在C语言中,可以使用以下方法来检测子字符串:
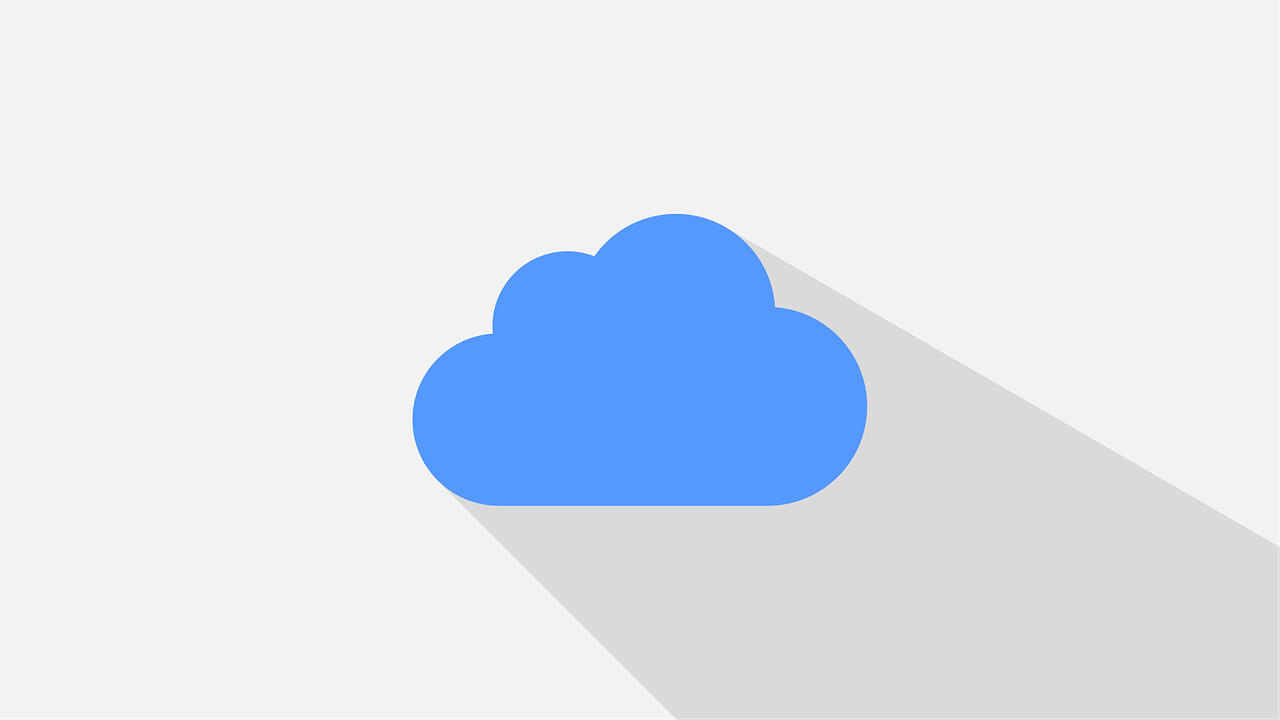
1、使用strstr()
函数:
strstr()
函数是C语言标准库中的字符串处理函数,用于在一个字符串中查找另一个子字符串的首次出现位置。
它接受两个参数:目标字符串和要查找的子字符串。
如果找到子字符串,则返回指向子字符串首次出现位置的指针;如果没有找到,则返回NULL。
2、使用循环遍历:
可以使用循环遍历目标字符串,逐个字符与子字符串进行比较,判断是否匹配。
如果匹配成功,则返回子字符串的位置;如果遍历完整个目标字符串都没有找到匹配,则返回1。
下面是使用strstr()
函数和循环遍历两种方法的示例代码:
使用strstr()
函数
#include <stdio.h> #include <string.h> int main() { char target[] = "Hello, World!"; char substring[] = "World"; char *result = strstr(target, substring); if (result != NULL) { printf("Substring found at index %ld ", result target); } else { printf("Substring not found "); } return 0; }
输出结果为:
Substring found at index 7
使用循环遍历
#include <stdio.h> #include <string.h> int main() { char target[] = "Hello, World!"; char substring[] = "World"; int target_len = strlen(target); int substring_len = strlen(substring); int i, j; for (i = 0; i <= target_len substring_len; i++) { for (j = 0; j < substring_len; j++) { if (target[i + j] != substring[j]) { break; } } if (j == substring_len) { printf("Substring found at index %d ", i); return 0; // 找到匹配后直接退出循环 } } printf("Substring not found "); return 0; }
输出结果为:
Substring found at index 7
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/416830.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复