服务器多线程Java
在现代网络应用中,服务器需要同时处理多个客户端的请求,为了提高响应速度和处理效率,使用多线程技术是一个常见的解决方案,Java提供了多种方式来实现多线程服务器,本文将详细介绍这些方法及其实现细节。
一、使用JAVA.IO中的ServerSocket和Socket类
1.
在java.io
包中,我们可以使用ServerSocket
类创建服务器端的套接字,使用Socket
类创建客户端的套接字,主线程监听客户端的连接请求,当接收到请求后,主线程会创建一个新的工作线程去处理这个请求,主线程继续监听新的请求。
2. 示例代码
import java.io.*; import java.net.*; public class Server { public static void main(String[] args) throws IOException { ServerSocket serverSocket = new ServerSocket(8000); while (true) { Socket socket = serverSocket.accept(); new Thread(new ClientHandler(socket)).start(); } } } class ClientHandler implements Runnable { private Socket socket; public ClientHandler(Socket socket) { this.socket = socket; } @Override public void run() { try { InputStream input = socket.getInputStream(); OutputStream output = socket.getOutputStream(); BufferedReader reader = new BufferedReader(new InputStreamReader(input)); PrintWriter writer = new PrintWriter(output, true); String line; while ((line = reader.readLine()) != null) { writer.println("Echo: " + line); } socket.close(); } catch (IOException e) { e.printStackTrace(); } } }
3. 解释
ServerSocket serverSocket = new ServerSocket(8000);
:创建一个服务器套接字并绑定到端口8000。
while (true) { ... }
:无限循环,持续监听客户端连接请求。
Socket socket = serverSocket.accept();
:阻塞方法,直到有客户端连接。
new Thread(new ClientHandler(socket)).start();
:为每个客户端连接创建一个新的线程进行处理。
ClientHandler
类实现了Runnable
接口,负责读取客户端消息并响应。
二、使用JAVA.NIO中的ServerSocketChannel和SocketChannel类
1.
在java.nio
包中,我们可以使用ServerSocketChannel
和SocketChannel
类来实现非阻塞式的服务器,这种方式可以用一个线程处理多个连接,而不是每个连接都需要一个线程。
2. 示例代码
import java.io.IOException; import java.net.InetSocketAddress; import java.nio.ByteBuffer; import java.nio.channels.*; import java.util.Iterator; import java.util.Set; public class NioServer { public static void main(String[] args) throws IOException { ServerSocketChannel serverSocketChannel = ServerSocketChannel.open(); serverSocketChannel.bind(new InetSocketAddress(8000)); serverSocketChannel.configureBlocking(false); Selector selector = Selector.open(); serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT); while (true) { int readyChannels = selector.select(); if (readyChannels == 0) continue; Set<SelectionKey> selectedKeys = selector.selectedKeys(); Iterator<SelectionKey> keyIterator = selectedKeys.iterator(); while (keyIterator.hasNext()) { SelectionKey key = keyIterator.next(); if (key.isAcceptable()) { ServerSocketChannel serverChannel = (ServerSocketChannel) key.channel(); SocketChannel socketChannel = serverChannel.accept(); socketChannel.configureBlocking(false); socketChannel.register(selector, SelectionKey.OP_READ); } else if (key.isReadable()) { SocketChannel socketChannel = (SocketChannel) key.channel(); ByteBuffer buffer = ByteBuffer.allocate(256); int read = socketChannel.read(buffer); if (read > 0) { buffer.flip(); socketChannel.write(buffer); } else if (read < 0) { socketChannel.close(); } } keyIterator.remove(); } } } }
3. 解释
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
:打开一个服务器套接字通道。
serverSocketChannel.bind(new InetSocketAddress(8000));
:绑定到端口8000。
serverSocketChannel.configureBlocking(false);
:设置为非阻塞模式。
Selector selector = Selector.open();
:打开一个选择器。
serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT);
:注册选择器以接受连接。
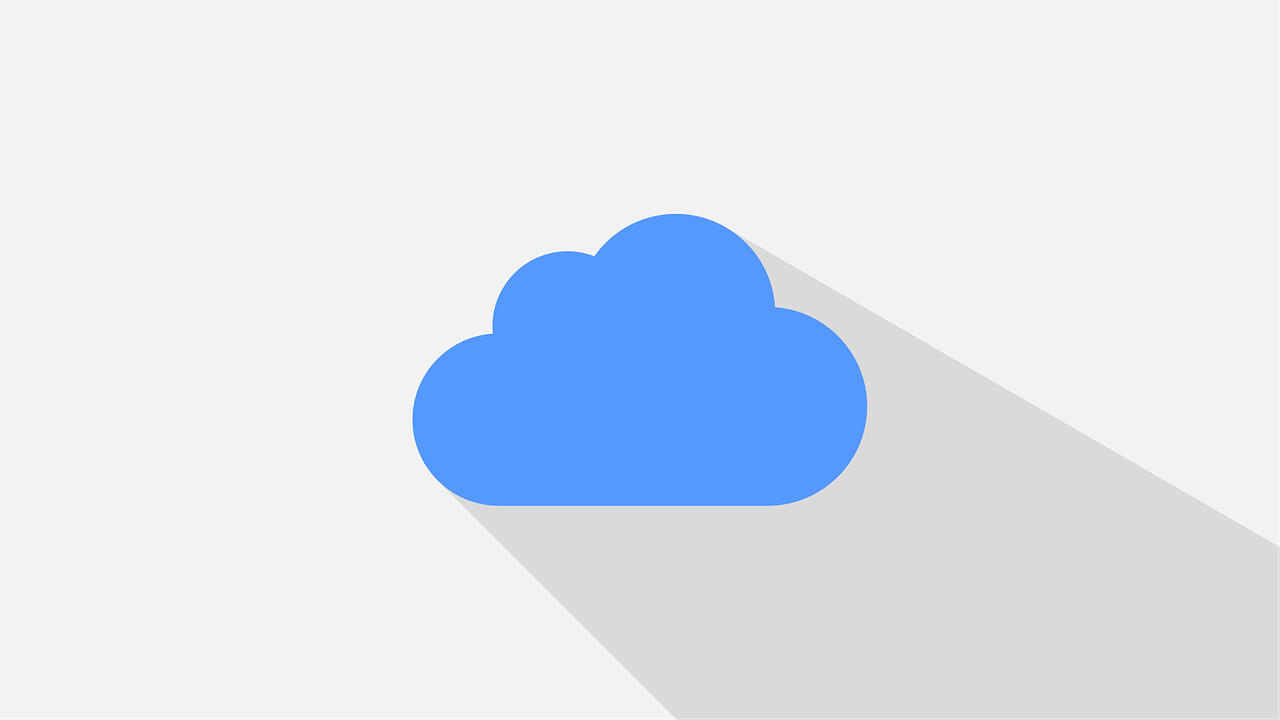
selector.select();
:阻塞方法,直到有准备好的通道。
keyIterator.hasNext()
:迭代准备好的键集合。
根据不同的事件类型(接受连接或读取数据)进行处理。
三、使用JAVA.NET中的AsynchronousServerSocketChannel和AsynchronousSocketChannel类
1.
