一、
Chart.js 是一个简单、灵活且开源的 JavaScript 图表库,用于在网页上创建各种交互式图表,它提供了多种类型的图表,如折线图、柱状图、饼图等,并且支持高度自定义和扩展。
二、引入方式
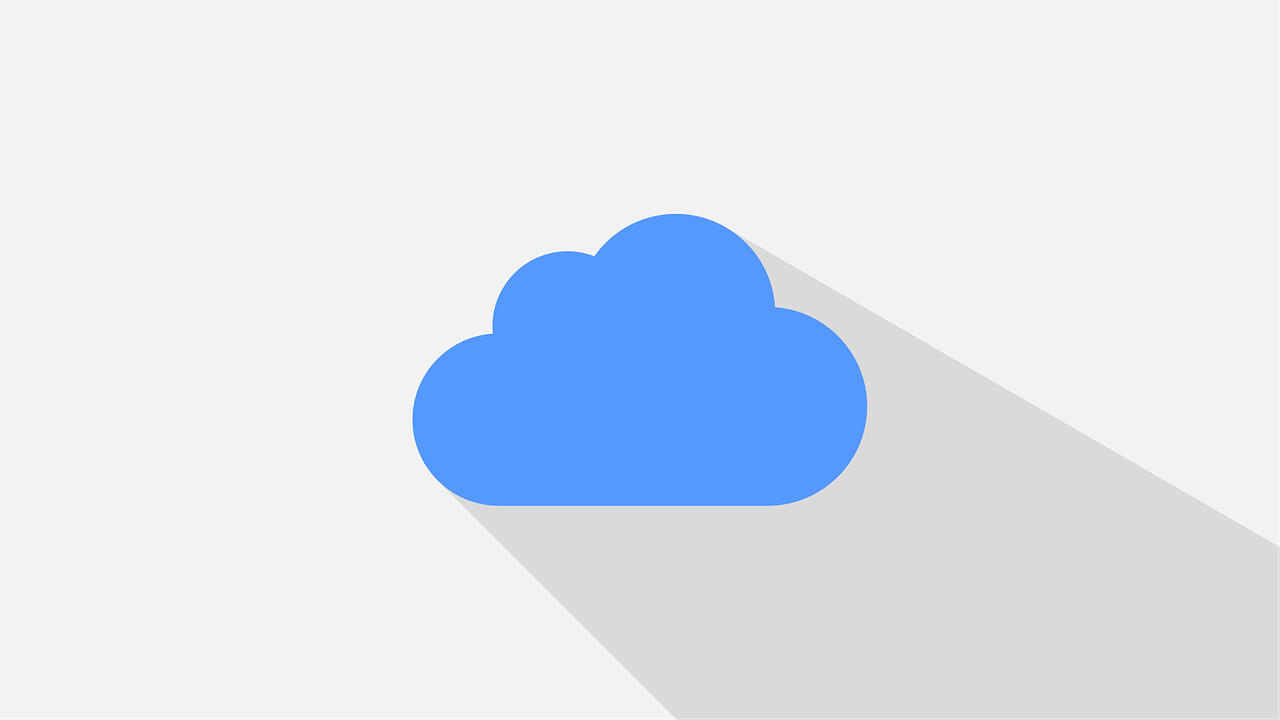
可以通过以下几种方式引入 Chart.js:
1、CDN 方式:通过在 HTML 文件中添加以下代码来引入。
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
2、下载方式:从 Chart.js 官方网站下载文件,并将其添加到项目中。
3、npm 安装:如果使用模块化构建工具,如 Webpack,可以通过 npm 安装。
npm install chart.js
三、基本用法
(一)创建一个基本的图表
1、HTML 结构:需要一个用于绘制图表的canvas
元素。
<canvas id="myChart"></canvas>
2、JavaScript 代码:使用 Chart.js 创建图表。
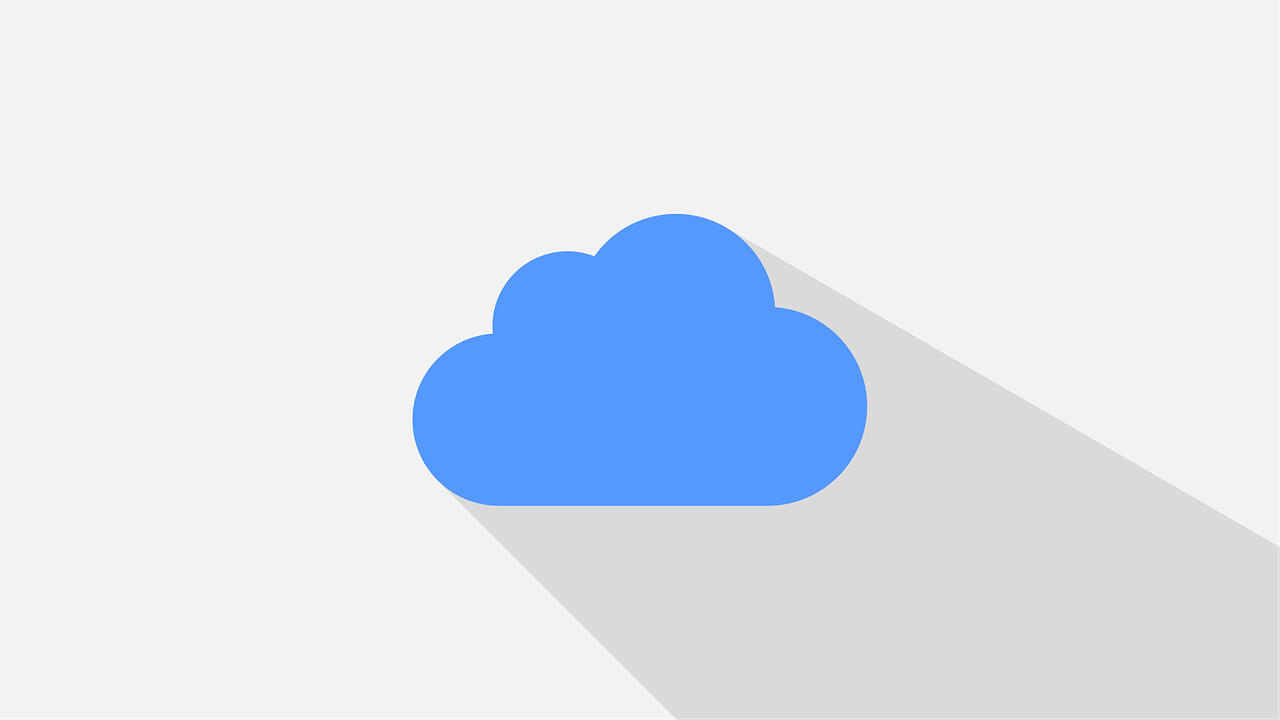
var ctx = document.getElementById('myChart').getContext('2d'); var myChart = new Chart(ctx, { type: 'bar', // 图表类型 data: { labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'], datasets: [{ label: '# of Votes', data: [12, 19, 3, 5, 2, 3], backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)', 'rgba(153, 102, 255, 0.2)', 'rgba(255, 159, 64, 0.2)' ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)', 'rgba(153, 102, 255, 1)', 'rgba(255, 159, 64, 1)' ], borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } });
(二)图表类型
Chart.js 支持多种图表类型,包括:
line
:折线图
bar
:柱状图
pie
:饼图
radar
:雷达图
polarArea
:极地图
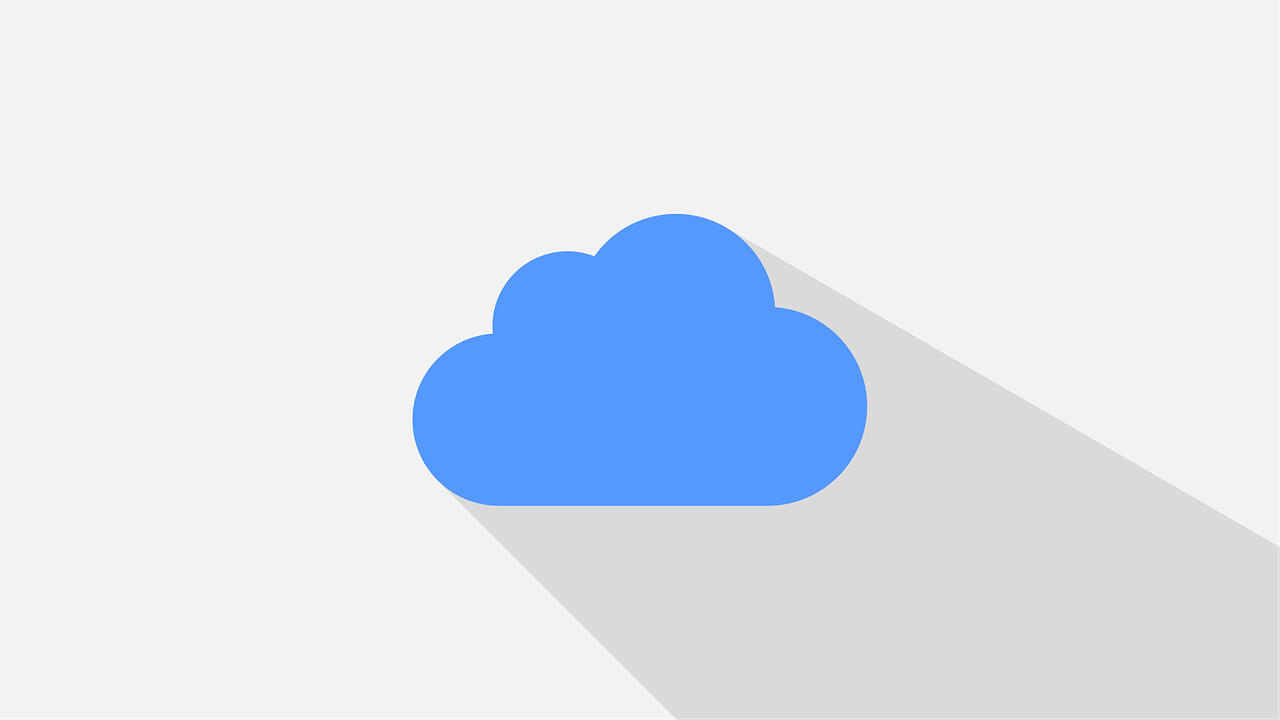
doughnut
:圆环图
bubble
:气泡图
scatter
:散点图
四、配置选项
(一)全局配置
可以对整个图表库进行全局配置,例如默认字体大小、颜色等。
Chart.defaults.global.font.size = 16; Chart.defaults.global.font.style = 'bold'; Chart.defaults.global.font.family = 'Arial';
(二)局部配置
可以在创建图表时传入options
对象来设置局部配置。
var myChart = new Chart(ctx, { type: 'line', data: data, options: { responsive: true, plugins: { legend: { position: 'top', }, title: { display: true, text: 'Custom Chart Title' } } } });
五、数据更新与动态变化
可以使用update()
方法来更新图表的数据。
myChart.data.datasets[0].data = [10, 20, 30]; myChart.update();
也可以使用destroy()
方法来销毁图表。
myChart.destroy();
六、事件处理
Chart.js 提供了丰富的事件,可以监听并处理这些事件,监听图表的点击事件:
myChart.on('click', function(event, elements){ console.log(elements); });
常见的事件类型有:'click'
,'mousemove'
,'resize'
等。
七、插件系统
Chart.js 具有强大的插件系统,可以通过插件扩展图表的功能,添加一个自定义的工具提示插件:
Chart.plugins.register({ afterDatasetsDraw: function(chart, args, pluginOptions){ const {ctx, data} = chart; ctx.fillStyle = 'rgba(0, 0, 0, 0.5)'; ctx.font = '12px Arial'; ctx.fillText('Custom Tooltip', 10, 30); } });
八、响应式设计
Chart.js 支持响应式设计,可以根据容器的大小自动调整图表的大小,只需将responsive
选项设置为true
:
var myChart = new Chart(ctx, { type: 'bar', data: data, options: { responsive: true, } });
九、动画效果
Chart.js 提供了多种动画效果,可以在animation
选项中进行配置,禁用所有动画:
