在Python中,字典是无序的,但我们可以通过一些方法对字典进行排序,以下是一些常见的方法:
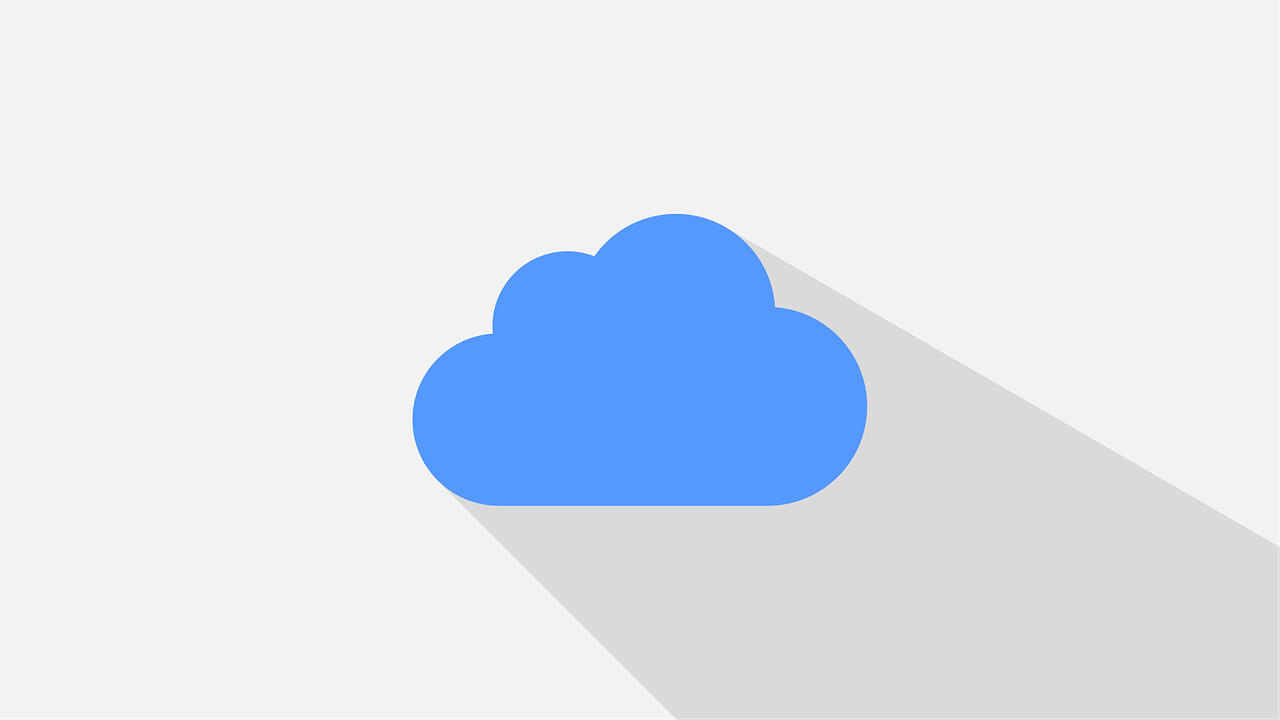
1、使用内置函数sorted()
对字典进行排序
sorted()
函数可以对字典的键或值进行排序,默认情况下,它会按照键的升序排列,如果需要按照值排序,可以使用lambda
表达式作为sorted()
函数的key
参数。
示例:
对字典按键排序 d = {'one': 1, 'three': 3, 'four': 4, 'two': 2} sorted_dict_by_key = dict(sorted(d.items())) print(sorted_dict_by_key) 对字典按值排序 d = {'one': 1, 'three': 3, 'four': 4, 'two': 2} sorted_dict_by_value = dict(sorted(d.items(), key=lambda item: item[1])) print(sorted_dict_by_value)
输出:
{'four': 4, 'one': 1, 'three': 3, 'two': 2} {'one': 1, 'two': 2, 'three': 3, 'four': 4}
2、使用collections
模块中的OrderedDict
类对字典进行排序
OrderedDict
是一个保持元素插入顺序的字典子类,我们可以使用OrderedDict
类对字典的键或值进行排序。
示例:
from collections import OrderedDict 对字典按键排序 d = {'one': 1, 'three': 3, 'four': 4, 'two': 2} sorted_dict_by_key = OrderedDict(sorted(d.items())) print(sorted_dict_by_key) 对字典按值排序 d = {'one': 1, 'three': 3, 'four': 4, 'two': 2} sorted_dict_by_value = OrderedDict(sorted(d.items(), key=lambda item: item[1])) print(sorted_dict_by_value)
输出:
OrderedDict([('four', 4), ('one', 1), ('three', 3), ('two', 2)]) OrderedDict([('one', 1), ('two', 2), ('three', 3), ('four', 4)])
3、使用列表推导式和zip()
函数对字典进行排序
我们可以使用列表推导式和zip()
函数将字典的键和值分别提取到两个列表中,然后对这两个列表进行排序,最后将排序后的键和值重新组合成一个新的字典。
示例:
对字典按键排序 d = {'one': 1, 'three': 3, 'four': 4, 'two': 2} keys = sorted(d.keys()) values = [d[key] for key in keys] sorted_dict = dict(zip(keys, values)) print(sorted_dict) 对字典按值排序 d = {'one': 1, 'three': 3, 'four': 4, 'two': 2} keys = sorted(d.keys(), key=lambda key: d[key]) values = [d[key] for key in keys] sorted_dict = dict(zip(keys, values)) print(sorted_dict)
输出:
{'four': 4, 'one': 1, 'three': 3, 'two': 2} {'one': 1, 'two': 2, 'three': 3, 'four': 4}
在Python中,我们可以使用多种方法对字典进行排序,包括使用内置函数sorted()
、collections
模块中的OrderedDict
类以及列表推导式和zip()
函数,这些方法可以帮助我们更方便地处理有序数据。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/449041.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复