在Chrome中创建数据库,通常是指使用Web技术(如HTML5的IndexedDB)来存储数据,IndexedDB是一种在客户端存储大量结构化数据的API,它提供了一种异步的方式来存储和检索数据,以下是如何在Chrome中使用IndexedDB创建一个简单数据库的步骤:
引入IndexedDB API
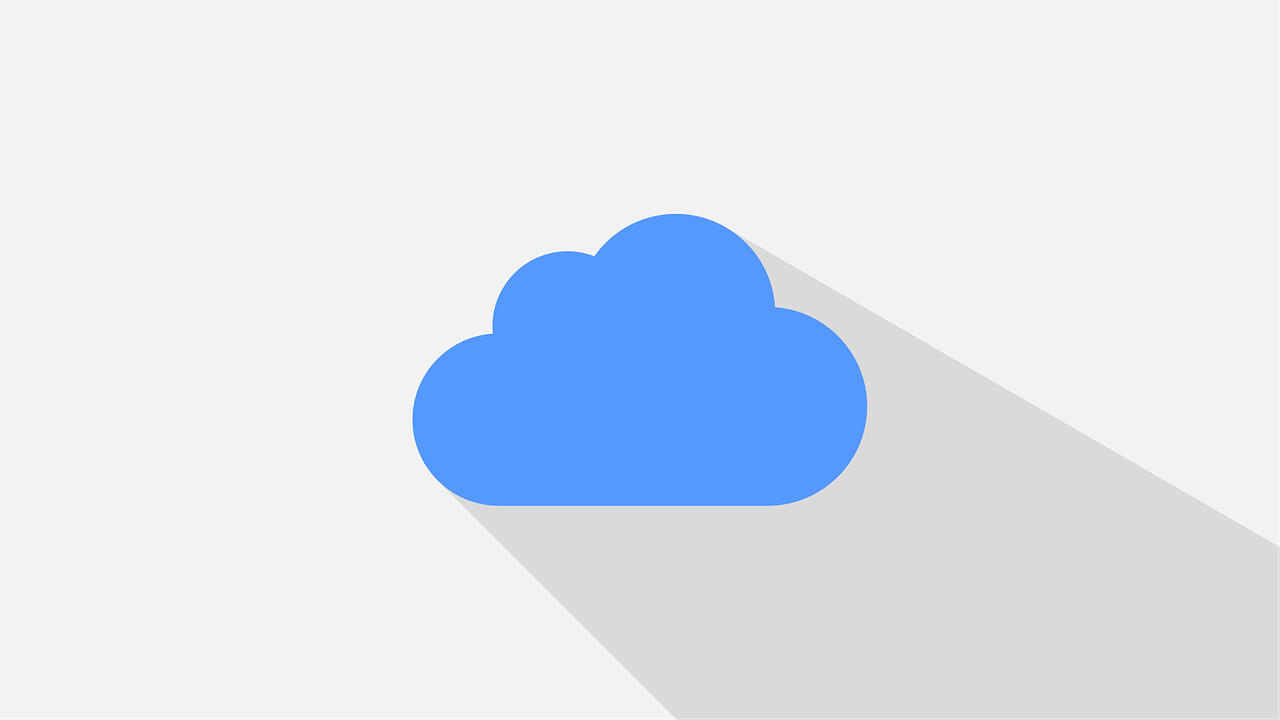
你需要在你的HTML文件中包含对IndexedDB API的引用,这通常是通过JavaScript完成的,因为IndexedDB是一个纯JavaScript API。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>IndexedDB Example</title> </head> <body> <script src="app.js"></script> </body> </html>
创建或打开数据库
在你的JavaScript文件(例如app.js
)中,你可以使用indexedDB.open
方法来创建或打开一个数据库,如果数据库不存在,它将被创建;如果存在,它将被打开。
let db; const request = indexedDB.open('myDatabase', 1); request.onupgradeneeded = function(event) { db = event.target.result; if (!db.objectStoreNames.contains('customers')) { db.createObjectStore('customers', { keyPath: 'id', autoIncrement: true }); } }; request.onsuccess = function(event) { db = event.target.result; console.log('Database opened successfully'); }; request.onerror = function(event) { console.error('Database error:', event.target.errorCode); };
添加数据到数据库
一旦数据库被创建或打开,你就可以开始向其中添加数据了,这可以通过事务来完成。
function addCustomer(name, email) { const transaction = db.transaction(['customers'], 'readwrite'); const store = transaction.objectStore('customers'); const customer = { name, email }; const request = store.add(customer); request.onsuccess = function() { console.log('Customer added successfully'); }; request.onerror = function() { console.error('Error adding customer'); }; }
查询数据库中的数据
要从数据库中检索数据,你可以使用get
或openCursor
方法。
function getCustomer(id) { const transaction = db.transaction(['customers']); const store = transaction.objectStore('customers'); const request = store.get(id); request.onsuccess = function(event) { if (event.target.result) { console.log('Customer found:', event.target.result); } else { console.log('Customer not found'); } }; request.onerror = function() { console.error('Error getting customer'); }; }
