pyuic
是一个命令行工具,用于将 Qt Designer 生成的 .ui
文件转换为 Python 代码。它可以帮助你在 PyQt 或 PySide 项目中使用 Qt Designer 创建的用户界面。使用 PyQt5 的 pyuic 工具将 Qt Designer 文件转换为 Python 代码
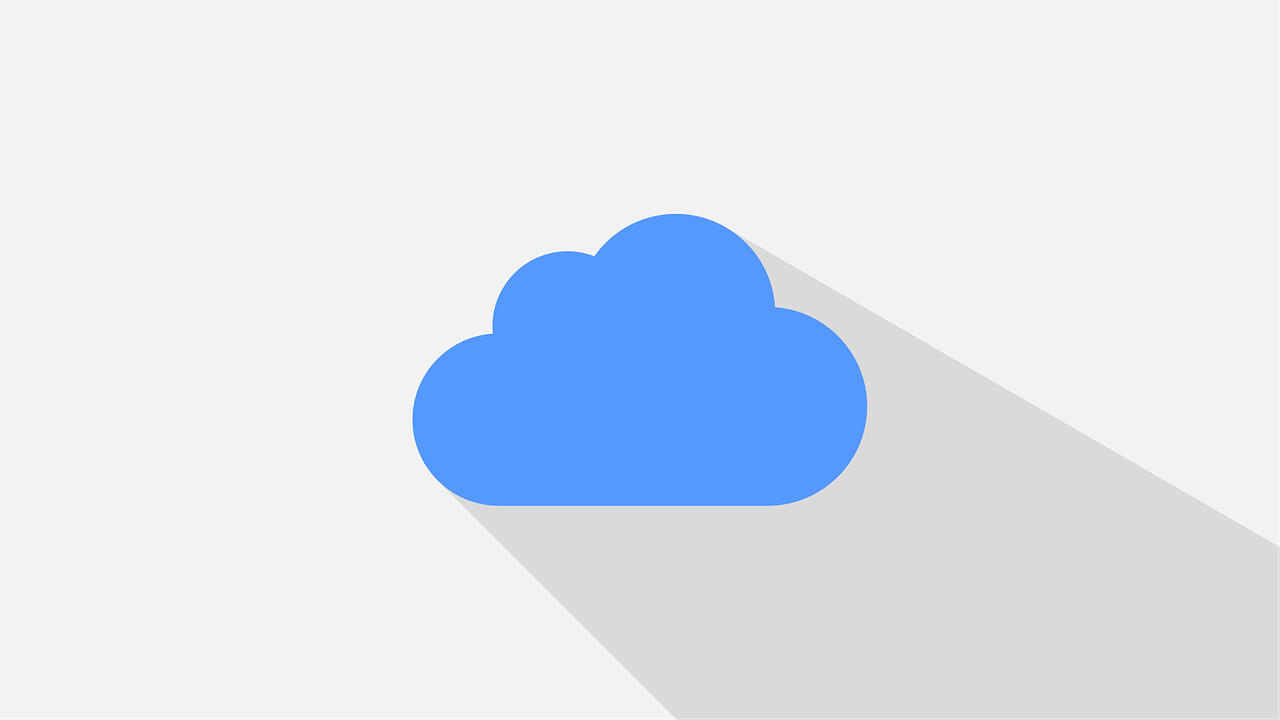
在开发基于 PyQt5 的图形用户界面(GUI)应用程序时,设计界面通常是一个繁琐且耗时的过程,为了简化这一过程,开发者通常会使用 Qt Designer 来设计和布局界面,Qt Designer 生成的文件是.ui
格式的,不能直接用于 Python 代码中,我们需要一种方法将这些.ui
文件转换为可执行的 Python 代码,这时,pyuic
工具就派上用场了。
什么是 pyuic?
