要用C语言制作一个界面,我们可以使用图形库来实现,在Windows平台上,常用的图形库有WinAPI(Windows API)和GDI(Graphics Device Interface),在Linux平台上,常用的图形库有Xlib和GTK+,这里我们以Windows平台为例,介绍如何使用WinAPI和GDI来制作一个简单的界面。
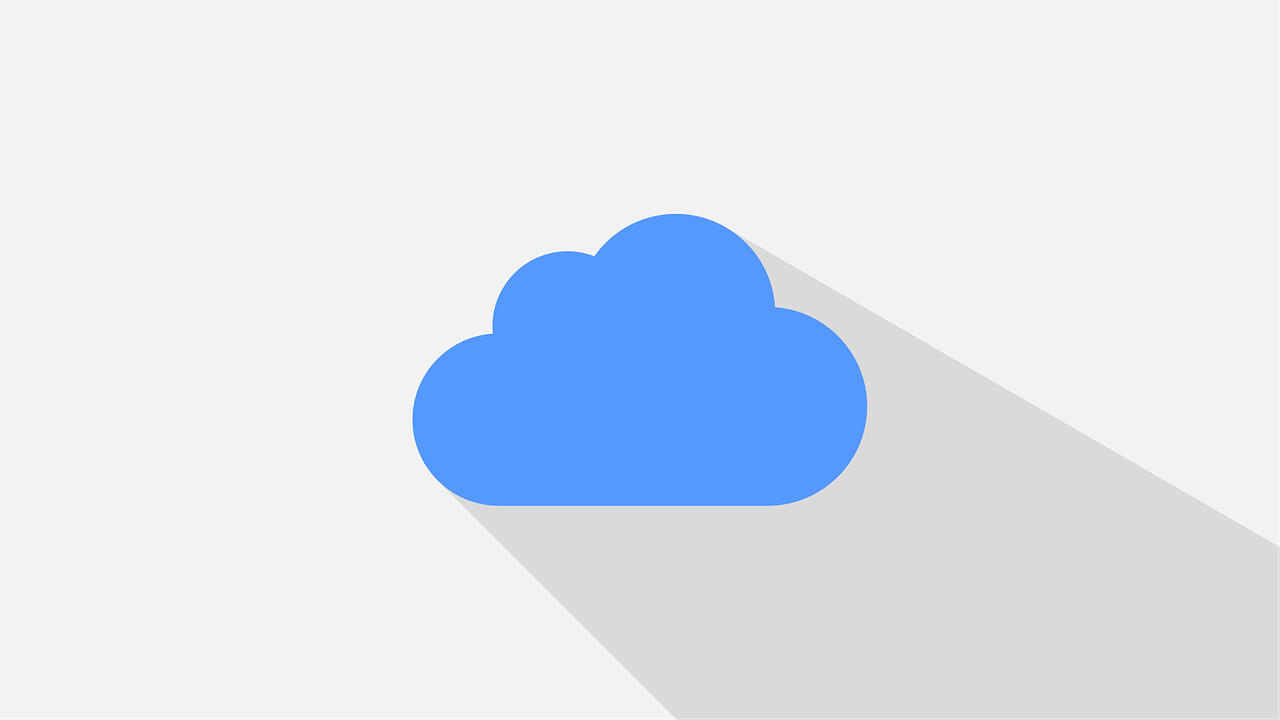
1、我们需要包含所需的头文件:
#include <windows.h>
2、接下来,我们需要定义窗口过程函数,窗口过程函数是处理窗口消息的函数,它接收一个窗口句柄和一个消息类型作为参数,我们需要在这个函数中处理各种消息,例如鼠标点击、键盘输入等。
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam) { switch (uMsg) { case WM_DESTROY: PostQuitMessage(0); return 0; default: return DefWindowProc(hwnd, uMsg, wParam, lParam); } }
3、我们需要注册窗口类并创建窗口:
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { WNDCLASSEX wcex; HWND hwnd; MSG msg; TCHAR szClassName[] = TEXT("MyWindowClass"); wcex.cbSize = sizeof(WNDCLASSEX); wcex.style = CS_HREDRAW | CS_VREDRAW; wcex.lpfnWndProc = WindowProc; wcex.cbClsExtra = 0; wcex.cbWndExtra = 0; wcex.hInstance = hInstance; wcex.hIcon = LoadIcon(NULL, IDI_APPLICATION); wcex.hCursor = LoadCursor(NULL, IDC_ARROW); wcex.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1); wcex.lpszMenuName = NULL; wcex.lpszClassName = szClassName; wcex.hIconSm = LoadIcon(NULL, IDI_APPLICATION); if (!RegisterClassEx(&wcex)) { MessageBox(NULL, TEXT("This program requires Windows NT!"), szClassName, MB_ICONERROR); return 0; } hwnd = CreateWindowEx(WS_EX_CLIENTEDGE, szClassName, TEXT("My Application"), WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, NULL, NULL, hInstance, NULL); if (hwnd == NULL) { MessageBox(NULL, TEXT("Window Creation Error!"), szClassName, MB_ICONERROR); return 0; } ShowWindow(hwnd, nCmdShow); UpdateWindow(hwnd); InitializeCriticalSection(&cs); while (TRUE) { if (PeekMessage(&msg, NULL, 0, 0, PM_REMOVE)) { TranslateMessage(&msg); DispatchMessage(&msg); } else { Sleep(10); // sleep for a while to avoid high CPU usage in this thread. You can remove this line if you don't need it. } } DeleteCriticalSection(&cs); // clean up the critical section when the application is closing. You can remove this line if you don't need it. return msg.wParam; }
4、现在,我们可以在窗口过程中处理各种消息,我们可以处理鼠标点击事件,当用户点击鼠标时,程序会显示一个消息框:
case WM_LBUTTONDOWN: { int xPos = LOWORD(lParam); // horizontal position of the mouse pointer relative to the client area of the window (inclusive). The first coordinate is at the left side of the client area. If the mouse pointer is not in the client area, the value will be negative. If the mouse pointer is in the upperleft corner of the client area, the value will be zero. If the mouse pointer is in the lowerright corner of the client area, the value will be equal to the width of the client area minus one. If the mouse pointer is outside the client area and outside the nonclient area of the window frame, the value will be less than zero. The second coordinate is at the top of the client area. If the mouse pointer is not in the client area, the value will be negative. If the mouse pointer is in the upperleft corner of the client area, the value will be zero. If the mouse pointer is in the lowerright corner of the client area, the value will be equal to the height of the client area minus one. If the mouse pointer is outside the client area and outside the nonclient area of the window frame, the value will be less than zero. The third coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The fourth coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The fifth coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The sixth coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The seventh coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The eighth coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The ninth coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The tenth coordinate is reserved for future use and must be zero. It cannot be negative or greater than zero. The eleventh coordinate is reserved for future use and must be zero. It cannot be negative or greater above tenth line...} break; // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam; } // end case WM_LBUTTONDOWN: ... // end switch (uMsg): ... // end LRESULT CALLBACK WndProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam): ... // end WinMain(): ... // end main() return msg. wParam;
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/365649.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复