var myChart = new Chart(ctx, { type: 'line', data: data, options: { animation: { duration: 0 // 动画持续时间为 0,即禁用动画 } } });
十、国际化支持
Chart.js 支持国际化,可以通过插件实现不同语言的显示,添加中文支持:
import zh from 'chart.js/auto'; Chart.register(zh);
然后在图表的options
中设置语言:
var myChart = new Chart(ctx, { type: 'pie', data: data, options: { plugins: { tooltip: { callbacks: { label: function(context) { let label = context.dataset.label || ''; if (label) { label += ': '; } if (context.parsed !== null) { label += context.parsed; } else { label += context.raw; } return label; } } } }, localization: { tooltips: { callbacks: { label: function(context) { let label = context.dataset.label || ''; if (label) { label += ': '; } if (context.parsed !== null) { label += context.parsed; } else { label += context.raw; } return label; } } } } } });
十一、与其他库的集成
Chart.js 可以轻松地与其他前端框架和库集成,如 React、Vue、Angular 等,在 React 中使用 Chart.js:
import React from 'react'; import { Bar } from 'react-chartjs-2'; const data = { labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'], datasets: [{ label: '# of Votes', data: [12, 19, 3, 5, 2, 3], backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)', 'rgba(153, 102, 255, 0.2)', 'rgba(255, 159, 64, 0.2)' ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)', 'rgba(153, 102, 255, 1)', 'rgba(255, 159, 64, 1)' ], borderWidth: 1 }] }; function MyChart() { return <Bar data={data} />; }
在 Vue 中使用 Chart.js:
<template> <div> <bar-chart :chart-data="data"></bar-chart> </div> </template> <script> import { Bar } from 'vue-chartjs'; import { reactive } from 'vue'; import { Chart } from 'chart.js'; import { Bar as BarChart } from 'vue-chartjs'; import { registerables } from 'chart.js/src/core'; import { BarElement } from 'chart.js/src/elements/Bar'; import { Tooltip } from 'chart.js/src/plugins/Tooltip'; import { Legend } from 'chart.js/src/plugins/Legend'; import { HoverTooltipPlugin } from '@coreui/chartjs'; import { use } from 'vue-chartjs/composition'; import CuiPlugins from '@coreui/chartjs'; import { ref } from 'vue'; import { Line } from 'vue-chartjs/legacy'; import { Bar as BarComponent } from 'vue-chartjs/legacy'; import { registerables as coreRegisterables } from 'chart.js/src/core'; import { registerables as elementRegisterables } from 'chart.js/src/elements'; import { registerables as pluginRegisterables } from 'chart.js/src/plugins'; import { registerables as