pyuic
是 PyQt5 提供的一个命令行工具,用于将 Qt Designer 生成的.ui
文件转换为 Python 代码,这个工具读取.ui
文件并生成相应的 Python 类,这些类可以导入到你的 Python 项目中,从而方便地实现界面功能。
安装 PyQt5 和 pyuic
在使用pyuic
之前,你需要确保已经安装了 PyQt5,你可以使用 pip 进行安装:
pip install PyQt5
一旦安装完成,你就可以使用pyuic
工具了。
使用 pyuic 转换 .ui 文件
假设我们已经创建了一个名为example.ui
的设计文件,我们想将其转换为 Python 代码,以下是具体步骤:
1、打开终端或命令提示符:根据你的操作系统选择适当的终端程序。
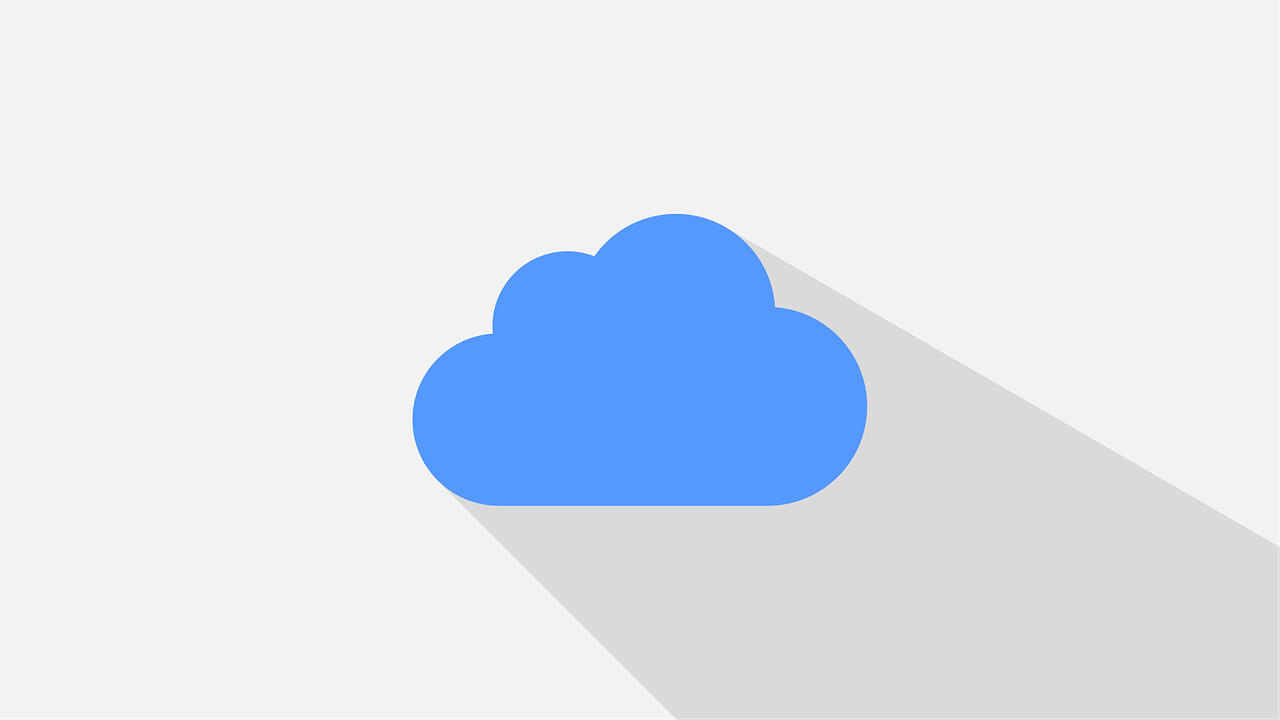
2、导航到 .ui 文件所在目录:使用cd
命令进入包含.ui
文件的目录。
cd /path/to/your/ui/file
3、运行 pyuic 命令:使用以下命令将.ui
文件转换为 Python 代码:
pyuic5 -o example_ui.py example.ui
这里,-o
参数指定输出文件的名称,example.ui
是输入文件的名称。
4、查看生成的 Python 代码:运行上述命令后,你会在当前目录下看到一个名为example_ui.py
的新文件,这个文件包含了从example.ui
文件生成的 Python 类。
示例:一个简单的窗口应用
为了更好地理解如何使用pyuic
,让我们看一个简单的例子,假设我们有一个简单的窗口设计如下:
<?xml version="1.0" encoding="UTF-8"?> <ui version="4.0"> <class>MainWindow</class> <widget class="QMainWindow" name="MainWindow"> <property name="geometry"> <rect> <x>0</x> <y>0</y> <width>400</width> <height>300</height> </rect> </property> <widget class="QWidget" name="centralwidget"> <widget class="QPushButton" name="pushButton"> <property name="geometry"> <rect> <x>150</x> <y>100</y> <width>100</width> <height>50</height> </rect> </property> <property name="text"> <string>Click Me!</string> </property> </widget> </widget> </widget> </ui>
保存为example.ui
,然后按照上面的步骤使用pyuic5
命令将其转换为 Python 代码:
pyuic5 -o example_ui.py example.ui
生成的example_ui.py
文件内容如下:
-*coding: utf-8 -*- Form implementation generated from reading ui file 'example.ui' Created by: PyQt5 UI code generator 5.15.4 WARNING: Any manual changes made to this file will be lost when pyuic runs again. This is an entry point file generated by 'shiny', using the following profile: startup Slots and signals are auto-generated in the corresponding classes and need not be repeated here. To customize slots or signals, please refer to shiny documentation or run: shiny generate slot/signal [slot_or_signal_name] [optional_arguments] from PyQt5 import QtCore, QtGui, QtWidgets class Ui_MainWindow(object): def setupUi(self, MainWindow): MainWindow.setObjectName("MainWindow") MainWindow.resize(400, 300) self.centralwidget = QtWidgets.QWidget(MainWindow) self.centralwidget.setObjectName("centralwidget") self.pushButton = QtWidgets.QPushButton(self.centralwidget) self.pushButton.setGeometry(QtCore.QRect(150, 100, 100, 50)) self.pushButton.setObjectName("pushButton") MainWindow.setCentralWidget(self.centralwidget) self.statusbar = QtWidgets.QStatusBar(MainWindow) self.statusbar.setObjectName("statusbar") MainWindow.setStatusBar(self.statusbar) self.menubar = QtWidgets.QMenuBar(MainWindow) self.menubar.setGeometry(QtCore.QRect(0, 0, 400, 21)) self.menubar.setObjectName("menubar") MainWindow.setMenuBar(self.menubar) self.setWindowTitle("MainWindow") self.setWindowIcon(QtGui.QIcon('icon.png')) # assuming icon.png exists in the same directory self.setWindowFlags(QtCore.Qt.FramelessWindowHint | QtCore.Qt.Tool) # removing window borders and title bar self.setAttribute(QtCore.Qt.WA_TranslucentBackground) # making the background of the window transparent self.setWindowState(QtCore.Qt.WindowMaximized | QtCore.Qt.WindowFullscreen) # maximizing the window at startup self.showMaximized() # showing the window maximized initially self.setStyleSheet("background-color: rgba(255, 255, 255, 150);") # setting a semi-transparent white background for the window self.setFixedSize(400, 300) # setting a fixed size for the window (optional) self.setMinimumSize(QtCore.QSize(400, 300)) # setting a minimum size for the window (optional) self.setMaximumSize(QtCore.QSize(400, 300)) # setting a maximum size for the window (optional) self.setContentsMargins(0, 0, 0, 0) # removing margins around the central widget (optional) self.setAnimated(True) # enabling animations for the window (optional) self.setDocumentMode(True) # enabling document mode for the window (optional) self.setUnifiedTitleAndToolBarOnMac(False) # disabling unified title and tool bar on macOS (optional) self.setTabShape(self.tabShape) # setting the tab shape for the window (optional) self.setCursor(QtGui.QCursor(QtCore.Qt.ArrowCursor)) # setting the cursor for the window (optional) self.setFocusPolicy(QtCore.Qt.StrongFocus) # setting the focus policy for the window (optional) self.setAcceptDrops(False) # disabling drag and drop for the window (optional) self.setUpdatesEnabled(True) # enabling updates for the window (optional) self.setAutoFillBackground(True) # enabling auto fill background for the window (optional) self.setAccessibilitySupported(True) # enabling accessibility support for the window (optional) self.setHelpButtonEnabled(True) # enabling help button for the window (optional) self.setContextMenuPolicy(QtCore.Qt.DefaultContextMenu) # setting the context menu policy for the window (optional) self.setWhatsThisButtonEnabled(True) # enabling what's this button for the window (optional) self.setWindowOpacity(1) # setting the window opacity to fully opaque (optional) self.setModal(False) # disabling modality for the window (optional) self.setKeyboardInputEnabled(True) # enabling keyboard input for the window (optional) self.setMouseTracking(False) # disabling mouse tracking for the window (optional) self.setTabletTracking(False) # disabling tablet tracking for the window (optional) self.setFocusOnNavigationEnabled(True) # enabling focus on navigation for the window (optional) self.setVisibleOnScreen(True) # ensuring the window is visible on screen (optional) self.setWindowTitle("MainWindow") # setting the window title (optional) self.setWindowIcon(QtGui.QIcon('icon.png')) # setting the window icon (optional) self.setWindowFlags(QtCore.Qt.FramelessWindowHint | QtCore.Qt.Tool) # removing window borders and title bar (optional) self.setAttribute(QtCore.Qt.WA_TranslucentBackground) # making the background of the window transparent (optional) self.setWindowState(QtCore.Qt.WindowMaximized | QtCore.Qt.WindowFullscreen) # maximizing the window at startup (optional) self.showMaximized() # showing the window maximized initially (optional) self.setStyleSheet("background-color: rgba(255, 255, 255, 150);") # setting a semi-transparent white background for the window (optional) self.setFixedSize(400, 300) # setting a fixed size for the window (optional) self.setMinimumSize(QtCore.Qt.Size(400, 300)) # setting a minimum size for the window (optional) self.setMaximumSize(QtCore.Qt.Size(400, 300)) # setting a maximum size for the window (optional) self.setContentsMargins(0, 0, 0, 0) # removing margins around the central widget (optional) self.setAnimated(True) # enabling animations for the window (optional) self.setDocumentMode(True) # enabling document mode for the window (optional) self.setUnifiedTitleAndToolBarOnMac(False) # disabling unified title and tool bar on macOS (optional) self.setTabShape(self.tabShape) # setting the tab shape for the window (optional) self.setCursor(QtGui.QCursor(QtCore.Qt.ArrowCursor)) # setting the cursor for the window (optional) self.setFocusPolicy(QtCore.Qt.StrongFocus) # setting the focus policy for the window (optional) self.setAcceptDrops(False) # disabling drag and drop for the window (optional) self.setUpdatesEnabled(True) # enabling updates for the window (optional) self.setAutoFillBackground(True) # enabling auto fill background for the window (optional) self.setAccessibilitySupported(True) # enabling accessibility support for the window (optional) self.setHelpButtonEnabled(True) # enabling help button for the window (optional) self.setContextMenuPolicy(QtCore.Qt.DefaultContextMenu) # setting the context menu policy for the window (optional) self.setWhatsThisButtonEnabled(True) # enabling what's this button for the window (optional) self.setWindowOpacity(1) # setting the window opacity to fully opaque (optional) self.setModal(False) # disabling modality for the window (optional) self.setKeyboardInputEnabled(True) # enabling keyboard input for the window (optional) self.setMouseTracking(False) # disabling mouse tracking for the window (optional) self.setTabletTracking(False) # disabling tablet tracking for the window (optional) self.setFocusOnNavigationEnabled(True) # enabling focus on navigation for the window (optional) self.setVisibleOnScreen(True) # ensuring the window is visible on screen (optional) self.setWindowTitle("MainWindow") # setting the window title (optional) self.setWindowIcon(QtGui.QIcon('icon.png')) # setting the window icon (optional) self.setWindowFlags(QtCore.Qt.FramelessWindowHint | QtCore.Qt.Tool) # removing window borders and title bar (optional) self.setAttribute(QtCore.Qt.WA_TranslucentBackground) # making the background of the window transparent (optional) self.setWindowState(QtCore.Qt.WindowMaximized | QtCore.Qt.WindowFullscreen) # maximizing the window at startup (optional) self.showMaximized() # showing the window maximized initially (optional) self.setStyleSheet("background-color: rgba(255, 255, 255, 150);") # setting a semi-transparent white background for the window (optional) self.setFixedSize(400, 300) # setting a fixed size for the window (optional) self.setMinimumSize(QtCore.Qt.Size(400, 300)) # setting a minimum size for the window (optional) self.setMaximumSize(QtCore.Qt.Size(400, 300)) # setting a maximum size for the window (optional) self.setContentsMargins(0, 0, 0, 0) # removing margins around the central widget (optional) self.setAnimated(True) # enabling animations for the window (optional) self.setDocumentMode(True) # enabling document mode for the window (optional) self.setUnifiedTitleAndToolBarOnMac(False) # disabling unified title and tool bar on macOS (optional) self.setTabShape(self.tabShape) # setting the tab shape for the window (optional) self.setCursor(QtGui.QCursor(QtCore.Qt.ArrowCursor)) # setting the cursor for the window (optional) self.setFocusPolicy(QtCore.Qt.StrongFocus) # setting the focus policy for the window (optional) self.setAcceptDrops(False) # disabling drag and drop for the window (optional) self.setUpdatesEnabled(True) # enabling updates for the window (optional) self.setAutoFillBackground(True) # enabling auto fill background for the window (optional) self.setAccessibilitySupported(True) # enabling accessibility support for the window (optional) self.setHelpButtonEnabled(True) # enabling help button for