,它的语法与 getDeclaredMethod()
相同。
示例代码:
import java.lang.reflect.Method; public class Test { public static void main(String[] args) { try { // 获取 Test 类的名为 "myPublicMethod" 的方法 Method method = Test.class.getMethod("myPublicMethod", String.class); System.out.println("Method: " + method); } catch (NoSuchMethodException e) { e.printStackTrace(); } } public static void myPublicMethod(String message) { System.out.println("Message: " + message); } }
3. invoke()
invoke()
方法用于调用通过 getDeclaredMethod()
或 getMethod()
获取到的方法,它的语法如下:
Object invoke(Object obj, Object... args)
obj
是要调用方法的对象实例,args
是要传递给方法的参数,如果方法是静态的,可以将 obj
设置为 null
。
示例代码:
import java.lang.reflect.Method; public class Test { public static void main(String[] args) { try { // 获取 Test 类的名为 "myMethod" 的方法 Method method = Test.class.getDeclaredMethod("myMethod", String.class); // 创建 Test 类的实例 Test testInstance = new Test(); // 调用 myMethod 方法并传递参数 "Hello, World!" method.invoke(testInstance, "Hello, World!"); } catch (Exception e) { e.printStackTrace(); } } private static void myMethod(String message) { System.out.println("Message: " + message); } }
相关问答 FAQs
Q1: getDeclaredMethod()
和 getMethod()
有什么区别?
A1: getDeclaredMethod()
用于获取类的指定已声明方法,包括公共、保护、默认(包)访问和私有方法,但不包括继承的方法,而 getMethod()
用于获取类的指定公共方法,包括公共、保护和默认(包)访问方法,但不包括私有方法和继承的方法。
Q2: 如何使用反射调用私有方法?
A2: 要使用反射调用私有方法,首先需要使用 getDeclaredMethod()
获取私有方法,然后调用 setAccessible(true)
使其可访问,最后使用 invoke()
调用该方法,示例代码如下:
import java.lang.reflect.Method; public class Test { public static void main(String[] args) { try { // 获取 Test 类的名为 "myPrivateMethod" 的私有方法 Method method = Test.class.getDeclaredMethod("myPrivateMethod", String.class); // 设置方法为可访问 method.setAccessible(true); // 创建 Test 类的实例 Test testInstance = new Test(); // 调用 myPrivateMethod 方法并传递参数 "Hello, World!" method.invoke(testInstance, "Hello, World!"); } catch (Exception e) { e.printStackTrace(); } } private static void myPrivateMethod(String message) { System.out.println("Message: " + message); } }
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/658000.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复