在Web开发中,checkbox
(复选框)是一种常见的表单元素,用于让用户选择多个选项,使用JavaScript可以增强checkbox
的功能,例如动态添加或删除选项、检查所有选项的状态等,本文将详细介绍如何使用JavaScript操作checkbox
,并提供相关的代码示例和常见问题解答。
基本操作
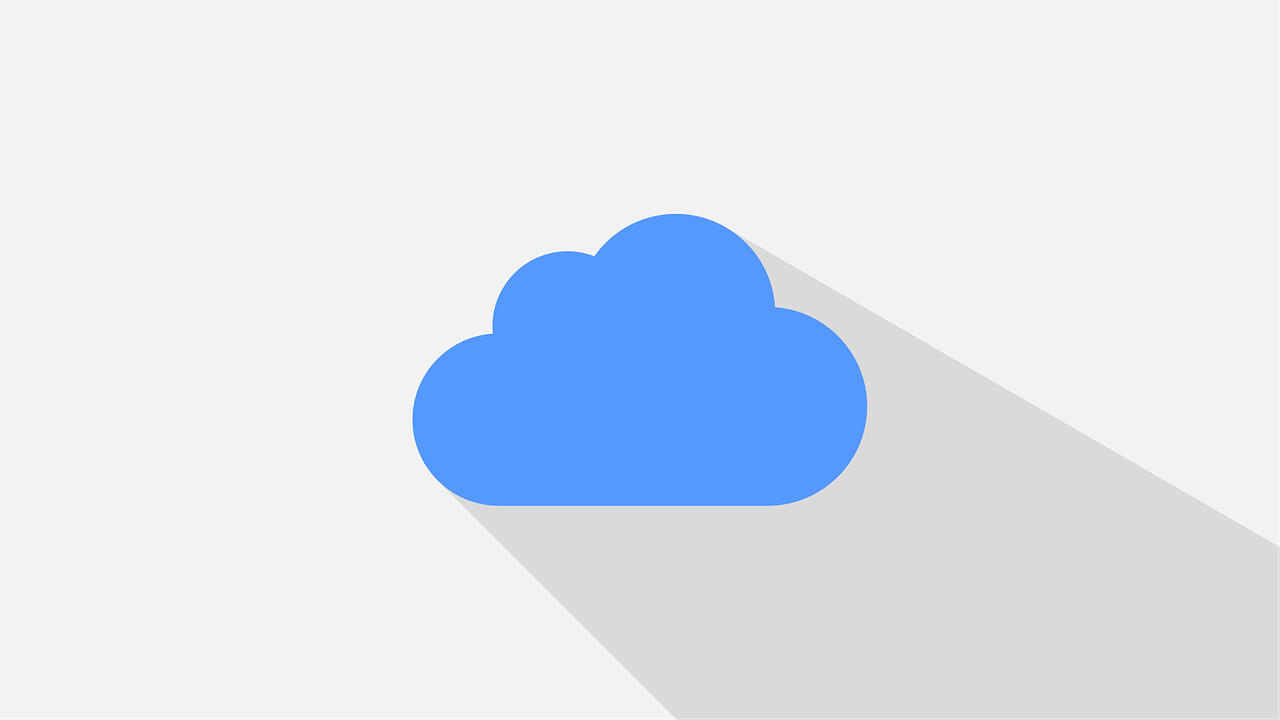
1.1 创建和删除Checkbox
使用JavaScript可以动态地创建和删除checkbox
,以下是一个示例:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Checkbox Example</title> </head> <body> <div id="checkbox-container"></div> <button onclick="addCheckbox()">Add Checkbox</button> <button onclick="removeCheckbox()">Remove Checkbox</button> <script> function addCheckbox() { const container = document.getElementById('checkbox-container'); const checkbox = document.createElement('input'); checkbox.type = 'checkbox'; checkbox.id = 'checkbox-' + (container.children.length + 1); checkbox.name = 'example'; container.appendChild(checkbox); container.appendChild(document.createTextNode(' Checkbox ' + (container.children.length + 1))); } function removeCheckbox() { const container = document.getElementById('checkbox-container'); if (container.lastChild) { container.removeChild(container.lastChild); } } </script> </body> </html>
在这个示例中,点击“Add Checkbox”按钮会在页面上添加一个新的checkbox
,点击“Remove Checkbox”按钮会删除最后一个checkbox
。
1.2 获取Checkbox状态
可以使用JavaScript获取checkbox
的选中状态,以下是一个示例:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Checkbox Status</title> </head> <body> <input type="checkbox" id="myCheckbox"> Click me <button onclick="checkStatus()">Check Status</button> <p id="status"></p> <script> function checkStatus() { const checkbox = document.getElementById('myCheckbox'); const status = checkbox.checked ? 'Checked' : 'Unchecked'; document.getElementById('status').innerText = 'Checkbox is ' + status; } </script> </body> </html>
在这个示例中,点击“Check Status”按钮会在页面上显示当前checkbox
的状态。
高级操作
2.1 全选/取消全选
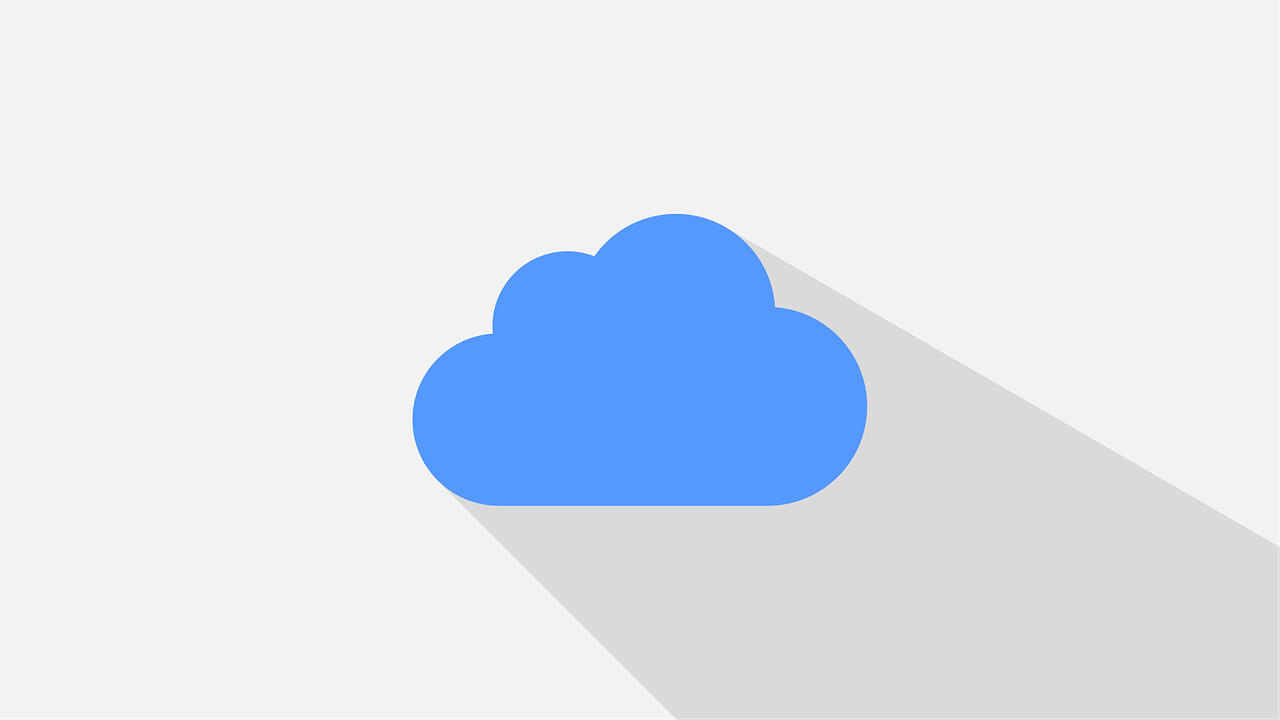