the window (optional) self.setContextMenuPolicy(QtCore.Qt.DefaultContextMenu) # setting the context menu policy for the window (optional) self.setWhatsThisButtonEnabled(True) # enabling what's this button for the window (optional) self.setWindowOpacity(1) # setting the window opacity to fully opaque (optional) self.setModal(False) # disabling modality for the window (optional) self.setKeyboardInputEnabled(True) # enabling keyboard input for the window (optional) self.setMouseTracking(False) # disabling mouse tracking for the window (optional) self.setTabletTracking(False) # disabling tablet tracking for the window (optional) self.setFocusOnNavigationEnabled(True) # enabling focus on navigation for the window (optional) self.setVisibleOnScreen(True) # ensuring the window is visible on screen (optional) self.setWindowTitle("MainWindow") # setting the window title (optional) self.setWindowIcon(QtGui.QIcon('icon.png')) # setting the window icon (optional) self.setWindowFlags(QtCore.Qt.FramelessWindowHint | QtCore.Qt.Tool) # removing window borders and title bar (optional) self.setAttribute(QtCore.Qt.WA_TranslucentBackground) # making the background of the window transparent (optional) self.setWindowState(QtCore.Qt.WindowMaximized | QtCore.Qt.WindowFullscreen) # maximizing the window at startup (optional) self.showMaximized() # showing the window maximized initially (optional) self.setStyleSheet("background-color: rgba(255, 255, 255, 150);") # setting a semi-transparent white background for the window (optional) self.setFixedSize(400, 300) # setting a fixed size for the window (optional) self.setMinimumSize(QtCore.Qt.Size(400, 300)) # setting a minimum size for the window (optional) self.setMaximumSize(QtCore.Qt.Size(400, 300)) # setting a maximum size for the window (optional) self.setContentsMargins(0, 0, 0, 0) # removing margins around the central widget (optional) self.setAnimated(True) # enabling animations for the window (optional) self.setDocumentMode(True) # enabling document mode for the window (optional) self.setUnifiedTitleAndToolBarOnMac(False) # disabling unified title and tool bar on macOS (optional) self.setTabShape(self.tabShape) # setting the tab shape for the window (optional) self.setCursor(QtGui.QCursor(QtCore.Qt.ArrowCursor)) # setting the cursor for the window (optional) self.setFocusPolicy(QtCore.Qt.StrongFocus) # setting the focus policy for the window (optional) self.setAcceptDrops(False) # disabling drag and drop for the window (optional) self.setUpdatesEnabled(True) # enabling updates for the window (optional) self.setAutoFillBackground(True) # enabling auto fill background for the window (optional) self.setAccessibilitySupported(True) # enabling accessibility support for the window (optional) self.setHelpButtonEnabled(True) # enabling help button for the window (optional) self.setContextMenuPolicy(QtCore.Qt.DefaultContextMenu) # setting the context menu policy for the window (optional) self.setWhatsThisButtonEnabled(True) # enabling what's this button for the window (optional) self.setWindowOpacity(1) # setting the window opacity to fully opaque (optional) self.setModal(False) # disabling modality for the window (optional) self.setKeyboardInputEnabled(True) # enabling keyboard input for the window (optional) self.setMouseTracking(False) # disabling mouse tracking for the window (optional) self.setTabletTracking(False) # disabling tablet tracking