在HTML中发送PUT和DELETE请求,通常需要使用JavaScript的XMLHttpRequest对象或者Fetch API,这里我们将详细介绍如何使用这两种方法发送PUT和DELETE请求。
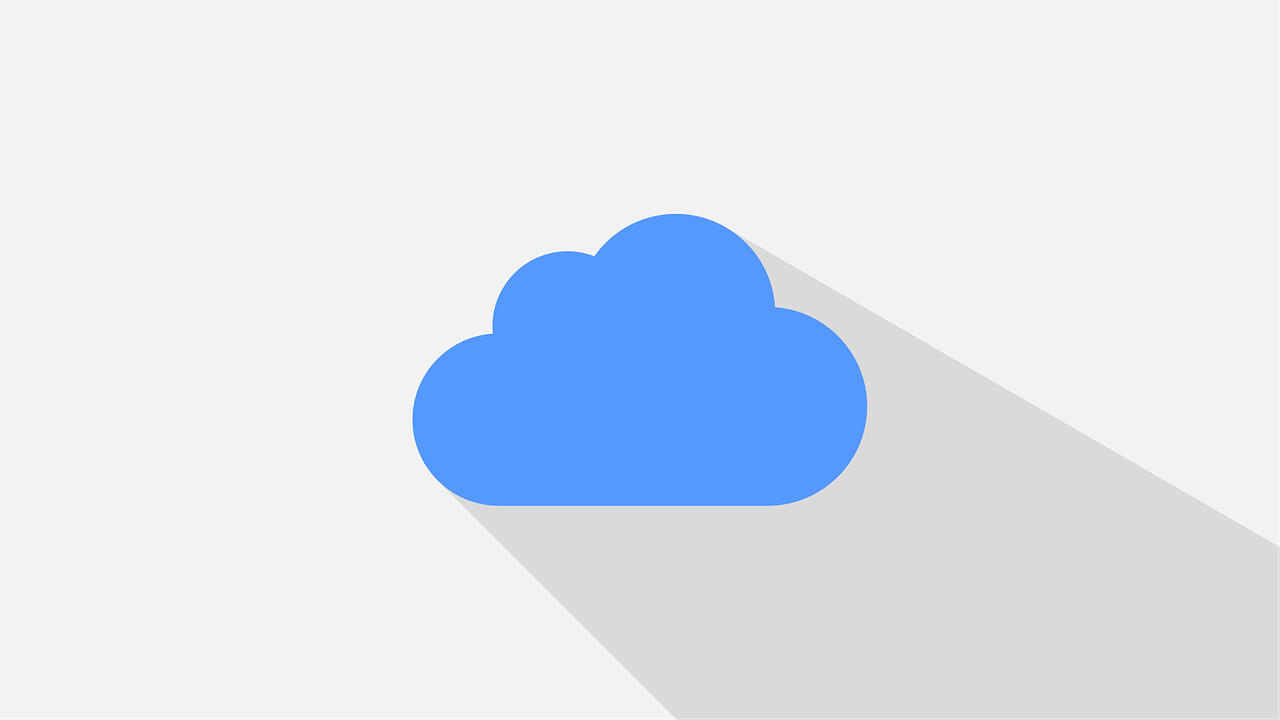
1、使用XMLHttpRequest对象发送PUT和DELETE请求
我们需要创建一个XMLHttpRequest对象,然后设置其onreadystatechange属性为一个函数,该函数将在服务器响应时被调用,我们使用open方法设置请求的类型(PUT或DELETE)和URL,最后使用send方法发送请求。
示例代码如下:
<script> function sendPutRequest() { var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { console.log("PUT request sent successfully"); } }; xhr.open("PUT", "https://example.com/api/resource", true); xhr.setRequestHeader("ContentType", "application/json;charset=UTF8"); xhr.send(JSON.stringify({key: "value"})); } function sendDeleteRequest() { var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { console.log("DELETE request sent successfully"); } }; xhr.open("DELETE", "https://example.com/api/resource", true); xhr.send(); } </script>
2、使用Fetch API发送PUT和DELETE请求
Fetch API提供了一个全局的方法fetch,这个方法可以用来替代XMLHttpRequest对象,使用fetch方法发送PUT和DELETE请求的基本步骤与使用XMLHttpRequest对象类似,但是语法更加简洁。
示例代码如下:
<script> function sendPutRequest() { fetch('https://example.com/api/resource', { method: 'PUT', headers: { 'ContentType': 'application/json' }, body: JSON.stringify({key: "value"}) }) .then(response => response.json()) .then(data => console.log('PUT request sent successfully')) .catch((error) => console.error('Error:', error)); } function sendDeleteRequest() { fetch('https://example.com/api/resource', { method: 'DELETE', }) .then(response => response.json()) .then(data => console.log('DELETE request sent successfully')) .catch((error) => console.error('Error:', error)); } </script>
以上就是在HTML中发送PUT和DELETE请求的方法。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/477274.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复