Chrome插件JS开发指南
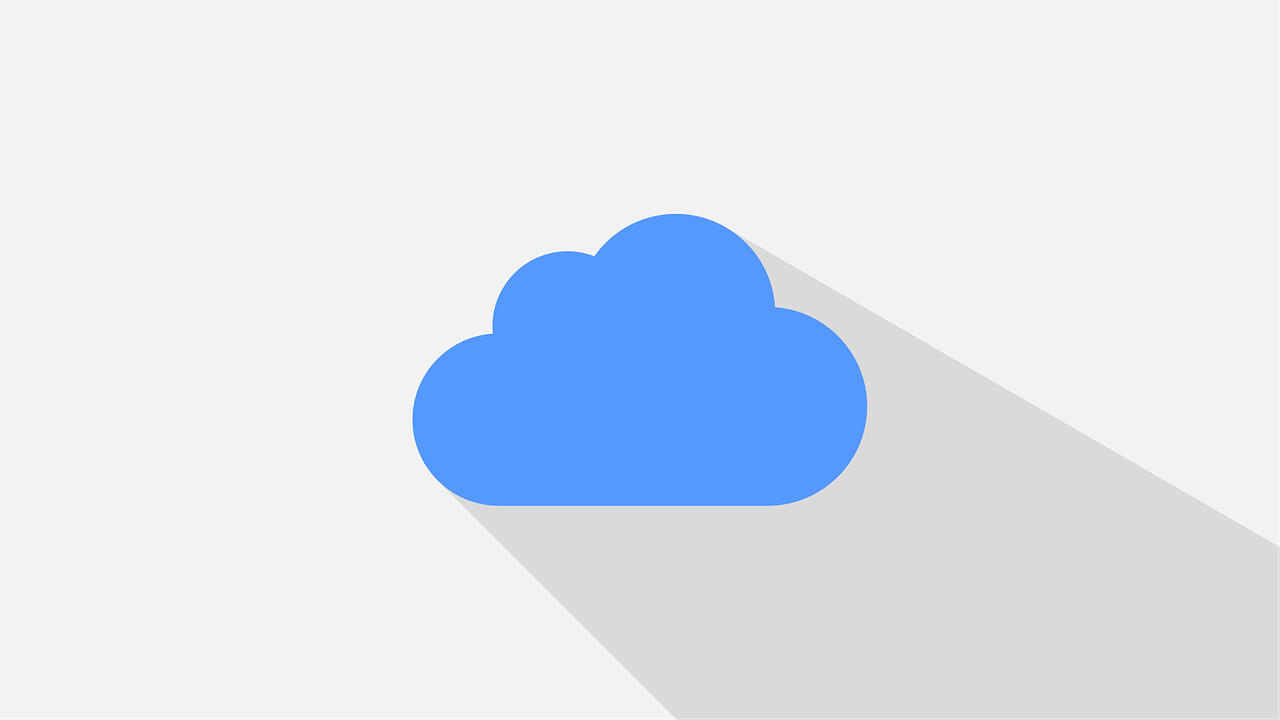
Chrome浏览器插件,也称为扩展程序,是增强浏览器功能的小程序,通过使用HTML、CSS和JavaScript等前端技术,开发者可以创建功能强大的插件,以提升用户的浏览体验,本文将详细介绍如何开发一个简单的Chrome插件,从安装到发布,涵盖各个关键步骤。
一、安装插件
1. Chrome Web Store安装
在Chrome Web Store中,你可以搜索并安装各种插件,只需点击“添加到Chrome”按钮即可完成安装。
2. 开发者模式安装
要手动安装自己的插件,需要打开Chrome浏览器,进入设置,选择扩展程序,然后启用开发者模式,点击“加载已解压的扩展程序”,选择你开发的插件文件夹即可。
二、创建Manifest文件
Manifest文件是Chrome插件的配置文件,通常命名为manifest.json
,这个文件定义了插件的基本信息和权限,以下是一个示例:
{ "manifest_version": 2, "name": "My Chrome Extension", "version": "1.0", "description": "A simple Chrome extension.", "permissions": ["activeTab"], "background": { "scripts": ["background.js"], "persistent": false }, "content_scripts": [ { "matches": ["<all_urls>"], "js": ["content.js"] } ], "browser_action": { "default_popup": "popup.html", "default_icon": { "16": "images/icon16.png", "48": "images/icon48.png", "128": "images/icon128.png" } } }
脚本是插件中用于操作网页DOM的脚本,它们在网页的上下文中运行,可以直接访问和修改网页内容。
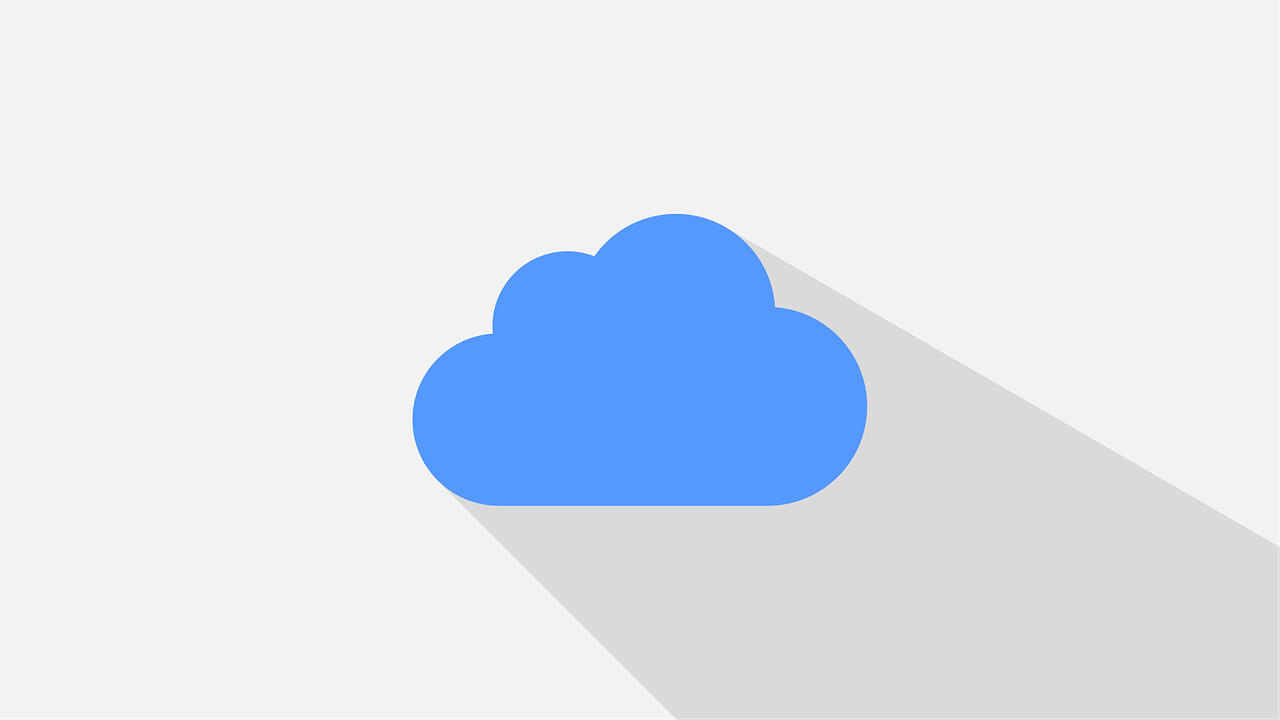
// content.js document.body.style.backgroundColor = "yellow";
在manifest.json
脚本:
"content_scripts": [ { "matches": ["<all_urls>"], "js": ["content.js"] } ]
四、使用背景脚本
背景脚本是插件的核心逻辑部分,通常用于处理事件、管理状态等,它们在后台运行,并且可以与浏览器的API进行交互。
// background.js chrome.browserAction.onClicked.addListener(function(tab) { chrome.tabs.executeScript({ file: 'content.js' }); });
在manifest.json
中注册背景脚本:
"background": { "scripts": ["background.js"], "persistent": false }
