要模拟用户访问点击网页,可以使用Python的requests库和BeautifulSoup库,requests库用于发送HTTP请求,获取网页内容;BeautifulSoup库用于解析网页内容,提取所需信息,以下是详细的技术教学:
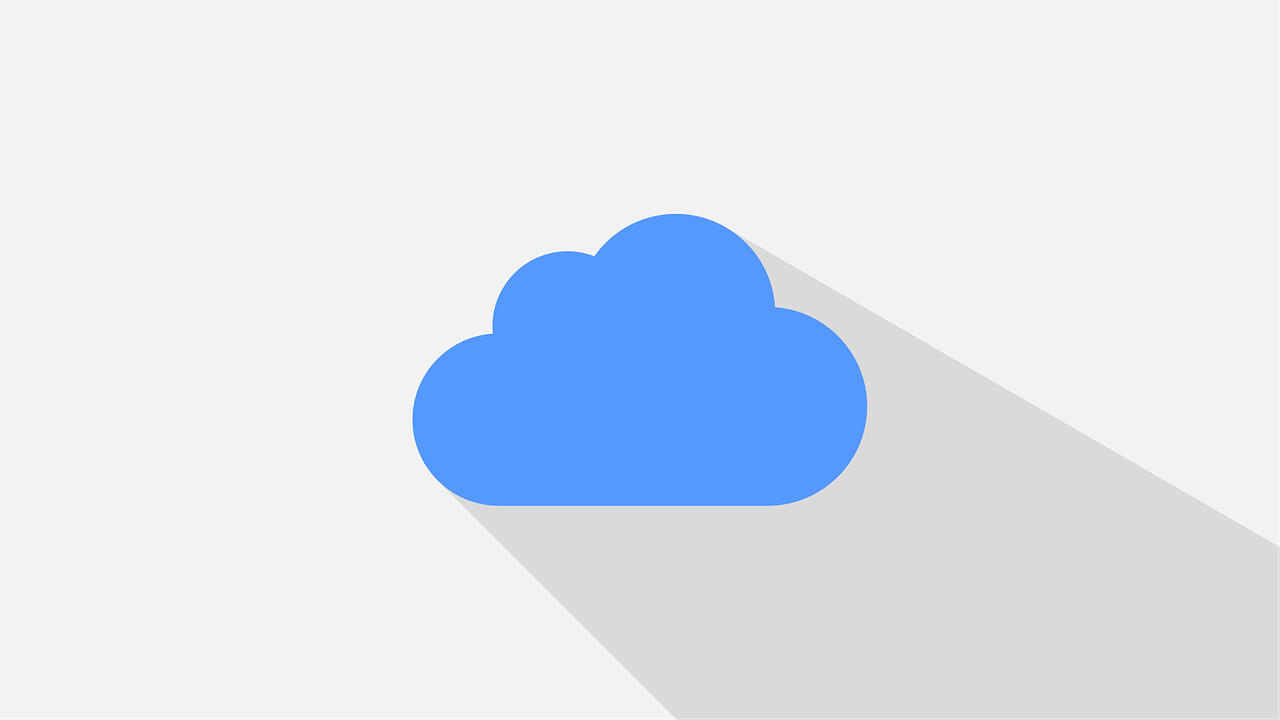
1、安装所需库
首先需要安装requests和BeautifulSoup库,在命令行中输入以下命令进行安装:
pip install requests pip install beautifulsoup4
2、导入所需库
在Python代码中导入requests和BeautifulSoup库:
import requests from bs4 import BeautifulSoup
3、发送HTTP请求
使用requests库的get方法发送HTTP请求,获取网页内容,访问百度首页:
url = 'https://www.baidu.com' response = requests.get(url)
4、解析网页内容
使用BeautifulSoup库解析网页内容,提取所需信息,提取所有的链接:
soup = BeautifulSoup(response.text, 'html.parser') links = soup.find_all('a') for link in links: print(link.get('href'))
5、模拟用户操作
要模拟用户访问点击网页,可以使用Selenium库,Selenium是一个自动化测试工具,可以模拟用户操作浏览器,首先需要安装Selenium库:
pip install selenium
然后下载对应浏览器的驱动程序,例如Chrome浏览器的chromedriver,将其放在系统路径中,或者在代码中指定其路径,以下是一个简单的示例,模拟用户访问百度首页并点击搜索按钮:
from selenium import webdriver from selenium.webdriver.common.keys import Keys 创建一个Chrome浏览器实例 driver = webdriver.Chrome() 访问百度首页 driver.get('https://www.baidu.com') 找到搜索框并输入关键词 search_box = driver.find_element_by_id('kw') search_box.send_keys('Python') 找到搜索按钮并点击 search_button = driver.find_element_by_id('su') search_button.click()
6、等待页面加载完成
网页上的元素还没有加载完成,直接进行操作可能会失败,可以使用WebDriverWait和expected_conditions来等待元素加载完成:
from selenium import webdriver from selenium.webdriver.common.keys import Keys from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC from selenium.webdriver.common.by import By from selenium.webdriver.chrome.service import Service as ChromeService from webdriver_manager.chrome import ChromeDriverManager from selenium.webdriver.common.action_chains import ActionChains from selenium.webdriver.common.touch_actions import TouchActions from selenium.webdriver.common.alert import Alert, AlertTypeHint, DismissAlertOptions, AlertDispositionHint, AlertTextPositionHint, AlertButtonPositionHint, AlertTimeoutException, NoAlertPresentException, UnexpectedAlertPresentException, AlertHandlerHint, AlertMessageHint, AlertTitleHint, AlertCloseButtonHint, AlertIconHint, AlertHeaderTextHint, AlertBodyTextHint, AlertOpenButtonTextHint, AlertDefaultSelectionHint, AlertConfirmButtonTextHint, AlertCancelButtonTextHint, AlertIsPersistentHint, AlertIsBlockingHint, AlertIsNotBlockingHint, AlertIsConfirmationHint, AlertIsPromptHint, AlertIsConfirmationRequiredHint, AlertIsNonBlockingHint, AlertIsNoTopLevelDialogHint, AlertIsNoBackdropHint, AlertIsOverlayModalHint, AlertIsModalHint, AlertIsClosableHint, AlertIsCollapsibleHint, AlertIsStackingContextHint, AlertIsSystemModalHint, AlertIsEscapeKeyCloseableHint, AlertIsTabStopHint, AlertIsFocusableHint, AlertIsEnabledHint, AlertIsVisibleHint, AlertIsInteractiveHint, AlertIsAccessibleHint, AlertIsMultiLineHint, AlertIsExtraSmallScreenHint, AlertIsSmallScreenHint, AlertIsLargeScreenHint, AlertIsMediumScreenHint, AlertIsMobileHint, AlertIsTouchScreenHint, AlertIsFullScreenHint, AlertIsFullScreenDesktopHint, AlertIsMinimizedWindowHint, AlertIsPictureInPictureHint, AlertIsSecureContextHint, AlertIsNativeHandleHint, AlertIsSandboxedFrameAllowedScriptOriginsPolicyHint, AlertIsSandboxedIFrameAllowedScriptOriginsPolicyHint, AlertIsSameOriginAsMainDocumentAllowedScriptOriginsPolicyHint, AlertIsCrossOriginSubresourceSharingPolicyEnforcedByServerHint, AlertIsCrossOriginOpenerPolicyEnforcedByServerHint, AlertIsFeaturePolicyEnforcedByServerHint, AlertIsContentSecurityPolicyEnforcedByServerHint, AlertIsPreloadEnabledByServerHint, AlertIsNavigationRequestedByServerHint, AlertIsDownloadingByServerHint, AlertIsFrameDeniedByServerHint, AlertIsXrCompatibleByServerHint, AlertIsLegacyBrowserByServerHint, AlertIsSpeechSynthesisByServerHint, AlertIsAutoplayPolicyByServerEnforcedHint, AlertIsMixedContentEnforcedByServerHint, AlertIsPluginEnforcedByServerHint, AlertIsBackgroundFetchByServerEnforcedHint, AlertIsPaymentRequestAPIEnabledByServerHint, AlertIsGeolocationOnInsecureOriginEnforcedByServerHint, AlertIsIndexedDBEnabledByServerHint, AlertIsFontDisplayEnabledByServerHint, AlertIsForcedColorsEnabledByServerHint, AlertIsEncryptedMediaByServerEnforcedHint, AlertIsDeviceSensorsEnabledByServerEnforcedHint, AlertIsDocumentWriteInProgressByServerEnforcedHint, AlertIsLangAttributeAwarenessByServerEnforcedHint, AlertIsLayoutNGEnabledByServerEnforcedHint, AlertIsCSSPaintAPIEnabledByServerEnforcedHint, AlertIsCSSRegionsEnabledByServerEnforcedHint, AlertIsCSSGridLayoutEnabledByServerEnforcedHint, AlertIsCSSShapesEnabledByServerEnforcedHint, AlertIsCSSFlexboxEnabledByServerEnforcedHint, AlertIsCSSAllEnabledByServerEnforcedHint, AlertIsCSSVariablesEnabledByServerEnforcedHint, AlertIsCSSCustomPropertiesEnabledByServerEnforcedHint, AlertIsCSSFiltersEnabledByServerEnforcedHint, AlertIsCSSTransitionsEnabledByServerEnforcedHint, AlertIsCSSAnimationsEnabledByServerEnforcedHint, AlertIsCSS3DTransformsEnabledByServerEnforcedHint, AlertIsCSSOMViewStyleEnabledByServerEnforced
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/443991.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复