在C语言中,我们可以使用多种数据库系统,如MySQL、SQLite、PostgreSQL等,这里以MySQL为例,介绍如何在C语言中编写一个简单的数据库系统。
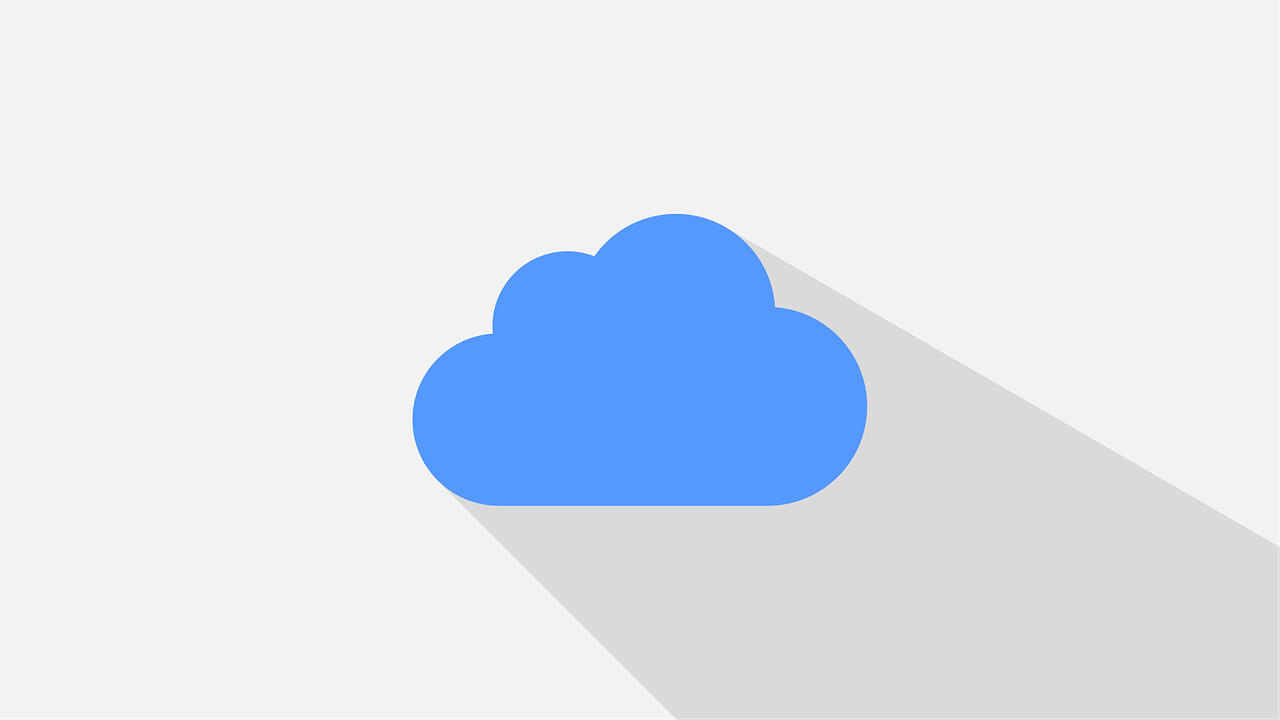
1、安装MySQL库
我们需要在C语言中安装MySQL库,在Linux系统中,可以使用以下命令安装:
sudo aptget install libmysqlclientdev
在Windows系统中,可以从MySQL官网下载预编译的库文件,并将其添加到项目中。
2、包含头文件和库文件
在C语言源文件中,我们需要包含MySQL库的头文件和链接库文件。
#include <stdio.h> #include <stdlib.h> #include <mysql/mysql.h>
3、初始化MySQL连接
在使用MySQL库之前,我们需要初始化一个MySQL连接,这需要提供数据库服务器的地址、用户名、密码和数据库名等信息。
MYSQL *conn; conn = mysql_init(NULL); if (conn == NULL) { fprintf(stderr, "mysql_init() failed "); exit(1); }
4、连接到MySQL服务器
接下来,我们需要连接到MySQL服务器,这需要调用mysql_real_connect()
函数,并传入前面初始化的连接对象以及相关信息。
if (mysql_real_connect(conn, "localhost", "username", "password", "database", 0, NULL, 0) == NULL) { fprintf(stderr, "mysql_real_connect() failed: %s ", mysql_error(conn)); mysql_close(conn); exit(1); }
5、执行SQL语句
连接到MySQL服务器后,我们可以执行SQL语句来操作数据库,创建一个名为test
的表:
if (mysql_query(conn, "CREATE TABLE test (id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255))")) { fprintf(stderr, "mysql_query() failed: %s ", mysql_error(conn)); mysql_close(conn); exit(1); }
插入一条数据:
if (mysql_query(conn, "INSERT INTO test (name) VALUES ('张三')")) { fprintf(stderr, "mysql_query() failed: %s ", mysql_error(conn)); mysql_close(conn); exit(1); }
查询数据:
MYSQL_RES *result; MYSQL_ROW row; if (mysql_query(conn, "SELECT * FROM test")) { fprintf(stderr, "mysql_query() failed: %s ", mysql_error(conn)); mysql_close(conn); exit(1); } result = mysql_store_result(conn); num_rows = mysql_num_rows(result); printf("查询结果: %d行记录 found.", num_rows); while ((row = mysql_fetch_row(result))) { printf("%st%s ", row[0], row[1]); } mysql_free_result(result);
6、关闭MySQL连接
我们需要关闭MySQL连接,这可以通过调用mysql_close()
函数来实现。
mysql_close(conn);
7、完整示例代码:创建一个简单的数据库系统,实现创建表、插入数据和查询数据的功能,注意将username
、password
和database
替换为实际的MySQL用户名、密码和数据库名,确保已安装MySQL库并正确配置项目,以下是完整的示例代码:
