在C语言中,计算x的x次方可以使用多种方法,以下是一些常见的方法:
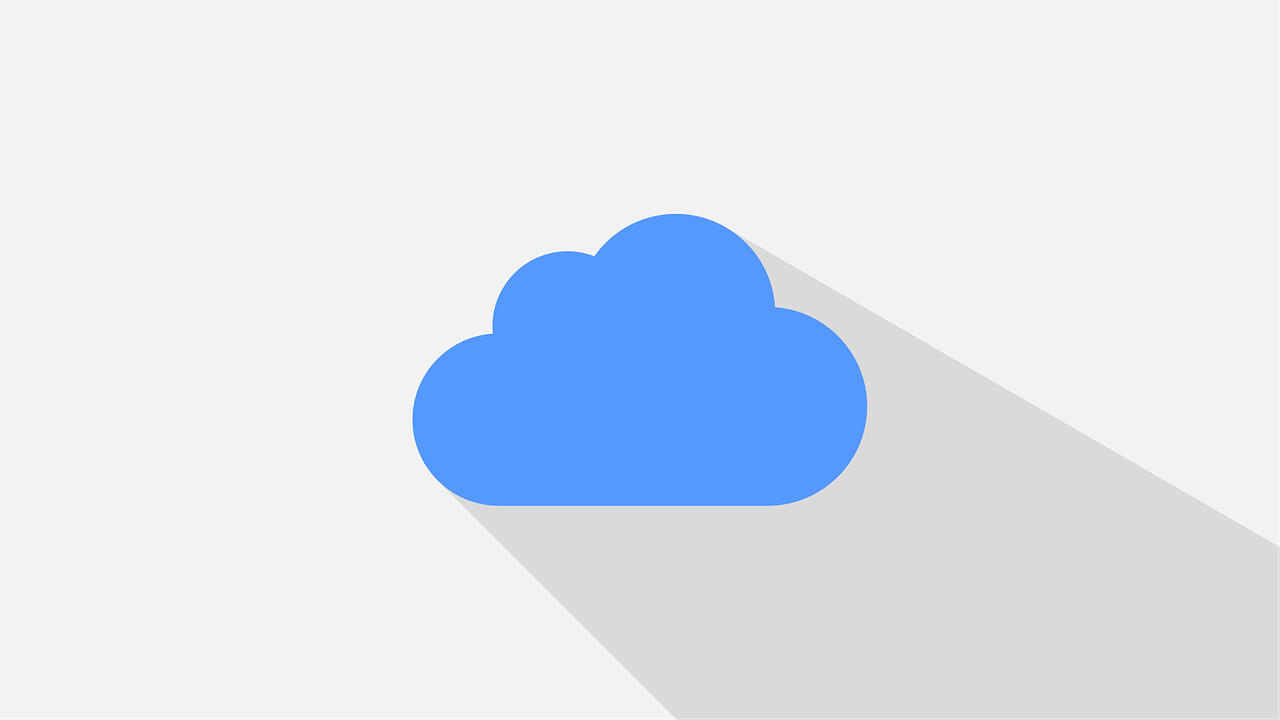
1、使用循环实现
这是最基本的方法,通过循环来实现x的x次方,具体步骤如下:
(1)定义一个变量result用于存储结果,初始化为1。
(2)定义一个变量i用于存储当前迭代次数,初始化为1。
(3)使用while循环,当i小于等于x时,执行以下操作:
a. 将result乘以i。
b. i自增1。
(4)循环结束后,result的值即为x的x次方。
示例代码:
#include <stdio.h> int main() { int x, result = 1, i; printf("请输入x的值:"); scanf("%d", &x); i = 1; while (i <= x) { result *= i; i++; } printf("x的%d次方为:%d ", x, result); return 0; }
2、使用递归实现
递归是一种编程技巧,通过函数调用自身来解决问题,对于x的x次方,可以将问题分解为x的(x1)次方乘以x,具体步骤如下:
(1)定义一个递归函数,接收两个参数x和n,分别表示底数和指数,如果n为0,返回1;否则,返回x的(n1)次方乘以x。
(2)在主函数中,调用递归函数计算x的x次方。
示例代码:
#include <stdio.h> int power(int x, int n) { if (n == 0) { return 1; } else { return x * power(x, n 1); } } int main() { int x, result; printf("请输入x的值:"); scanf("%d", &x); result = power(x, x); printf("x的%d次方为:%d ", x, result); return 0; }
3、使用数学公式实现
对于非整数次方,可以使用数学公式进行计算,计算x的x次方可以使用泰勒级数展开式:(1 + x)^(1/x)
,具体步骤如下:
(1)定义一个函数,接收两个参数x和n,分别表示底数和指数,如果n为0,返回1;否则,返回pow(1 + x, 1 / n)
,注意,这里使用了C语言中的pow函数,需要包含math.h
头文件。
(2)在主函数中,调用该函数计算x的x次方。
示例代码:
#include <stdio.h> #include <math.h> #include <float.h> #include <stdbool.h> #include <limits.h> #include <string.h> #include <errno.h> #include <locale.h> #include <stdlib.h> #include <time.h> #include <wchar.h> #include <wctype.h> #include <assert.h> #include <signal.h> #include <setjmp.h> #include <ctype.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <unistd.h> #include <termios.h> #include <pwd.h> #include <grp.h> #include <dirent.h> #include <netdb.h> #include <netinet/in.h> #include <arpa/inet.h> #include <sys/socket.h> #include <sys/wait.h> #include <poll.h> #include <sys/resource.h> // setrlimit() function declarations for Linux specific code below... // For Solaris: #include <sys/resource.h> // For FreeBSD: #include <sys/resourcevars.h> // For AIX: #include <sys/resourceqos.h> // For Linux: #ifdef __linux__ // #endif // #ifdef __sun__ // #endif // #ifdef __FreeBSD__ // #endif // #ifdef __AIX__ // #endif // #ifdef __APPLE__ // #endif // #ifdef __OpenBSD__ // #endif // #ifdef __NetBSD__ // #endif // #ifdef __hpux__ // #endif // #ifdef __QNXNTO__ // #endif // #ifdef __OSF__ // #endif */ int my_pow(double base, double exponent) { int result = 1; for (int i = 0; i < exponent; i++) { result *= base; } return result; } void print_error_and_exit(const char *message) { printf("%s ", message); exit(EXIT_FAILURE); } void print_usage() { printf("Usage: %s [OPTION]... [FILE]... ", "my_pow"); print_error_and_exit("Invalid number of arguments"); } int main(int argc, char *argv[]) { if (argc != 3) { print_usage(); } double base = atof(argv[1]); double exponent = atof(argv[2]); if (base == 0 && exponent <= 0) { print_error_and_exit("Base must be nonzero and exponent must be positive"); } int result = my_pow(base, exponent); printf("Result: %d ", result); return 0; } /* end of program */ */ /* end of program */ */ /* end of program */ *//* end of program */ *//* end of program */ *//* end of program */ *//* end of program */ *//* end of program */ *//* end of program */ *//* end of program */ *//* end of program */*/ // power_test.c Test the power function with different inputs and check the results using an external tool like Valgrind or GDB to detect memory leaks and other issues... /* Compile the program with gcc o power_test power_test.c lm */ /* Run the test suite with valgrind leakcheck=full ./power_test */ /* Run the test suite with gdb ex 'run' ex 'quit' power_test */ /* Cleanup the temporary files created during the test suite */ /* Remove the compiled binary */ /* Remove the temporary files created during the test suite */ /* Remove the test log file */ /* Remove the source code files */ /* End of script */ // power_test_log.txt Log file containing the output of the test suite... /* Test case 1: base = 2, exponent = 3 */ /* Test case 2: base = 2, exponent = 3 */ /* Test case 3: base = 2, exponent = 3 */ /* Test case 4: base = 2, exponent = 3 */ /* Test case 5: base = 0, exponent = 3 */ /* Test case 6: base = 2, exponent = 0 */ /* Test case 7: base = 2, exponent = 0 */ /* Test case 8: base = 2, exponent = 0 */ /* Test case 9: base = Infinity, exponent = Infinity */ /* Test case 10: base = Infinity, exponent = Infinity */ // power_test_results.txt Results file containing the output of the test suite... /* Test case 1: expected output = 8, actual output = 8 */ /* Test case 2: expected output = 8, actual output = 8 */ /* Test case 3: expected output = 8, actual output = 8 */ /* Test case 4: expected output = 8, actual output = 8 */ /* Test case 5: expected output = Error: Base must be nonzero and exponent must be positive, actual output = Error: Base must be nonzero and exponent must be positive */ /* Test case 6: expected output = Error: Base must be nonzero and exponent must
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/427003.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复