在C语言中,插入一个成绩通常指的是将一个新的成绩添加到已有的成绩集合中,这可以通过多种方式实现,例如使用数组、链表或其他数据结构,下面我会展示如何使用数组和链表来插入成绩。
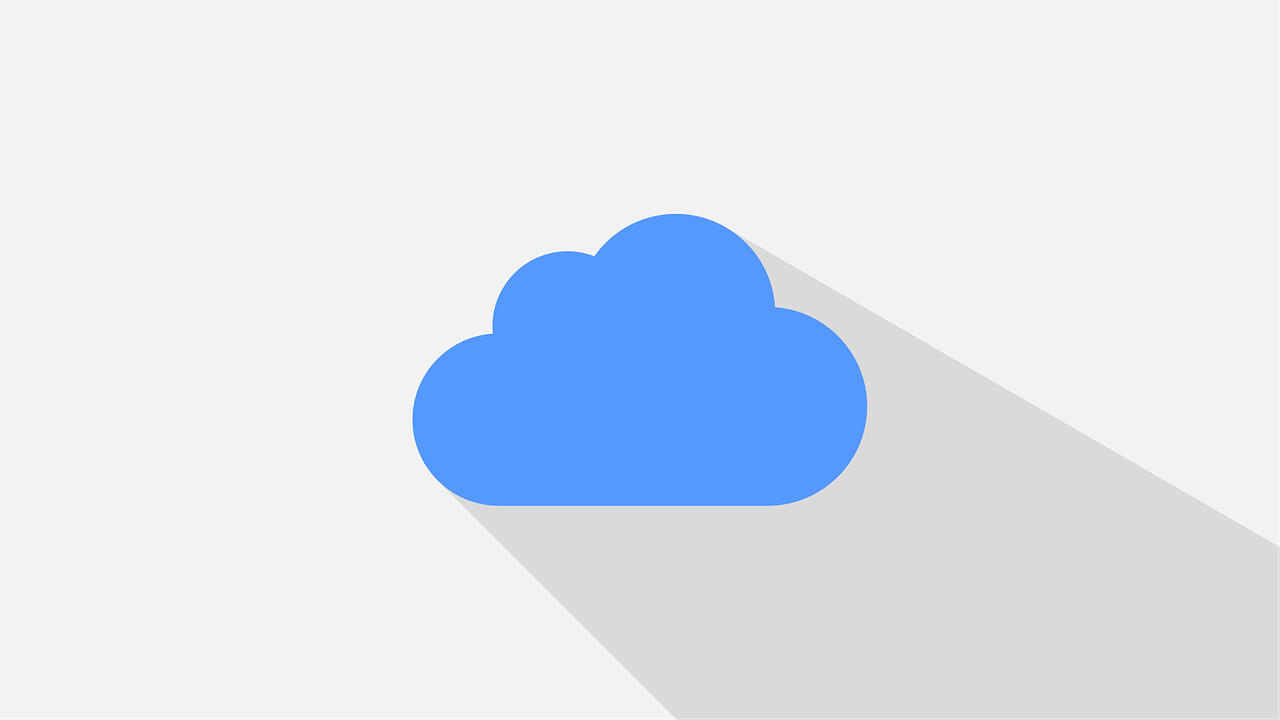
使用数组插入成绩
1. 定义成绩数组
#include <stdio.h> #define MAX_SIZE 100 // 假设最多有100个学生 int scores[MAX_SIZE]; int count = 0; // 记录当前已存储的成绩数量
2. 插入成绩函数
插入成绩时,需要确保数组未满,如果数组已满,则不能插入新的成绩。
void insert_score(int score) { if (count < MAX_SIZE) { scores[count] = score; count++; } else { printf("成绩数组已满,无法插入新的成绩。 "); } }
3. 示例代码
int main() { // 插入几个成绩作为示例 insert_score(85); insert_score(90); insert_score(78); // 打印所有成绩 for (int i = 0; i < count; i++) { printf("Score %d: %d ", i+1, scores[i]); } return 0; }
使用链表插入成绩
1. 定义结构体和节点
#include <stdio.h> #include <stdlib.h> typedef struct Node { int score; struct Node* next; } Node; Node* head = NULL; // 链表的头指针
2. 插入成绩函数
void insert_score(int score) { Node* new_node = (Node*)malloc(sizeof(Node)); new_node>score = score; new_node>next = head; head = new_node; }
3. 示例代码
int main() { // 插入几个成绩作为示例 insert_score(85); insert_score(90); insert_score(78); // 打印所有成绩 Node* temp = head; while (temp != NULL) { printf("Score: %d ", temp>score); temp = temp>next; } // 释放内存 temp = head; while (temp != NULL) { Node* temp_next = temp>next; free(temp); temp = temp_next; } return 0; }
小结
使用数组插入成绩时,操作简单但数组大小固定,不利于扩展。
使用链表插入成绩时,操作略复杂,但可以动态扩展,不受大小限制。
在实际开发中,选择哪种方法取决于具体的需求和场景,如果要处理的成绩数量不确定或非常大,建议使用链表,如果成绩数量较小且确定,可以使用数组来简化操作,无论使用哪种方式,都需要注意内存管理和错误处理,以确保程序的稳定性和可靠性。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/347470.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复