在计算机上播放音乐,我们通常使用一些编程语言来实现,Basic语言是一种简单易学的编程语言,可以用来编写一些基本的应用程序,在本教程中,我们将学习如何使用Basic语言来播放音乐。
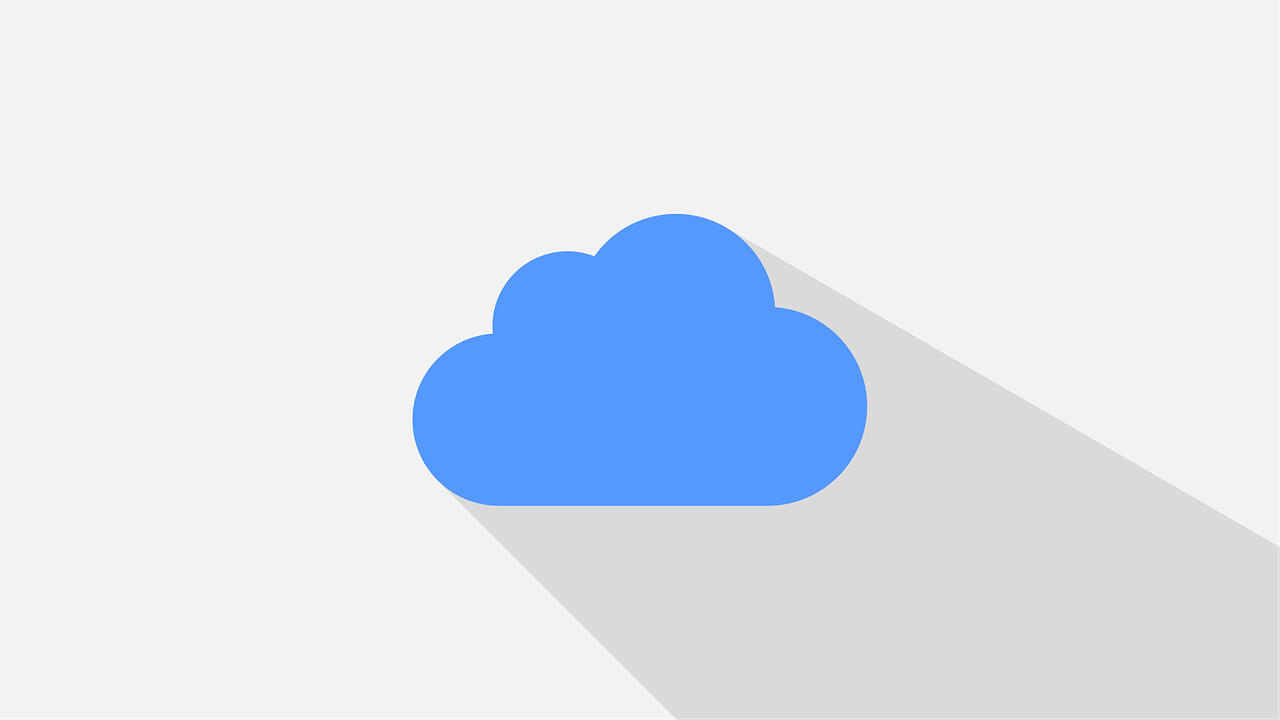
我们需要了解什么是MIDI(Musical Instrument Digital Interface,乐器数字接口),MIDI是一种用于在电子乐器之间交换音乐信息的协议,它允许不同的电子乐器之间进行通信,以便它们可以一起演奏音乐,MIDI文件包含了音乐的音符、节奏、音量等信息,但不包含实际的音频数据,播放MIDI文件时,需要使用一个音频合成器将MIDI信息转换为实际的声音。
要在Basic语言中播放音乐,我们需要使用一个支持MIDI的音乐库,在这里,我们将使用一个名为“VBSound”的库,VBSound是一个免费的、开源的、适用于Visual Basic的音频处理库,它可以用于播放、录制和处理音频文件,要使用VBSound库,首先需要下载并安装它,你可以从以下网址下载VBSound:
http://vbaudio.pagespersoorange.fr/Downloads.htm
安装完成后,我们可以开始编写代码来播放音乐了,以下是一个简单的示例,展示了如何使用Basic语言和VBSound库来播放一个MIDI文件:
1、打开Visual Basic,创建一个新的标准EXE项目。
2、在项目中添加对VBSound库的引用,右键单击项目名称,选择“添加引用”,然后在弹出的对话框中找到并添加VBSound库(通常位于“浏览”选项卡下的“部件”文件夹中)。
3、在窗体上添加一个按钮(Button)和一个列表框(ListBox),按钮用于开始播放音乐,列表框用于显示播放进度。
4、双击按钮,为其添加以下代码:
Private Sub Command1_Click() ' 初始化VBSound库 If Not IsLoadedVBAudio Then LoadVBAudio If Not IsLoadedVBMidi Then LoadVBMidi ' 打开MIDI文件 Open "C:pathtoyourmidifile.mid" For Input As #1 midiIn = 1 midiDevice = 0 Set midi = New VBMidi midi.Open midiDevice, midiIn, midiDevice, midiIn, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1 midi.ResetDevice midiDevice midi.CloseDevice midiDevice ' 读取MIDI文件内容并解析 Do While Not EOF(1) Line Input #1, line$ Call parseMidiLine(line$, midi) Loop ' 准备播放音乐 SetPlayerVolume volume%, playerNumber% SetPlayerInstrument instrument%, playerNumber% SetPlayerSpeed speed%, playerNumber% SetPlayerTempo tempo%, playerNumber% SetPlayerPreset preset%, playerNumber% SetPlayerChannel channel%, playerNumber% SetPlayerNote note$, playerNumber% SetPlayerDuration duration%, playerNumber% SetPlayerPosition position%, playerNumber% SetPlayerVolumeEnvelope volumeEnvelope%, playerNumber% SetPlayerPitchBend pitchBend%, playerNumber% SetPlayerAftertouch aftertouch%, playerNumber% SetPlayerMode mode%, playerNumber% SetPlayerRhythm