五、调试与发布
1. 使用开发者工具调试
你可以右键点击插件图标,选择“检查弹出窗口”或“检查背景页”,打开开发者工具进行调试。
2. 发布插件
当插件开发完成后,可以通过Chrome Web Store发布,你需要创建一个开发者账号,上传插件并填写相关信息,然后提交审核。
六、进阶使用
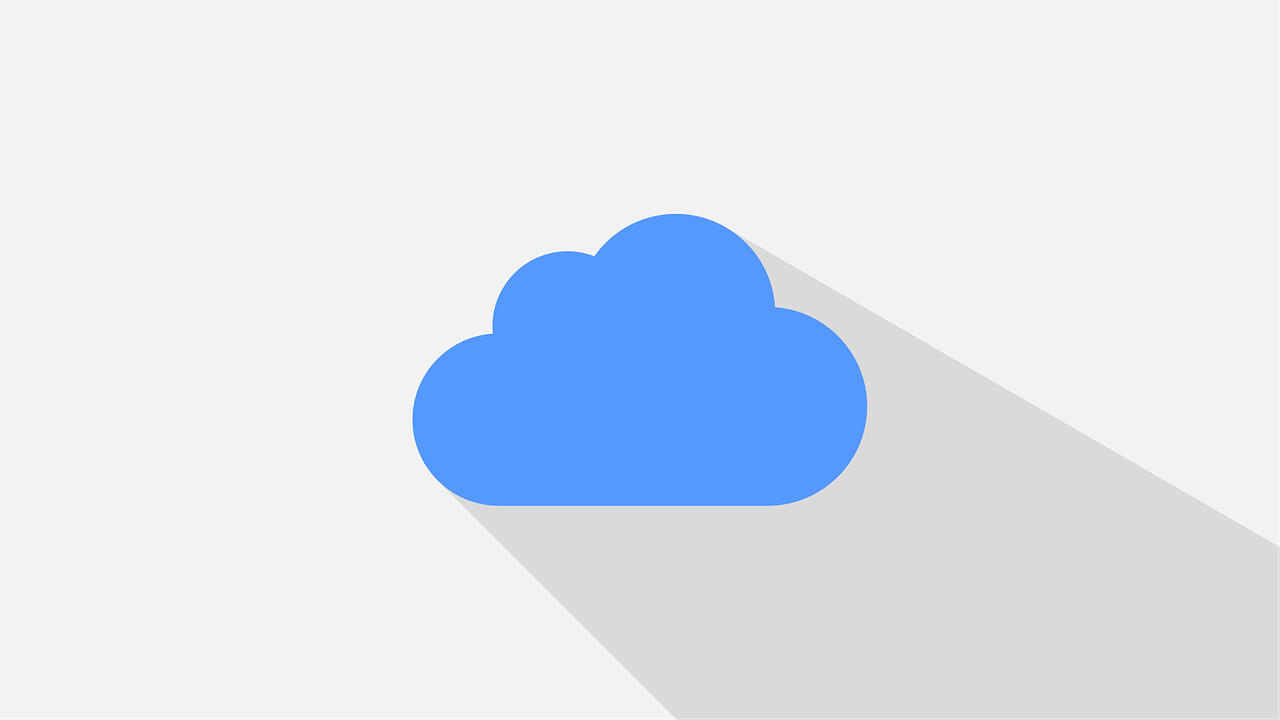
1. 使用存储API
Chrome插件提供了存储API,可以方便地存储和读取数据。
// 保存数据 chrome.storage.sync.set({key: 'value'}, function() { console.log('Data saved'); }); // 读取数据 chrome.storage.sync.get(['key'], function(result) { console.log('Data retrieved: ' + result.key); });
2. 使用消息传递
背景脚本和内容脚本之间可以通过消息传递进行通信。
// content.js chrome.runtime.sendMessage({greeting: "hello"}, function(response) { console.log(response.farewell); }); // background.js chrome.runtime.onMessage.addListener(function(request, sender, sendResponse) { if (request.greeting == "hello") sendResponse({farewell: "goodbye"}); });
七、实例项目
为了更好地理解如何使用JavaScript开发Chrome浏览器插件,我们可以创建一个简单的实例项目,项目需求如下:当用户点击插件图标时,改变当前网页的背景颜色,并在插件弹出窗口中显示一个输入框,允许用户输入自定义颜色。
1. 项目结构
my-chrome-extension/ ├── manifest.json ├── background.js ├── content.js ├── popup.html ├── popup.js ├── style.css └── images/ ├── icon16.png ├── icon48.png └── icon128.png
2. manifest.json配置
{ "manifest_version": 2, "name": "Custom Background Color", "version": "1.0", "description": "Change the background color of the current page.", "permissions": ["activeTab"], "background": { "scripts": ["background.js"], "persistent": false }, "content_scripts": [ { "matches": ["<all_urls>"], "js": ["content.js"] } ], "browser_action": { "default_popup": "popup.html", "default_icon": { "16": "images/icon16.png", "48": "images/icon48.png", "128": "images/icon128.png" } } }
