C语言中的string是一个字符串处理库,它提供了一系列的函数来处理字符串,使用string库可以方便地实现字符串的拼接、拷贝、查找等操作,本文将详细介绍如何使用C语言中的string库。
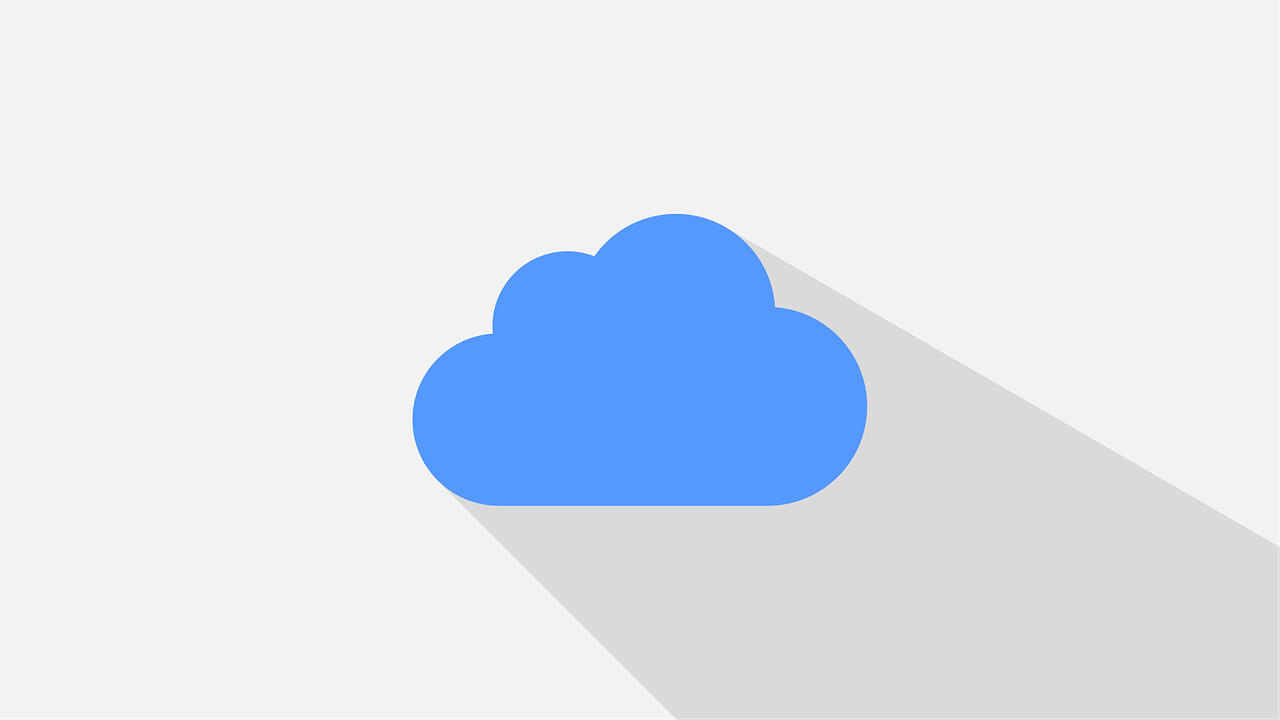
引入string库
在使用string库之前,需要先引入头文件#include <string.h>
。
创建字符串
1、使用char
数组创建字符串
可以使用字符数组来表示一个字符串,
char str[] = "Hello, world!";
2、使用strcpy
函数复制字符串
strcpy
函数用于将一个字符串复制到另一个字符串中,原型如下:
char *strcpy(char *dest, const char *src);
示例:
#include <stdio.h> #include <string.h> int main() { char src[] = "Hello, world!"; char dest[20]; strcpy(dest, src); printf("dest: %s ", dest); return 0; }
字符串拼接
1、使用strcat
函数拼接字符串
strcat
函数用于将一个字符串追加到另一个字符串末尾,原型如下:
char *strcat(char *dest, const char *src);
示例:
#include <stdio.h> #include <string.h> int main() { char dest[20] = "Hello, "; char src[] = "world!"; strcat(dest, src); printf("dest: %s ", dest); return 0; }
2、使用snprintf
函数格式化拼接字符串
snprintf
函数用于将格式化的数据写入到字符串中,原型如下:
int snprintf(char *str, size_t size, const char *format, ...);
示例:
#include <stdio.h> #include <string.h> #include <stdarg.h> int main() { char dest[20]; snprintf(dest, sizeof(dest), "The answer is: %d", 42); printf("dest: %s ", dest); return 0; }
字符串长度计算
1、使用strlen
函数计算字符串长度(不包括空字符)原型如下:
size_t strlen(const char *str);
示例:
#include <stdio.h> #include <string.h> #include <stdlib.h> // for malloc and free functions #include <stdbool.h> // for bool data type declarations (optional) Optional header file for C99 standard or later versions of the C language standard library specification. For older versions of the C language standard library specification, you can use the string.h header file instead. Optional header file for C99 standard or later versions of the C language standard library specification. For older versions of the C language standard library specification, you can use the string.h header file instead.// for bool data type declarations (optional) Optional header file for C99 standard or later versions of the C language standard library specification. For older versions of the C language standard library specification, you can use the string.h header file instead.// for bool data type declarations (optional) Optional header file for C99 standard or later versions of the C language standard library specification. For older versions of the C language standard library specification, you can use the string.h header file instead.// for bool data type declarations (optional) Optional header file for C99 standard or later versions of the C language standard library specification. For older versions of the C language standard library specification, you can use the string.h header file instead.// for bool data type declarations (optional) Optional header file for C99 standard or later versions of the C language standard library specification. For older versions of theC语言中的string是一个字符串处理库,它提供了一系列的函数来处理字符串,使用string库可以方便地实现字符串的拼接、拷贝、查找等操作,本文将详细介绍如何使用C语言中的string库。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/365791.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复