在java.net
包中,我们可以使用AsynchronousServerSocketChannel
和AsynchronousSocketChannel
类来实现异步的服务器,这种方式的优点是可以异步地处理多个连接,而不是阻塞地等待每个连接。
2. 示例代码
import java.io.IOException; import java.net.InetSocketAddress; import java.nio.ByteBuffer; import java.nio.channels.AsynchronousServerSocketChannel; import java.nio.channels.AsynchronousSocketChannel; import java.nio.channels.CompletionHandler; import java.util.concurrent.Future; public class AsyncServer { public static void main(String[] args) throws IOException { AsynchronousServerSocketChannel serverChannel = AsynchronousServerSocketChannel.open().bind(new InetSocketAddress(8000)); while (true) { Future<AsynchronousSocketChannel> future = serverChannel.accept(); future.get(); // This will block until a connection is made AsynchronousSocketChannel clientChannel = future.getResult(); new Thread(new ClientHandler(clientChannel)).start(); } } } class ClientHandler implements Runnable { private AsynchronousSocketChannel clientChannel; public ClientHandler(AsynchronousSocketChannel clientChannel) { this.clientChannel = clientChannel; } @Override public void run() { try { ByteBuffer buffer = ByteBuffer.allocate(256); while (true) { Future<Integer> readResult = clientChannel.read(buffer); readResult.get(); // Block until data is read or the connection is closed buffer.flip(); clientChannel.write(buffer).get(); // Echo back to client buffer.clear(); } } catch (Exception e) { e.printStackTrace(); } finally { try { clientChannel.close(); } catch (IOException e) { e.printStackTrace(); } } } }
3. 解释
AsynchronousServerSocketChannel serverChannel = AsynchronousServerSocketChannel.open().bind(new InetSocketAddress(8000));
:打开并绑定异步服务器套接字通道。
future.get();
:阻塞方法,直到有客户端连接。
new Thread(new ClientHandler(clientChannel)).start();
:为每个客户端连接创建一个新的线程进行处理。
ClientHandler
类实现了Runnable
接口,负责读取客户端消息并响应。
四、性能对比及适用场景分析
方法 | 优点 | 缺点 | 适用场景 |
JAVA.IO | 实现简单,易于理解。 | 每个连接需要一个线程,资源消耗较大。 | 适用于连接数较少且处理时间较短的场景。 |
JAVA.NIO | 单个线程可以处理多个连接,资源利用率高。 | 编程复杂度较高,需要处理非阻塞I/O操作。 | 适用于高并发、低延迟的场景。 |
JAVA.NET | 异步处理,进一步提高资源利用率。 | 编程复杂度最高,需要处理异步回调逻辑。 | 适用于极高并发、需要高性能的场景。 |
通过上述表格可以看出,不同的方法各有优缺点,选择哪种方法取决于具体的应用场景和需求,对于简单的聊天室应用,可以使用JAVA.IO
;对于高并发的Web服务器,可以考虑使用JAVA.NIO
或JAVA.NET
。
五、FAQs(常见问题解答)
Q1: Java多线程服务器如何优化性能?
A1: 优化Java多线程服务器性能可以从以下几个方面入手:使用线程池来管理和复用线程,减少线程创建和销毁的开销;选择合适的I/O模型(如NIO),提高资源利用率;合理配置服务器参数(如TCP缓冲区大小),避免不必要的系统调用;使用高效的数据结构和算法,减少CPU和内存的使用,还可以通过监控和调优工具(如JProfiler、VisualVM等)来发现性能瓶颈并进行针对性优化。
Q2: Java多线程服务器如何处理大量并发连接?
A2: 处理大量并发连接的关键在于使用高效的并发模型和资源管理策略,可以使用线程池来限制同时运行的线程数量,防止系统过载,采用非阻塞I/O(如NIO)或异步I/O(如AIO)来提高资源利用率,允许一个线程处理多个连接,还可以通过负载均衡、集群部署等方式来分散单点压力,确保代码是线程安全的,避免并发问题导致的异常情况。
到此,以上就是小编对于“服务器多线程java”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1425180.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复