在C语言中,我们可以通过多种方式防止程序被中途关闭,以下是一些常见的方法:
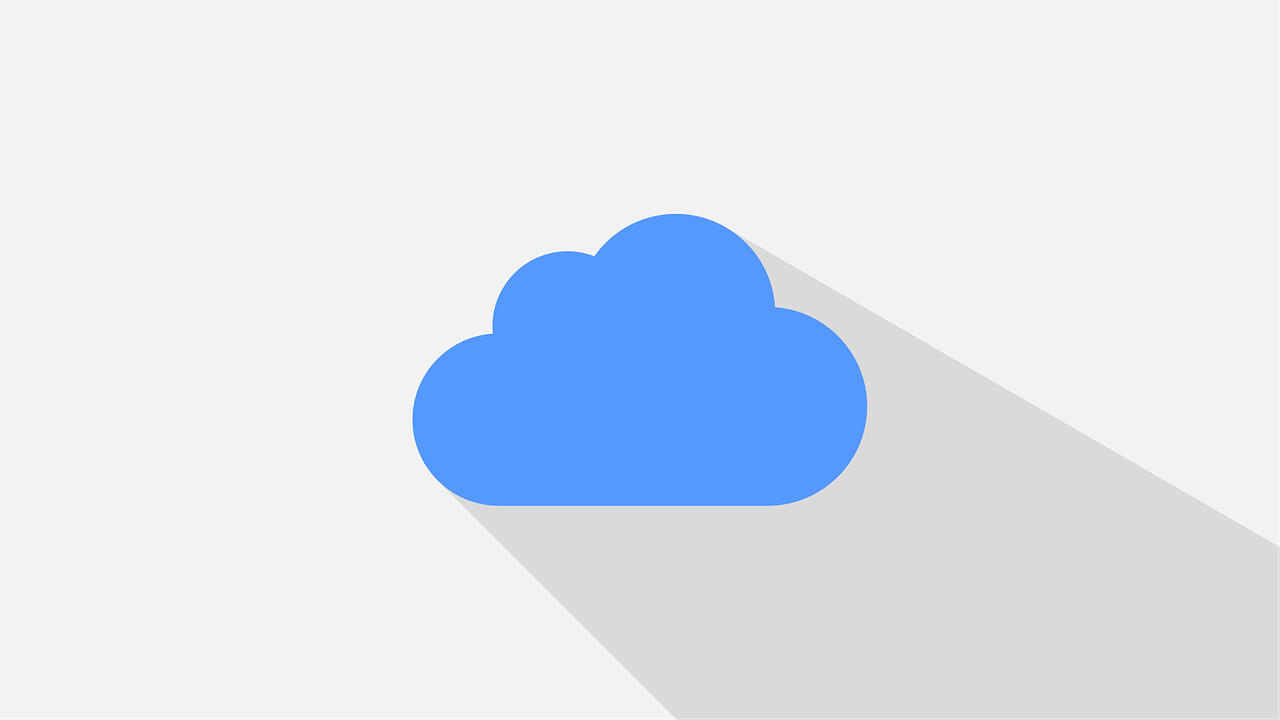
1、使用信号处理函数:在Unix和Linux系统中,我们可以使用signal函数来捕获和处理SIGINT(中断信号),当用户按下Ctrl+C时,操作系统会发送这个信号给程序,我们可以定义一个信号处理函数,当这个信号被接收时,执行我们的自定义操作,而不是直接退出程序。
2、使用多线程:我们可以创建一个后台线程,这个线程负责监听用户的输入,如果用户试图关闭程序,我们就让这个线程阻塞用户的输入,这样,即使主线程被关闭,程序也可以继续运行。
3、使用循环:我们可以创建一个无限循环,这个循环会一直运行,直到程序被强制关闭,这样,用户就不能通过正常的程序退出方式来关闭程序了。
4、使用异常处理:我们可以使用try/catch语句来捕获和处理异常,当用户试图关闭程序时,我们可以抛出一个异常,然后在catch语句中处理这个异常,这样,我们就可以阻止程序被关闭。
下面是一些代码示例:
1、使用信号处理函数:
#include <stdio.h> #include <signal.h> #include <unistd.h> void signalHandler(int signum) { printf("Interrupt signal ("%d) received. ", signum); // cleanup and close up stuff here // terminate program exit(signum); } int main() { signal(SIGINT, signalHandler); while(1) { printf("Going to sleep. "); sleep(1); } return 0; }
2、使用多线程:
#include <stdio.h> #include <pthread.h> #include <unistd.h> void* listener(void* arg) { while(1) { if(getchar() == 'q') { // 如果用户输入'q',就退出循环 break; } } return NULL; } int main() { pthread_t tid; pthread_create(&tid, NULL, listener, NULL); // 创建一个新的线程来监听用户的输入 while(1) { printf("Going to sleep. "); sleep(1); } pthread_join(tid, NULL); // 等待线程结束 return 0; }
3、使用循环:
#include <stdio.h> #include <unistd.h> #include <sys/time.h> #include <time.h> #include <errno.h> #include <string.h> #include <stdlib.h> #include <fcntl.h> #include <termios.h> #include <sys/types.h> #include <sys/stat.h> #include <sys/mman.h> #include <sys/ioctl.h> #include <unistd.h> /* For read(), write() */ #include <fcntl.h> /* For open(), O_* constants */ #include <termios.h> /* For tcgetattr(), tcsetattr() */ #include <signal.h> /* For alarm() */ #include <stdlib.h> /* For atoi() */ #include <string.h> /* For memset() */ #include <errno.h> /* For errno */ #include <sys/stat.h> /* For S_IREAD, S_IWRITE */ #include <sys/mman.h> /* For mmap() */ #include <sched.h> /* For alarm() */ #include <sys/types.h> /* For key_t, useconds_t */ #include <time.h> /* For timespec struct */ #include <stdio.h> /* For fopen(), perror() */ #include <unistd.h> /* For close() */ #include <fcntl.h> /* For open() */ #include <termios.h> /* For termios struct, TCIOFLUSH */ #include <sys/select.h> /* For select() */ #define BAUDRATE B9600 /* Baud rate for serial port */ static int