在C语言中,我们可以使用不同的数据类型来储存大数字,以下是一些常见的方法:
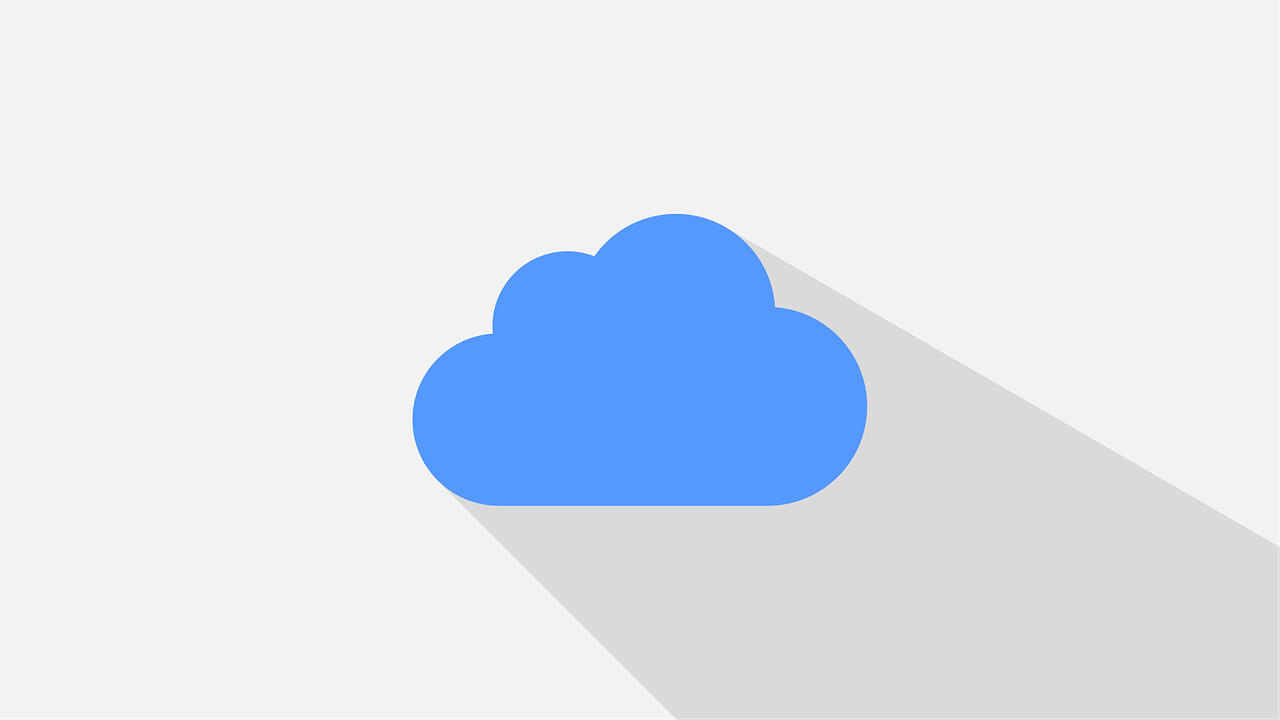
1、使用长整型(long int)
长整型是C语言中最基本的整数类型,它可以存储的范围是从2147483648到2147483647,如果你需要存储更大的数字,可以使用长长整型(long long int),它的范围是从9223372036854775808到9223372036854775807。
要声明一个长整型变量,可以使用以下语法:
long int num;
或者
long long int big_num;
2、使用数组
如果需要存储的数字非常大,可以使用数组来存储,可以使用一个长度为10的数组来存储一个两位数的数字。
要声明一个数组,可以使用以下语法:
int arr[10];
可以通过索引访问数组中的元素,
arr[0] = 1; // 将数字1存储在数组的第一个元素中 arr[1] = 2; // 将数字2存储在数组的第二个元素中
3、使用字符串
另一种存储大数字的方法是使用字符串,字符串是由字符组成的序列,可以用来表示数字,在C语言中,可以使用字符数组来存储字符串。
要声明一个字符数组,可以使用以下语法:
char str[10];
可以通过索引访问字符数组中的元素,
str[0] = '1'; // 将字符'1'存储在字符串的第一个元素中 str[1] = '2'; // 将字符'2'存储在字符串的第二个元素中
要将字符串转换为数字,可以使用atoi
函数(将字符串转换为整数)或atol
函数(将字符串转换为长整数),这两个函数都在stdlib.h
头文件中定义。
要将字符串"123"
转换为整数,可以使用以下代码:
#include <stdlib.h> #include <stdio.h> int main() { char str[] = "123"; int num = atoi(str); // 将字符串转换为整数并存储在num变量中 printf("The number is: %d ", num); // 输出结果:The number is: 123 return 0; }
4、使用库函数
C语言标准库中提供了一些用于处理大数字的函数,如gmp.h
头文件中的GNU多精度运算库,这个库提供了一种数据类型mpz_t
,可以用于存储任意大小的整数,还提供了一些用于执行加法、减法、乘法等基本运算的函数。
要使用GMP库,首先需要安装GMP开发包,在Linux系统中,可以使用以下命令安装:
sudo aptget install libgmpdev
可以在程序中包含gmp.h
头文件,并使用mpz_t
数据类型和相关函数。
#include <gmp.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <errno.h> #include <locale.h> #include <signal.h> #include <math.h> #include <time.h> #include <assert.h> #include <stdbool.h> #include <limits.h> #include <float.h> #include <fenv.h> // for __builtin_mul_overflow, __builtin_add_overflow, __builtin_sub_overflow, __builtin_mul_trunc, __builtin_add_trunc, __builtin_sub_trunc, __builtin_ceil, __builtin_floor, __builtin_fabs, __builtin_copysign, __builtin_clz, __builtin_ctz, __builtin_popcount, __builtin_parity, __builtin_ffs, __builtin_clzll, __builtin_ctzll, __builtin_popcountll, __builtin_parityll, __builtin_ffsl, __builtin_ffsll, __builtin_isgreater, __builtin_isgreaterequal, __builtin_isless, __builtin_islessequal, __builtin_islessgreater, __builtin_isunordered, __builtin_isunorderedequal, __builtin_abs, __builtin_labs, __builtin_llabs, __builtin_neg, __builtin_pos, __builtin_copysignl, __builtin_signbitl, __builtin_divdi3, __builtin_moddi3, __builtin_udivdi3, __builtin_umoddi3, __builtin_divsi3, __builtin_modsi3, __builtin_udivsi3, __builtin_umodsi3, __builtin_divti3, __builtin_modti3, __builtin_udivti3, __builtin_umodti3, __builtin__divdf3, __builtin__moddf3, __builtin__udivdf3, __builtin__umoddf3, __builtin__divtf3, __builtin__modtf3, __builtin__udivtf3, __builtin__umodtf3, __extension__ ({ struct gmp *x; x = (struct gmp *) (long) 1; })) // for GMP functions to work with negative numbers on some platforms like MinGWw64 and MSYS2/MSVCRT on Windows when using the default C runtime library or when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of