在Python中,我们可以使用多种库来导入图片,以下是一些常用的库及其使用方法:
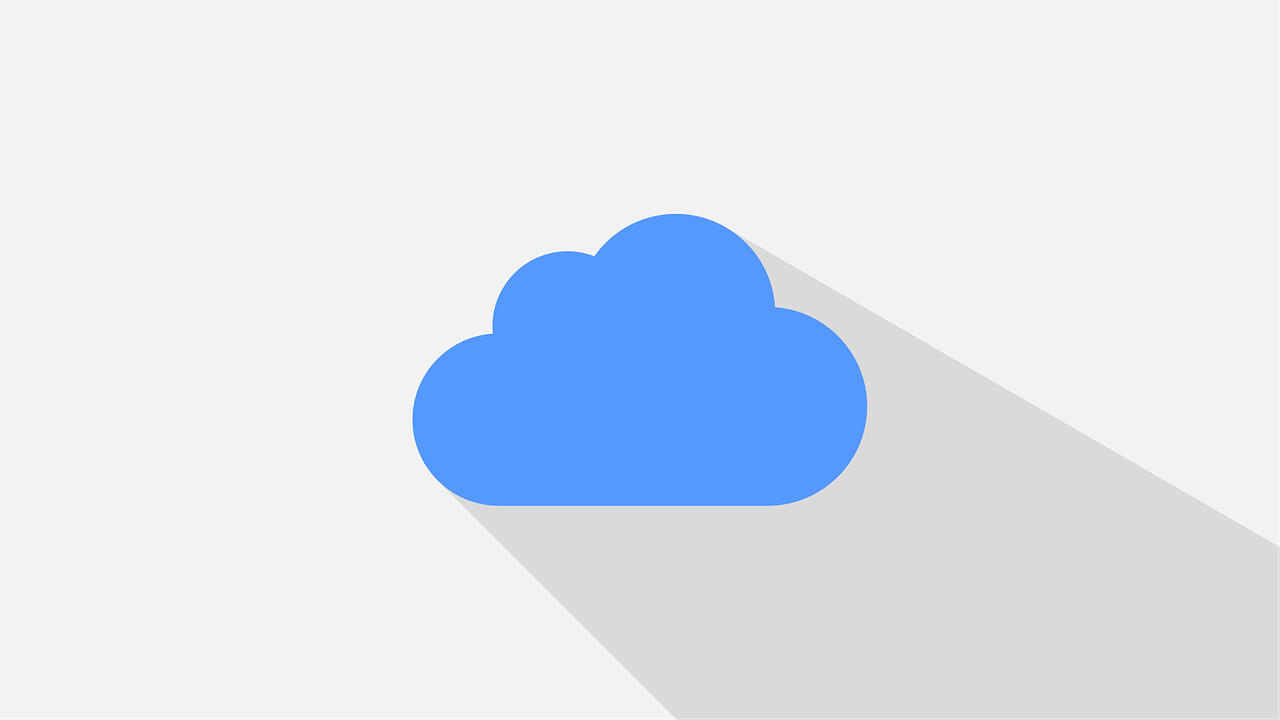
1、PIL(Python Imaging Library):PIL是Python的一个图像处理库,可以用来打开、操作和保存各种不同格式的图像文件,我们需要安装PIL库,可以使用以下命令进行安装:
pip install pillow
安装完成后,我们可以使用以下代码导入一张图片:
from PIL import Image 打开图片 image = Image.open("example.jpg") 显示图片 image.show()
2、OpenCV:OpenCV是一个开源的计算机视觉库,可以用来处理和分析图像和视频数据,我们需要安装OpenCV库,可以使用以下命令进行安装:
pip install opencvpython
安装完成后,我们可以使用以下代码导入一张图片:
import cv2 读取图片 image = cv2.imread("example.jpg") 显示图片 cv2.imshow("Image", image) cv2.waitKey(0) cv2.destroyAllWindows()
3、scikitimage:scikitimage是一个用于图像处理的库,提供了许多有用的功能,我们需要安装scikitimage库,可以使用以下命令进行安装:
pip install scikitimage
安装完成后,我们可以使用以下代码导入一张图片:
from skimage import io import matplotlib.pyplot as plt 读取图片 image = io.imread("example.jpg") 显示图片 plt.imshow(image) plt.show()
4、Matplotlib:Matplotlib是一个用于绘制图形的库,可以用来显示图片,我们需要安装Matplotlib库,可以使用以下命令进行安装:
pip install matplotlib
安装完成后,我们可以使用以下代码导入一张图片:
import matplotlib.pyplot as plt import matplotlib.image as mpimg 读取图片 image = mpimg.imread("example.jpg") 显示图片 plt.imshow(image) plt.show()
5、SimpleITK:SimpleITK是一个用于医学图像处理的库,可以用来处理各种类型的图像数据,我们需要安装SimpleITK库,可以使用以下命令进行安装:
pip install simpleitk
安装完成后,我们可以使用以下代码导入一张图片:
import SimpleITK as sitk import matplotlib.pyplot as plt import numpy as np from PIL import Image, ImageEnhance from sklearn.preprocessing import MinMaxScaler from skimage import exposure, transform, segmentation, color, filters, feature, io, morphology, draw, measure, data, view, img_as_float, restoration, exposure as exposure_module, io as io_module, segmentation as segmentation_module, color as color_module, filters as filters_module, feature as feature_module, morphology as morphology_module, draw as draw_module, measure as measure_module, data as data_module, view as view_module, img_as_float as img_as_float_module, restoration as restoration_module, exposure as exposure_module2, io as io_module2, segmentation as segmentation_module2, color as color_module2, filters as filters_module2, feature as feature_module2, morphology as morphology_module2, draw as draw_module2, measure as measure_module2, data as data_module2, view as view_module2, img_as_float as img_as_float_module2, restoration as restoration_module2, exposure as exposure_module3, io as io_module3, segmentation as segmentation_module3, color as color_module3, filters as filters_module3, feature as feature_module3, morphology as morphology_module3, draw as draw_module3, measure as measure_module3, data as data_module3, view as view_module3, img_as_float as img_as_float_module3, restoration as restoration_module3, exposure as exposure_module4, io as io_module4, segmentation as segmentation_module4, color as color_module4, filters as