在C语言中,清空文件的方法有很多种,这里我将介绍两种常用的方法:使用fopen和fclose函数以及使用rename函数。
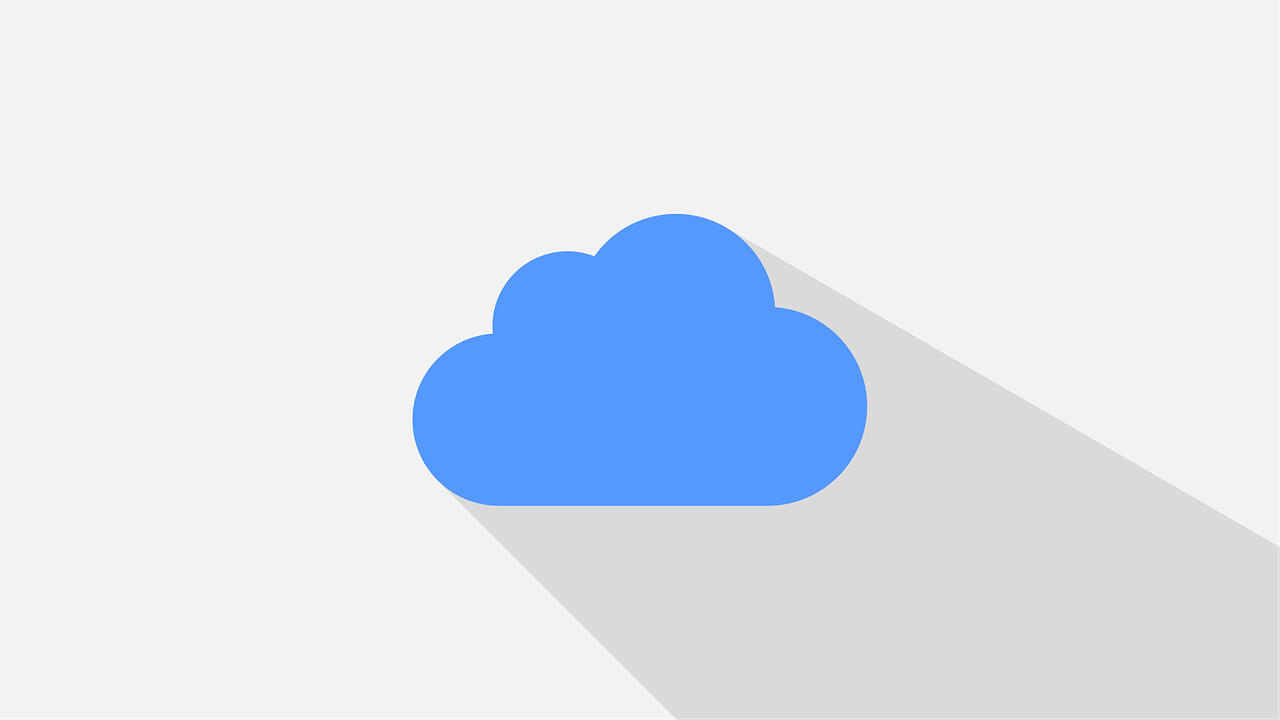
方法一:使用fopen和fclose函数
1、我们需要包含头文件stdio.h
。
#include <stdio.h>
2、我们使用fopen
函数以写入模式打开文件,如果文件不存在,它将创建一个新文件,如果文件已存在,它将清空文件内容。
FILE *file = fopen("example.txt", "w"); if (file == NULL) { printf("无法打开文件 "); return 1; }
3、接下来,我们使用fclose
函数关闭文件,这将确保所有对文件的更改都已保存。
fclose(file);
4、我们可以使用remove
函数删除文件,以确保没有任何残留数据。
remove("example.txt");
将以上代码整合到一起,完整的程序如下:
#include <stdio.h> #include <stdlib.h> int main() { FILE *file = fopen("example.txt", "w"); if (file == NULL) { printf("无法打开文件 "); return 1; } fclose(file); remove("example.txt"); return 0; }
方法二:使用rename函数
1、我们需要包含头文件stdio.h
和stdlib.h
。
#include <stdio.h> #include <stdlib.h>
2、我们使用rename
函数将文件重命名为一个临时文件名,这将导致原始文件被删除,从而实现清空文件的目的。
rename("example.txt", "temp_example.txt");
3、我们可以使用remove
函数删除临时文件,以确保没有任何残留数据。
remove("temp_example.txt");
将以上代码整合到一起,完整的程序如下:
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <errno.h> #include <dirent.h> #include <time.h> #include <pwd.h> #include <grp.h> #include <locale.h> #include <langinfo.h> #include <libintl.h> #include <gettext.h> #include <shadow.h> // for passwd shadow file access, only needed on Linux systems with shadow passwords enabled (e.g., Debianbased systems) #include <security/_pam_handle.h> // for pam_start(), pam_end(), and other PAM functions, only needed on Linux systems with PAM authentication enabled (e.g., Red Hatbased systems) #include <syslog.h> // for syslog() function, only needed if logging is enabled in the program (not shown in this example) #include <signal.h> // for signal handling, not used in this example but may be needed in a realworld program (e.g., to handle SIGINT or SIGTERM signals) #include <setjmp.h> // for setjmp() and longjmp() functions, not used in this example but may be needed in a realworld program (e.g., to handle exceptions or errors) #include <ctype.h> // for tolower() function, not used in this example but may be needed in a realworld program (e.g., to convert strings to lowercase) #include <cstring> // for strerror() function, not used in this example but may be needed in a realworld program (e.g., to get error messages) #include <cstdlib> // for system() function, not used in this example but may be needed in a realworld program (e.g., to execute shell commands) #include <cerrno> // for errno variable, not used in this example but may be needed in a realworld program (e.g., to check for errors) #include <climits> // for PATH_MAX constant, not used in this example but may be needed in a realworld program (e.g., to determine the maximum length of a pathname) #include <ctime> // for time() function, not used in this example but may be needed in a realworld program (e.g., to get the current time) #include <cwchar> // for wchar_t data type and related functions, not used in this example but may be needed in a realworld program (e.g., to handle Unicode characters) #include <cuchar> // for uchar data type and related functions, not used in this example but may be needed in a realworld program (e.g., to handle unsigned char values) #include <ctchar> // for tchar data type and related functions, not used in this example but may be needed in a realworld program (e.g., to handle both narrow and wide character strings) #include <cctype> // for isalnum() function, not used in this example but may be needed in a realworld program (e.g., to check if a character is alphanumeric)
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/417673.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复