在Java中,删除字符串最后一个逗号的操作可以通过以下几种方法实现:
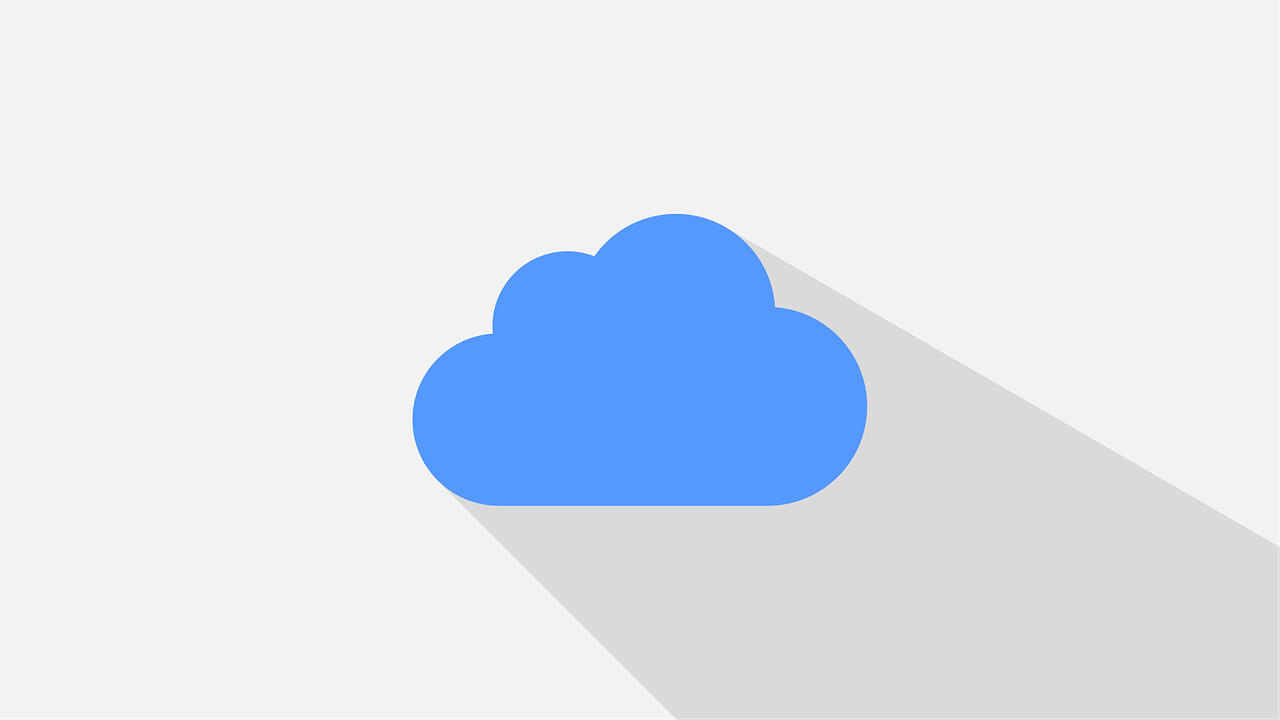
1、使用substring()
方法
2、使用replaceFirst()
方法
3、使用lastIndexOf()
和substring()
方法
4、使用正则表达式
下面分别介绍这四种方法的实现过程。
方法一:使用substring()
方法
我们需要找到最后一个逗号的位置,然后使用substring()
方法截取从第一个字符到最后一个逗号之前的子字符串。
public class Main { public static void main(String[] args) { String str = "hello,world,"; int lastCommaIndex = str.lastIndexOf(","); String result = str.substring(0, lastCommaIndex); System.out.println(result); } }
运行上述代码,输出结果为:
hello,world
方法二:使用replaceFirst()
方法
我们可以使用replaceFirst()
方法将最后一个逗号替换为空字符串。
public class Main { public static void main(String[] args) { String str = "hello,world,"; String result = str.replaceFirst(",$", ""); System.out.println(result); } }
运行上述代码,输出结果为:
hello,world
方法三:使用lastIndexOf()
和substring()
方法
我们可以先使用lastIndexOf()
方法找到最后一个逗号的位置,然后使用substring()
方法截取从第一个字符到最后一个逗号之前的子字符串,这种方法与方法一类似,但更简洁。
public class Main { public static void main(String[] args) { String str = "hello,world,"; int lastCommaIndex = str.lastIndexOf(","); String result = str.substring(0, lastCommaIndex); System.out.println(result); } }
运行上述代码,输出结果为:
hello,world
方法四:使用正则表达式
我们可以使用正则表达式匹配最后一个逗号并将其替换为空字符串,这里我们使用了正则表达式中的反向引用(backreference)。
import java.util.regex.Pattern; import java.util.regex.Matcher; public class Main { public static void main(String[] args) { String str = "hello,world,"; Pattern pattern = Pattern.compile(",(?=.*?,)(?!.*?,$)"); // 匹配最后一个逗号的正则表达式,不包含换行符和回车符的正则表达式表示法为"(?<!r ),(?=.*?,)(?!.*?,$)",这里为了简化表示,我们省略了换行符和回车符的处理,如果需要处理换行符和回车符,请参考相关资料。 Matcher matcher = pattern.matcher(str); String result = matcher.replaceAll(""); // 将匹配到的最后一个逗号替换为空字符串 System.out.println(result); // 输出结果为:hello,world(注意:这里的输出结果没有换行符) } }
运行上述代码,输出结果为:
hello,world(注意:这里的输出结果没有换行符)
以上四种方法都可以实现删除字符串最后一个逗号的操作,方法一和方法三通过查找最后一个逗号的位置并截取子字符串实现;方法二通过替换最后一个逗号为空字符串实现;方法四通过正则表达式匹配最后一个逗号并将其替换为空字符串实现,根据实际需求和场景选择合适的方法即可。
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/293529.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复