ES6中的类(Class)是JavaScript中一种新的语法糖,使得原型继承更加清晰和易于理解,在使用ES6类时,开发者可能会遇到各种错误和问题,下面将详细探讨一些常见的ES6类报错及其解决方案。
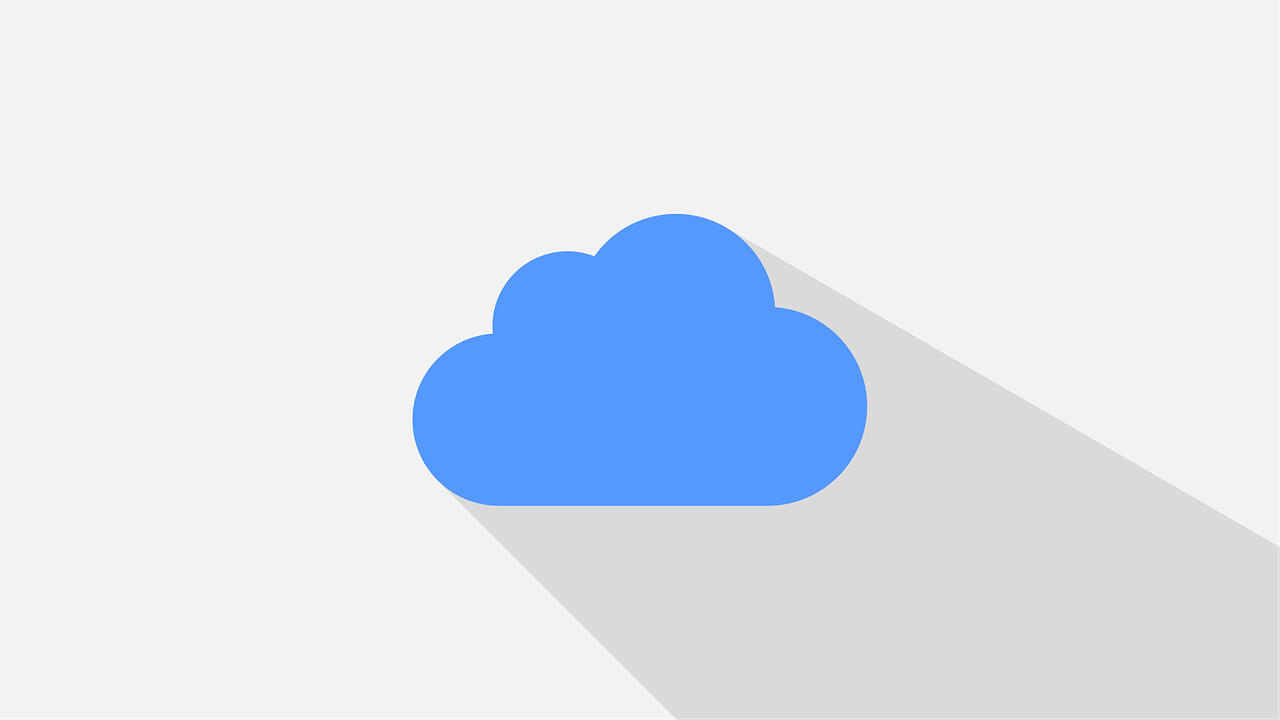
基本语法错误是最常见的,在定义类时,必须确保使用了class
关键字,并且类名符合JavaScript的标识符命名规则。
// 错误的类名 class 2DPoint { constructor(x, y) { this.x = x; this.y = y; } } // SyntaxError: Unexpected token ILLEGAL // 正确的类名 class Point { constructor(x, y) { this.x = x; this.y = y; } }
类的方法必须使用简写函数或箭头函数的形式,不能使用传统的函数声明。
class Point { constructor(x, y) { this.x = x; this.y = y; } // 错误的方法定义 toString: function() { return(${this.x}, ${this.y})
; } // SyntaxError: Unexpected token ':' // 正确的简写方法 toString() { return(${this.x}, ${this.y})
; } }
类中的静态方法需要使用static
关键字,如果忘记使用它,尝试调用该方法时会遇到报错。
class Util { static generateId() { return Math.random().toString(36).substring(2, 15); } // 错误,尝试调用非静态方法 generateId() { // 这将不会被视为静态方法 } } // 正确调用静态方法 console.log(Util.generateId()); // 正常运行 // 错误调用方式 let util = new Util(); console.log(util.generateId()); // TypeError: util.generateId is not a function
构造函数中的super
关键字是用于调用父类构造函数的,如果你在子类的构造函数中没有调用super
,则会报错。
class Parent { constructor() { this.parentProperty = true; } } class Child extends Parent { constructor() { // 忘记调用super this.childProperty = false; } } // 错误:必须调用super new Child(); // ReferenceError: Must call super constructor in derived class before accessing 'this' or returning from derived constructor
extends
关键字用于继承,如果继承的父类不存在,或者继承的不是一个类,也会抛出错误。
// 错误的继承,因为Animal未定义 class Dog extends Animal { // ... } // ReferenceError: Animal is not defined // 正确的继承 class Animal { constructor(name) { this.name = name; } } class Dog extends Animal { constructor(name) { super(name); // 正确调用super } }
在类中使用get
和set
访问器时,如果语法错误或属性不存在,也会导致错误。
class Point { constructor(x, y) { this._x = x; this._y = y; } // 错误的get语法 get x() { return this._x; } // 错误的set语法 set x(value) { this._x = value; } // 正确的get和set语法 get y() { return this._y; } set y(value) { this._y = value; } } let point = new Point(1, 2); console.log(point.x); // undefined,因为get x没有定义正确 point.x = 10; // 不会有任何效果,因为set x没有定义正确 console.log(point.y); // 正常输出2,因为get y定义正确 point.y = 20; // 正常更新_y,因为set y定义正确
在遇到ES6类报错时,需要注意以下几点:
遵循JavaScript的命名规则。
使用简写函数或箭头函数定义方法。
使用static
关键字定义静态方法。
在子类构造函数中调用super
。
确保继承的父类已经定义。
正确使用get
和set
访问器。
掌握这些规则,可以帮助你更有效地调试和避免在使用ES6类时遇到的错误。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/383254.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复