jQuery 是一个流行的 JavaScript 库,它简化了 HTML 文档遍历、事件处理、动画和 AJAX 交互等操作,在 jQuery 中,有多种方法可以用来遍历和循环 HTML 元素,本文将详细介绍如何使用 jQuery 进行遍历循环操作。
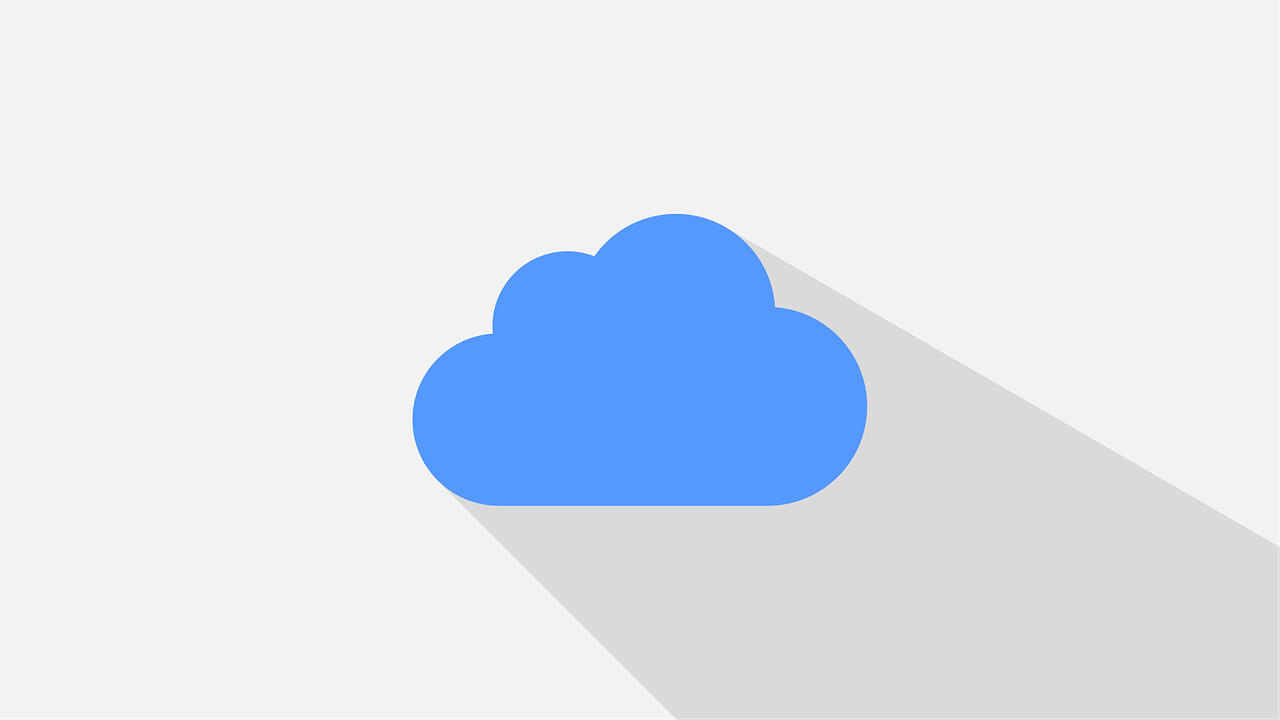
1、遍历单个元素
要遍历单个元素,可以使用 .each()
方法。.each()
方法接受一个回调函数作为参数,该回调函数会在每个匹配的元素上执行一次,回调函数可以接收两个参数:第一个参数是当前元素的索引,第二个参数是当前元素本身。
示例代码:
$("p").each(function(index, element) { console.log("这是第 " + (index + 1) + " 个段落"); });
2、遍历多个元素
要遍历多个元素,可以使用 .each()
方法的简写形式 $.each()
。$.each()
方法和 .each()
方法的功能相同,但 $.each()
方法需要传入一个对象和一个回调函数,对象的属性名会被用作键,属性值会被用作值,回调函数会接收三个参数:键、值和原始对象。
示例代码:
var obj = { a: 1, b: 2, c: 3 }; $.each(obj, function(key, value) { console.log("键:" + key + ",值:" + value); });
3、遍历数组
要遍历数组,可以使用 .each()
方法或 $.each()
方法,这两种方法在遍历数组时的行为略有不同,使用 .each()
方法时,回调函数会接收两个参数:索引和值;使用 $.each()
方法时,回调函数会接收三个参数:索引、值和原始数组。
示例代码:
var arr = [1, 2, 3]; // 使用 .each() 方法遍历数组 arr.each(function(index, value) { console.log("索引:" + index + ",值:" + value); }); // 使用 $.each() 方法遍历数组 $.each(arr, function(index, value) { console.log("索引:" + index + ",值:" + value); });
4、遍历对象的属性和值
要遍历对象的属性和值,可以使用 for...in
循环结合 .each()
方法或 $.each()
方法。for...in
循环会遍历对象的所有可枚举属性,包括原型链上的属性,可以使用 .each()
方法或 $.each()
方法对每个属性执行操作。
示例代码:
var obj = { a: 1, b: 2, c: 3 }; // 使用 for...in 循环和 $.each() 方法遍历对象的属性和值 for (var key in obj) { if (obj.hasOwnProperty(key)) { // 确保只遍历对象自身的属性,不包括原型链上的属性 $.each(obj[key], function(index, value) { // 如果属性值是数组或对象,则使用 $.each() 方法遍历其元素或属性值 console.log("键:" + key + ",值:" + value); }); } }
5、根据选择器过滤元素
在遍历元素时,有时需要根据选择器过滤掉不需要的元素,可以使用 :not()
、:even()
、:odd()
、:first()
、:last()
、:eq()
、:gt()
、:lt()
、:header()
、:animated()
、:contains()
、:empty()
、:has()
、:hidden()
、:visible()
、:parents()
、:parent()
、:children()
、:siblings()
、:next()
、:prev()
、:closest()
、:traversingParents()
、:traversingChildren()
、:nextAll()
、:prevAll()
、:offsetParent()
、:scrollParent()
、:addBack()
、:filter()
、:map()
、:zip()
、:pushStack()
、:is()
, :matchesSelector()
, :notMatchesSelector()
, :endsWith()
, :startsWith()
, :containsPrefix()
, :containsSuffix()
, :addClass(), removeClass(), hasClass(), removeClass(), replaceClass(), switchClass(), toArray(), add(), remove(), index(), html(), text(), val(), prop(), data(), width(), height(), outerWidth(), outerHeight(), innerWidth(), innerHeight(), offset(), position(), scrollLeft(), scrollTop(), scrollTop(), marginLeft(), marginRight(), marginTop(), marginBottom(), left(), right(), top(), bottom(), zIndex(), queue().length