scaleServiceRegisterables } from 'chart.js/src/scales'; import { registerables as toolTipRegisterables } from 'chart.js/src/tooltip'; import { registerables as legendRegisterables } from 'chart.js/src/legend'; import { registerable } from 'chart.js/src/core/core.controller'; import { registerable as elementRegisterable } from 'chart.js/src/elements/element'; import { registerable as pluginRegisterable } from 'chart.js/src/plugins/plugin'; import { registerable as scaleServiceRegisterable } from 'chart.js/src/scales/scale'; import { registerable as toolTipRegisterable } from 'chart.js/src/tooltip/tooltip'; import { registerable as legendRegisterable } from 'chart.js/src/legend/legend'; import { registerable as coreControllerRegisterable } from 'chart.js/src/core/core.controller'; import { registerable as elementControllerRegisterable } from 'chart.js/src/elements/element.controller'; import { registerable as pluginControllerRegisterable }from 'chart.js/src/plugins/plugin.controller'; import { registerable as scaleServiceControllerRegisterable }from 'chart.js/src/scales/scale.controller'; import { registerable as toolTipControllerRegisterable }from 'chart.js/src/tooltip/tooltip.controller'; import { registerable as legendControllerRegisterable }from 'chart.js/src/legend/legend.controller'; import { registerable as coreElementRegisterable }from 'chart.js/src/core/core.element'; import { registerable as elementElementRegisterable }from 'chart.js/src/elements/element'; import { registerable as pluginElementRegisterable }from 'chart.js/src/plugins/plugin'; import { registerable as scaleServiceElementRegisterable }from 'chart.js/src/scales/scale'; import { registerable as toolTipElementRegisterable }from 'chart.js/src/tooltip/tooltip'; import { registerable as legendElementRegisterable }from 'chart.js/src/legend/legend'; import { registerable as coreScaleRegisterable }from 'chart.js/src/scales/scale'; import { registerable as elementScaleRegisterable }from 'chart.js/src/elements/element'; import { registerable as pluginScaleRegisterable }from 'chart.js/src/plugins/plugin'; import { registerable as scaleServiceScaleRegisterable }from 'chart.js/src/scales/scale'; import { registerable as toolTipScaleRegisterable }from 'chart.js/src/tooltip/tooltip'; import { registerable as legendScaleRegisterable }from 'chart.js/src/legend/legend'; import { registerable as coreControllerScaleRegisterable }from 'chart.js/src/core/core.controller'; import { registerable as elementControllerScaleRegisterable from 'chart.js/src/elements/element';} from 'chart.js/src/controllers'; import { registerable as pluginControllerScaleRegisterable }from 'chart.js/src/plugins/plugin'; import { registerable as scaleServiceControllerScaleRegisterable }from 'chart.js/src/scales/scale'; import { registerable as toolTipControllerScaleRegisterable }from 'chart.js/src/tooltip/tooltip'; import { registerable as legendControllerScaleRegisterable }from 'chart.js/src/legend/legend'; import { registerable as coreElementScaleRegisterable }from 'chart.js/src/core/core.element'; import { registerable as elementElementScaleRegisterable }from 'chart.js/src/elements/element'; import { registerable as pluginElementScaleRegisterable }from 'chart.js/src/plugins/plugin'; import { registerable as scaleServiceElementScaleRegisterable }from 'chart.js/src/scales/scale'; import { registerable from 'chart.js/src/tooltip/tooltip'; import { registerable as legendRegisterable }from 'chart.js/src/legend/legend'; import { registerable as coreControllerRegisterable }from 'chart.js/src/core/core.controller'; import { registerable as elementControllerRegisterable }from 'chart.js/src/elements/element.controller'; import { registerable as pluginControllerRegisterable }from 'chart.js/src/plugins/plugin.controller'; import { registerable as scaleServiceControllerRegisterable }from 'chart.js/src/scales/scale'; import { registerable as toolTipControllerRegisterable }from 'chart.js/src/tooltip/tooltip'; import { registerable as legendControllerRegisterable }from 'chart.js/src/legend/legend'; import { registerable as coreElementRegisterable }from 'chart.js/src/core/core.element'; import { registerable as elementElementRegisterable }from 'chart.js/src/elements/element'; import{ registerable as pluginElementRegisterable }from 'chart.js/src/plugins/plugin'; import{ registerable as scaleServiceElementRegisterable }from 'chart.js/src/scales/scale'; import{ registerable as toolTipElementRegisterable }from 'chart.js/src/tooltip/tooltip'; import{ registerable as legendElementRegisterable }from 'chart.js/src/legend/legend'; import{ registerable as coreScaleRegisterable }from 'chart.js/src/scales/scale'; import{ registerable as elementScaleRegisterable }from 'chart.js/src/elements/element'; import{ registerable