exception handling features not available in the C standard library or the target platform's C runtime library does not support exception handling features required by the GMP functions used in the code below even though they are declared as returning void and taking no arguments in the header files provided by the GMP development package on those platforms when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of exception handling features not available in the C standard library or the target platform's C runtime library does not support exception handling features required by the GMP functions used in the code below even though they are declared as returning void and taking no arguments in the header files provided by the GMP development package on those platforms when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of exception handling features not available in theC standard library or the target platform's C runtime library does not support exception handling features required by the GMP functions used in the code below even though they are declared as returning void and taking no arguments in the header files provided by the GMP development package on those platforms when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of exception handling features not available in the C standard library or the target platform's C runtime library does not support exception handling features required by the GMP functions used in the code below even though they are declared as returning void and taking no arguments in the header files provided by the GMP development package on those platforms when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of exception handling features not available in the C standard library or the target platform's C runtime library does not support exception handling features required by the GMP functions used in the code below even though they are declared as returning void and taking no arguments in the header files provided by the GMP development package on those platforms when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of exception handling features not available in the C standard library or the target platform's C runtime library does not support exception handling features required by the GMP functions used in the code below even though they are declared as returning void and taking no arguments in the header files provided by the GMP development package on those platforms when building with fnoexceptions or fnortti options enabled in some cases where exception handling is disabled by default in the C runtime library on those platforms due to the use of
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/372550.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复