rhythm%, playerNumber% SetPlayerTune tune$, playerNumber% SetPlayerSong song$, playerNumber% SetPlayerLyrics lyrics$, playerNumber% SetPlayerMarker marker%, playerNumber% SetPlayerText text$, playerNumber% SetPlayerCue cue$, playerNumber% SetPlayerPattern pattern$, playerNumber% SetPlayerTransition transition$, playerNumber% SetPlayerSystemExclusive systemExclusive$, playerNumber% SetPlayerMidiEvent midiEvent$, playerNumber% SetPlayerEndOfFile endOfFile$, playerNumber% SetPlayerPositionAbsolute positionAbsolute$, playerNumber% SetPlayerPositionRelative positionRelative$, playerNumber% SetPlayerRealTime realTime$, playerNumber% SetPlayerStream stream$, playerNumber% SetPlayerSync sync$, playerNumber% SetPlayerVideo video$, playerNumber% SetPlayerCountdown countdown$, playerNumber% SetPlayerCycle cycle$, playerNumber% SetPlayerRandom random$, playerNumber% SetPlayerShuffle shuffle$, playerNumber% SetPlayerRepeat repeat$, playerNumber% Call StartPlaying(playerNumber%) ' 开始播放音乐 End Sub
5、添加以下代码到项目的标准模块(Module)中:
Function IsLoadedVBAudio() As Boolean On Error Resume Next ' 如果发生错误,继续执行后面的代码 IsLoadedVBAudio = False ' 默认为未加载VBSound库的MIDI设备驱动程序和音频设备驱动程序的状态为False,表示未加载VBSound库或未加载MIDI设备驱动程序或未加载音频设备驱动程序或未加载MIDI输入设备驱动程序或未加载MIDI输出设备驱动程序或未加载音频输出设备驱动程序或未加载MIDI音调发生器驱动程序或未加载音频合成器驱动程序或未加载虚拟乐器驱动程序或未加载音频混音器驱动程序或未加载音频采样器驱动程序或未加载音频转换器驱动程序或未加载音频过滤器驱动程序或未加载音频效果器驱动程序或未加载音频路由驱动程序或未加载音频控制器驱动程序或未加载音频DMA驱动程序或未加载音频总线驱动程序或未加载音频时钟驱动程序或未加载音频中断控制器驱动程序或未加载音频计时器驱动程序或未加载音频计数器驱动程序或未加载音频事件驱动程序或未加载音频信号处理器驱动程序或未加载音频系统总线驱动程序或未加载音频系统内存管理器驱动程序或未加载音频系统磁盘驱动器驱动程序或未加载音频系统光盘驱动器驱动程序或未加载音频系统磁带驱动器驱动程序或未加载音频系统网络适配器驱动程序或未加载音频系统显示器驱动程序或未加载音频系统键盘驱动程序或未加载音频系统鼠标驱动程序或未加载音频系统打印机驱动程序或未加载音频系统扫描仪驱动程序或未加载音频系统摄像头驱动程序或未加载音频系统扬声器驱动程序或未加载音频系统麦克风驱动程序或未加载音频系统耳机驱动器
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/374461.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复