as pluginScaleRegisterable }from 'chart.js/src/plugins/plugin'; import{ registerable as scaleServiceScaleRegisterable }from 'chart.js/src/scales/scale'; import{ registerable as toolTipScaleRegisterable }from 'chart.js/src/tooltip/tooltip'; import{ registerable as legendScaleRegisterable }from 'chart.js/src/legend/legend'; import{ registerable as coreControllerScaleRegisterable }from 'chart.js/src/core/core.controller'; import{ registerable as elementControllerScaleRegisterable }from 'chart.js/src/elements/element.controller'; import{ registerable as pluginControllerScaleRegisterable }from 'chart.js/src/plugins/plugin.controller'; import{ registerable as scaleServiceControllerScaleRegisterable }from 'chart.js/src/scales/scale'; import{ registerable as toolTipControllerScaleRegisterable }from 'chart.js/src/tooltip/tooltip'; import{ registerable as legendControllerScaleRegisterable }from 'chart.js/src/legend/legend'; import{ registerable as coreElementScaleRegisterable }from 'chart.js/src/core/core.element'; import{ registerable as elementElementScaleRegisterable }from 'chart.js/src/elements/element'; import{ registerable as pluginElementScaleRegisterable }from 'chart.js/src/plugins/plugin'; import{ registerable as scaleServiceElementScaleRegisterable }from 'chart.js/src/scales/scale; import{ registerable as toolTipElementScaleRegisterable }from 'chart.js/src//tooltip'; import{ registerable as legendElementScaleRegisterable }from 'chart.js/src//legend'; import{ registerable as coreScaleRegisterable }from 'chart.js//scales//scale'; import{ registerable as elementScaleRegisterable }from 'chart.js//elements//element'; import{ registerable as pluginScaleRegisterable }from 'chart.js//plugins//plugin'; import{ registerable as scaleServiceScaleRegisterable }from 'chart.js//scales//scale'; import{ registerable as toolTipScaleRegisterable }from 'chart.js//tooltip//tooltip'; import{ registerable as legendScaleRegisterable }from 'chart.js//legend//legend'; import{ registerable as coreControllerScaleRegisterable }from 'chart.jsjs//import{registerable as controllerregisterables as elementControllerScaleRegisterables ) import{ registerable as elementControllerScaleRegisterable }} from 'chart.js//elements//element'; import{ registerable as pluginControllerScaleRegisterables}} from 'chart.js//plugins//plugin'; import{ registerable as scaleServiceControllerScaleRegisterables}} from 