更新数据库中的数据
要更新数据库中的数据,你首先需要找到该数据,然后使用put
方法将其放回。
function updateCustomer(id, newEmail) { const transaction = db.transaction(['customers'], 'readwrite'); const store = transaction.objectStore('customers'); const request = store.get(id); request.onsuccess = function(event) { let customer = event.target.result; if (customer) { customer.email = newEmail; const putRequest = store.put(customer); putRequest.onsuccess = function() { console.log('Customer updated successfully'); }; putRequest.onerror = function() { console.error('Error updating customer'); }; } else { console.log('Customer not found'); } }; request.onerror = function() { console.error('Error getting customer'); }; }
删除数据库中的数据
要从数据库中删除数据,你可以使用delete
方法。
function deleteCustomer(id) { const transaction = db.transaction(['customers'], 'readwrite'); const store = transaction.objectStore('customers'); const request = store.delete(id); request.onsuccess = function() { console.log('Customer deleted successfully'); }; request.onerror = function() { console.error('Error deleting customer'); }; }
关闭数据库连接
当你完成所有操作后,应该关闭数据库连接以释放资源。
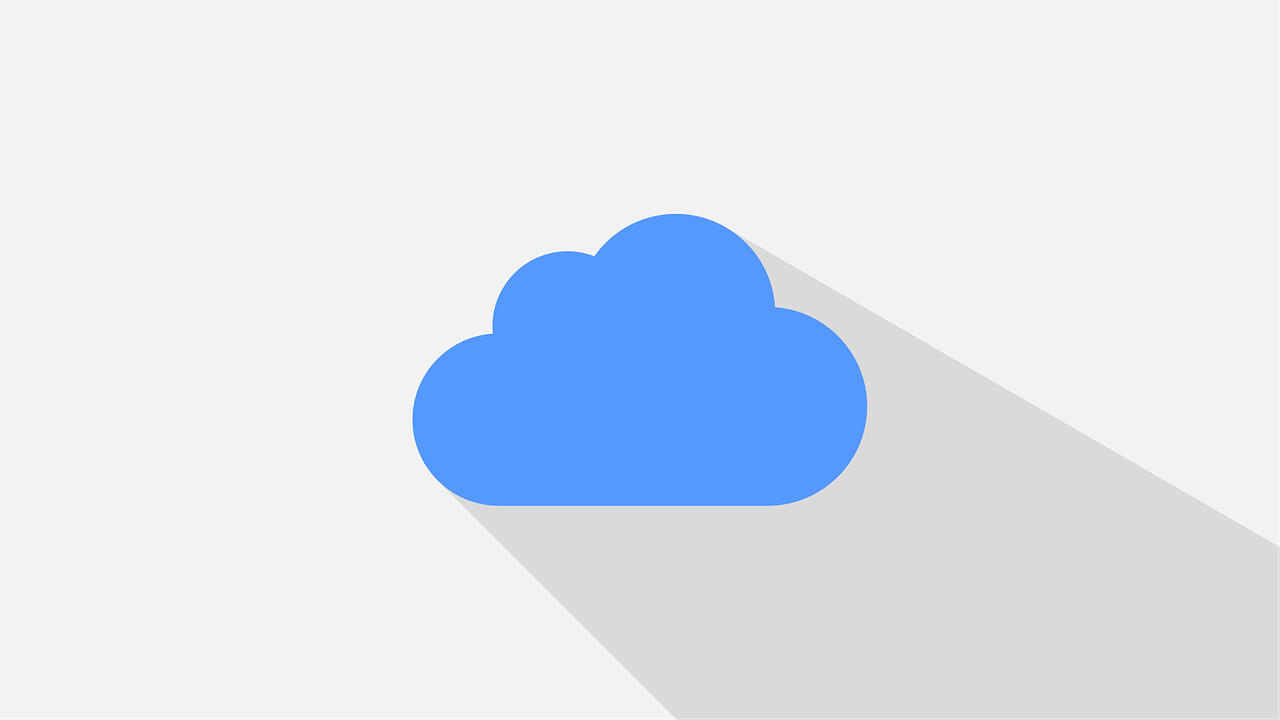
function closeDatabase() { if (db) { db.close(); console.log('Database connection closed'); } }
是在Chrome中使用IndexedDB创建和管理数据库的基本步骤,通过这些步骤,你可以在用户的浏览器中存储大量的结构化数据,而无需依赖服务器端数据库,这对于离线应用程序或需要快速访问数据的应用非常有用。
到此,以上就是小编对于“chrome 创建数据库”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1417247.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复