在HTML中编写计数器通常涉及到使用JavaScript,因为HTML本身不具备逻辑处理能力,下面是如何通过HTML、CSS和JavaScript实现一个简单的点击计数器的详细步骤:
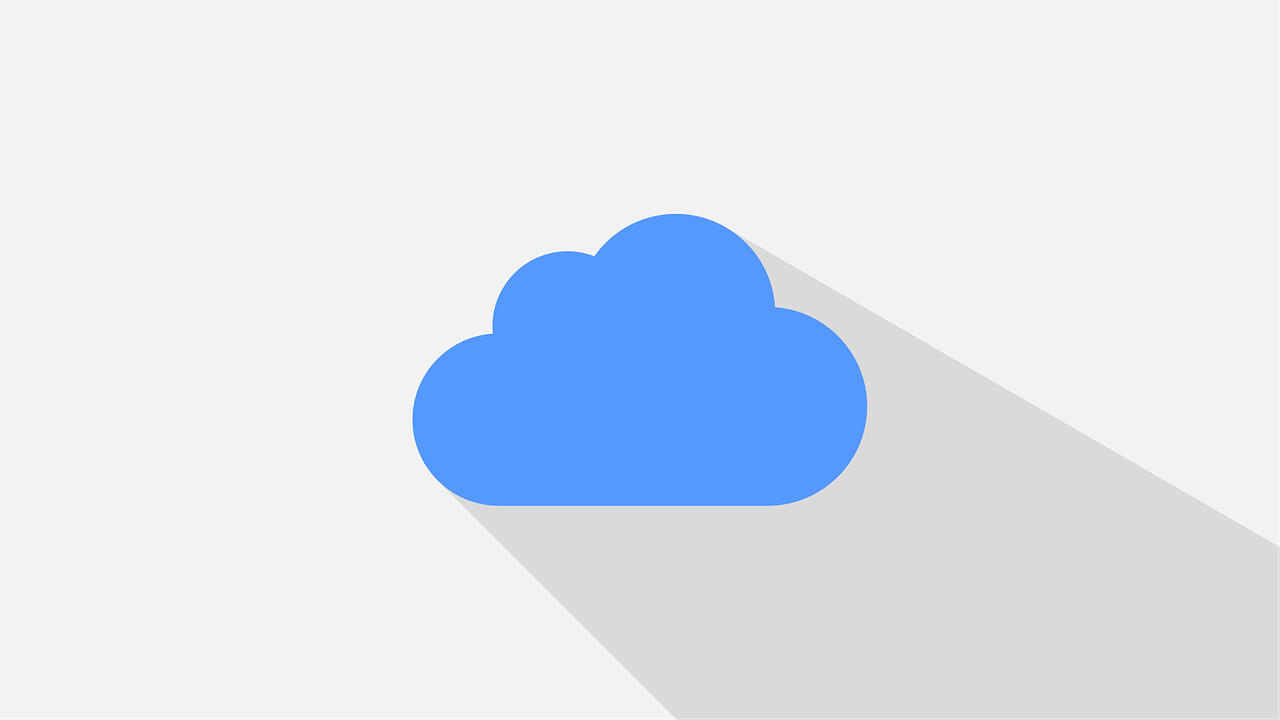
步骤1:创建HTML结构
我们需要创建一个按钮和一个显示点击次数的文本区域。
<!DOCTYPE html> <html lang="zh"> <head> <meta charset="UTF8"> <title>点击计数器</title> </head> <body> <button id="counterButton">点击我</button> <p>点击次数: <span id="counterDisplay">0</span></p> </body> </html>
这里我们为按钮设置了一个ID counterButton
,用于后续的JavaScript操作,同样地,我们也给显示点击次数的<span>
元素设置了一个ID counterDisplay
。
步骤2:添加样式(可选)
接下来,你可以使用CSS来美化你的计数器,我们可以给计数器添加一些基本样式:
body { fontfamily: Arial, sansserif; textalign: center; } #counterButton { padding: 10px 20px; fontsize: 16px; } #counterDisplay { fontweight: bold; margintop: 10px; }
步骤3:编写JavaScript逻辑
现在我们需要编写JavaScript代码来增加点击事件,并在每次点击时更新显示的点击次数。
<script> let count = 0; // 初始化计数器变量 const counterDisplay = document.getElementById('counterDisplay'); // 获取显示点击次数的元素 const button = document.getElementById('counterButton'); // 获取按钮元素 // 定义一个函数来更新计数器和显示值 function updateCounter() { count++; // 每次调用时,计数器加一 counterDisplay.textContent = count; // 更新显示的点击次数 } // 为按钮添加点击事件监听器,并绑定updateCounter函数 button.addEventListener('click', updateCounter); </script>
步骤4:将以上代码整合到一起
将上述HTML、CSS和JavaScript代码组合到一个完整的HTML文件中:
<!DOCTYPE html> <html lang="zh"> <head> <meta charset="UTF8"> <title>点击计数器</title> <style> body { fontfamily: Arial, sansserif; textalign: center; } #counterButton { padding: 10px 20px; fontsize: 16px; } #counterDisplay { fontweight: bold; margintop: 10px; } </style> </head> <body> <button id="counterButton">点击我</button> <p>点击次数: <span id="counterDisplay">0</span></p> <script> let count = 0; const counterDisplay = document.getElementById('counterDisplay'); const button = document.getElementById('counterButton'); function updateCounter() { count++; counterDisplay.textContent = count; } button.addEventListener('click', updateCounter); </script> </body> </html>
现在当你在浏览器中打开这个HTML文件,你将看到一个按钮,每当你点击它时,旁边的数字就会递增,显示你点击的次数,这就是一个基本的HTML点击计数器,当然,你可以根据需要进一步美化样式或添加更多功能,比如使用本地存储来保持计数状态或者添加重置按钮等。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/350121.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复