在iOS开发中,我们经常需要解析HTML标签以获取其中的内容,为了实现这个功能,我们可以使用多种方法,如正则表达式、NSAttributedString等,在这里,我们将详细介绍如何使用Foundation框架中的NSAttributedString类来解析HTML标签。
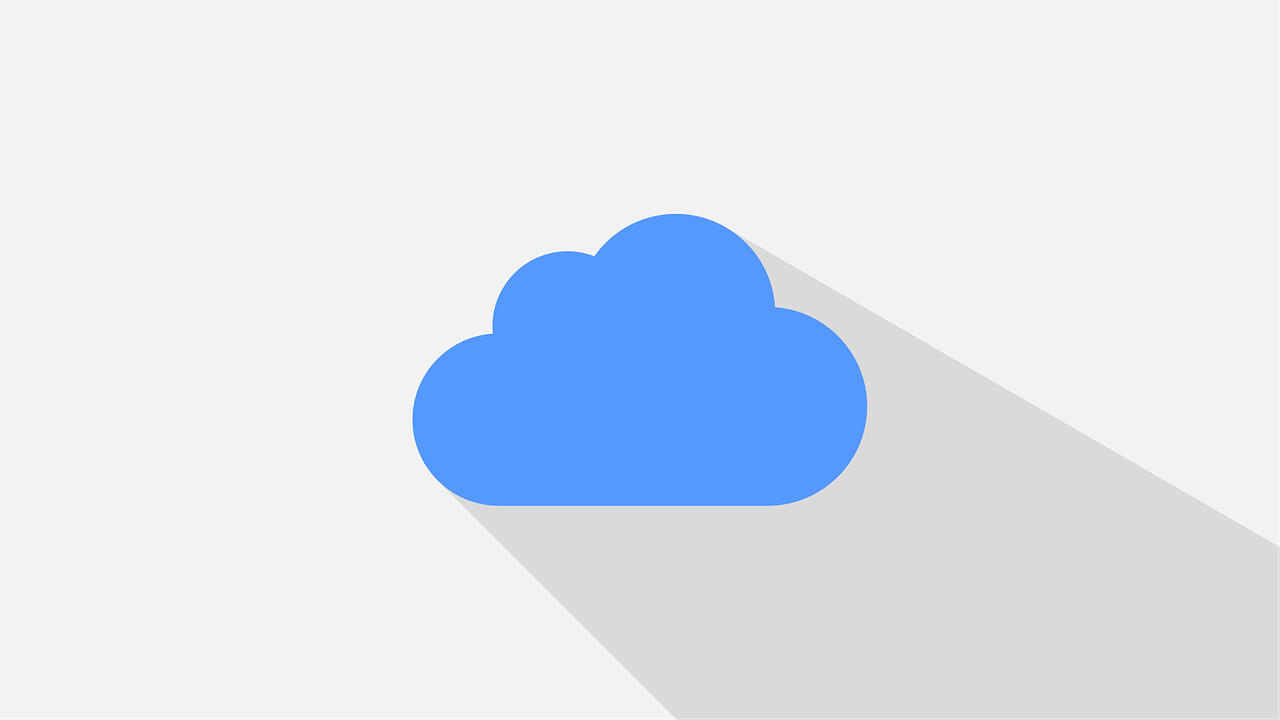
我们需要导入Foundation框架:
#import <Foundation/Foundation.h>
接下来,我们创建一个名为HTMLParser
的类,该类将负责解析HTML标签:
@interface HTMLParser : NSObject + (NSAttributedString *)attributedStringFromHTML:(NSString *)html; @end
我们在HTMLParser.m
文件中实现attributedStringFromHTML:
方法:
#import "HTMLParser.h" @implementation HTMLParser + (NSAttributedString *)attributedStringFromHTML:(NSString *)html { NSError *error = nil; NSAttributedString *attributedString = [[NSAttributedString alloc] initWithData:[html dataUsingEncoding:NSUTF8StringEncoding] options:@{NSDocumentTypeDocumentAttribute: NSHTMLTextDocumentType, NSCharacterEncodingDocumentAttribute: @(NSUTF8StringEncoding)} documentAttributes:nil error:&error]; if (error) { NSLog(@"Error parsing HTML: %@", error.localizedDescription); } return attributedString; } @end
现在,我们可以在其他类中使用HTMLParser
来解析HTML标签了,在一个名为ViewController
的类中,我们可以这样使用:
#import "ViewController.h" #import "HTMLParser.h" @interface ViewController () <UIWebViewDelegate> @property (weak, nonatomic) IBOutlet UIWebView *webView; @property (strong, nonatomic) NSString *htmlContent; @end @implementation ViewController (void)viewDidLoad { [super viewDidLoad]; self.htmlContent = @"<p>这是一个<b>示例</b>文本。</p>"; self.webView.delegate = self; [self.webView loadHTMLString:self.htmlContent baseURL:nil]; } (void)webViewDidFinishLoad:(UIWebView *)webView { NSAttributedString *attributedString = [HTMLParser attributedStringFromHTML:self.htmlContent]; NSMutableAttributedString *mutableAttributedString = [[NSMutableAttributedString alloc] initWithAttributedString:attributedString]; [mutableAttributedString addAttribute:NSForegroundColorAttributeName value:[UIColor redColor] range:NSMakeRange(0, mutableAttributedString.length)]; // 设置字体颜色为红色 [self.webView loadHTMLString:[mutableAttributedString string] baseURL:nil]; } @end
在上面的代码中,我们首先创建了一个名为htmlContent
的字符串变量,用于存储HTML内容,我们在viewDidLoad
方法中加载HTML内容到UIWebView
中,当UIWebView
加载完成时,我们调用HTMLParser
的attributedStringFromHTML:
方法来解析HTML标签,并将结果存储在attributedString
变量中,我们创建一个可变的NSMutableAttributedString
对象,并为其添加一个红色字体颜色属性,我们将修改后的字符串重新加载到UIWebView
中。
通过这种方法,我们可以在iOS应用中轻松地解析HTML标签,需要注意的是,这种方法仅适用于简单的HTML内容,对于复杂的HTML结构,可能需要使用其他第三方库或自己编写解析器来实现更精确的解析。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/449015.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复