在Python中,字符串是比较常用的数据类型,我们经常需要对字符串进行比较,本文将详细介绍如何在Python中比较字符串,以及相关的技术教学。
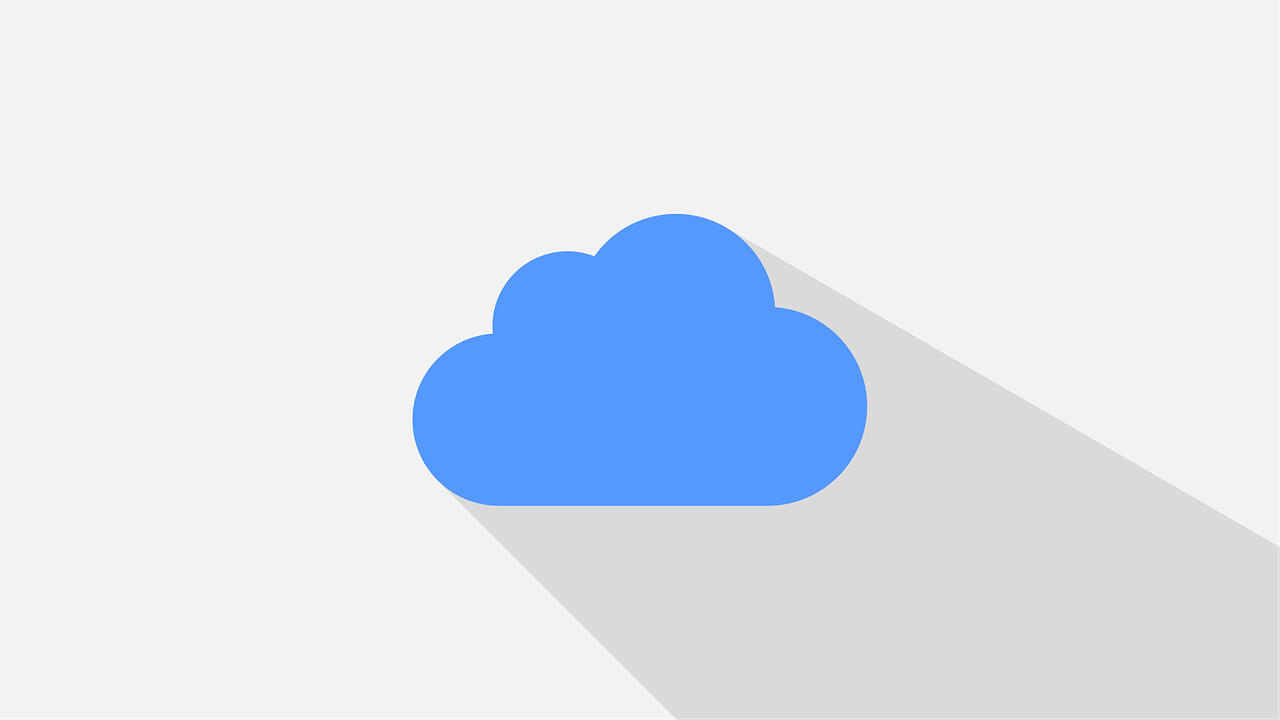
字符串比较的基本概念
在Python中,字符串比较是基于字符的Unicode编码进行的,比较时,会逐个比较字符串中的字符,直到找到不同的字符或者比较完所有字符,字符串比较的结果有以下三种情况:
1、字符串1 < 字符串2:表示字符串1小于字符串2;
2、字符串1 == 字符串2:表示字符串1等于字符串2;
3、字符串1 > 字符串2:表示字符串1大于字符串2。
字符串比较的方法
在Python中,可以使用双等号(==)和单等号(=)进行字符串比较,双等号用于判断两个字符串是否相等,单等号用于赋值。
str1 = "hello" str2 = "world" str3 = "hello" 判断两个字符串是否相等 if str1 == str2: print("str1 and str2 are equal") else: print("str1 and str2 are not equal") 判断两个字符串是否不相等 if str1 != str2: print("str1 and str2 are not equal") else: print("str1 and str2 are equal") 判断一个字符串是否大于另一个字符串 if str1 > str2: print("str1 is greater than str2") else: print("str1 is not greater than str2") 判断一个字符串是否小于另一个字符串 if str1 < str2: print("str1 is less than str2") else: print("str1 is not less than str2")
字符串比较的技巧
1、使用lower()方法将字符串转换为小写后再进行比较,可以避免因大小写不同导致的比较错误。
str1 = "Hello" str2 = "hello" if str1.lower() == str2.lower(): print("str1 and str2 are equal") else: print("str1 and str2 are not equal")
2、使用startswith()和endswith()方法判断字符串是否以某个特定字符串开头或结尾。
str1 = "hello world" if str1.startswith("hello"): print("str1 starts with 'hello'") else: print("str1 does not start with 'hello'") if str1.endswith("world"): print("str1 ends with 'world'") else: print("str1 does not end with 'world'")
实际应用案例
假设我们需要编写一个程序,判断用户输入的密码是否符合以下要求:
1、密码长度至少为8个字符;
2、密码必须包含至少一个大写字母;
3、密码必须包含至少一个小写字母;
4、密码必须包含至少一个数字。
我们可以使用字符串比较的方法来实现这个功能:
def check_password(password): if len(password) < 8: return "Password length should be at least 8 characters" if not any(char.isupper() for char in password): return "Password should contain at least one uppercase letter" if not any(char.islower() for char in password): return "Password should contain at least one lowercase letter" if not any(char.isdigit() for char in password): return "Password should contain at least one digit" return "Password is valid" password = input("Please enter your password: ") print(check_password(password))
本文详细介绍了在Python中如何比较字符串,包括字符串比较的基本概念、方法、技巧以及实际应用案例,通过学习本文,你将掌握字符串比较的相关技术,并能在实际编程中灵活运用。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/303104.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复