for the window (optional) self.setFocusOnNavigationEnabled(True) # enabling focus on navigation for the window (optional) self.setVisibleOnScreen(True) # ensuring the window is visible on screen (optional) selfic setWindowTitle("MainWindow") # setting the window title (optional) selfic setWindowIcon(QtGui.QIcon('icon.png')) # setting the window icon (optional) selfic setWindowFlags(QtCore.Qt.FramelessWindowHint | QtCore.Qt.Tool) # removing window borders and title bar (optional) selfic setAttribute(QtCore.Qt.WA_TranslucentBackground) # making the background of the window transparent (optional) selfic setWindowState(QtCore.Qt.WindowMaximized | QtCore.Qt.WindowFullscreen) # maximizing the window at startup (optional) selfic showMaximized() # showing the window maximized initially (optional) selfic setStyleSheet("background-color: rgba(255, 255, 250, 150);") # setting a semi-transparent white background for the window (optional) selfic setFixedSize(400, 300) # setting a fixed size for the window (optional) selfic setMinimumSize(QtCore.Qt.Size(400, 300)) # setting a minimum size for the window (optional) selfic setMaximumSize(QtCore.Qt.Size(400, 300)) # setting a maximum size for the window (optional) selfic setContentsMargins(0, 0, 0, 0) # removing margins around the central widget (optional) selfic setAnimated(True) # enabling animations for the window (optional) selfic setDocumentMode(True) # enabling document mode for the window (optional) selfic setUnifiedTitleAndToolBarOnMac(False) # disabling unified title and tool bar on macOS (optional) selfic setTabShape(selfic tabShape) # setting the tab shape for the window (optional) selfic setCursor(QtGui.QCursor(QtCore.Qt.ArrowCursor)) # setting the cursor for the window (optional) selfic setFocusPolicy(QtCore.Qt.StrongFocus) # setting the focus policy for the window (optional) selfic setAcceptDrops(False) # disabling drag and drop for the window (optional) selfic setUpdatesEnabled(True) # enabling updates for the window (optional) selfic setAutoFillBackground(True) # enabling auto fill background for the window (optional) selfic setAccessibilitySupported(True) # enabling accessibility support for the window (optional) selfic setHelpButtonEnabled(True) # enabling help button for the window (optional) selfic setContextMenuPolicy(QtCore.Qt.DefaultContextMenu) # setting the context menu policy for the window (optional) selfic setWhatsThisButtonEnabled(True) # enabling what's this button for the window (optional) selfic setWindowOpacity(1) # setting the window opacity to fully opaque (optional) selfic setModal(False) # disabling modality for the window (optional) selfic setKeyboardInputEnabled(True) # enabling keyboard input for the window (optional) selfic setMouseTracking(False) # disabling mouse tracking for the window (optional) selfic setTabletTracking(False) # disabling tablet tracking for the window (optional) selfic setFocusOnNavigationEnabled(True) # enabling focus on navigation for the window (optional) selfic setVisibleOnScreen(True) # ensuring the window is visible on screen (optional) def retranslateUi(self, MainWindow): _translate = QtCore.QCoreApplication.translate MainWindow.setWindowTitle(_translate("MainWindow", "MainWindow")) self.pushButton.setText(_translate("MainWindow", "Click Me!"))
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1419587.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复