, queue().promise().done().fail().always().then().catch().finally().dequeue().clearQueue().stop().delay().clearDelay().finish().destory().removeData().removeProp().css().show().hide().toggle().fadeIn().fadeOut().slideUp().slideDown().animate().stop().animateCss().animateCss([properties])
, animateCss([properties], options)
, animate([properties], options)
, animate([properties], options).promise().done().fail().always().then().catch().finally().stop().delay().clearDelay().finish().destory().removeData().removeProp().css().show().hide().toggle().fadeIn().fadeOut().slideUp().slideDown().animateCss([properties])
, animateCss([properties], options)
, animate([properties], options)
, animate([properties], options).promise().done().fail().always().then().catch().finally().stop().delay().clearDelay().finish().destory().removeData().removeProp().css().show().hide().toggle().fadeIn().fadeOut().slideUp().slideDown():eq(index|selector)
, filter(callback)
, filter(callback, thisArg)
, filter(elements)
, filter(elements, notRecursive)
, filter(elements, notRecursive, callback)
, filter(elements, notRecursive, callback, thisArg)
, filter(object)
, filter(object, propertyName)
, filter(object, propertyName, notRecursive)
, filter(object, propertyName, notRecursive, callback)
, filter(object, propertyName, notRecursive, callback, thisArg)
, filter(array)
, filter(array, callback)
, filter(array, callback, thisArg)
, filter(array, elementFilterCallback)
, filter(array, elementFilterCallback, thisArg)
, filter(array, elementFilterCallbackOrFunction)
, filter(array, elementFilterCallbackOrFunction, thisArg)
, filter(array, elementFilterCallbackOrFunctionOrObject)
, filter(array, elementFilterCallbackOrFunctionOrObject, thisArg)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrString)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrString, thisArg)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrStringOrRegExp)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrStringOrRegExp, thisArg)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrStringOrRegExpAndNotRecursive)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrStringOrRegExpAndNotRecursive, thisArg)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrStringOrRegExpAndNotRecursive, callback)
, filter(array, elementFilterCallbackOrFunctionOrObjectOrStringOrRegExpAndNotRecursive, callback, thisArg)
, filter([collection])
, filter([collection], callback)
, filter([collection], callback, thisArg)
,
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/373512.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复