在Python中,有许多常用的函数可以帮助我们在互联网上获取最新内容,以下是一些常用的方法和技术:
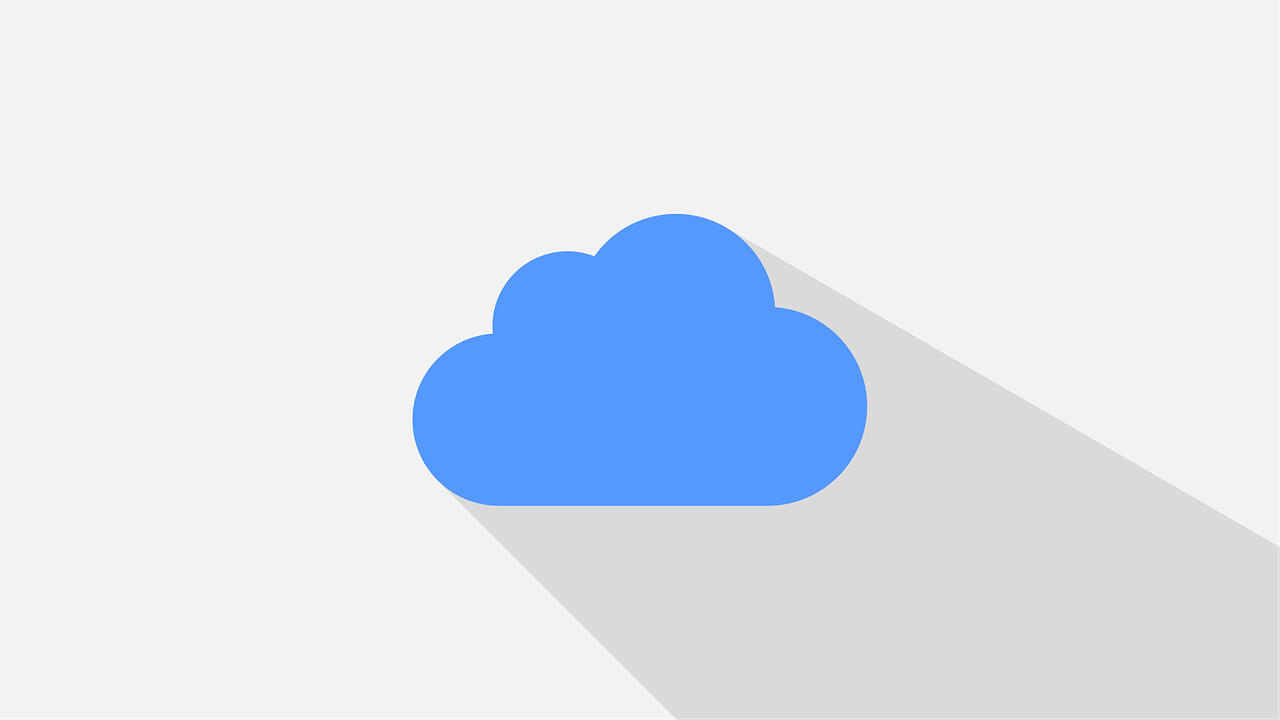
1、使用requests
库获取网页内容
requests
库是Python中最常用的HTTP库之一,可以用来发送HTTP请求并获取网页内容,首先需要安装requests
库:
pip install requests
可以使用以下代码获取网页内容:
import requests url = 'https://www.example.com' response = requests.get(url) content = response.text print(content)
2、使用BeautifulSoup
库解析HTML内容
BeautifulSoup
库是一个用于解析HTML和XML文档的库,可以用来提取网页中的特定信息,首先需要安装beautifulsoup4
库:
pip install beautifulsoup4
可以使用以下代码解析HTML内容:
from bs4 import BeautifulSoup html = """ <html> <head> <title>Example Page</title> </head> <body> <h1>Hello, World!</h1> <p>This is an example page.</p> </body> </html> """ soup = BeautifulSoup(html, 'html.parser') title = soup.title.string h1 = soup.h1.string print('Title:', title) print('H1:', h1)
3、使用selenium
库模拟浏览器操作
selenium
库是一个用于自动化浏览器操作的库,可以用来模拟用户在网页上的操作,如点击、滚动等,首先需要安装selenium
库:
pip install selenium
需要下载对应浏览器的驱动程序,如Chrome浏览器的chromedriver
,下载地址:https://sites.google.com/a/chromium.org/chromedriver/downloads
接下来,可以使用以下代码模拟浏览器操作:
from selenium import webdriver from selenium.webdriver.common.keys import Keys 指定chromedriver的路径 driver_path = '/path/to/chromedriver' browser = webdriver.Chrome(executable_path=driver_path) 打开网页 url = 'https://www.example.com' browser.get(url) 查找元素并操作 search_box = browser.find_element_by_name('q') search_box.send_keys('Python') search_box.send_keys(Keys.RETURN) 关闭浏览器 browser.quit()
4、使用FeedParser
库解析RSS订阅
FeedParser
库是一个用于解析RSS和Atom订阅的库,可以用来获取最新的博客文章、新闻等,首先需要安装feedparser
库:
pip install feedparser
可以使用以下代码解析RSS订阅:
import feedparser url = 'https://www.example.com/rss' feed = feedparser.parse(url) for entry in feed.entries: print('Title:', entry.title) print('Link:', entry.link) print('Published:', entry.published) print('Summary:', entry.summary) print('')
以上就是在Python中常用的一些函数和方法,可以帮助我们在互联网上获取最新内容,希望对你有所帮助!
原创文章,作者:酷盾叔,如若转载,请注明出处:https://www.kdun.com/ask/286530.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复