在C语言中,函数是一段完成特定任务的代码,可以被程序的其他部分调用,以下是一些常用的C语言库函数及其调用方式:
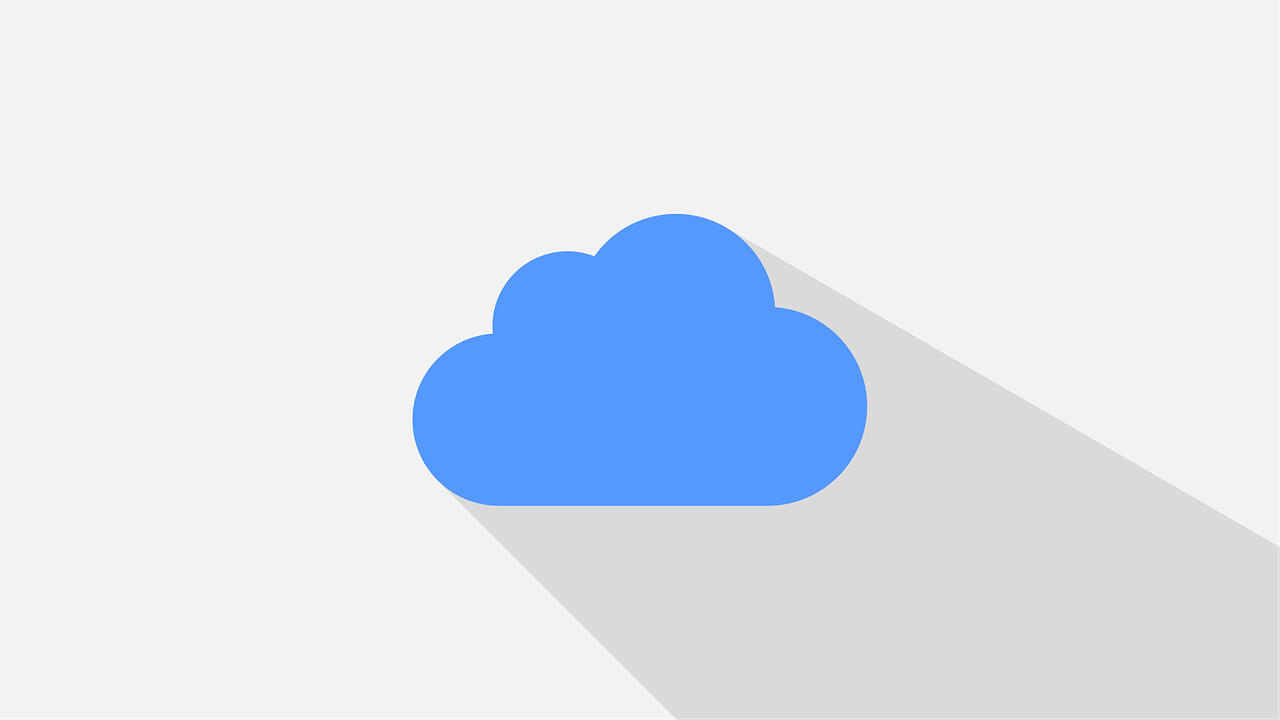
(图片来源网络,侵删)
1. 输入/输出函数
1.1 printf()
printf()
函数用于向标准输出设备(通常是屏幕)打印格式化的输出。
#include <stdio.h> int main() { printf("Hello, World! "); return 0; }
1.2 scanf()
scanf()
函数用于从标准输入设备(通常是键盘)读取格式化的输入。
#include <stdio.h> int main() { int num; printf("Enter a number: "); scanf("%d", &num); printf("You entered: %d ", num); return 0; }
2. 数学函数
2.1 sqrt()
sqrt()
函数用于计算一个数的平方根。
#include <math.h> #include <stdio.h> int main() { double num = 9.0; double root = sqrt(num); printf("The square root of %.2f is %.2f ", num, root); return 0; }
2.2 pow()
pow()
函数用于计算一个数的指数。
#include <math.h> #include <stdio.h> int main() { double base = 2.0; double exponent = 3.0; double result = pow(base, exponent); printf("The result of %.2f raised to the power of %.2f is %.2f ", base, exponent, result); return 0; }
3. 字符串函数
3.1 strlen()
strlen()
函数用于获取字符串的长度。
#include <string.h> #include <stdio.h> int main() { char str[] = "Hello, World!"; int length = strlen(str); printf("The length of the string is %d ", length); return 0; }
3.2 strcpy()
strcpy()
函数用于复制字符串。
#include <string.h> #include <stdio.h> int main() { char source[] = "Hello, World!"; char destination[20]; strcpy(destination, source); printf("The copied string is: %s ", destination); return 0; }
以上就是一些常用的C语言库函数及其调用方式,实际使用中还有许多其他函数,可以根据需要进行选择和使用。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/396717.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复