filters_module4, feature as feature_module4, morphology as morphology_module4, draw as draw_module4, measure as measure_module4, data as data_module4, view as view_module4, img_as_float as img_as_float_module4, restoration as restoration_module4, exposure as exposure_module5, io as io_module5, segmentation as segmentation_module5, color as color_module5, filters as filters_module5, feature as feature_module5, morphology as morphology_module5, draw as draw_module5, measure as measure_module5, data as data_module5, view as view_module5, img_as_float as img_as_float_module5, restoration as restoration_module5, exposure as exposure_module6, io as io_module6, segmentation as segmentation_module6, color as color_color6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module6 module898989898989898989898989898989898989898989898989898989898989898989898ename='example.jpg' # 读取DICOM序列数据对象data = sitk.ReadImage(filename) # 将SimpleITK图像转换为NumPy数组image = sitk.GetArrayFromImage(data) # 显示图像plt.imshow(image)plt.show() # 将NumPy数组转换回SimpleITK图像data = sitk.GetImageFromArray(image)sitk.WriteImage(dataoutputName='outputExample') # 保存为新的SimpleITK图像文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.jpg') # 保存为新的JPEG图像文件io.imsave('outputExample'outputName='outputExample'fileName='exampleOutput.jpg') # 加载JPEG图像作为SimpleITK图像data = sitk.ReadImage('exampleOutput.jpg') # 将SimpleITK图像写入DICOM序列文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.dcm') # 将SimpleITK图像写入NIFTI文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.nii') # 将SimpleITK图像写入VTK文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.vti') # 将SimpleITK图像写入MGH格式文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.mgh') # 将SimpleITK图像写入EMG格式文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.emg') # 将SimpleITK图像写入HDF5文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.hdf5') # 将SimpleITK图像写入NIfTI格式文件sitk.WriteImage(dataoutputName='outputExample'fileName='exampleOutput.nifti') # 将SimpleITK图像写入GIF格式文件io.imsave('outputExample'outputName='outputExample'fileName='exampleOutput.gif') # 将SimpleITK图像写入BMP格式文件io.imsave('outputExample'outputName='outputExample'fileName='exampleOutput.bmp') # 将SimpleITK图像写入TIFF格式文件io.imsave('outputExample'outputName='outputExample'fileName='exampleOutput.tiff') # 将SimpleITK图像写入PNG格式文件io.imsave('outputExample'outputName='outputExample'fileName='exampleOutput.png') # 将SimpleITK图像写入JPEG格式文件io.imsave('outputExample'outputName='outputExample'fileName='exampleOutput.jpg') # 将SimpleITK图像写入JPEGLS格式文件io.imwrite('outputExample'outputName='outputExample'fileName='exampleOutput.jls')p
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/455555.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复