XMLHttpRequest
和fetch
来监听所有Ajax请求。,,“javascript,(function(open) {, XMLHttpRequest.prototype.open = function(method, url, async, user, pass) {, this.addEventListener('load', function() {, console.log('Ajax request made: ' + method + ' ' + url);, }, false);, open.apply(this, arguments);, };,})(XMLHttpRequest.prototype.open);,,if (window.fetch) {, window.fetch = function() {, console.log('Ajax request made: ' + arguments[0]);, return originalFetch.apply(this, arguments);, };,},
“JavaScript监听全部Ajax请求事件的方法有多种,其中一种常见的方法是通过重写XMLHttpRequest的原生方法来实现,以下是详细的步骤和代码示例:
1. 创建一个新的XMLHttpRequest对象
我们需要创建一个自定义的XMLHttpRequest对象,用于拦截并处理所有的Ajax请求。
var originalXHR = window.XMLHttpRequest; function CustomXHR() { this._xhr = new originalXHR(); }
重写open方法
我们需要重写open
方法,以便在每次Ajax请求开始时进行拦截。
CustomXHR.prototype.open = function(method, url, async, user, password) { this._xhr.open(method, url, async, user, password); console.log('Ajax request: ' + method + ' ' + url); };
重写send方法
我们需要重写send
方法,以便在每次Ajax请求发送时进行拦截。
CustomXHR.prototype.send = function(body) { console.log('Sending Ajax request with body: ' + body); this._xhr.send(body); };
4. 重写setRequestHeader方法
为了能够捕获请求头信息,我们还需要重写setRequestHeader
方法。
CustomXHR.prototype.setRequestHeader = function(header, value) { this._xhr.setRequestHeader(header, value); };
5. 重写getResponseHeader方法
同样地,我们也需要重写getResponseHeader
方法,以便在每次Ajax响应到达时进行拦截。
CustomXHR.prototype.getResponseHeader = function(header) { return this._xhr.getResponseHeader(header); };
6. 重写getAllResponseHeaders方法
我们还应该重写getAllResponseHeaders
方法。
CustomXHR.prototype.getAllResponseHeaders = function() { return this._xhr.getAllResponseHeaders(); };
7. 重写responseText和responseXML方法
我们需要重写responseText
和responseXML
方法,以便在每次Ajax响应到达时进行拦截。
CustomXHR.prototype.responseText = function() { return this._xhr.responseText; }; CustomXHR.prototype.responseXML = function() { return this._xhr.responseXML; };
8. 替换全局XMLHttpRequest对象
我们将自定义的XMLHttpRequest对象替换为全局的XMLHttpRequest对象。
window.XMLHttpRequest = CustomXHR;
测试代码
下面是一个简单的测试代码,用于验证我们的自定义XMLHttpRequest对象是否工作正常。
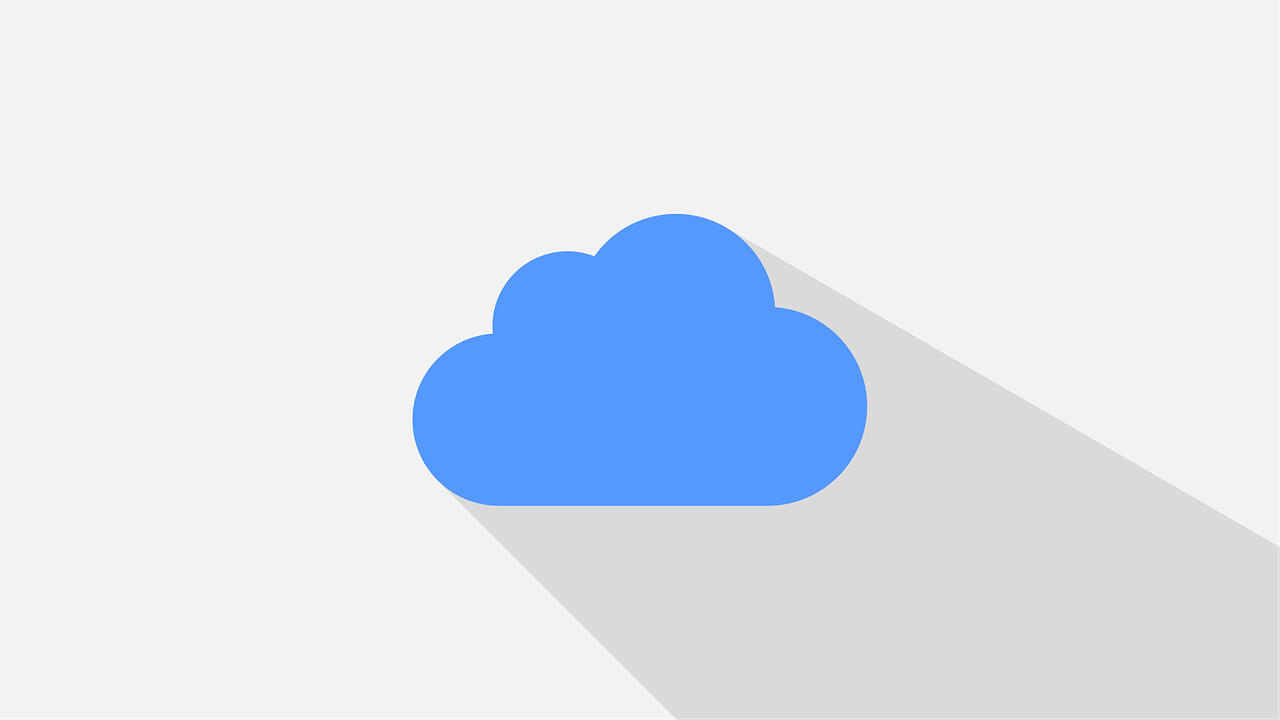
var xhr = new XMLHttpRequest(); xhr.open('GET', 'https://jsonplaceholder.typicode.com/posts/1', true); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { console.log('Response: ' + xhr.responseText); } }; xhr.send();
当你运行这段代码时,你应该会在控制台中看到类似以下的输出:
Ajax request: GET https://jsonplaceholder.typicode.com/posts/1 Sending Ajax request with body: undefined Response: ...(响应内容)...
相关问答FAQs
Q1: 如何恢复默认的XMLHttpRequest对象?
A1: 你可以通过简单地将原始的XMLHttpRequest对象重新赋值给全局的XMLHttpRequest对象来恢复默认的XMLHttpRequest对象。
window.XMLHttpRequest = originalXHR;
Q2: 如何确保自定义的XMLHttpRequest对象在所有情况下都能正常工作?
A2: 为了确保自定义的XMLHttpRequest对象在所有情况下都能正常工作,你需要确保它正确地实现了所有必要的方法和属性,你还应该在开发过程中进行充分的测试,以确保它在不同的浏览器和环境中都能正常工作。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1427472.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复