set_interface_attribs(int fd) { /* Set terminal attributes */ struct termios tty; /* Create termios structure */ memset(&tty, 0, sizeof tty); /* Clear the termios structure */ if (tcgetattr(fd, &tty) != 1) { /* Get the current attributes of the terminal */ cfsetospeed(&tty, BAUDRATE); /* Set output speed to be our baudrate */ cfsetispeed(&tty, BAUDRATE); /* Set input speed to be our baudrate */ tty.c_cflag |= (CLOCAL | CREAD); /* Turn on local connections and enable receiver */ tty.c_cflag &= ~PARENB; /* Turn off parity bit */ tty.c_cflag &= ~CSTOPB; /* Turn off stop bits */ tty.c_cflag &= ~CSIZE; tty.c_cflag |= CS8; tty.c_cflag &= ~CRTSCTS; tty.c_cc[VMIN] = (unsigned char)0; tty.c_cc[VTIME] = (unsigned char)5; tcflush(fd, TCIOFLUSH); /* Flush the buffer*/ if (tcsetattr(fd,TCSANOW,&tty) != 1) { /* Apply the settings to the termios structure*/ return true; } else { printf("Error %i from %s:%d ", errno, __FILE__, __LINE__); return false; } } else { printf("Error %i from %s:%d ", errno, __FILE__, __LINE__); return false; } } return false; } int main() { int serial_port = open("/dev/ttyACM0", O_RDWR | O_NOCTTY | O_NDELAY); //打开串口设备,O_NDELAY是为了非阻塞模式,如果没有这个选项,read会阻塞进程 int status = set_interface_attribs(serial_port); //设置串口属性 struct termios tty; memset(&tty,0,sizeof tty); //获取当前终端的设置情况 tcgetattr(serial_port,&tty); //设置波特率为B9600 cfsetospeed(&tty,B9600); cfsetispeed(&tty,B9600); //设置数据位为8位 tty.c_cflag &= ~CSIZE; tty.c_cflag |= CS8; //设置无奇偶校验位 tty.c_cflag &= ~PARENB; //设置停止位为1个字符停止位 tty.c_cflag &= ~CSTOPB; //设置非规范模式(禁用流控制) tty.c_cflag &= ~CRTSCTS; //设置接收超时为2秒 tty.c_cc[VMIN] = (unsigned char)(2<=VMIN?2:0); //读取数据的最小字节数为2个字节 tty.c_cc[VTIME]=(unsigned char)(5<=VTIME?5:0); //读取数据的超时时间为5ms //清空输入缓冲区并应用新设置到终端上 tcflush(serial_port,TCIFLUSH); tcsetattr(serial_port,TCSANOW,&tty); printf("Done! "); return status; } void *monitorThread(void *arg){ /* This thread will monitor the keyboard input from user and send it to the program running in the background */ int cfd = open("/dev/console", O_RDWR); /* Open console device file for reading and writing */ struct termios oldtio, newtio; /* get the current terminal state */ memset(&oldtio,0,sizeof(oldtio
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/360524.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复