要使用Python编写爬虫,可以按照以下步骤进行:
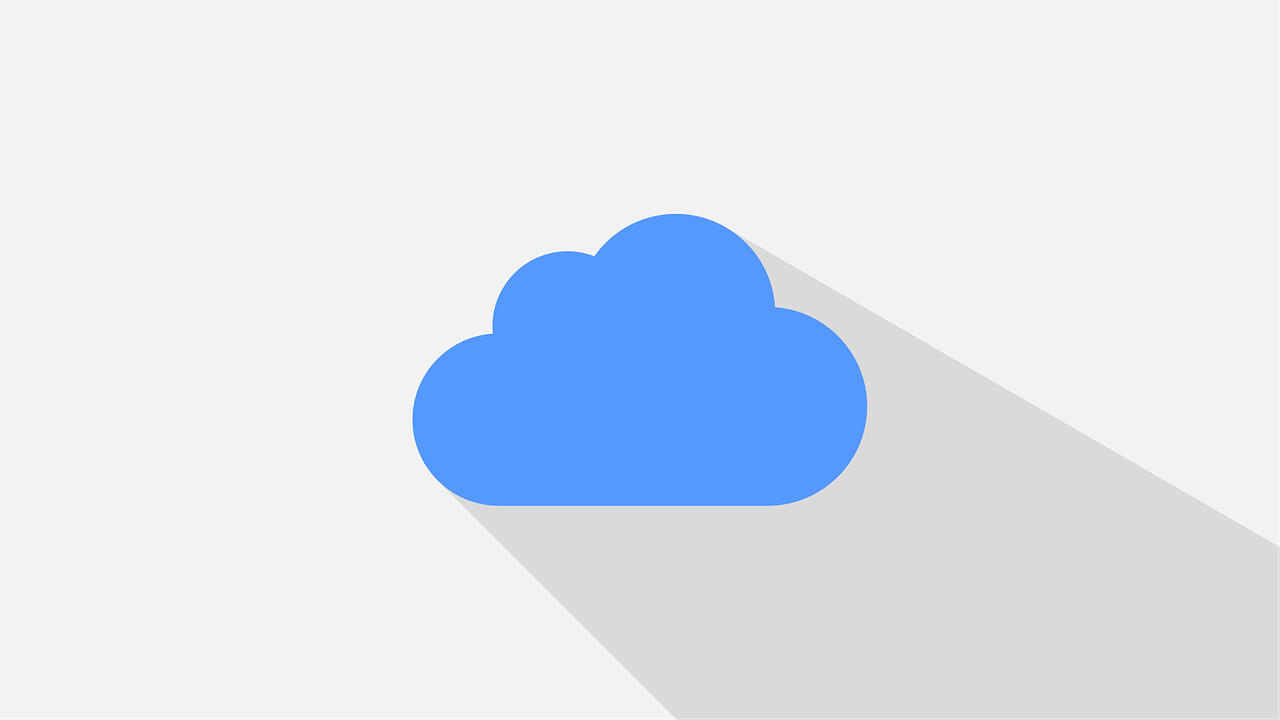
1、安装所需库
确保已经安装了Python,需要安装一些常用的库,如requests
和BeautifulSoup
,可以使用以下命令安装这些库:
“`
pip install requests
pip install beautifulsoup4
“`
2、导入所需库
在Python脚本中,导入所需的库:
“`python
import requests
from bs4 import BeautifulSoup
“`
3、发送HTTP请求
使用requests
库发送HTTP请求,获取网页的HTML内容,要获取百度首页的内容,可以使用以下代码:
“`python
url = ‘https://www.baidu.com’
response = requests.get(url)
html_content = response.text
“`
4、解析HTML内容
使用BeautifulSoup
库解析HTML内容,提取所需的信息,要提取网页中的所有标题(<h1>
标签),可以使用以下代码:
“`python
soup = BeautifulSoup(html_content, ‘html.parser’)
titles = soup.find_all(‘h1’)
for title in titles:
print(title.text)
“`
5、处理数据
根据需求对提取到的数据进行处理,可以将数据保存到文件或数据库中,或者进行进一步的分析。
6、循环爬取多个页面
如果需要爬取多个页面,可以使用循环结构,要爬取百度搜索结果的第一页,可以使用以下代码:
“`python
base_url = ‘https://www.baidu.com/s?wd=’
keyword = ‘Python’
for i in range(0, 10): # 爬取前10个结果
search_url = base_url + keyword + ‘&pn=’ + str(i * 10)
response = requests.get(search_url)
html_content = response.text
# 解析HTML内容并处理数据…
“`
7、设置爬取速度和反爬策略
为了避免被封禁IP,需要设置合适的爬取速度,可以使用time.sleep()
函数来控制爬取速度,还可以设置UserAgent、Referer等请求头信息,以模拟正常浏览器访问。
“`python
headers = {
‘UserAgent’: ‘Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3’,
‘Referer’: ‘https://www.baidu.com’
}
response = requests.get(search_url, headers=headers)
“`
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/469741.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复