有时需要提供一个“全选”或“取消全选”的功能,可以使用JavaScript来实现,以下是一个示例:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Select All Checkboxes</title> </head> <body> <input type="checkbox" id="selectAll"> Select All<br><br> <input type="checkbox" class="item"> Item 1<br> <input type="checkbox" class="item"> Item 2<br> <input type="checkbox" class="item"> Item 3<br> <input type="checkbox" class="item"> Item 4<br> <script> const selectAll = document.getElementById('selectAll'); const items = document.querySelectorAll('.item'); selectAll.addEventListener('change', () => { items.forEach(item => { item.checked = selectAll.checked; }); }); items.forEach(item => { item.addEventListener('change', () => { if (!item.checked) { selectAll.checked = false; } else { const allChecked = [...items].every(i => i.checked); selectAll.checked = allChecked; } }); }); </script> </body> </html>
在这个示例中,当用户点击“Select All”复选框时,所有的item
复选框都会相应地被选中或取消选中,如果所有的item
复选框都被选中,则“Select All”复选框也会被选中;否则,它会被取消选中。
2.2 动态更新Checkbox列表
有时需要根据用户的输入或其他条件动态更新checkbox
列表,以下是一个示例:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Dynamic Checkbox List</title> </head> <body> <input type="text" id="newItem" placeholder="Enter new item"> <button onclick="addNewItem()">Add Item</button> <div id="checkbox-list"></div> <script> function addNewItem() { const newItemValue = document.getElementById('newItem').value.trim(); if (newItemValue) { const list = document.getElementById('checkbox-list'); const checkbox = document.createElement('input'); checkbox.type = 'checkbox'; checkbox.id = 'checkbox-' + list.children.length; checkbox.name = 'dynamicItems'; checkbox.value = newItemValue; list.appendChild(checkbox); list.appendChild(document.createTextNode(' ' + newItemValue)); list.appendChild(document.createElement('br')); document.getElementById('newItem').value = ''; // Clear input field } else { alert('Please enter a valid item name.'); } } </script> </body> </html>
在这个示例中,用户可以在文本框中输入新项的名称,然后点击“Add Item”按钮将其添加到checkbox
列表中。
常见问题解答 (FAQs)
3.1 如何禁用或启用所有Checkbox?
