In the journey of programming in C, encountering compiletime errors is an inevitable part of the learning process. These errors are the compiler’s way of telling you that there is something wrong with your code that it cannot proceed with the compilation. Understanding and resolving these errors is crucial to successfully compiling and running your program. Below, I’ll discuss some common C language compiletime errors, their meanings, and possible ways to resolve them.
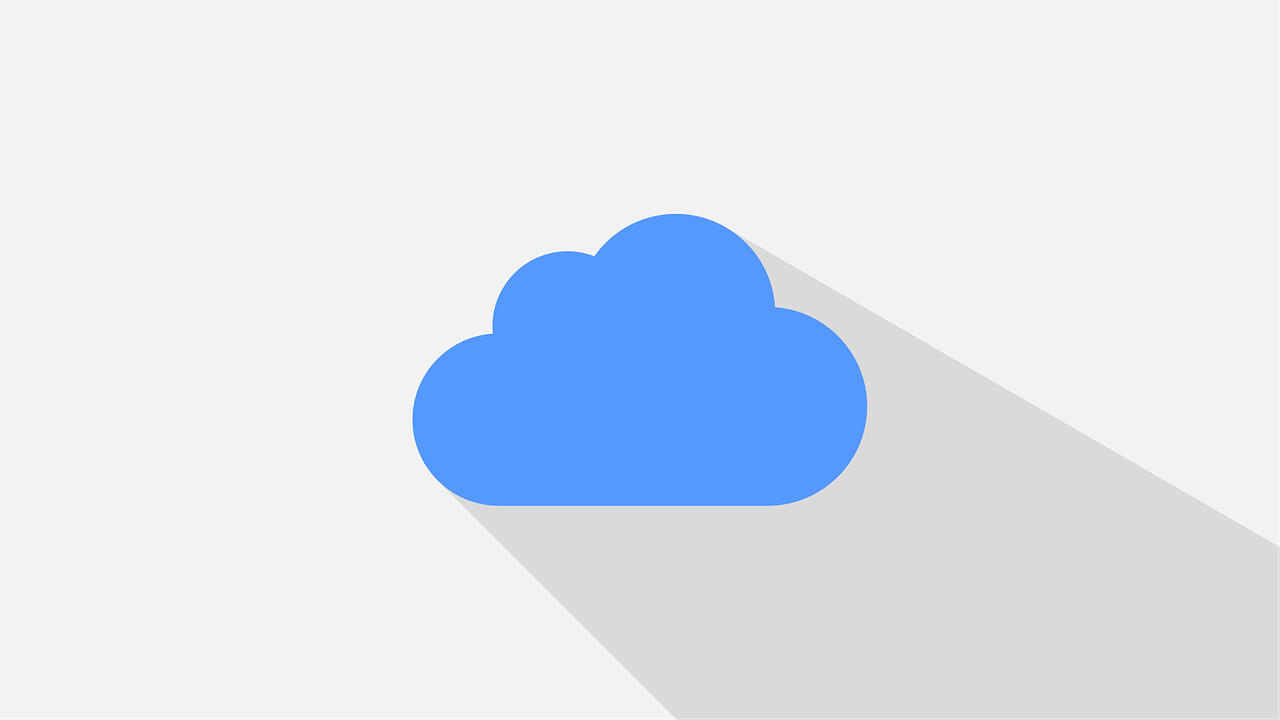
1、Syntax Errors:
Syntax errors are the most common type of compiletime errors. They occur when the code does not conform to the rules of C language syntax. The compiler usually points out the location where it encountered the error.
Example:
int x = 5; if (x = 5) { // Should be '==' instead of '=' // code }
Error:
error: expected expression before '=' token
Solution:
Correct the comparison operator from =
to ==
.
2、undeclared identifiers:
This error occurs when you try to use a variable or function without declaring or defining it first.
Example:
printf("Hello, World!"); // Without including stdio.h
Error:
error: implicit declaration of function 'printf' is invalid in C99
Solution:
Include the appropriate header file where the function is declared:
#include <stdio.h>
3、Type Mismatch Errors:
Type mismatch errors occur when you try to perform operations between incompatible data types.
Example:
int x = 5; char ch = 'a'; x = x + ch; // Trying to add an int and a char
Error:
error: incompatible types when assigning to type 'int' from type 'char'
Solution:
Convert the char
to an int
before adding:
x = x + (int)ch;
4、Missing Semicolons:
In C, every statement should end with a semicolon. Forgetting to add a semicolon leads to a compiletime error.
Example:
int x = 5
Error:
error: expected ';' before '}' token
Solution:
Add a semicolon at the end of the statement:
int x = 5;
5、Unbalanced Parentheses:
Unbalanced parentheses can lead to unexpected behavior and compiletime errors.
Example:
int x = (5 + 3 * 2;
Error:
error: expected ')' before ';' token
Solution:
Balance the parentheses:
int x = (5 + 3) * 2;
6、Missing Braces:
Curly braces {}
are used to define the beginning and end of a block of code. Forgetting to include them can result in errors.
Example:
int main() { if (true) printf("True!"); else printf("False!");
Error:
error: expected '{' at end of input
Solution:
Add the missing braces:
int main() { if (true) { printf("True!"); } else { printf("False!"); } }
7、Conflicting Types:
This error occurs when the same symbol is declared with different types in the same scope.
Example:
int x = 5; char x = 'a'; // Conflicting types
Error:
error: redeclaration of 'x' with a different type
Solution:
Use different names for variables of different types:
int x = 5; char ch = 'a';
8、Incompatible Pointers:
Pointers in C must point to compatible data types. Assigning a pointer of one type to a pointer of another type without casting can result in an error.
Example:
int *iptr = NULL; char *cptr = iptr; // Incompatible pointer types
Error:
error: assignment from incompatible pointer type
Solution:
Cast the pointer to the appropriate type:
char *cptr = (char *)iptr;
In conclusion, compiletime errors are the compiler’s way of guiding you to correct your code. Each error message provides valuable information about what went wrong and where. Understanding these errors and their solutions is key to becoming proficient in the C language. By carefully examining the error messages and making the necessary corrections, you can ensure that your code compiles successfully and behaves as expected.
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/366034.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复