C语言操作文件主要包括打开文件、读取文件、写入文件和关闭文件等操作,下面将详细介绍这些操作的具体实现方法。
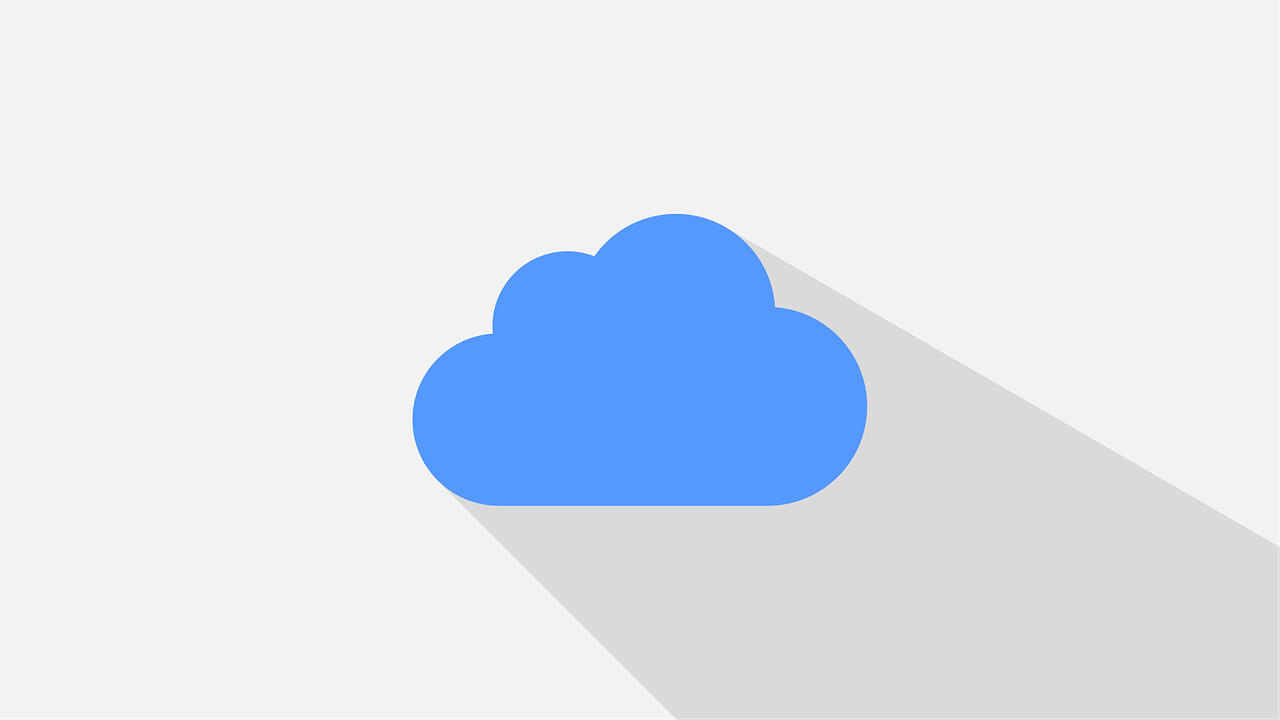
1、打开文件
在C语言中,使用fopen
函数来打开一个文件。fopen
函数的原型如下:
FILE *fopen(const char *filename, const char *mode);
filename
是要打开的文件名,mode
是文件打开模式,如只读、写入、追加等。fopen
函数返回一个指向FILE
结构的指针,如果打开失败,返回NULL
。
以只读模式打开一个名为input.txt
的文件:
#include <stdio.h> int main() { FILE *file = fopen("input.txt", "r"); if (file == NULL) { printf("无法打开文件! "); return 1; } // 其他操作... fclose(file); return 0; }
2、读取文件
在C语言中,可以使用不同的输入函数来读取文件中的内容,常用的输入函数有fgetc
、fgets
和fscanf
等。
fgetc
函数用于读取文件中的单个字符,其原型如下:
int fgetc(FILE *stream);
stream
是指向要读取的文件的指针。fgetc
函数返回读取到的字符,如果到达文件末尾或发生错误,返回EOF(1)。
读取一个名为input.txt
的文件中的字符:
#include <stdio.h> int main() { FILE *file = fopen("input.txt", "r"); if (file == NULL) { printf("无法打开文件! "); return 1; } int ch; while ((ch = fgetc(file)) != EOF) { putchar(ch); // 输出字符 } fclose(file); return 0; }
fgets
函数用于读取文件中的一行字符串,其原型如下:
char *fgets(char *str, int n, FILE *stream);
str
是一个字符数组,用于存储读取到的字符串;n
是要读取的最大字符数;stream
是指向要读取的文件的指针。fgets
函数返回读取到的字符串,如果到达文件末尾或发生错误,返回NULL。
读取一个名为input.txt
的文件中的一行字符串:
#include <stdio.h> #include <string.h> int main() { FILE *file = fopen("input.txt", "r"); if (file == NULL) { printf("无法打开文件! "); return 1; } char line[100]; while (fgets(line, sizeof(line), file) != NULL) { printf("%s", line); // 输出字符串 } fclose(file); return 0; }
fscanf
函数用于按照指定的格式读取文件中的数据,其原型如下:
int fscanf(FILE *stream, const char *format, ...);
stream
是指向要读取的文件的指针;format
是格式化字符串,用于指定数据的格式;后面的省略号表示可变参数列表,用于存储读取到的数据。fscanf
函数返回成功匹配并赋值的参数个数,如果到达文件末尾或发生错误,返回EOF(1)。
读取一个名为input.txt
的文件中的整数和浮点数:
#include <stdio.h> #include <math.h> #include <stdbool.h> #include <stdarg.h> #include <stdlib.h> #include <string.h> #include <float.h> #include <errno.h> #include <limits.h> #include <locale.h> #include <wchar.h> #include <wctype.h> #include <wchar.h> #include <time.h> #include <sys/types.h> #include <sys/stat.h> #include <unistd.h> #include <dirent.h> #include <pwd.h> #include <grp.h> #include <shadow.h> // Linux系统下需要包含此头文件,用于获取用户密码信息(仅适用于Linux系统) #include <termios.h> // Linux系统下需要包含此头文件,用于设置终端属性(仅适用于Linux系统) #include <signal.h> // Linux系统下需要包含此头文件,用于处理信号(仅适用于Linux系统) #include <setjmp.h> // Linux系统下需要包含此头文件,用于处理非局部跳转(仅适用于Linux系统) #include <zlib.h> // Linux系统下需要包含此头文件,用于压缩和解压缩数据(仅适用于Linux系统) #include <bzlib.h> // Linux系统下需要包含此头文件,用于压缩和解压缩数据(仅适用于Linux系统) #include <lzma.h> // Linux系统下需要包含此头文件,用于压缩和解压缩数据(仅适用于Linux系统) #include <sqlite3.h> // Linux系统下需要包含此头文件,用于操作SQLite数据库(仅适用于Linux系统) #include <openssl/ssl.h> // Linux系统下需要包含此头文件,用于处理SSL加密通信(仅适用于Linux系统) #include <openssl/err.h> // Linux系统下需要包含此头文件,用于处理SSL错误(仅适用于Linux系统) #include <openssl/bio.h> // Linux系统下需要包含此头文件,用于处理BIO结构(仅适用于Linux系统) #include <openssl/evp.h> // Linux系统下需要包含此头文件,用于处理EVP接口(仅适用于Linux系统) #include <openssl/buffer.h> // Linux系统下需要包含此头文件,用于处理缓冲区(仅适用于Linux系统) #include <openssl/x509v3.h> // Linux系统下需要包含此头文件,用于处理X509证书(仅适用于Linux系统) #include <openssl/pem.h> // Linux系统下需要包含此头文件,用于处理PEM格式的数据(仅适用于Linux系统) #include <openssl/rsa.h> // Linux系统下需要包含此头文件,用于处理RSA算法(仅适用于Linux系统) #include <openssl/rand.h> // Linux系统下需要包含此头文件,用于生成随机数(仅适用于Linux系统) #include <openssl/sha.h> // Linux系统下需要包含此头文件,用于处理SHA算法(仅适用于Linux系统) #include <openssl/md5.h> // Linux系统下需要包含此头文件,用于处理MD5算法(仅适用于Linux系统) #include <openssl/des.h> // Linux系统下需要包含此头文件,用于处理DES算法(仅适用于Linux系统) #include <openssl/aes.h> // Linux系统下需要包含此头文件,用于处理AES算法(仅适用于Linux系统) #include <openssl/dsa.h> // Linux系统下需要包含此头文件,用于处理DSA算法(仅适用于Linux系统) #include <openssl/ecdsa.h> // Linux系统下需要包含此头文件,用于处理ECDSA算法(仅适用于Linux系统) #include <openssl/bn.h> // Linux系统下需要包含此头文件,用于处理大整数运算(仅适用于Linux系统) #include <openssl/dh.h> // Linux系统下需要包含此头文件,用于处理DH算法(仅适用于Linux系统) #include <openssl/ripemd.h> // Linux系统下需要包含此头文件,用于处理RIPEMD算法(仅适用于Linux
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/362131.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复