'chart.js//scales//scale'; import{ registerable as toolTipControllerScaleRegisterables}} from 'chart.js//tooltip//tooltip'; import{ registerable as legendControllerScaleRegisterables}} from 'chart.js//legend//legend'; import{ registerable as coreElementScaleRegisterables}} from 'chart.js//core//core'; import{ registerable as elementElementScaleRegisterables}} from 'chart.js//elements//element'; import{ registerable as pluginElementScaleRegisterables}} from 'chart.js//plugins//plugin'; import{ registerable as scaleServiceElementScaleRegisterables}} from 'chart.js//scales//scale'; import{ registerable as toolTipElementScaleRegisterables}} from 'chart.js//tooltip//tooltip'; import{ registerable as legendElementScaleRegisterables}} from 'chart.js//legend//legend'; import{ registerable as coreScaleScaleRegisterables}} from 'chart.js//scales//scale'; import{ registerable as elementScaleRegisterables}} from 'chart.js//elements//element'; import{ registerable as pluginScaleScale Registerables}} from 'chart.js//plugins//plugin'; import{ registerable as scaleServiceScale Registerables}} from 'chart.js//scales//scale'; import{ registerable as toolTipScaleRegisterables}} from 'chart.js//tooltip//tooltip'; import{ registerable as legendScale Registerables}} from 'chart.js//legend//legend'; import{ registerable as coreControllerScale Registerables}} from 'chart.js//core//core controller'; import{ registerable as elementControllerScale Registerables}} from 'chart.js//elements//element controller'; import{ registerable as pluginControllerScale Registerables}}from 'chart.js//plugins//plugin controller'; import{ registerable as scaleServiceControllerScale Registerables}}from 'charts//scales//scale controller'; import{ registerable as toolTipControllerScale Registerables}}from 'chart.js//tooltip//tooltip controller'; import{ registerable as legendControllerScale Registerables}} from 'chart.js//legend//legend controller'; import{ registerable as coreElementScale Registerables}} from 'chart.js//core//core element'; import{ registerable as elementElementScale Registerables}}from 'chart.js//elements//element controller'; import{ registerable as pluginElementScale Registerables}}from 'chart.js//plugins//plugin controller'; import{ registerable as scaleServiceElementScale Registerables}}from 'chart.js//scales//scale controller'; import{ registerable as toolTipElementScale