在Python中查找字符串是一项基本的操作,通常涉及到几种不同的方法和场景,以下是一些常用的方法来在字符串中查找子字符串,以及它们的具体使用方式和示例代码。
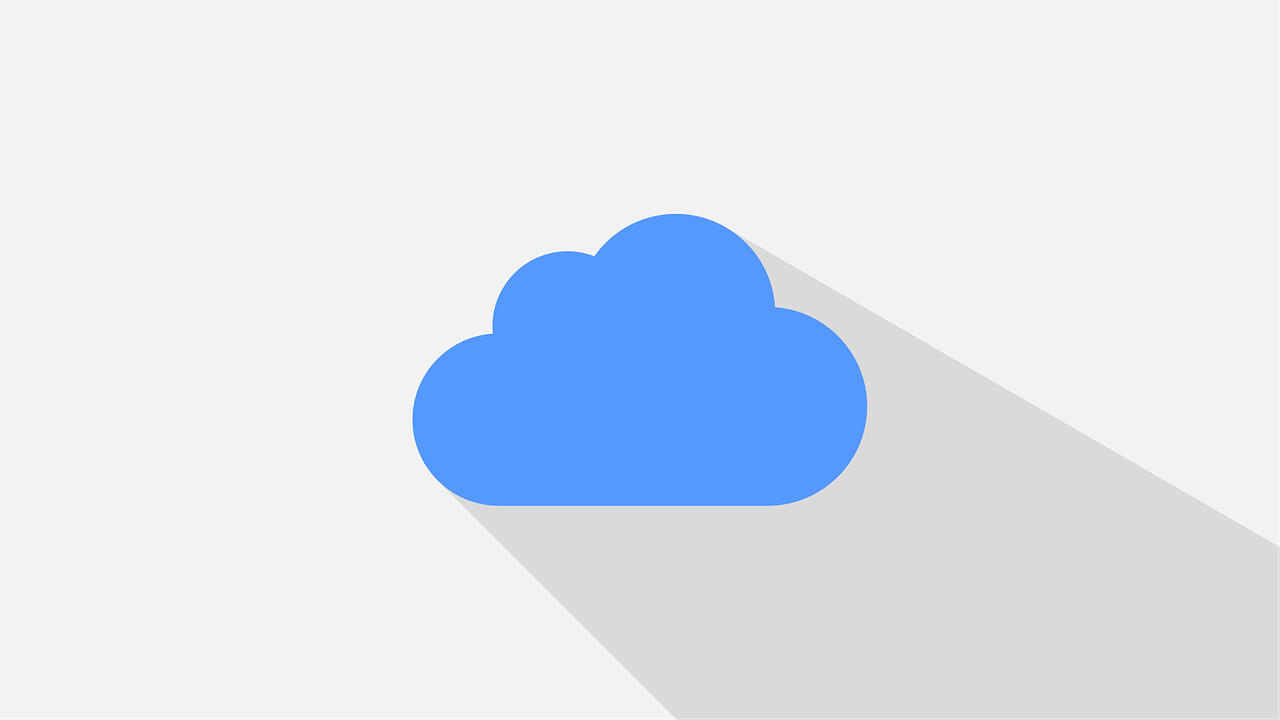
1. 直接使用 in
关键字
最简单的方法是使用 in
关键字来检查一个字符串是否包含另一个字符串。
text = "Python编程语言" search_str = "编程" if search_str in text: print("找到了!") else: print("未找到!")
2. 使用 find()
方法
find()
方法可以返回子字符串在字符串中首次出现的位置(索引),如果没有找到则返回1。
text = "Python编程语言" search_str = "编程" position = text.find(search_str) if position != 1: print(f"找到了,位置在:{position}") else: print("未找到!")
3. 使用 index()
方法
index()
方法与 find()
类似,但如果找不到子字符串,它会引发一个异常。
text = "Python编程语言" search_str = "编程" try: position = text.index(search_str) print(f"找到了,位置在:{position}") except ValueError: print("未找到!")
4. 使用正则表达式 re
模块
对于复杂的查找和匹配操作,可以使用Python的 re
模块。
import re text = "Python编程语言" pattern = "编程" match = re.search(pattern, text) if match: print(f"找到了,位置在:{match.start()}") else: print("未找到!")
5. 使用 count()
方法
如果你需要知道某个子字符串在字符串中出现的次数,可以使用 count()
方法。
text = "Python编程语言Python" search_str = "Python" occurrences = text.count(search_str) print(f"'{search_str}' 出现了 {occurrences} 次")
6. 使用切片操作进行查找
切片可以用来获取字符串的一部分,并以此来判断某个子字符串是否存在。
text = "Python编程语言" search_str = "编程" if text[len(search_str):] == search_str: print("找到了!") else: print("未找到!")
7. 使用 startswith()
和 endswith()
方法
如果你想检查字符串是否以特定的子字符串开始或结束,可以使用 startswith()
和 endswith()
方法。
text = "Python编程语言" if text.startswith("Python"): print("文本以 'Python' 开头") if text.endswith("语言"): print("文本以 '语言' 结尾")
8. 使用循环逐个字符检查
尽管不是最高效的方法,但有时你可能需要通过遍历字符串中的每个字符来查找子字符串。
text = "Python编程语言" search_str = "编程" length = len(search_str) for i in range(len(text) length + 1): if text[i:i+length] == search_str: print("找到了!") break else: print("未找到!")
以上是Python中查找字符串的一些常用方法和技巧,根据你的具体需求,选择最合适的方法,在使用这些方法时,请注意字符串的大小写敏感性,必要时可以通过 lower()
或 upper()
方法将字符串转换为统一的大小写来进行比较。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/317223.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复