3. background.js代码
chrome.browserAction.onClicked.addListener(function(tab) { chrome.tabs.executeScript({ file: 'content.js' }); });
4. content.js代码
document.body.style.backgroundColor = localStorage.getItem('bgColor') || 'yellow';
5. popup.html代码
<!DOCTYPE html> <html> <head> <title>Custom Background Color</title> <link rel="stylesheet" href="style.css"> </head> <body> <h1>Change Background Color</h1> <input type="color" id="bgColorPicker" value="#FFFF00"> <button id="saveBtn">Save</button> <script src="popup.js"></script> </body> </html>
6. popup.js代码
document.getElementById('saveBtn').addEventListener('click', function() { var color = document.getElementById('bgColorPicker').value; localStorage.setItem('bgColor', color); alert('Background color changed to ' + color); });
7. style.css代码
body { width: 200px; text-align: center; } h1 { font-size: 16px; color: #333; } input[type="color"] { width: 100%; margin-bottom: 10px; } button { padding: 10px 15px; font-size: 14px; }
通过以上步骤,我们创建了一个简单的Chrome插件,它能够在用户点击插件图标时改变当前网页的背景颜色,并通过弹出窗口允许用户输入自定义颜色,这个实例项目涵盖了Chrome插件开发的基本流程和关键技术点,希望对你有所帮助。
以上就是关于“chrome插件js”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1424573.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复