要禁用或启用页面上的所有checkbox
,可以使用以下JavaScript代码:
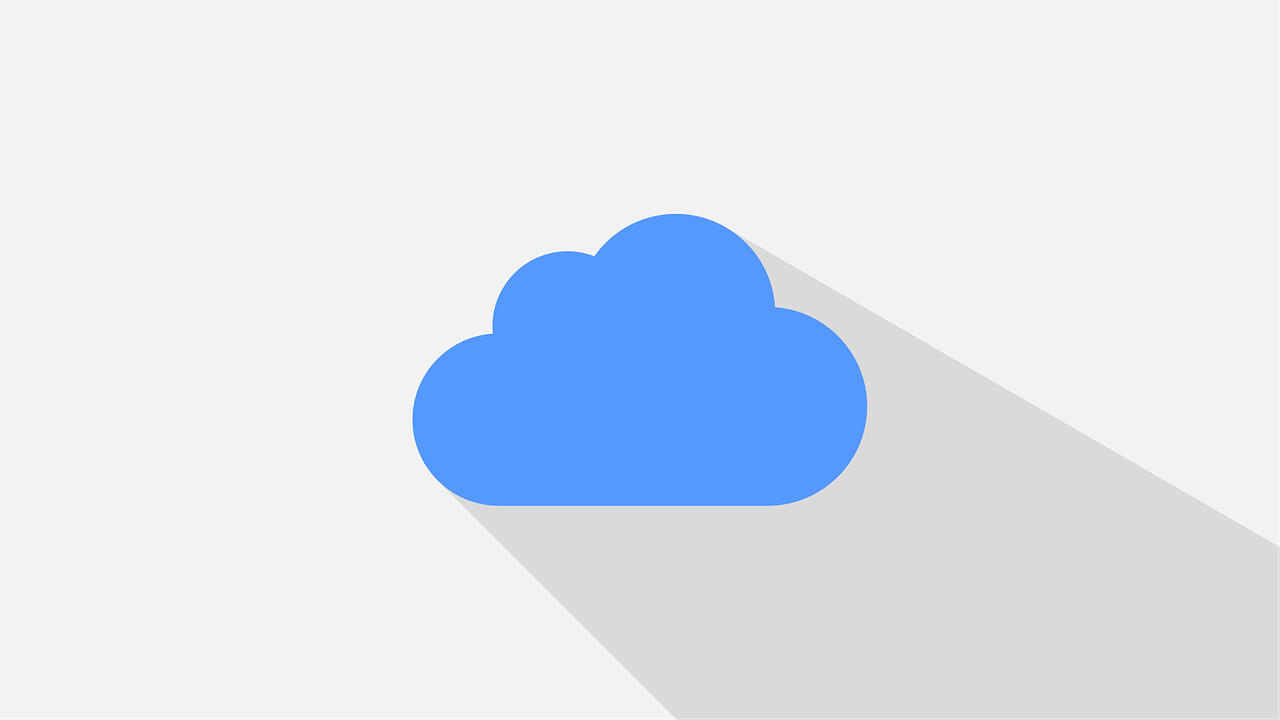
function disableAllCheckboxes() { const checkboxes = document.querySelectorAll('input[type="checkbox"]'); checkboxes.forEach(checkbox => { checkbox.disabled = true; }); } function enableAllCheckboxes() { const checkboxes = document.querySelectorAll('input[type="checkbox"]'); checkboxes.forEach(checkbox => { checkbox.disabled = false; }); }
调用disableAllCheckboxes()
函数可以禁用所有checkbox
,调用enableAllCheckboxes()
函数可以启用所有checkbox
。
3.2 如何获取所有选中的Checkbox的值?
要获取所有选中的checkbox
的值,可以使用以下JavaScript代码:
function getSelectedCheckboxValues() { const checkboxes = document.querySelectorAll('input[type="checkbox"]:checked'); const values = []; checkboxes.forEach(checkbox => { values.push(checkbox.value); }); return values; }
这个函数会返回一个包含所有选中的checkbox
值的数组。
到此,以上就是小编对于“checkboxjs传递”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1415358.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复