在C语言中,计算一个数的N次方通常可以使用标准库函数 pow()
,该函数定义在头文件 <math.h>
中,下面将详细介绍如何使用 pow()
函数以及如何自行实现求幂功能。
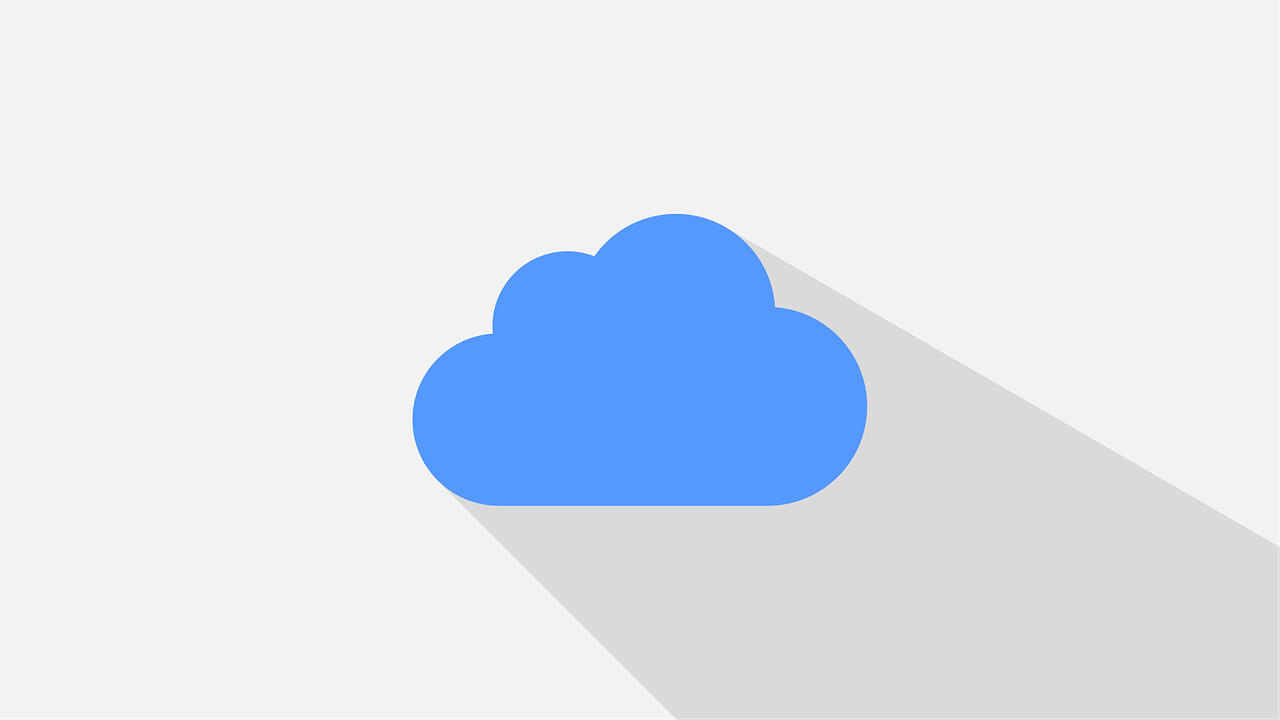
使用标准库函数 pow()
1、包含头文件
在使用 pow()
函数之前,需要包含 <math.h>
头文件。
“`c
#include <math.h>
“`
2、函数原型
pow()
函数原型如下:
“`c
double pow(double x, double y);
“`
它接受两个 double
类型的参数,分别表示底数和指数,并返回一个 double
类型的结果。
3、示例代码
下面是一个简单的示例,展示如何使用 pow()
函数计算 2 的 3 次方:
“`c
#include <stdio.h>
#include <math.h>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
printf("The result of %.2f raised to the power of %.2f is %.2f
", base, exponent, result);
return 0;
}
“`
4、注意事项
pow()
函数在计算时可能会有一定的误差,这是由于浮点数的表示和计算方式导致的。
如果指数是整数,并且底数和结果都是整数,那么可以考虑使用整数运算来避免浮点误差。
自行实现求幂功能
如果你不想使用标准库,或者需要在不支持 <math.h>
的环境中计算幂,可以自行实现求幂功能。
1、递归实现
一个简单的求幂实现是使用递归,这种方法适用于指数为正整数的情况。
“`c
#include <stdio.h>
double power(double base, int exponent) {
if (exponent == 0) {
return 1;
} else {
return base * power(base, exponent 1);
}
}
int main() {
double base = 2.0;
int exponent = 3;
double result = power(base, exponent);
printf("The result of %.2f raised to the power of %d is %.2f
", base, exponent, result);
return 0;
}
“`
2、迭代实现
对于较大的指数,递归可能会导致栈溢出,此时可以使用迭代方法。
“`c
#include <stdio.h>
double power(double base, int exponent) {
double result = 1.0;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
double base = 2.0;
int exponent = 3;
double result = power(base, exponent);
printf("The result of %.2f raised to the power of %d is %.2f
", base, exponent, result);
return 0;
}
“`
3、优化迭代实现
如果需要考虑效率,特别是当指数很大时,可以使用更高效的算法,例如快速幂算法(Binary Exponentiation)。
“`c
#include <stdio.h>
double power(double base, int exponent) {
if (exponent == 0) {
return 1;
}
double temp = power(base, exponent / 2);
if (exponent % 2 == 0) {
return temp * temp;
} else {
return base * temp * temp;
}
}
int main() {
double base = 2.0;
int exponent = 3;
double result = power(base, exponent);
printf("The result of %.2f raised to the power of %d is %.2f
", base, exponent, result);
return 0;
}
“`
快速幂算法的时间复杂度为 O(log N),N 是指数。
归纳一下,计算一个数的N次方在C语言中可以通过使用标准库函数 pow()
或自行实现求幂功能来完成,自行实现时可以考虑递归、迭代或更高效的算法,选择哪种方法取决于具体需求和环境限制。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/391016.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复