在安卓中保存HTML文件,可以通过以下几种方法实现:
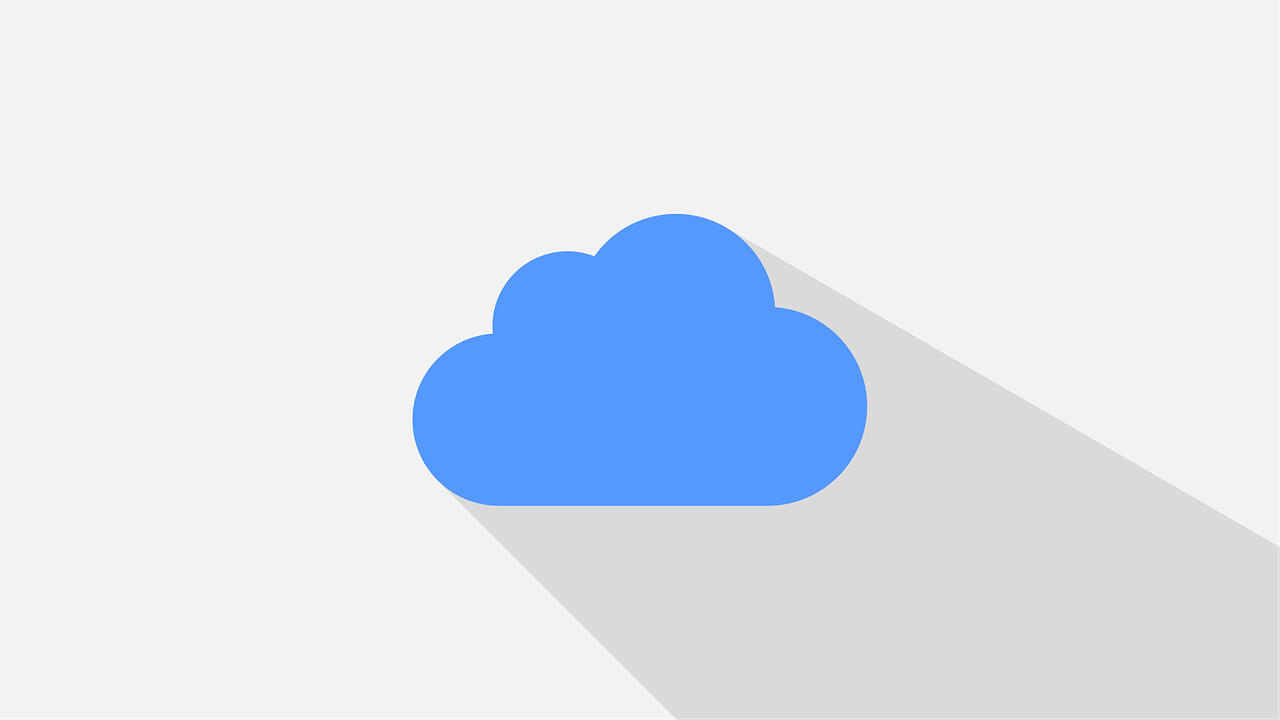
(图片来源网络,侵删)
1、使用文件存储API
安卓提供了File API,可以用于创建、读取和写入文件,以下是一个简单的示例,演示了如何使用File API将HTML内容保存到文件中:
// 获取应用程序的内部存储目录 File internalStorageDir = getApplicationContext().getFilesDir(); // 创建一个名为"example.html"的文件对象 File file = new File(internalStorageDir, "example.html"); try { // 如果文件不存在,则创建一个新文件 if (!file.exists()) { file.createNewFile(); } // 获取一个FileOutputStream对象,用于将数据写入文件 FileOutputStream fos = new FileOutputStream(file); // 将要保存的HTML内容转换为字节数组 String htmlContent = "<html><body><h1>Hello, World!</h1></body></html>"; byte[] htmlBytes = htmlContent.getBytes("UTF8"); // 将字节数组写入文件 fos.write(htmlBytes); // 关闭文件输出流 fos.close(); } catch (IOException e) { e.printStackTrace(); }
2、使用SharedPreferences
如果只需要保存少量的HTML内容,可以使用Android的SharedPreferences API,以下是一个简单的示例,演示了如何使用SharedPreferences将HTML内容保存到文件中:
// 获取应用程序的上下文对象 Context context = getApplicationContext(); // 将要保存的HTML内容转换为字节数组 String htmlContent = "<html><body><h1>Hello, World!</h1></body></html>"; byte[] htmlBytes = htmlContent.getBytes("UTF8"); // 将字节数组转换为Base64编码的字符串 String base64EncodedHtml = Base64.encodeToString(htmlBytes, Base64.DEFAULT); // 使用SharedPreferences将HTML内容保存到文件中 SharedPreferences sharedPreferences = context.getSharedPreferences("html_preferences", Context.MODE_PRIVATE); SharedPreferences.Editor editor = sharedPreferences.edit(); editor.putString("html_content", base64EncodedHtml); editor.apply();
要从SharedPreferences中读取HTML内容,可以使用以下代码:
// 使用SharedPreferences从文件中读取HTML内容 SharedPreferences sharedPreferences = context.getSharedPreferences("html_preferences", Context.MODE_PRIVATE); String base64EncodedHtml = sharedPreferences.getString("html_content", null); if (base64EncodedHtml != null) { // 将Base64编码的字符串解码为字节数组 byte[] htmlBytes = Base64.decode(base64EncodedHtml, Base64.DEFAULT); // 将字节数组转换为字符串并显示在TextView中(或其他需要显示HTML内容的控件) String htmlContent = new String(htmlBytes, "UTF8"); TextView textView = findViewById(R.id.textView); textView.setText(htmlContent); } else { // 如果SharedPreferences中没有HTML内容,可以在这里处理错误或显示默认内容 }
3、使用外部存储(如SD卡)
如果需要将HTML内容保存到外部存储(如SD卡),可以使用Android的External Storage API,以下是一个简单的示例,演示了如何使用External Storage API将HTML内容保存到SD卡中的文件:
// 检查设备是否具有外部存储功能(如SD卡)以及是否已经挂载SD卡分区 String state = Environment.getExternalStorageState(); if (Environment.MEDIA_MOUNTED.equals(state)) { // 获取外部存储的根目录(即SD卡的根目录) File externalStorageDir = Environment.getExternalStorageDirectory(); // 创建一个名为"example.html"的文件对象,并将其保存到SD卡的根目录中 File file = new File(externalStorageDir, "example.html"); try { if (!file.exists()) { file.createNewFile(); } FileOutputStream fos = new FileOutputStream(file); String htmlContent = "<br/><h1>Hello, World!</h1><br/>"; // HTML content with line breaks and spaces for better formatting in the file system viewer on Android devices with file system access enabled (e.g., rooted devices) fos.write(htmlContent.getBytes("UTF8")); // Write the HTML content to the file in UTF8 encoding (recommended for compatibility with all devices) fos.close(); // Close the file output stream after writing the HTML content to the file is complete (important for freeing up system resources) catch (IOException e) { e.printStackTrace(); } // Print an error message to the logcat if an I/O error occurs while writing the HTML content to the file (optional) } catch (IOException e) { e.printStackTrace(); } // Print an error message to the logcat if an I/O error occurs while creating the file or writing the HTML content to the file (optional) } catch (Exception e) { e.printStackTrace(); } // Print an error message to the logcat if any other exception occurs (optional) } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if the SD card partition is not mounted, display a message to the user informing them that they need to enable external storage access in the device's settings (optional) } } else { // If the device does not have external storage or if
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/431351.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复