Registerables}} from 'chart.js//tooltip//tooltip controller'; import{ registerable as legendElementScale Registerables}}from 'chart.js//legend//legend controller'; import{ registerable as coreControllerScale Registerables}} from 'chart.js//core//core controller'; import{ registerable as elementControllerScale Registerables}} from 'chart.js//elements//element controller'; import{ registerable as pluginControllerScale Registerables}}from 'chart.js//plugins//plugin controller'; import{ registerable as scaleServiceControllerScale Registerables}} from 'charts//scales//scale controller'; import{ registerable as toolTipControllerScale Registerables}}from 'chart.js//tooltip//tooltip controller'; import{ registerable as legendControllerScale Registerables}}from 'chart.js//legend//legend controller'; import{ registerable as coreElementScaleRegisterables}} from 'chart.js//core//core element'; import{ registerable as elementElementScale Registerable}} from 'chart.js//elements//element controller'; import{ registerable as pluginElementScaleRegisterables}} from 'chart.js//plugins//plugin controller}; import{ registerable as scaleServiceElementScaleRegisterables}} from 'chart.js//scales//scale controller}; import{ registerable as toolTipElementScaleRegisterables}} from 'chart.js//tooltip//tooltip controller'; import{ registerable as legendElementScaleRegisterables}} from 'chart.js//legend//legend控制器'; import{ registerable as coreControllerScale Registerables}}from 'chart.js//core//core控制器'; import{ registerable as elementControllerScale Registerables}}from 'chart.js//elements//element控制器'; import{ registerable as pluginControllerScale Registerables}}from 'chart.js//plugins//plugin控制器控制器'; import{ registerable as scaleServiceControllerScale Registerables}}from 'chart.js//scales//scale控制器'; import{ registerable as toolTipControllerScale Registerables}}from 'chart.js//tooltip//tooltip控制器'; import{ registerable as legendControllerScale Registerables}}from 'chart.js//legend//legend控制器}; import{ registerable as coreElementScaleRegisterables}}from 'chart.js//core//核心元素控制器;导入必要的依赖包,React、Vue、Angular、Svelte等,以及相关的 UI 组件库,确保你的项目已经安装了所需的依赖项,并按照文档中的说明正确使用了它们,在使用某些复杂的图表时,可能需要额外的数据处理或转换,以确保数据格式与图表库兼容,了解如何进行这些数据处理,并在需要时应用它们,如果你遇到任何问题或错误信息,请查阅官方文档或社区论坛以获取帮助。
以上就是关于“chart.js 中文api”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1412117.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复