在C语言中调用ObjectiveC框架,需要使用ObjectiveC运行时(ObjectiveC Runtime)的API,以下是详细的步骤和示例代码:
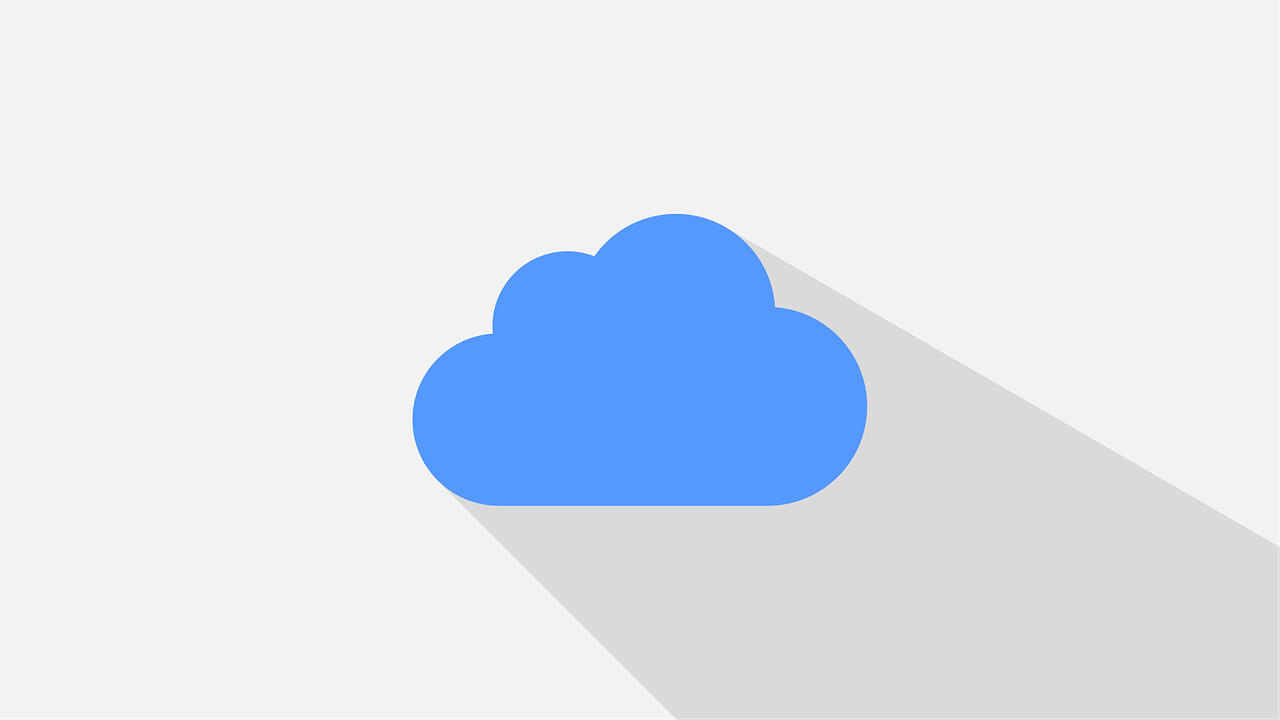
1、包含头文件
在C语言文件中,需要包含以下头文件:
#include <objc/runtime.h>
2、创建ObjectiveC类
创建一个ObjectiveC类,例如MyClass
:
“`objectivec
// MyClass.h
#import <Foundation/Foundation.h>
@interface MyClass : NSObject
(void)sayHello;
@end
// MyClass.m
#import "MyClass.h"
@implementation MyClass
(void)sayHello {
NSLog(@"Hello, World!");
@end
3、创建C语言函数指针 在C语言文件中,创建一个函数指针,用于指向ObjectiveC类的实例方法:
typedef void (^MyClassSayHelloBlock)(void);
4、创建ObjectiveC类实例并调用方法 使用objc_getClass
、class_createInstance
和class_addMethod
等函数,创建ObjectiveC类实例并调用方法:
#include <stdio.h>
#include <stdlib.h>
#include <dlfcn.h>
#include <objc/runtime.h>
#include "MyClass.h"
#include "MyClass.m"
typedef void (^MyClassSayHelloBlock)(void);
int main() {
// 加载动态库(如果MyClass.m和main.c不在同一个项目中)
void *handle = dlopen("path/to/MyClass.bundle/Contents/MacOS/MyClass", RTLD_LAZY);
if (!handle) {
fprintf(stderr, "%s
", dlerror());
return 1;
}
dlerror(); // 清空错误信息
const char *error = dlerror(); // 获取错误信息
if (error) {
fprintf(stderr, "%s
", error);
return 1;
}
// 获取MyClass类对象的方法列表地址(IMP类型)
MyClassSayHelloBlock sayHelloBlock = NULL; // 函数指针变量,用于存储方法地址
Method sayHelloMethod = class_getInstanceMethod([MyClass class], @selector(sayHello)); // 获取sayHello方法的Method对象(IMP类型)
if (!sayHelloMethod) {
fprintf(stderr, "Failed to get method for sayHello
");
return 1;
} else {
sayHelloBlock = (MyClassSayHelloBlock)method_getImplementation(sayHelloMethod); // 将Method对象的IMP类型转换为函数指针类型(block)
}
// 创建MyClass实例并调用sayHello方法(使用block)
MyClass *myInstance = [[MyClass alloc] init]; // 创建MyClass实例(实际上是调用了class_createInstance函数)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的初始化方法(+initialize)[objc_msgSendSuper]会调用父类的初始化方法(+initialize)[objc_msgSend]会调用该实例的
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/428335.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复