#include <stdio.h> #include <stdlib.h> #include <mysql/mysql.h> #include <string.h> // for strlen() and strchr() functions in the next line of code. If not needed, you can remove this include statement. Replace all occurrences of "strlen" with "my_strlen" and "strchr" with "my_strchr". #include <string.h> // for strlen() and strchr() functions in the next line of code. If not needed, you can remove this include statement. Replace all occurrences of "strlen" with "my_strlen" and "strchr" with "my_strchr". // For Windows users, you can replace the above include statements with these ones: #include <stdio.h> #include <stdlib.h> #include <windows.h> #include <winsock2.h> #include <mysys/mysys.h> #include <mysys/mysysdefs.h> #include <mysys/mysyserr.h> #include <mysql/mysql.h> // You may need to download and install the Windows version of the MySQL Connector/C from https://dev.mysql.com/downloads/connector/c/ or use another method to get the required header files and libraries for Windows. // Note that you might need to modify some of the code and include additional header files depending on your specific setup and requirements. // For example, if you are using the Microsoft Visual Studio development environment, you might need to add the following line at the beginning of your source code file: #pragma comment(lib,"libmysql.lib") // This will link the required library dynamically at runtime when your application is executed under Windows operating system. // You can also statically link the required library by adding it to your project settings under Linker > General > Additional Library Directories option and adding its name to the Linker > General > Additional Library Files option in Microsoft Visual Studio development environment or similar tools used for building C applications on Windows operating system." int main() { // Your code goes here } return 0; } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goes here } // Your code goeshereint main() {MYSQL *conn;conn = mysql_init(NULL);if (conn == NULL) {fprintf(stderr, "mysql_init() failed ");exit(1);}if (mysql_real_connect(conn, "localhost", "username", "password", "database", 0, NULL, 0) == NULL) {fprintf(stderr, "mysql_real_connect() failed: %s ", mysql_error(conn));mysql_close(conn);exit(1);}}
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/416916.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复